"SoloLearn C++ is an interactive platform that enables users to learn C++ programming through concise tutorials and hands-on coding practices."
Here’s a simple C++ code snippet that demonstrates the basic "Hello, World!" program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Getting Started with C++ Solo Learn
Setting Up Your Environment
Choosing a Development Environment
When you embark on your C++ solo learning journey, the first critical step is selecting the right environment for coding. This can greatly influence both your experience and productivity.
- IDE vs. Text Editors: Integrated Development Environments (IDEs) generally provide features like debugging, auto-completion, and project management, making them ideal for complex projects. On the other hand, text editors, while lightweight, offer limited functionalities.
- Recommended IDEs for C++: Popular options include Code::Blocks, which is user-friendly and lightweight; Visual Studio, known for its robust features and deep integration with Windows; and CLion, praised for its intelligent code assistance.
Installing C++ Compiler
To execute your C++ code, you'll need a compiler. GCC (GNU Compiler Collection) and MinGW (Minimalist GNU for Windows) are commonly used compilers.
- Steps for Installing GCC or MinGW: Download the installer from the official site. Follow the installation instructions, and set up your environment variables to allow easy access from the command line.
- Once installed, you can check the installation by running `g++ --version` in your command prompt.
Basic Concepts of C++
C++ Syntax and Structure
Understanding the syntax is essential in C++. Each C++ program has a basic structure that acts as a skeleton.
- The entry point of a C++ program is the `main` function, which is where execution starts. A simple program looks like this:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Here, `#include <iostream>` brings in the I/O library to enable output via `std::cout`. A well-structured program helps in readability and debugging.
Variables and Data Types
Variables store data that your program can manipulate. In C++, variables must be declared with a specific data type.
Common Data Types:
- int: For integer values.
- float: For floating-point numbers.
- char: For single characters.
Here's how you might declare and use variables in C++:
int age = 30;
float salary = 75000.50;
char grade = 'A';
Understanding data types is crucial because they determine the kind of operations you can perform on the variables.
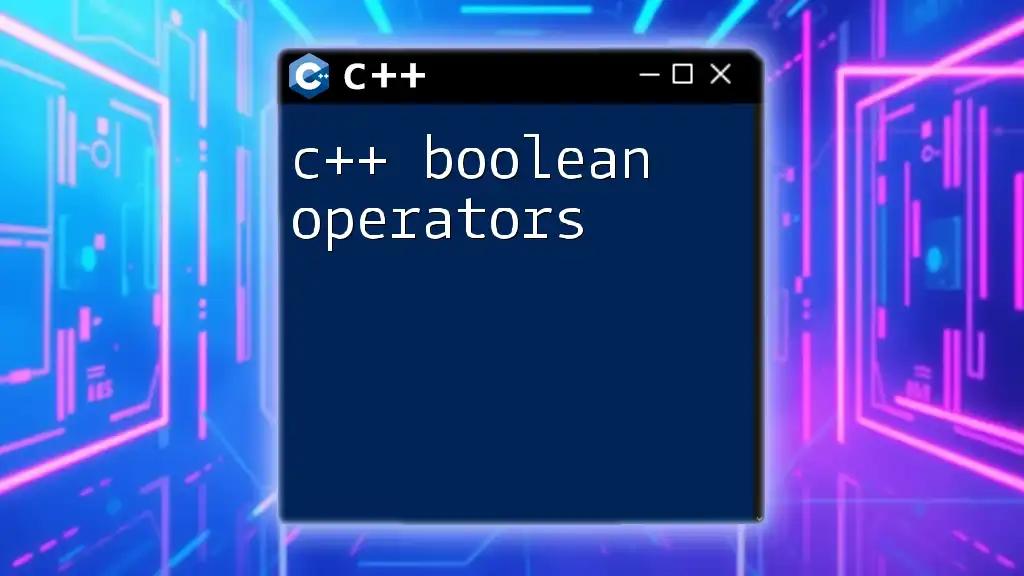
Diving Deeper into C++ Commands
Control Structures
Conditional Statements
Conditional statements allow your program to make decisions.
Example of `if`, `else if`, and `else` statements:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
This block checks whether an individual is an adult or minor, demonstrating how C++ can manage flow control based on conditions.
Loops in C++
Loops help you execute a sequence of instructions multiple times. Here are the types of loops available in C++:
- For Loop: Often used when the number of iterations is known.
Example of a `for` loop:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration " << i << std::endl;
}
This loop will iterate five times, printing the current iteration number at each step.
Functions and Scope
Defining and Calling Functions
Functions are blocks of code designed to perform particular tasks, allowing for code reusability.
Example of a simple function:
int add(int a, int b) {
return a + b;
}
This function takes two integer parameters, adds them together, and returns the result. Learning to define and call functions is key to maintaining clean code.
Understanding Scope in C++
Scope refers to the visibility of variables within different parts of a program.
-
Block Scope vs. Function Scope: Variables defined within a block are not accessible outside of it, while function-scoped variables are accessible throughout the function.
-
Using `static` and `extern` Keywords: The `static` keyword keeps a variable's value even after the function exits, and `extern` declares a variable that’s defined elsewhere.
