The `gets_s` function in C++ is a safer version of the `gets` function, which reads a line of input from `stdin` into a buffer while preventing buffer overflows by requiring the buffer size as an argument.
#include <cstdio>
int main() {
char buffer[100];
gets_s(buffer, sizeof(buffer)); // Safe input method
printf("You entered: %s", buffer);
return 0;
}
Understanding the Basics of Input in C++
The Need for Safe Input Functions
In C++ programming, handling input safely is crucial to prevent vulnerabilities such as buffer overflow, which can lead to severe consequences in software applications. Traditional input methods, like `gets`, can cause security issues as they do not check the bounds of the buffer into which they write. In contrast, modern C++ offers several safer alternatives to handle user input more securely.
Introduction to `gets_s`
`gets_s` is a function designed to provide a safer alternative to the traditional `gets` function. It originated from the C11 standard and aims to mitigate the risks associated with uncontrolled buffer access. One of the key aspects of `gets_s` is that it requires the programmer to specify the size of the buffer, thereby preventing overflow and enhancing security.
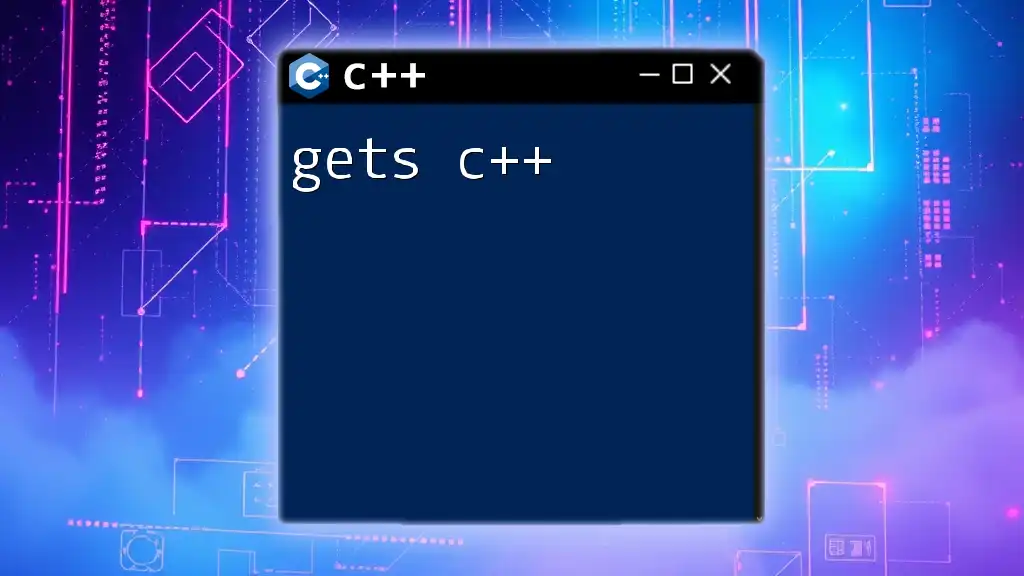
Syntax of `gets_s`
Basic Syntax Structure
The function signature for `gets_s` is as follows:
char* gets_s(char* buffer, size_t size);
Parameters:
- `buffer`: This is the destination array where the input text will be stored.
- `size`: This specifies the total capacity of the buffer, ensuring that the input does not exceed this limit.
Example of Usage
Here’s a practical example demonstrating how to use `gets_s` in a C++ program:
#include <cstdio>
int main() {
char str[50];
if (gets_s(str, sizeof(str))) {
printf("Input: %s\n", str);
} else {
printf("Error reading input\n");
}
return 0;
}
In this code:
- A character array `str` is created with a size of 50.
- The `gets_s` function reads user input into `str`, ensuring that it does not exceed the defined array bounds.
- If the input operation is successful, it prints the input; otherwise, it will display an error message.
