Here’s a one-sentence explanation of "ecs c++" along with a code snippet in markdown format:
"ECS (Entity-Component-System) in C++ is a design pattern used for organizing game and simulation codes, enabling better performance and easier management of entities and their behavior."
#include <iostream>
#include <vector>
#include <unordered_map>
class Component {};
class Transform : public Component { /* Position, rotation, etc. */ };
class Render : public Component { /* Rendering data */ };
class Entity {
public:
int id;
std::unordered_map<std::type_index, Component*> components;
template<typename T>
void addComponent(T* component) {
components[typeid(T)] = component;
}
};
int main() {
Entity e;
e.id = 1;
e.addComponent(new Transform());
e.addComponent(new Render());
std::cout << "Entity " << e.id << " created with components." << std::endl;
return 0;
}
What is ECS?
Entity Component System (ECS) is a design pattern used primarily in game development and software architecture that allows for efficient management of complex systems and data. ECS is based on the principle of separating data (components) from behavior (systems), leading to more organized and maintainable code. This architectural pattern is especially valuable when dealing with large-scale game projects. Its main focus is on performance, flexibility, and scalability.
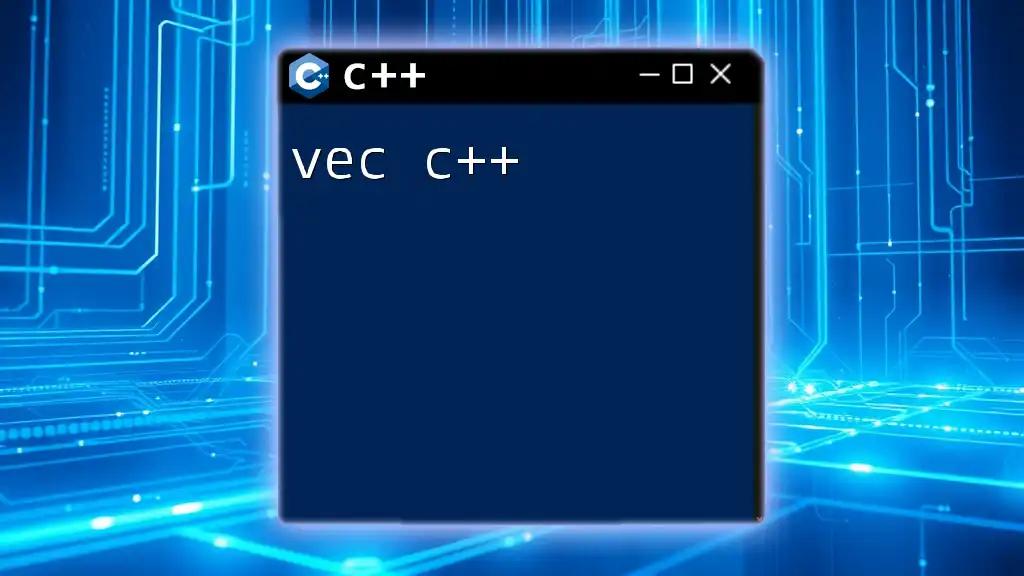
Advantages of Using ECS
ECS offers several significant advantages:
-
Improved Code Organization: By separating entities, components, and systems, developers can maintain a clean and logical structure, simplifying development.
-
Enhanced Performance: ECS leverages data-oriented design, improving cache coherence and minimizing memory access latency. In many scenarios, this design can lead to better performance as systems read from contiguous memory locations.
-
Facilitates Easier Debugging and Maintenance: Because data and behavior are decoupled, this architecture allows developers to test each component or system independently, making debugging more straightforward.