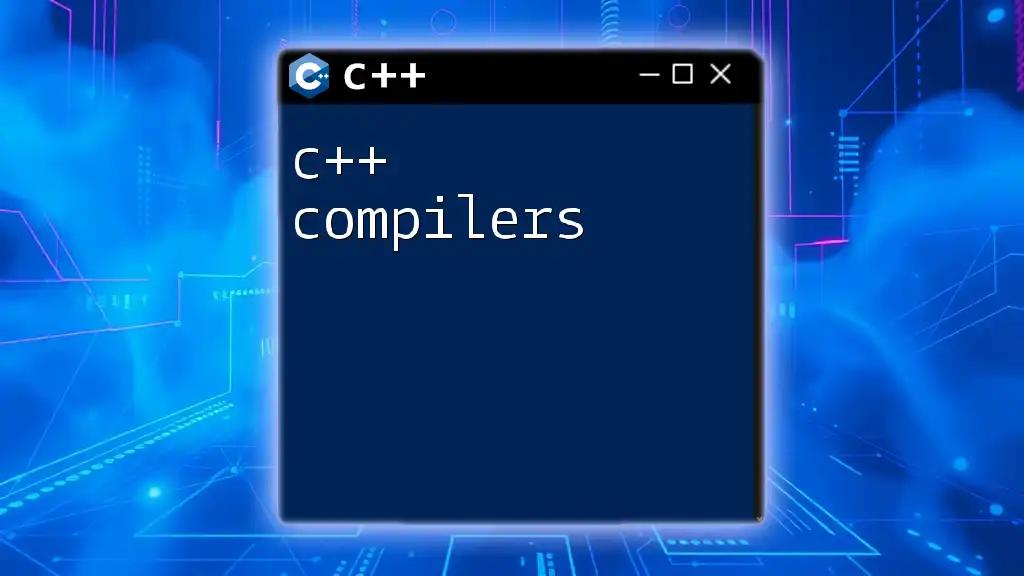
Advanced C++ Concepts
Object-Oriented Programming in C++
Introduction to Classes and Objects
C++ is famously known for its Object-Oriented Programming (OOP) features, which help in structuring applications for better design and modularity.
Example of defining a simple class:
class Car {
public:
void drive() {
std::cout << "Driving" << std::endl;
}
};
In this example, `Car` is a class with a public method `drive`. By creating objects from this class, you can call the `drive` method.
Inheritance and Polymorphism
Inheritance allows a new class to inherit properties and methods from an existing class, fostering code reusability and organization.
Example demonstrating inheritance:
class Vehicle {
public:
virtual void start() {
std::cout << "Vehicle starting" << std::endl;
}
};
class Bike : public Vehicle {
public:
void start() override {
std::cout << "Bike starting" << std::endl;
}
};
Here, `Bike` inherits from `Vehicle`, overriding the `start()` method to provide its specific implementation. This showcases polymorphism, where a derived class can redefine the behavior of a method defined in its base class.
Exception Handling in C++
What is Exception Handling?
Exception handling is a crucial part of robust programming. It allows programmers to manage runtime errors gracefully rather than crashing the program.
Basic syntax of try, catch, and throw:
try {
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cout << "Caught: " << e.what() << std::endl;
}
In the example above, a runtime error is thrown and caught, demonstrating how exceptions can be handled to ensure stability within your applications.

Resources for Continued Learning
Online Platforms for Interactive Learning
Solo Learn C++ Courses
The Solo Learn C++ courses are designed to engage learners through interactive lessons. The easy-to-use platform allows you to practice coding through assignments and real-time feedback. Additionally, the community features enable you to connect with other learners, enriching your educational experience.
Other Learning Resources
Apart from Solo Learn, many enriching resources can supplement your C++ journey:
- Books: "C++ Primer" by Lippman is a great start.
- Websites: platforms like Codecademy and freeCodeCamp offer coding exercises.
- Forums: Engage with the C++ community on platforms like Stack Overflow and Reddit to gain insights and solve coding challenges.
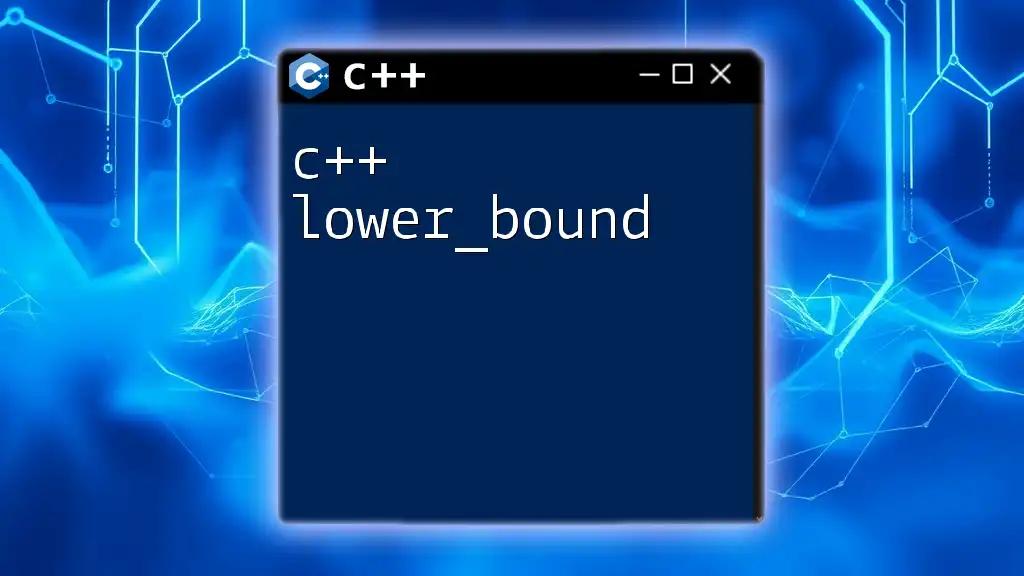
Conclusion
Embarking on a C++ solo learn journey is both challenging and immensely rewarding. The key is persistence and practice. As you progress, you will gain confidence to implement what you've learned into real-world applications, allowing for growth in your programming skills.
Call to Action
Join our C++ community to enhance your learning experience. Engage, share, and connect with fellow enthusiasts as you dive deeper into the world of C++. Start your journey today!