C++ provides an array of libraries and techniques to interact with Google's APIs, enabling developers to integrate Google's powerful services into their applications seamlessly.
Here's a simple example of using the Google API with C++:
#include <iostream>
#include <curl/curl.h>
int main() {
CURL *curl;
CURLcode res;
curl_global_init(CURL_GLOBAL_DEFAULT);
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "https://www.googleapis.com/books/v1/volumes?q=Harry+Potter");
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
Understanding C++ and Its Ecosystem
What is C++?
C++ is a powerful, high-level programming language that combines features from both high-level and low-level languages. It was developed in the early 1980s as an extension of the C programming language, with an emphasis on object-oriented programming (OOP). C++ is known for its performance, speed, and ability to manipulate hardware resources, making it widely used in various applications like game development, systems programming, and high-performance software.
Benefits of Learning C++
Embracing C++ can be advantageous in several ways:
-
Performance and Efficiency: C++ provides fine-grained control over system resources and memory management, which can lead to high-performance applications. Programs written in C++ often run faster than those written in higher-level languages.
-
Application Areas: From game engines like Unreal Engine to operating systems and embedded systems, C++ is a fundamental language in important technological domains. This versatility opens many doors for learners and professionals alike.
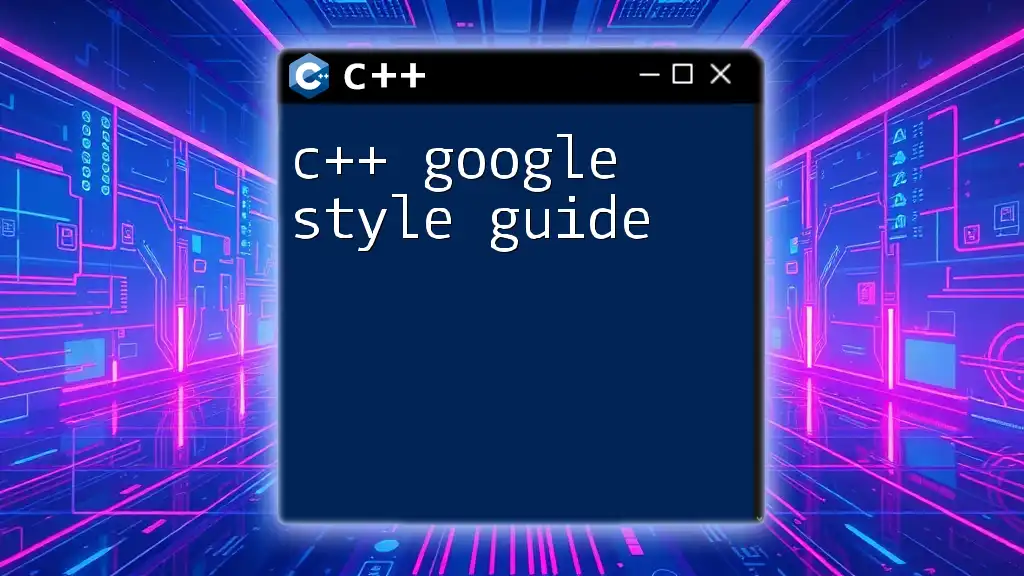
Using Google for C++ Programming
Searching for C++ Information Effectively
Utilizing Google effectively can make a significant difference in your C++ learning journey. The following strategies can help streamline your search process:
- Crafting Effective Search Queries: Use specific keywords when searching for C++ resources. Instead of searching for a vague term like "C++ tutorial," try more targeted searches like "C++ data structures tutorial" or "C++ class examples." This will yield more relevant results.
Google Search Operators for Better Results
Understanding Google's search operators can refine your search results even further. Here are some useful operators to consider:
-
`site:` Operator: Direct your search towards specific domains.
- Example: If you're looking for discussions or answers on Stack Overflow, use `site:stackoverflow.com C++ template`.
-
`filetype:` Operator: Find specific types of documents.
