In C++, "extends" is not a keyword; however, inheritance can be achieved using the `:` operator to create a derived class from a base class, enabling code reuse and polymorphism.
Here's a simple example of inheritance in C++:
#include <iostream>
using namespace std;
class Base {
public:
void display() {
cout << "Base class display method." << endl;
}
};
class Derived : public Base { // 'Derived' class extends 'Base' class
public:
void show() {
cout << "Derived class show method." << endl;
}
};
int main() {
Derived obj;
obj.display(); // Accessing base class method
obj.show(); // Accessing derived class method
return 0;
}
Understanding Class Extension in C++
What is Class Extension?
Class extension in C++ is a fundamental concept that allows a new class, known as a derived class, to inherit properties and behaviors (methods) from an existing class, referred to as the base class. This process enhances code reusability and enforces a modular design, thereby making the codebase easier to manage and maintain.
Benefits of Extending Classes
Extending classes come with several advantages:
- Encapsulation: It helps in bundling data and methods together while hiding the internal state from the outside world. This enhances security and maintainability.
- Code reuse: Instead of rewriting common functionalities, derived classes can use or override methods from their base classes, facilitating code reuse.
- Improved organization and readability: Code becomes more organized as related functionalities are grouped, improving overall readability for developers.
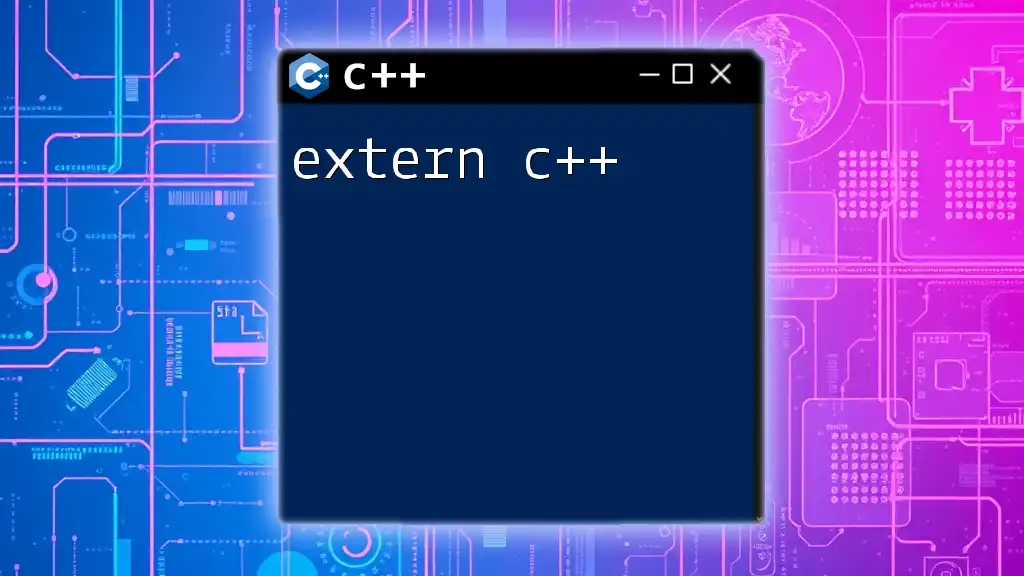
C++ Class Extends: The Basics
The Syntax of Class Extension in C++
To extend a class in C++, you use the following syntax:
class Derived : access_specifier Base {
// Derived class members
};
In this syntax, `Derived` is the new class, `Base` is the class being extended, and `access_specifier` can be one of `public`, `protected`, or `private`. The access specifier dictates the visibility of the base class's members in the derived class.
Example Code Snippet
class Base {
public:
void display() {
std::cout << "Base Class" << std::endl;
}
};
class Derived : public Base {
public:
void show() {
std::cout << "Derived Class" << std::endl;
}
};
Explanation of the Code
In the example above, the `Derived` class extends the `Base` class. The derived class can call any public methods from the `Base` class, such as the `display()` method, which can be used to show its functionality. This extension allows the `Derived` class to incorporate the properties and methods of the `Base` class without having to duplicate code.