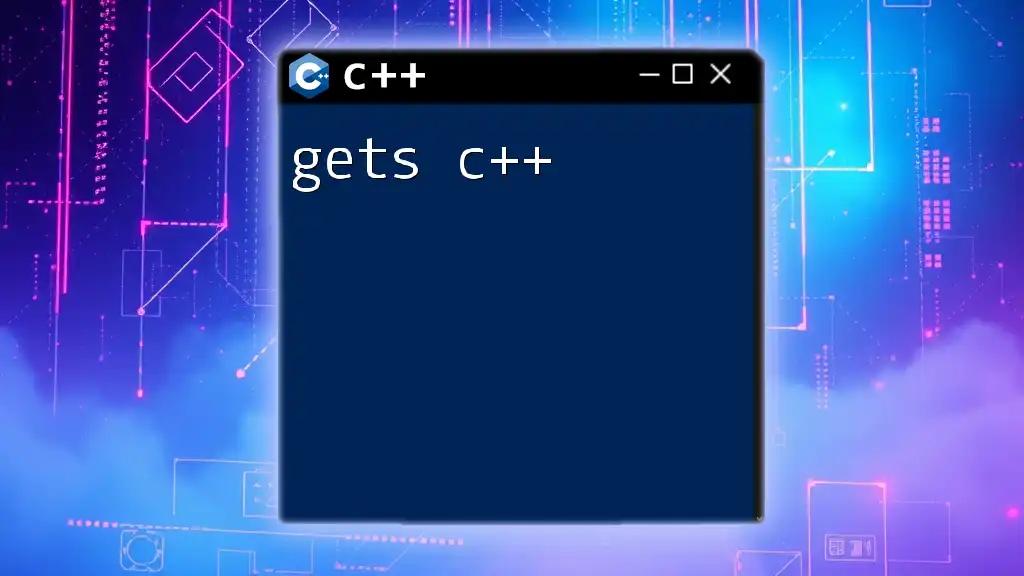
Core Concepts of ECS
Entities
In the ECS paradigm, an entity acts as a unique identifier, typically represented as an integer or a string. An entity is simply an object that represents something in the game world, such as a player, enemy, or item. Importantly, an entity itself does not contain any behavior or data; it is merely a placeholder for associated components.
Components
What Are Components?
Components are lightweight data structures that hold specific attributes of entities. Each component is responsible for a particular aspect of an entity, such as position, velocity, health, etc. By using components, developers can create complex behaviors through combinations of simpler, reusable parts.
Design Patterns for Components
When designing components, consider these best practices:
- Keep components simple: Each component should represent a single responsibility.
- Use composition over inheritance: Favor combining components to achieve behaviors rather than relying heavily on class hierarchies.
For example, consider a `Position` component that defines the location of an entity:
class Position {
public:
float x, y;
};
Systems
Understanding Systems
Systems are responsible for processing entities that possess specific components. A system defines the logic and behavior that should occur based on the components associated with the entities it interacts with.
Interaction Between Systems and Components
Systems usually work in an update loop, processing the relevant components for each entity. For example, consider a movement system that applies physics to entities with both position and velocity components:
class MovementSystem {
public:
void update(EntityManager& entityManager, float deltaTime) {
for (auto& entity : entityManager.getEntitiesWithComponents<Position, Velocity>()) {
auto& position = entityManager.getComponent<Position>(entity);
auto& velocity = entityManager.getComponent<Velocity>(entity);
position.x += velocity.dx * deltaTime;
position.y += velocity.dy * deltaTime;
}
}
};
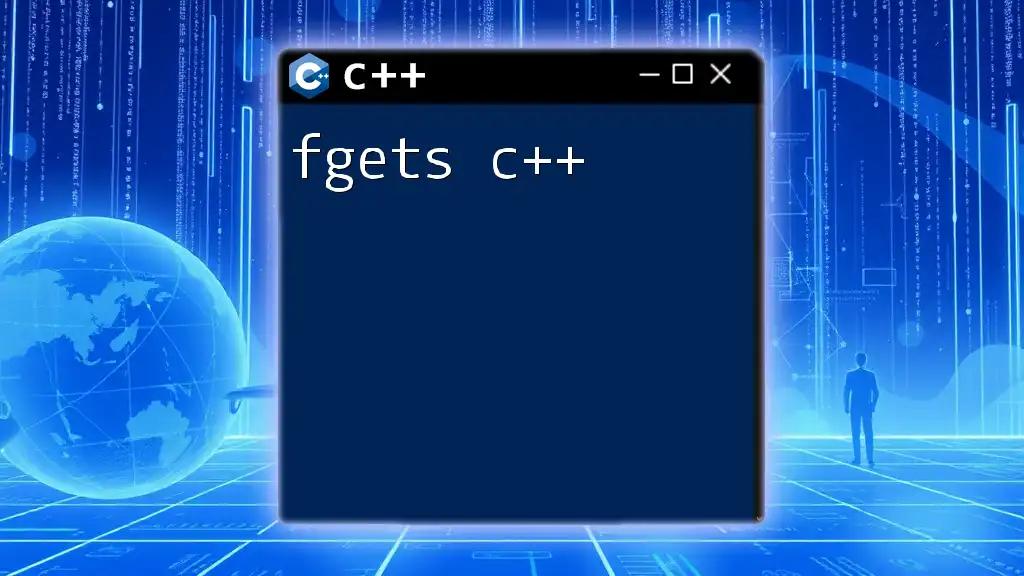
Building an ECS in C++
Setting Up the ECS Framework
Choosing Your Project Structure