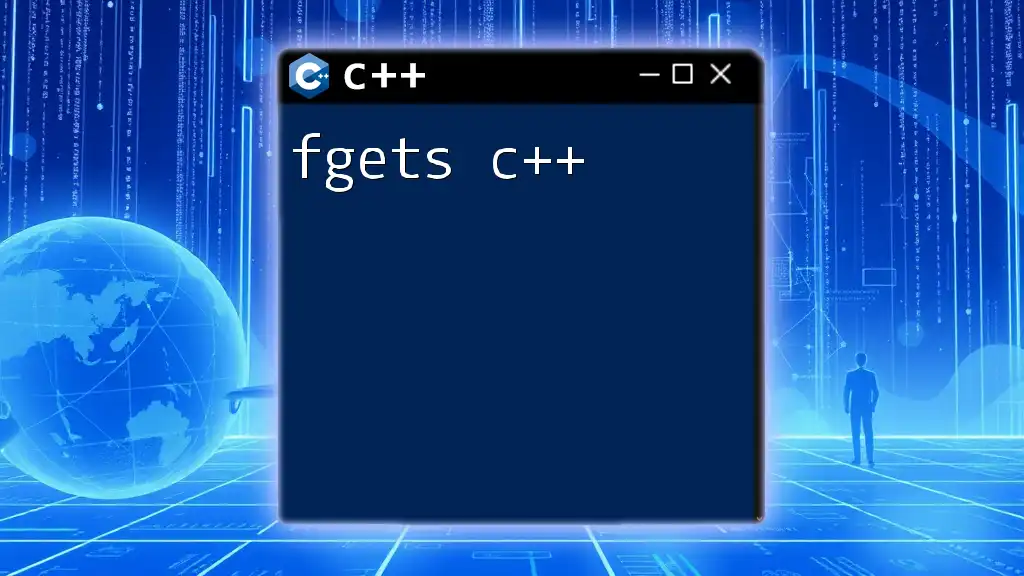
Benefits of Using `gets_s`
Enhanced Security
Using `gets_s` significantly improves the security of input handling in C++ applications. One of its main advantages is the mitigation of buffer overflow attacks. By requiring developers to provide the buffer size, this function restricts how much data is read based on the allocated memory, making overflowing the buffer virtually impossible.
In contrast, using functions like `gets` can lead to serious vulnerabilities because they do not enforce size checks. As a result, malicious users can exploit such weaknesses, leading to potential data breaches or system crashes.
User-Friendly Readability
Another advantage of `gets_s` is its approach to readability and usability. Unlike alternative methods that may require more complex setups, `gets_s` offers a straightforward interface for input handling. This simplicity allows developers to focus more on implementing functionality while maintaining safety.
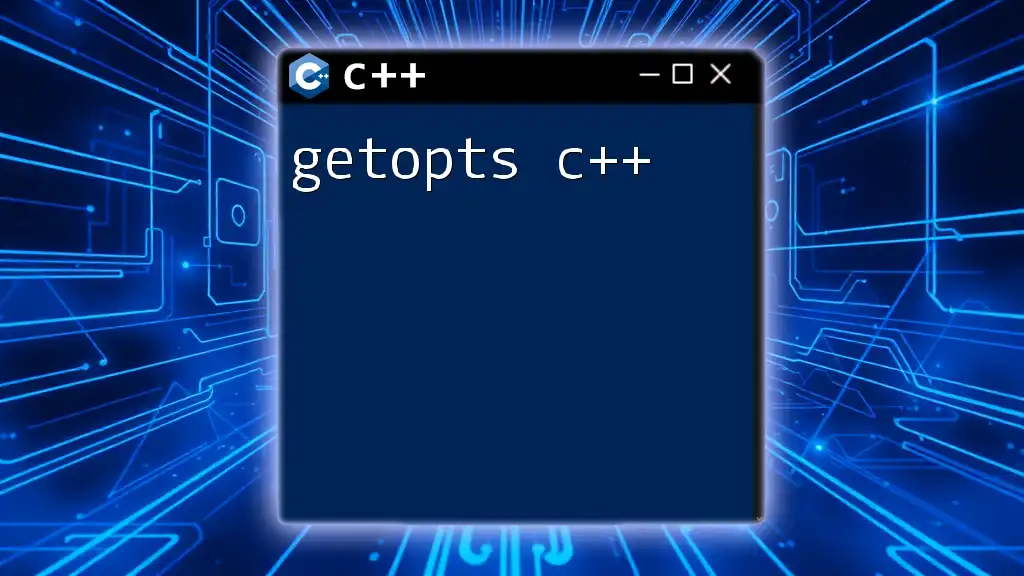
Limitations of `gets_s`
Availability and Compatibility
While `gets_s` is a part of the C11 standard, its widespread usage is limited in practice. Many older compilers and platforms may not support this function, making it less reliable for cross-platform development. Developers need to check compatibility and may need to consider alternative safe input methods in their projects if they require broader support.
Practical Use Cases
Using `gets_s` is ideal in scenarios where security and buffer management are paramount, particularly in applications that handle sensitive data. However, developers should evaluate whether the environment supports it effectively. In cases where `gets_s` is unavailable, exploring its alternatives may yield better compatibility and performance.