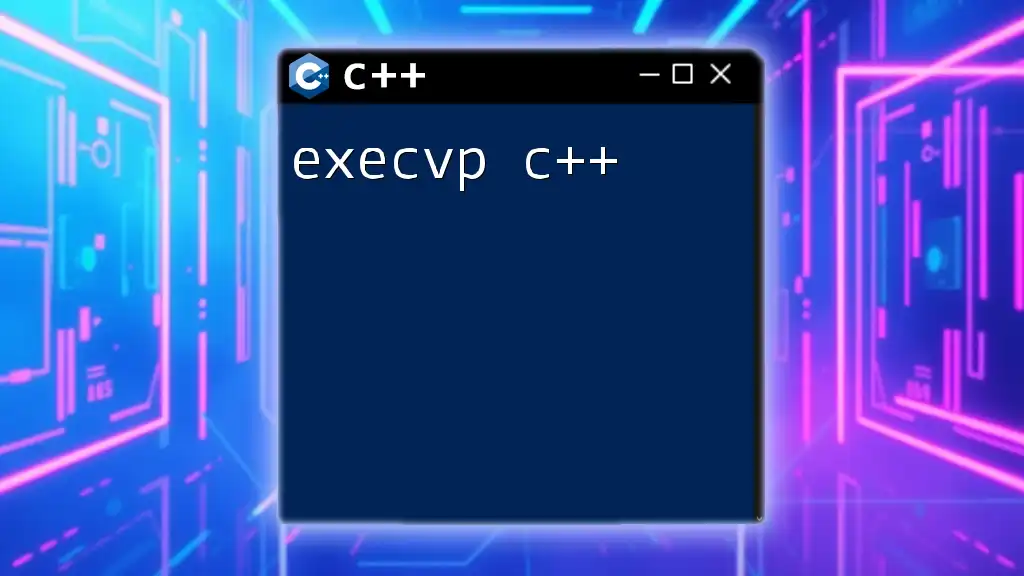
C++ Extend Class: Access Specifiers
Understanding Access Specifiers
Access specifiers play a crucial role in encapsulation and visibility in class inheritance:
- Public members are accessible from any function or object.
- Private members are accessible only within the class itself, including its constructors and destructors.
- Protected members share accessibility with both the class and its derived classes, but not with other classes or functions.
Example Code Snippet
class Base {
private:
int privateVar;
protected:
int protectedVar;
public:
int publicVar;
Base() : privateVar(1), protectedVar(2), publicVar(3) {}
};
class Derived : public Base {
public:
void display() {
// std::cout << privateVar; // Error: private member
std::cout << protectedVar << std::endl; // Accessible
std::cout << publicVar << std::endl; // Accessible
}
};
Explanation of Member Accessibility
In this example, while `privateVar` from the `Base` class is inaccessible in the `Derived` class (leading to a compile-time error if uncommented), `protectedVar` and `publicVar` are accessible. This showcases how access specifiers control accessibility in inheritance.
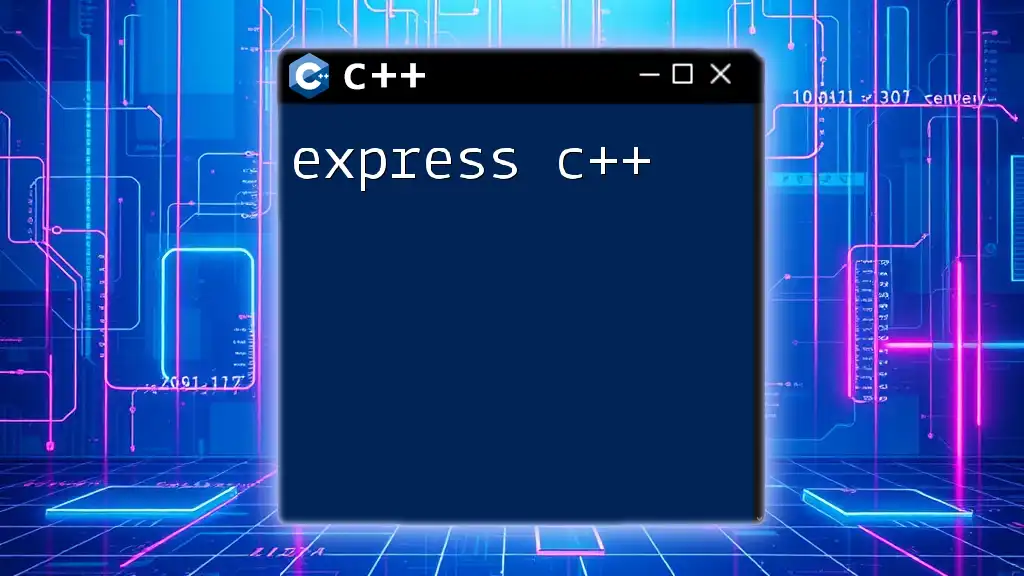
C++ Class Extension: Virtual Functions
What are Virtual Functions?
