C++ does follow the principles of PEMDAS (Parentheses, Exponents, Multiplication and Division, Addition and Subtraction) for operator precedence, ensuring that expressions are evaluated in a specific order.
Here's a simple code snippet demonstrating this:
#include <iostream>
int main() {
int result = 5 + 3 * 2; // Multiplication is done before addition
std::cout << "Result: " << result << std::endl; // Outputs: Result: 11
return 0;
}
Understanding PEMDAS in C++
C++ handles operations based on a defined order of execution, which is crucial to ensure that complex expressions yield the correct results. Understanding how C++ adheres to the rules of PEMDAS (Parentheses, Exponents, Multiplication and Division, Addition and Subtraction) is essential for any aspiring programmer.
The Order of Operations
Parentheses are the first level of operation in C++. They allow you to dictate the sequence in which calculations are performed. When parentheses are used, the enclosed operations are executed first, overriding the default precedence.
Example: Using Parentheses in C++
int a = 5;
int b = 10;
int result = (a + b) * 2; // Result is 30
In this example, the addition occurs before multiplication due to the parentheses.
Exponents are not natively represented in C++ as part of the basic arithmetic operators. Instead, to perform exponentiation, you would use the `pow()` function provided by the `<cmath>` library.
Using Library Functions Let's illustrate how to calculate powers in C++:
#include <cmath>
double powerResult = pow(2, 3); // Result is 8
Here, we’re using the `pow()` function to raise 2 to the power of 3, which yields 8.
For Multiplication and Division, C++ follows left-to-right associativity. This means that if there are multiple operations of the same precedence, they are executed in the order they appear from left to right.
Example: Multiplication and Division in C++
int val1 = 10;
int val2 = 2;
int val3 = 5;
int finalResult = val1 / val2 * val3; // Result is 25
In the above code, the division operation occurs first, yielding `5`, and then the result is multiplied by `5`, giving a final value of `25`.
When it comes to Addition and Subtraction, these operations are next in precedence, but like multiplication and division, they also follow left-to-right associativity.
Example: Addition and Subtraction in C++
int x = 20;
int y = 30;
int z = 10;
int result = x + y - z; // Result is 40
In this example, the addition takes place first, followed by subtraction, yielding the result of `40`.
Combining Operations
Complex expressions involving multiple operations can become tricky. The correct interpretation of PEMDAS is critical here to avoid errors.
Example: Complex C++ Expression
int complexResult = (10 + 5) * 2 - 8 / 4; // Result is 29
In the above expression, the operations are executed in the following order:
- The sum inside the parentheses, `(10 + 5)`, is calculated first, resulting in `15`.
- Then, we multiply it by `2` to get `30`.
- Subsequently, `8 / 4` evaluates to `2`.
- Finally, we subtract `2` from `30`, yielding a final result of `28`.
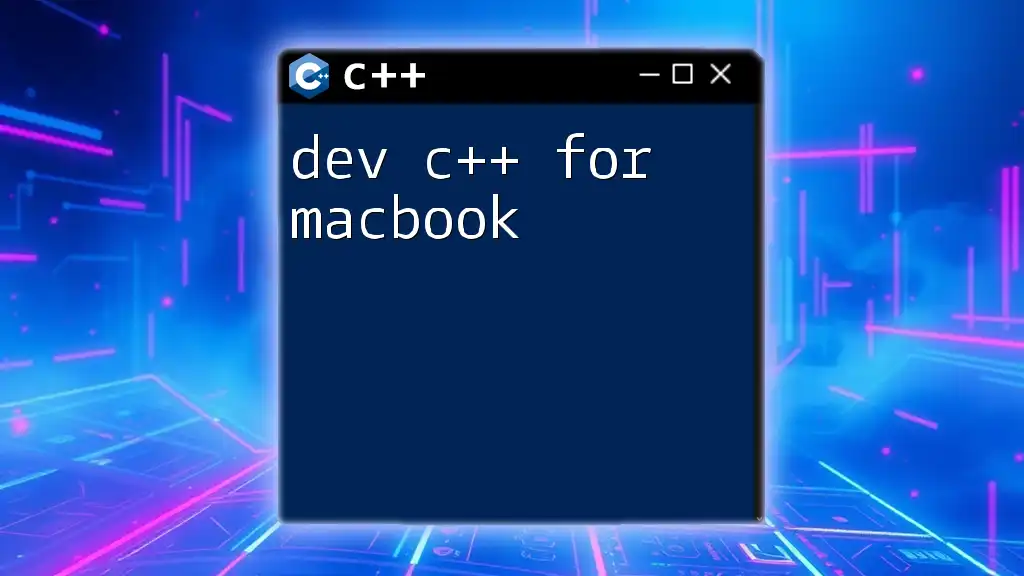
Common Mistakes in Interpreting PEMDAS
Misunderstandings regarding operator precedence can lead to unintended outcomes. Many programmers new to C++ may overlook the importance of parentheses, assuming multiplication and division will always take priority. This can result in incorrect calculations.