- Example: Use `filetype:pdf C++ programming guide` to locate downloadable PDF guides.
-
`intitle:` Operator: Narrow down searches by focusing on titles.
- Example: Searching for `intitle:"C++ best practices"` could lead you to highly relevant articles.
Leveraging Google Scholar
For those looking to dig deeper into more advanced topics in C++, Google Scholar is an invaluable resource. It allows you to find academic papers, theses, and books that cover complex aspects of C++ programming and its methodologies. Use phrases like "C++ design patterns" or "C++ performance optimization" to locate in-depth scholarly discussions.
Utilizing Google Groups and Forums
Google Groups and relevant C++ forums can be a treasure trove of information. Participating in these communities allows you to:
- Ask Questions: If you're struggling with a concept or code, don't hesitate to pose questions to the group. Frame your query clearly to maximize response quality.
- Engage in Discussions: Take the time to read and respond to others' questions. Active participation can enhance your understanding and help you build networks.
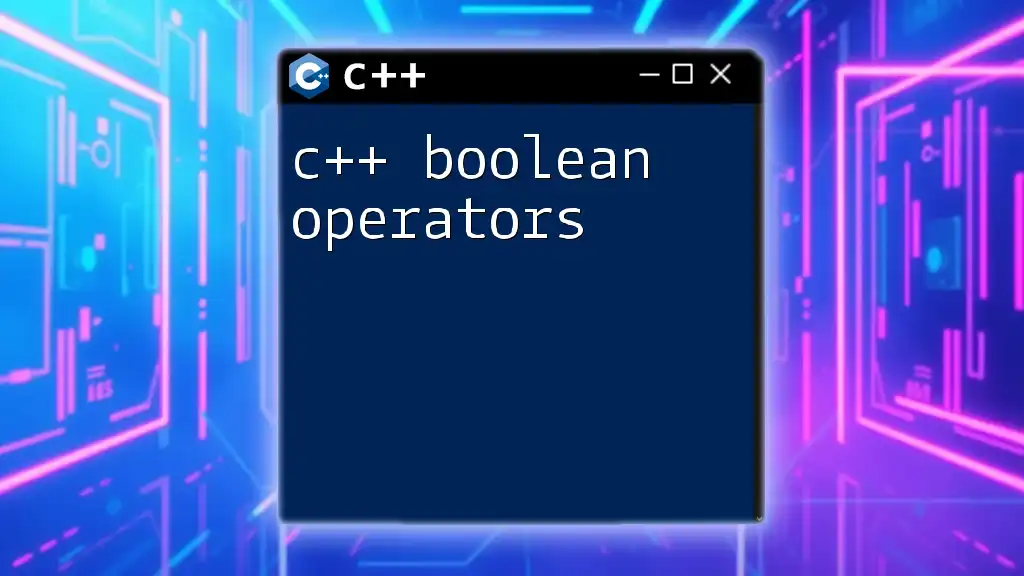
Finding C++ Libraries and Frameworks
How to Search for C++ Libraries
Finding appropriate libraries and frameworks can save you time and effort in your C++ projects. Effective search terms can guide your efforts:
- Use search phrases like "C++ networking library," "C++ graphics framework," or "C++ machine learning libraries" to uncover valuable resources.
Popular C++ Libraries and Frameworks
Several libraries and frameworks stand out for their utility in C++ development:
-
Standard Template Library (STL): A powerful set of C++ template classes that provide common data structures and algorithms.
- Example Usage: Sorting a list of numbers using STL:
#include <iostream> #include <vector> #include <algorithm> int main() { std::vector<int> numbers = {4, 1, 3, 9, 5}; std::sort(numbers.begin(), numbers.end()); for (const auto& num : numbers) { std::cout << num << " "; } return 0; }
-
Boost: An expansive library that extends the functionality available in the standard library. It includes modules for multi-threading, file systems, and robust data structures.
-
Qt: A framework for building cross-platform applications with a graphical user interface (GUI). Its signal and slot mechanism is particularly well-regarded.