Virtual functions allow us to achieve polymorphism in C++. When a function in a base class is declared as virtual, it enables derived classes to override it, allowing for dynamic binding at runtime. This is particularly useful for programming environments where the exact class of an object may not be known until runtime.
Example Code Snippet
class Base {
public:
virtual void show() {
std::cout << "Base Class Show" << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived Class Show" << std::endl;
}
};
Explanation of Virtual Functions
In the code snippet, the `show()` function in the `Base` class is declared as virtual, allowing the `Derived` class to provide its own implementation. The use of the `override` keyword emphasizes the intent to override a virtual function, providing clearer code and better error-checking during compilation. This setup allows for the correct method to be called depending on the object’s type at runtime.
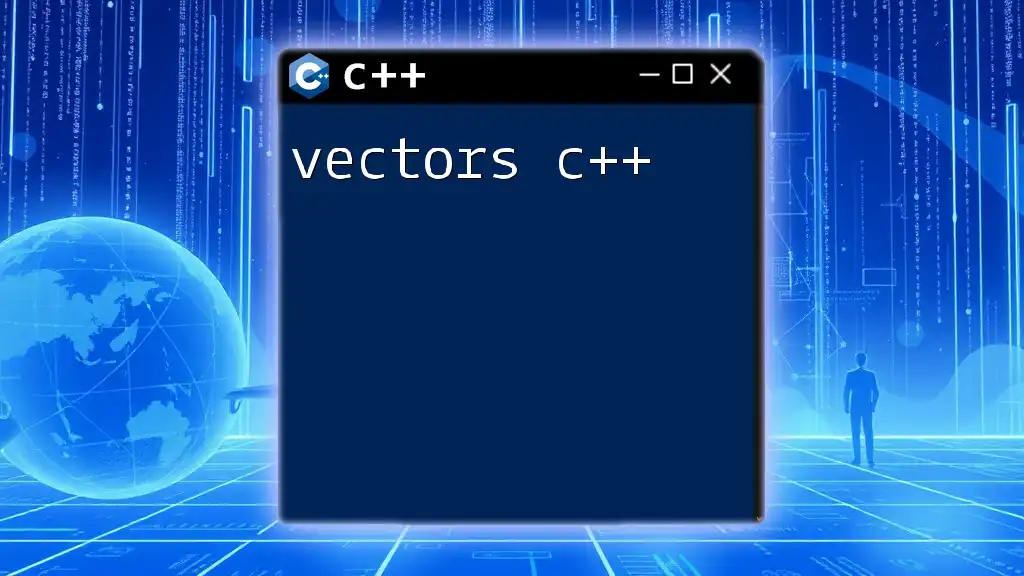
C++ Extend Class: Constructors and Destructors
Understanding Constructors and Destructors in Inheritance
When creating an object of a derived class, both the base class and derived class constructors are triggered. This ensures that the base class part of the object is initialized before the derived class.