A well-organized project structure is crucial for managing your ECS efficiently. A simple directory structure might look like this:
/ecs_project
/src
main.cpp
Entity.h
Component.h
System.h
/include
/lib
/tests
Essential Libraries and Tools
When building an ECS in C++, consider using libraries such as Boost for utility functions or SFML for graphics, input, and audio. Ensure to set up a build system like CMake, which helps manage your project dependencies efficiently.
The C++ ECS Implementation
Defining Entities
You can start by defining an entity class that generates unique IDs:
class Entity {
public:
static int idCounter;
int id;
Entity() : id(idCounter++) {}
};
int Entity::idCounter = 0;
Creating Components
Next, define some components. For instance, let’s create a `Position` component and a `Velocity` component:
class Velocity {
public:
float dx, dy;
};
Implementing Systems
Now, we implement a simple movement system that updates positions based on velocities:
class MovementSystem {
public:
void update(EntityManager& entityManager, float deltaTime) {
for (auto& entity : entityManager.getEntitiesWithComponents<Position, Velocity>()) {
auto& position = entityManager.getComponent<Position>(entity);
auto& velocity = entityManager.getComponent<Velocity>(entity);
position.x += velocity.dx * deltaTime;
position.y += velocity.dy * deltaTime;
}
}
};
Integrating Your ECS
Adding Entities and Components
To utilize your ECS, you need to create entities and assign components. This is typically done in the main function or through another initialization method.
EntityManager entityManager;
Entity player;
entityManager.addComponent(player.id, Position{0.0f, 0.0f});
entityManager.addComponent(player.id, Velocity{1.0f, 0.0f});
Updating Systems
Within your main game loop, call the update method of your systems, passing the time delta:
float deltaTime = 0.016f; // Simulate a frame time of 16ms
MovementSystem movementSystem;
movementSystem.update(entityManager, deltaTime);
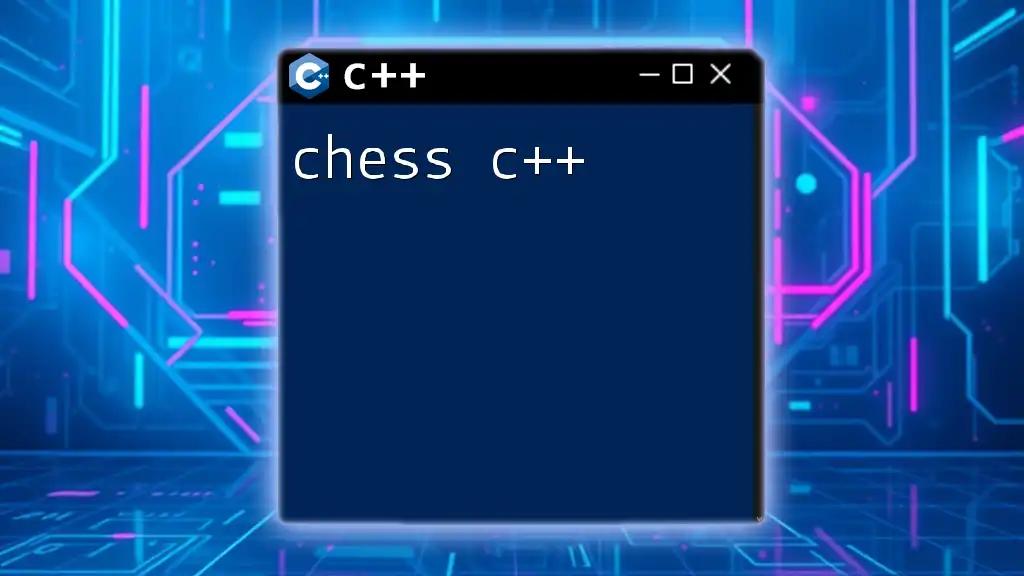
Optimizing Your ECS
Performance Tips for ECS
To optimize your ECS implementation, consider:
-
Minimizing cache misses: Organize your data structures to ensure that components accessed together are stored in contiguous memory. This practice improves memory access patterns and performance.