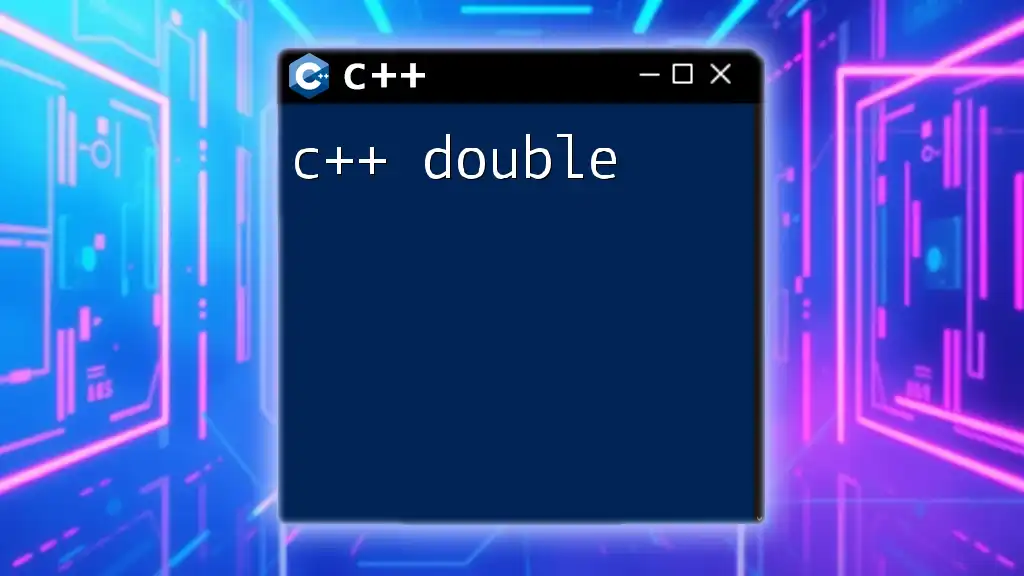
Searching for C++ Tutorials and Learning Resources
Where to Find C++ Tutorials
There are countless places to seek C++ tutorials, such as:
- Online platforms like Codecademy and Coursera offer structured C++ courses.
- Blogs such as GeeksforGeeks provide detailed tutorials along with code examples.
Reviewing C++ Documentation
Always refer to the official C++ documentation for accurate and up-to-date information. Websites like cplusplus.com and cppreference.com are excellent for understanding functions, classes, and libraries.
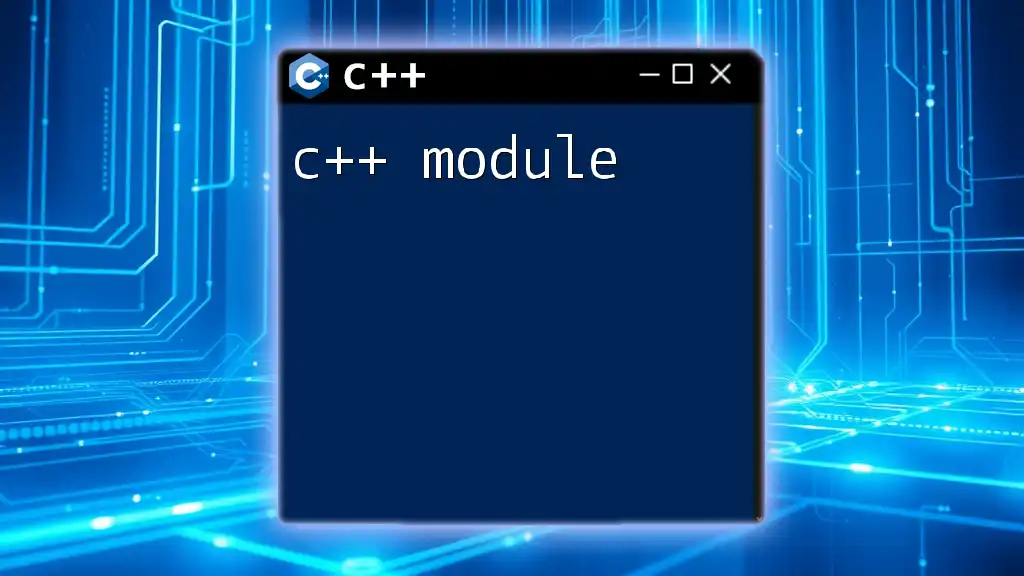
Debugging and Troubleshooting C++ Code
Using Google to Solve Common C++ Errors
When faced with C++ errors, a quick Google search can often provide solutions. Search for specific error messages to find troubleshooting steps.