Example Code Snippet
class Base {
public:
Base() { std::cout << "Base Constructor" << std::endl; }
~Base() { std::cout << "Base Destructor" << std::endl; }
};
class Derived : public Base {
public:
Derived() { std::cout << "Derived Constructor" << std::endl; }
~Derived() { std::cout << "Derived Destructor" << std::endl; }
};
Explanation of Constructor and Destructor Behavior
In this scenario, the output will follow this sequence when an object of `Derived` is created:
Base Constructor
Derived Constructor
Upon destruction, the destructors are called in the reverse order:
Derived Destructor
Base Destructor
This behavior reinforces the idea that a derived class’s existence relies on a correctly initialized base class.
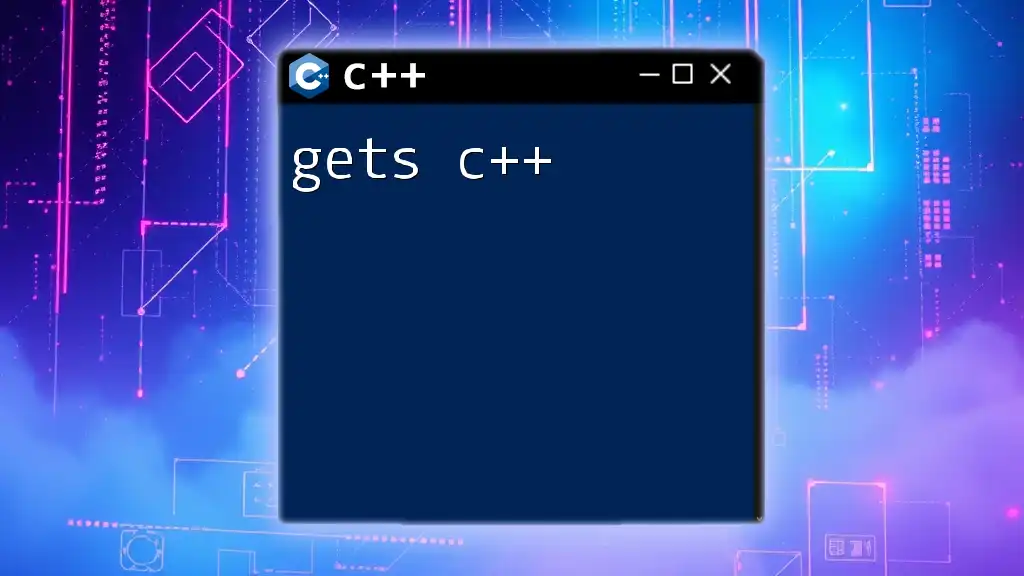
C++ Class Extension: Multiple Inheritance
What is Multiple Inheritance?
Multiple inheritance allows a C++ class to inherit from more than one base class. This can lead to richer class functionalities, but comes with challenges that need careful navigation.
Example Code Snippet
class Base1 {
public:
void showBase1() {
std::cout << "Base1" << std::endl;
}
};
class Base2 {
public:
void showBase2() {
std::cout << "Base2" << std::endl;
}
};
class Derived : public Base1, public Base2 {
public:
void showDerived() {
std::cout << "Derived" << std::endl;
}
};
Explanation of Multiple Inheritance
In the code above, the `Derived` class inherits from both `Base1` and `Base2`. This flexibility allows the `Derived` class to use methods from both base classes, increasing its functionality without code duplication. However, care must be taken to ensure there are no ambiguities when accessing members of the base classes.