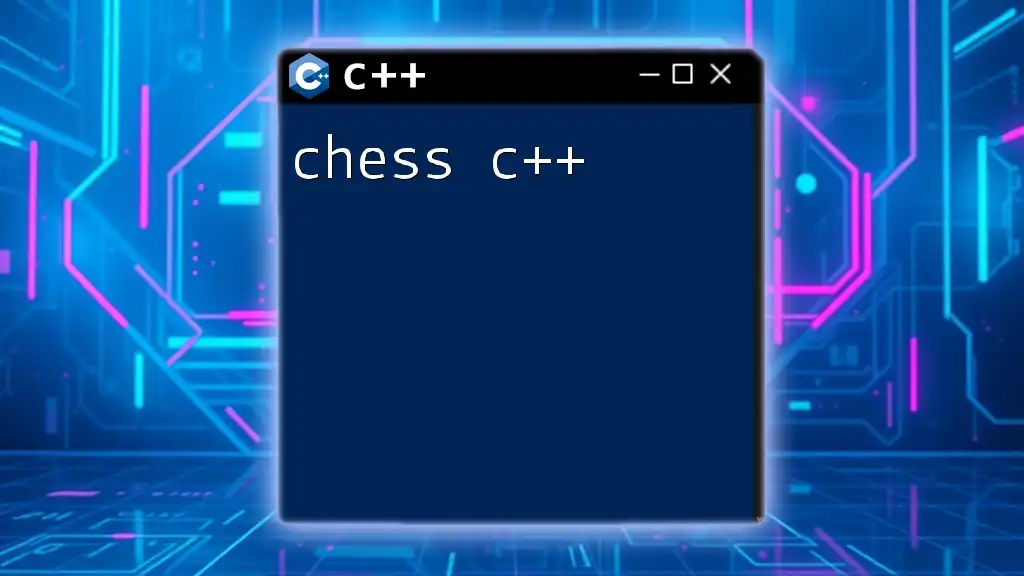
Alternatives to `gets_s`
Overview of Common Input Functions
Several input functions exist in C++ that can also ensure safe input handling. Understanding these alternatives provides developers the flexibility to choose what best suits their needs depending on the project requirements:
-
`cin`: The standard input stream. While convenient, it does not prevent buffer overflow inherently.
-
`getline`: Reads an entire line, allowing input of variable length. It automatically reallocates the needed memory, making it safe.
-
`fgets`: A traditional C function that reads a line from the specified input and allows for bounds checking.
Example Comparisons
Here are code snippets demonstrating how these alternatives work:
Using `cin`:
#include <iostream>
int main() {
std::string input;
std::cin >> input;
std::cout << "Input: " << input << std::endl;
return 0;
}
Using `getline`:
#include <iostream>
#include <string>
int main() {
std::string input;
std::getline(std::cin, input);
std::cout << "Input: " << input << std::endl;
return 0;
}
Using `fgets`:
#include <cstdio>
int main() {
char str[50];
fgets(str, sizeof(str), stdin);
printf("Input: %s\n", str);
return 0;
}
Each of these functions has its benefits and considerations. While `cin` and `getline` are more modern C++ approaches with built-in safety, `fgets` is a tried-and-true C method for tight control over input limits.
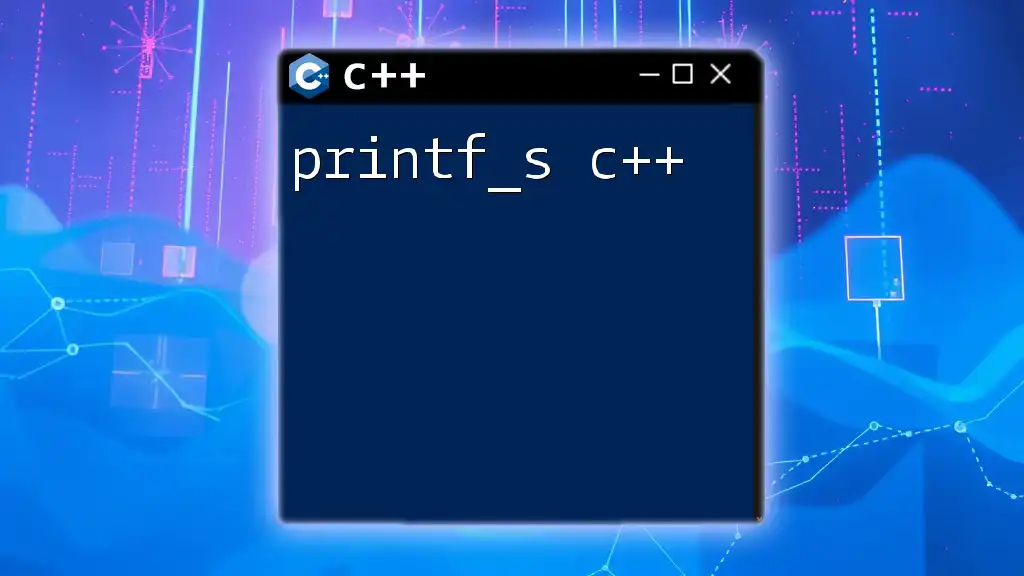
Conclusion
In summary, `gets_s` is an important function in the C++ landscape, offering enhanced security and simplicity for input handling. By understanding its syntax, benefits, and limitations, developers can make informed choices about whether to incorporate it into their applications. As with any programming tool, considering the implications of compatibility and use cases is essential to ensure robust and secure software.