- For instance, if you encounter "error: incompatible types," search the exact phrase in quotes. This can lead you to forums or articles discussing similar issues and their fixes, effectively speeding up your debugging process.
Forums and Stack Overflow
When searching for answers, don’t overlook the value of forums and platforms like Stack Overflow. Craft your question carefully, providing necessary context and code snippets. Additionally, before asking, always search to see if similar issues have already been resolved.
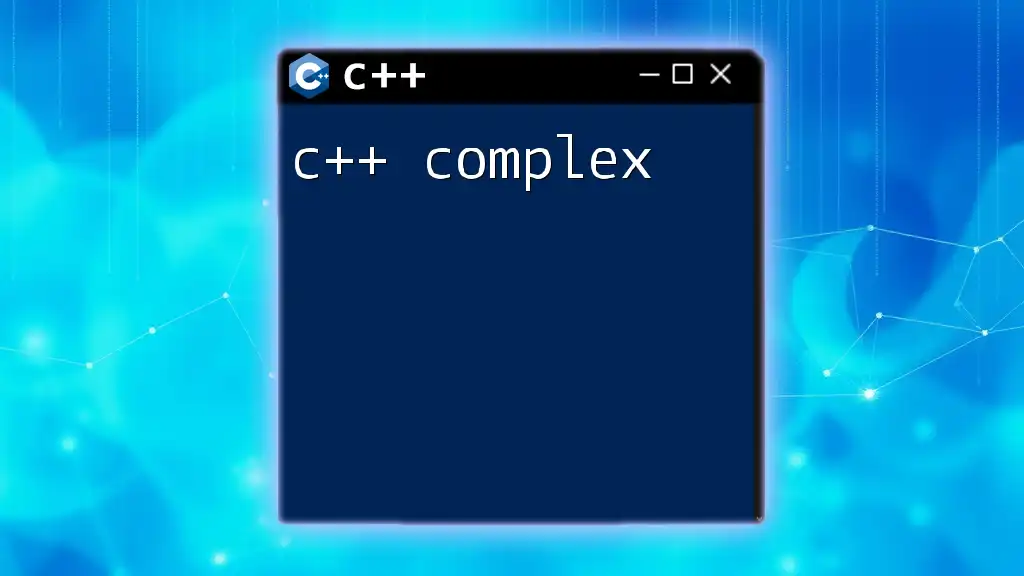
Best Practices for Efficient C++ Coding
Learning from Code Examples
Google can help you find a wealth of code snippets and library examples.
- Search for specific functionality like "C++ file handling example" to read and analyze well-crafted code. Understanding how others structure their code can be enlightening and improve your coding style.
Exploring GitHub Repositories
GitHub is a prime location to explore open-source C++ projects. Searching for "C++ projects" or "C++ repositories" can yield numerous examples of practical applications:
- Studying real-world codebases enhances your understanding of structured programming. Look for repositories with good documentation and active contributions to learn best practices.