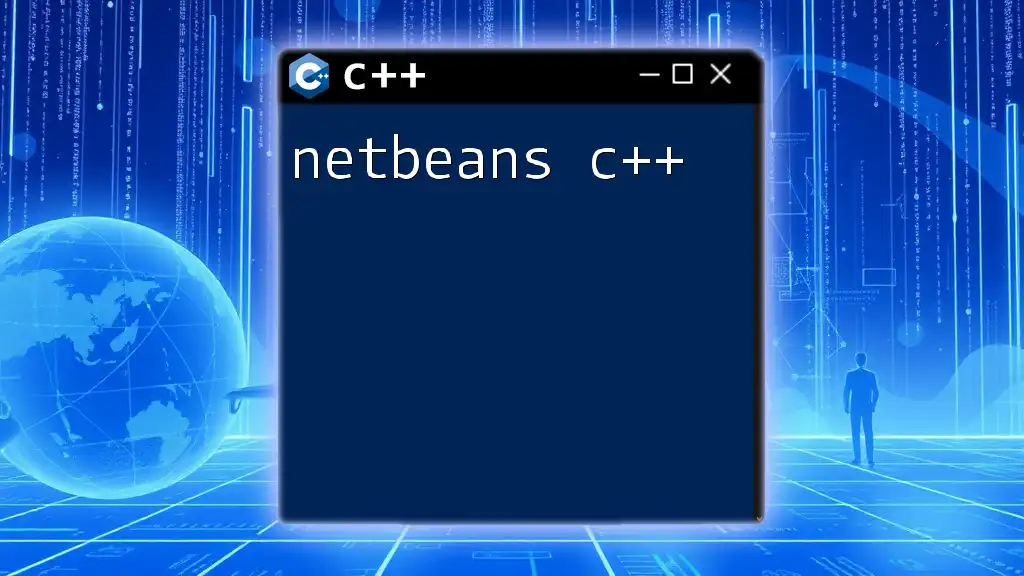
Best Practices for Extending Classes in C++
Choosing Between Composition and Inheritance
While inheritance is a powerful tool, it's essential to consider whether it’s the best design choice. Composition, where classes are designed to use other classes as components, can provide greater flexibility and lower coupling between classes. Always weigh the advantages of increased functionality through inheritance against the risks of creating tight coupling and complex hierarchies.
Avoiding Diamond Problems
A common issue with multiple inheritance is the diamond problem, which can occur when a derived class inherits from two base classes that share a common base. This can lead to ambiguity in method calls. Using virtual inheritance can help alleviate these issues by ensuring that the derived class only contains one instance of the common base class.
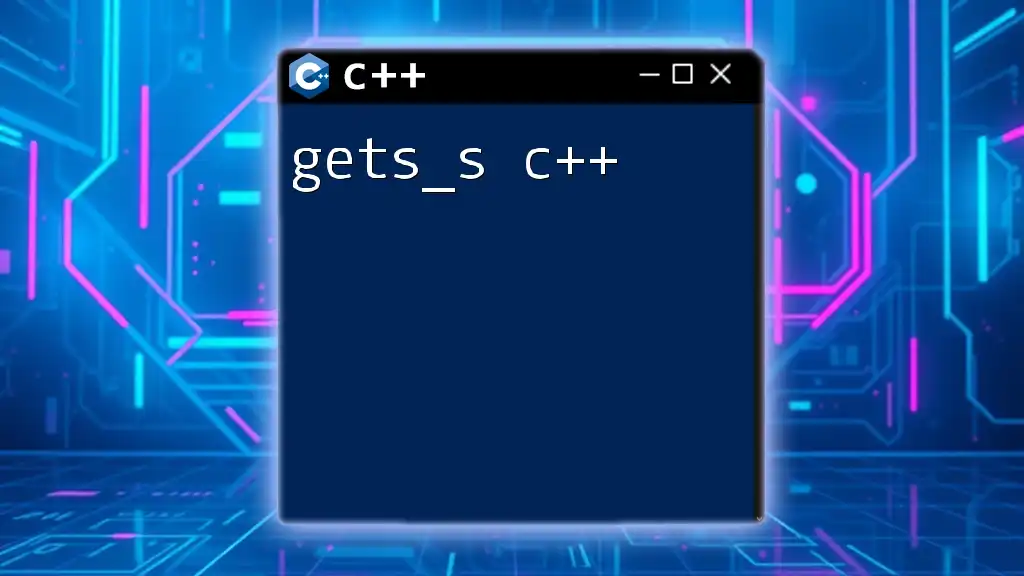
Conclusion
Extending classes in C++ is a powerful mechanism that fosters code reusability, organization, and flexibility. By understanding how to effectively extend classes, utilize access specifiers, implement virtual functions, manage constructors and destructors, and navigate the complexities of multiple inheritance, developers can leverage the full potential of object-oriented programming.
Call to Action
Practice the concepts covered in this article by implementing class extensions in your projects. Joining programming communities or workshops can also enhance your learning experience and provide you with practical insights into mastering C++.