It’s essential to use parentheses for clarity, even when they are not strictly necessary by rules of precedence. They can greatly enhance code readability and maintainability.
Debugging and Verifying Results
One effective way to confirm the correctness of calculations in C++ is by using print statements. Displaying intermediate results can help in identifying where things may have gone wrong.
Example: Debugging Expressions
std::cout << "The result is: " << complexResult << std::endl;
By printing out the result, you can verify if your calculations are yielding the expected outcome.
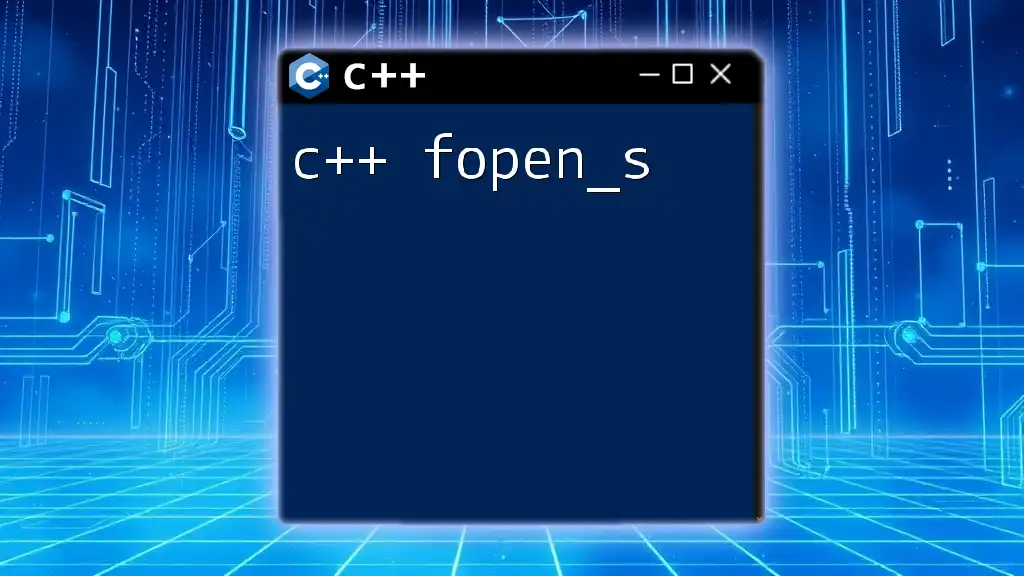
Tips for Programming with PEMDAS in C++
To avoid confusion and enhance your code’s clarity, use parentheses liberally, especially in complex expressions. It is also advisable to comment on your calculations to make your intent clear to others (and to your future self!).