-
Batch processing components: Process components in bulk where possible to reduce unnecessary iteration and branching, leading to more efficient CPU workload.
Debugging and Testing Your ECS
Effective debugging and testing are crucial for any system. Use tools such as gdb or Valgrind to trace issues within your systems. Make sure to implement unit tests for individual components and systems, ensuring they behave as expected:
void testMovementSystem() {
EntityManager em;
Entity player;
em.addComponent(player.id, Position{0.0f, 0.0f});
em.addComponent(player.id, Velocity{1.0f, 0.0f});
MovementSystem system;
system.update(em, 1.0f); // Simulate 1 second of movement
// Assert expected position
assert(em.getComponent<Position>(player.id).x == 1.0f);
}
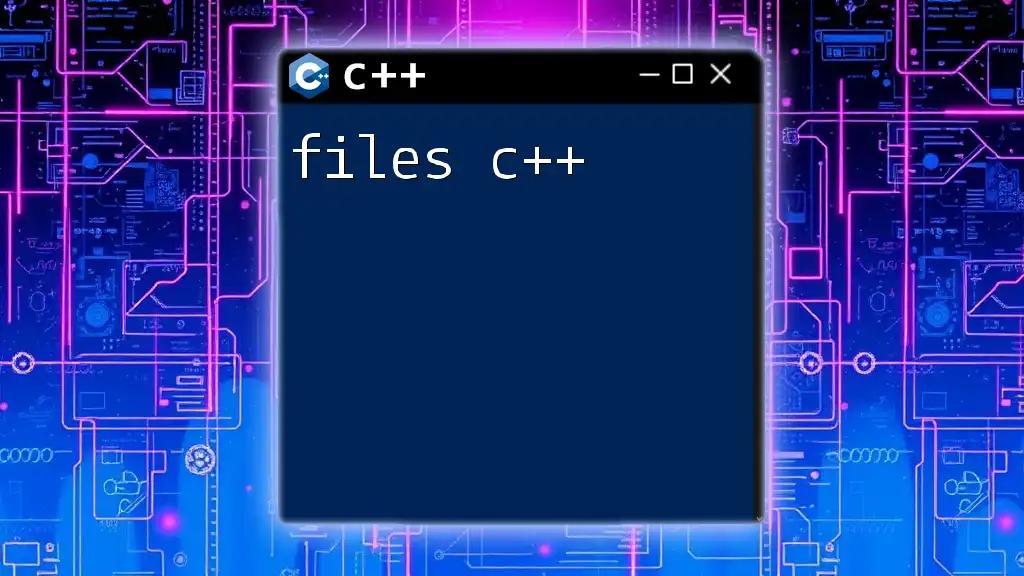
Conclusion
In summary, the ECS pattern in C++ presents a powerful methodology for organizing complex software systems, especially in game development. By embracing data-driven design, developers can enhance their applications' performance and maintainability.
As you explore ECS further, consider experimenting with your implementations and adapting designs to meet the specific needs of your projects. There’s a wealth of knowledge, tutorials, and communities available to support you on this journey.