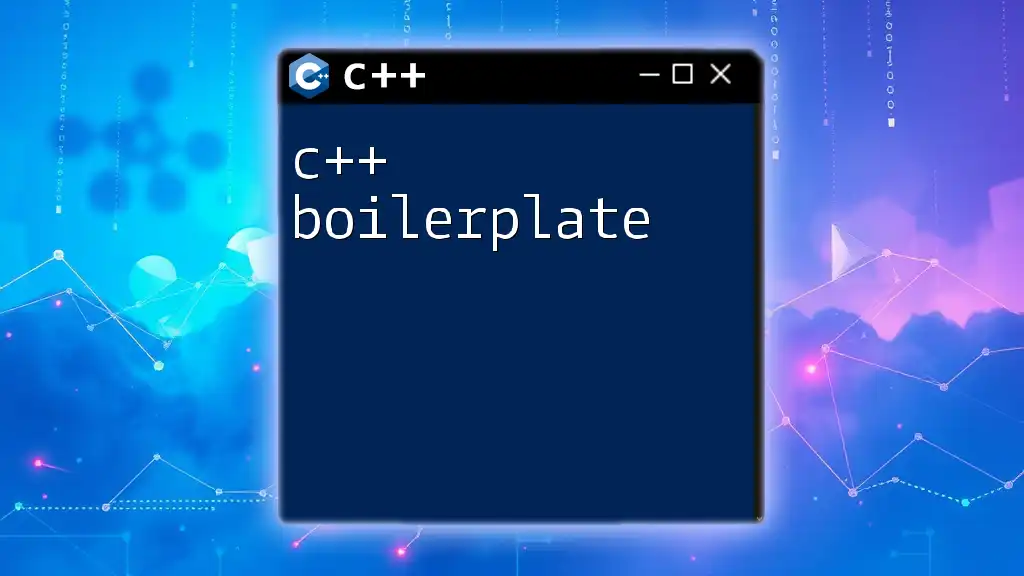
Conclusion
By harnessing the power of Google effectively, you can significantly enhance your C++ programming skills. From utilizing advanced search operators and engaging in forums to finding libraries, tutorials, and project examples, Google serves as an invaluable resource for both beginners and advanced programmers. Continuous learning and exploration will pave the way for mastering C++.
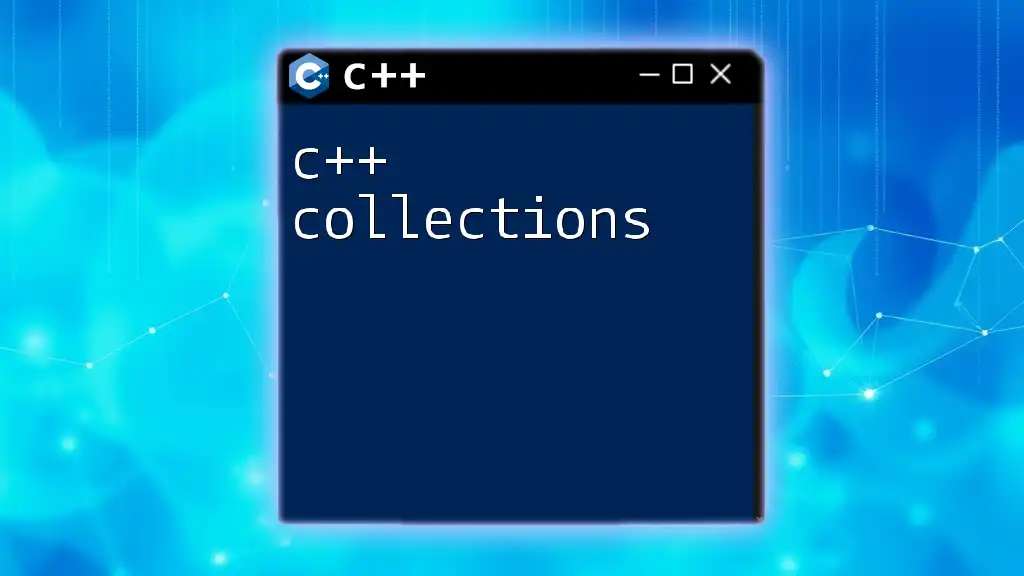
Call to Action
We invite you to share your favorite C++ resources and tips in the comments below. Join us on social media and participate in our forums for more interactive discussions to further enrich your C++ journey!