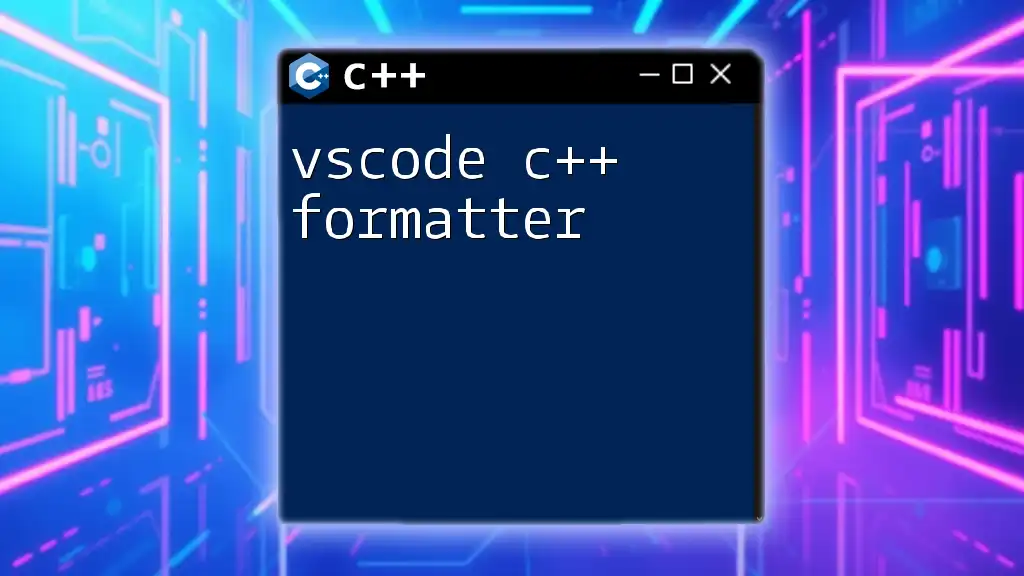
C++ Operator Precedence Table
Understanding C++ operator precedence is pivotal in ensuring the correct computation of expressions. A concise table can help navigate through the order of operations seamlessly. Always refer back to this table if you are ever in doubt.
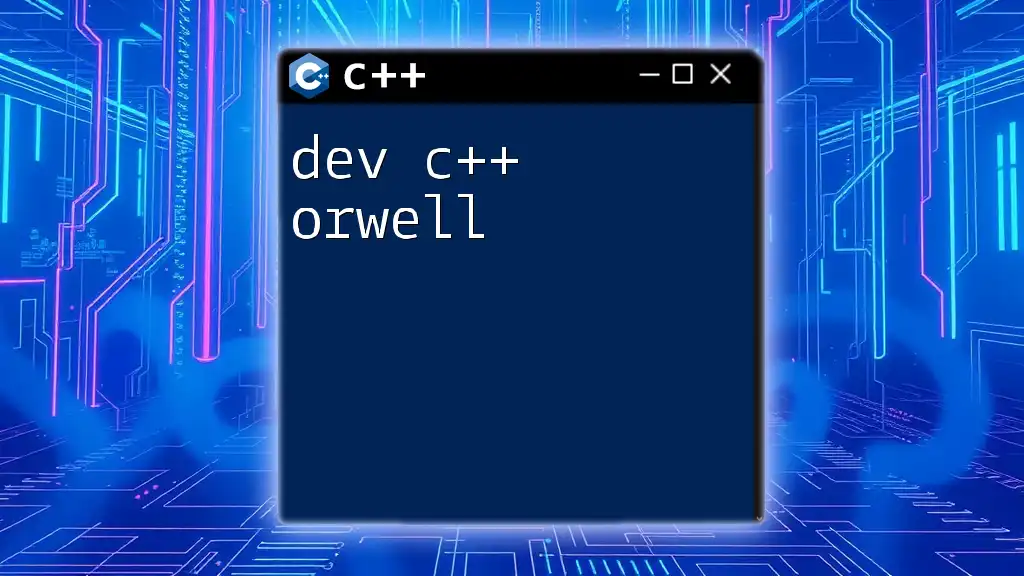
Conclusion
To wrap up, yes, C++ does follow PEMDAS! Understanding this fundamental principle is critical for writing effective and correct C++ code. As you delve deeper into the language, ensure you apply these rules judiciously in your expressions to avoid bugs and unintended behavior.