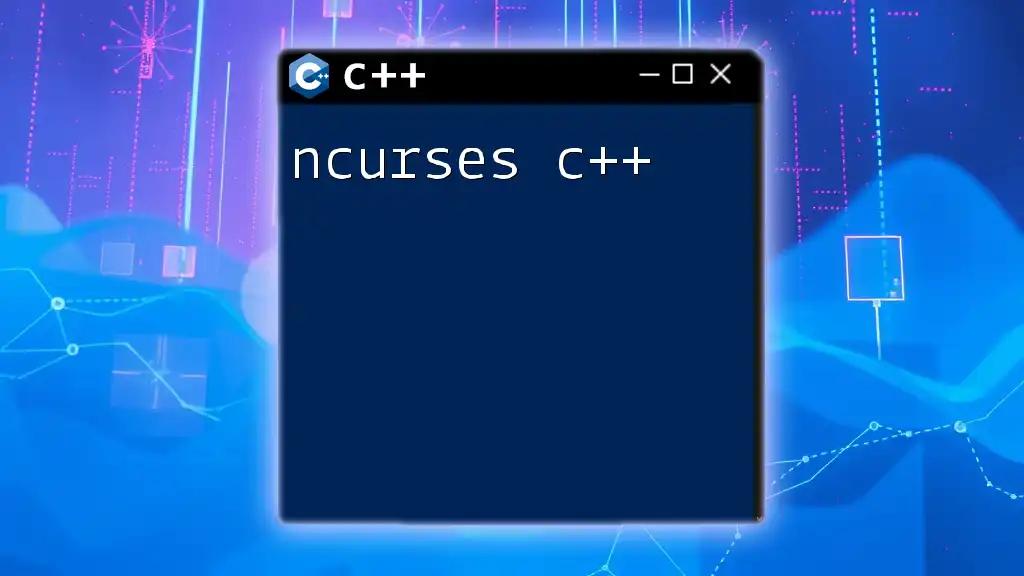
Additional Resources
For further reading, consider books on game development and software architecture, such as "Game Programming Patterns" by Robert Nystrom, and online platforms offering courses on C++ and ECS concepts.
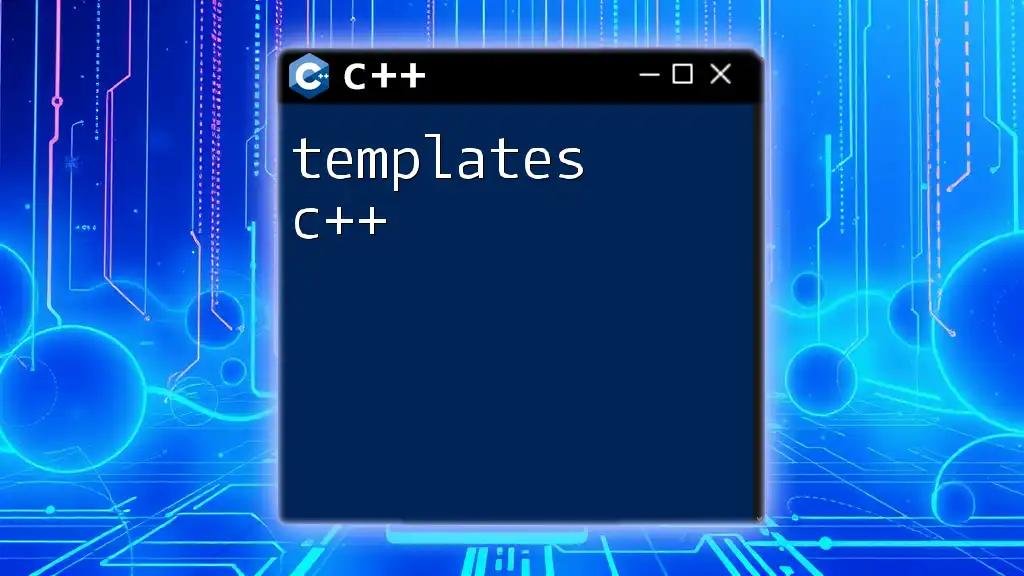
Call to Action
Start implementing ECS in your projects today! Don’t hesitate to reach out to our community or explore our resources for additional support as you embark on mastering ECS in C++.