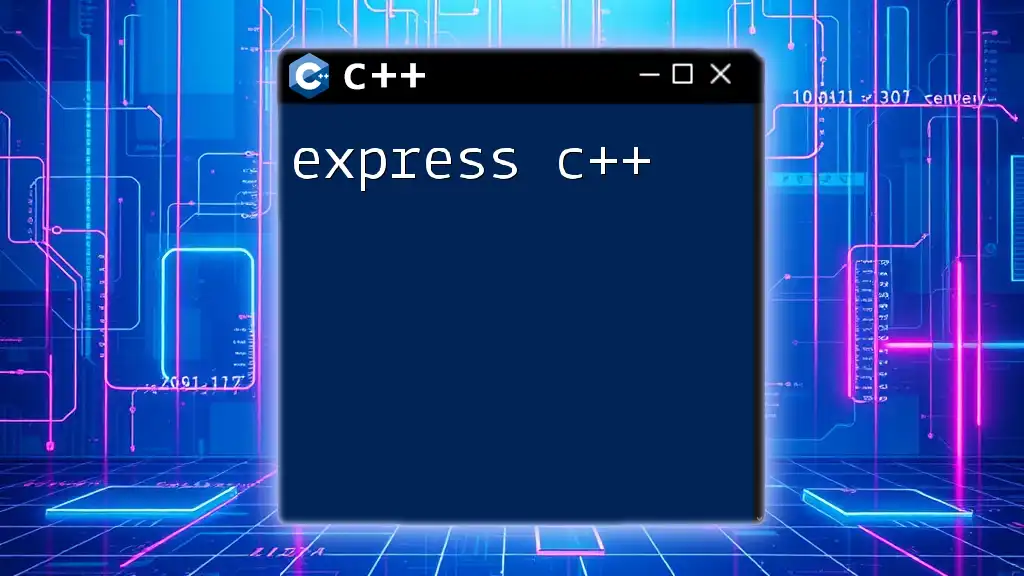
Additional Resources
Recommended Reading
For those eager to dive deeper into input handling in C++, consider exploring additional documentation, tutorials, and books focusing on modern C++ practices and input security.
Community and Forums
Engaging in community forums or exchanges provides avenues for developers to ask questions, share experiences, and gather insights related to C++ programming and input handling techniques.
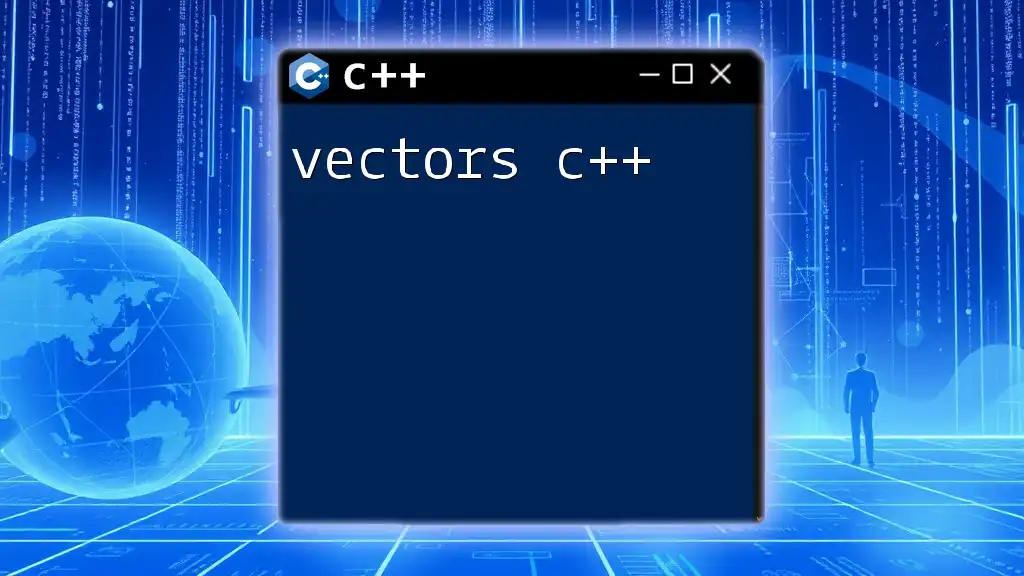
FAQs
Common Questions about `gets_s`
-
Is `gets_s` deprecated?
While it is not officially deprecated, its lack of widespread support means that alternatives are often preferred. -
How does `gets_s` compare to `scanf`?
`scanf` can lead to buffer overflow if not used carefully, while `gets_s` protects against this risk by requiring buffer size. -
What should developers know about cross-platform compatibility?
Not all compilers support `gets_s`, so developers should ensure their environment can effectively utilize this function or consider alternatives for broader compatibility.