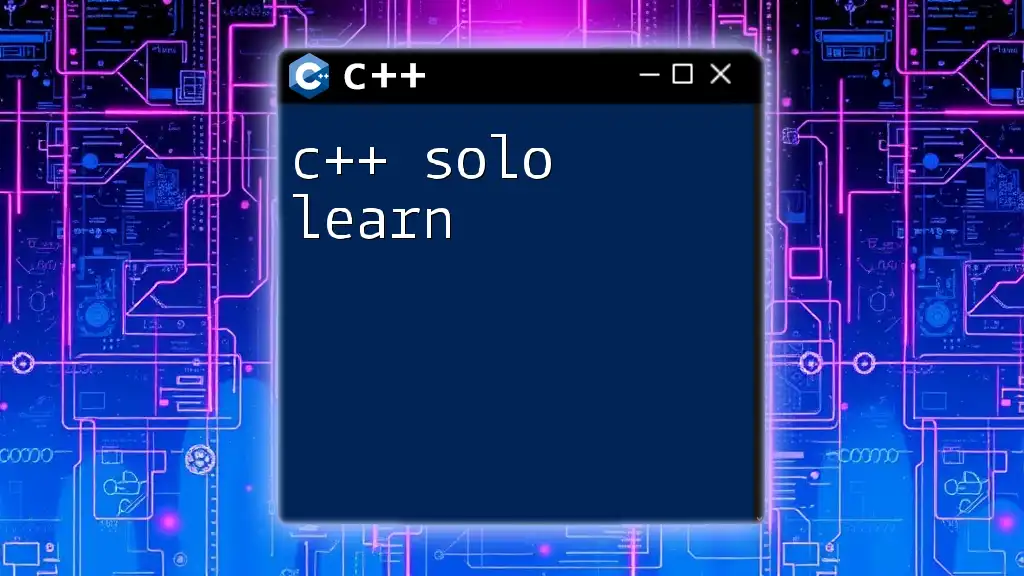
FAQs
Does C++ have any exceptions to PEMDAS?
Generally, C++ adheres to the PEMDAS rules strictly. However, nuances in expression formulation can sometimes lead to unexpected outcomes. Always verify the precedence when constructing your formulas.
Can you override default precedence in C++?
Yes! You can easily override the default precedence by using parentheses whenever necessary. It’s a best practice to employ parentheses even in simple calculations to avoid ambiguity and maintain clarity in your code.
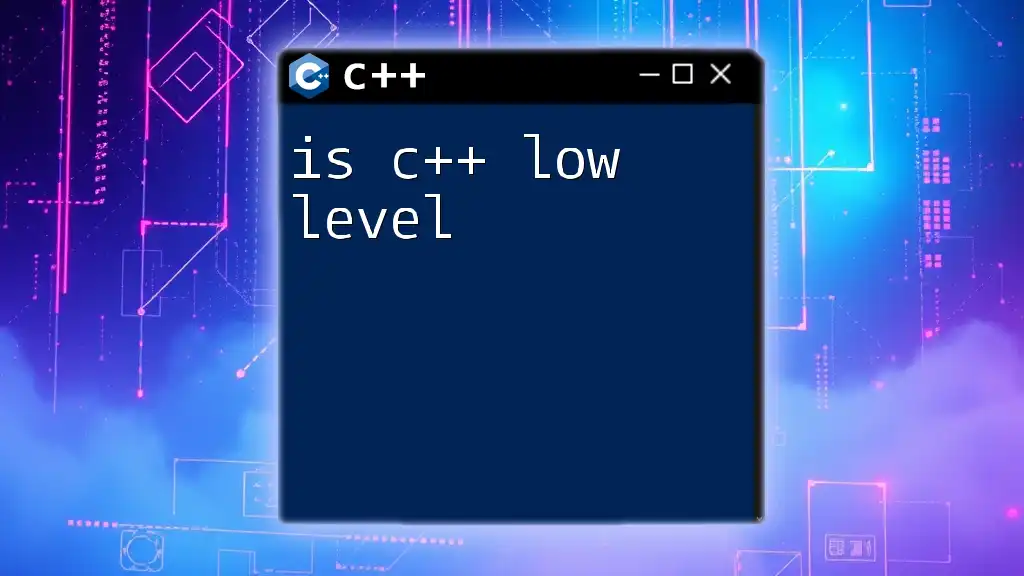
Call to Action
If you're eager to sharpen your skills in C++ and learn how to navigate complex operations effectively, consider joining our community or enrolling in our courses. Subscribe for more tutorials and updates that will take your programming knowledge to the next level.