C++ good practices involve writing clear, efficient, and maintainable code by adhering to principles such as using meaningful variable names and properly managing memory.
#include <iostream>
#include <vector>
// Use descriptive variable names and RAII to manage resources
class DataProcessor {
public:
void addData(int value) {
data.push_back(value); // Clear and concise variable usage
}
void processData() {
for (int value : data) {
std::cout << value * 2 << std::endl; // Meaningful output operation
}
}
private:
std::vector<int> data; // Utilize STL to manage memory effectively
};
int main() {
DataProcessor processor;
processor.addData(5);
processor.addData(10);
processor.processData();
return 0;
}
Understanding C++ Good Practices
What Are Good Practices?
Good practices in coding are essential guidelines that help developers write clear, efficient, and maintainable code. These practices not only improve the quality of individual codebases but also facilitate collaboration among team members, making it easier for others to understand and contribute to a project.
Why Focus on C++ Best Practices?
C++ is a powerful and versatile programming language, but it comes with its own set of complexities. Following best practices can help mitigate the potential pitfalls inherent to C++, such as memory leaks and undefined behavior. By adhering to established guidelines, developers can create robust and high-performing applications that are easier to manage, update, and debug.
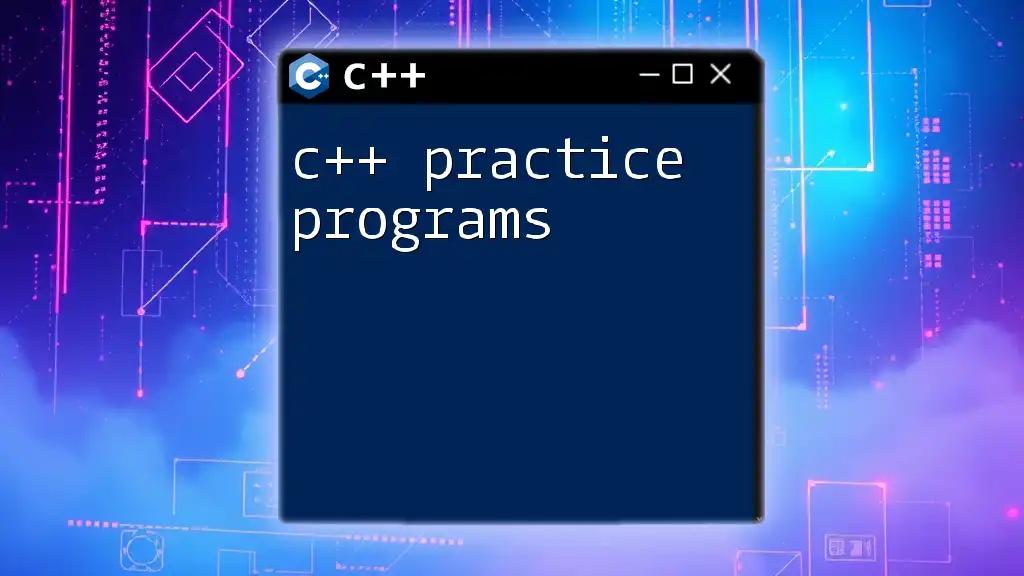
C++ Best Practices: Key Principles
Code Readability and Maintainability
Use Meaningful Variable and Function Names
Choosing meaningful names for variables and functions is crucial for code readability. Names should convey the purpose of the variable or function without requiring additional context. For instance, instead of using vague names, you can use descriptive ones like:
int numberOfStudents; // Clear and descriptive
float averageScore; // More informative than avg
Using clear names helps other programmers (and your future self) quickly understand your code, leading to fewer misunderstandings and mistakes.
Commenting Code Effectively
While writing clear and expressive code can minimize the need for comments, they still play a vital role in understanding complex logic or decisions made. Comments should be concise and provide essential context.
For example, consider the following code snippet:
// Calculates the tax for a given price
float calculateTax(float price) {
return price * 0.05;
}
Here, the code is straightforward, but if you were to implement a complex algorithm, thorough commenting could significantly enhance its understandability.
Consistency in Coding Style
Follow a Consistent Indentation and Formatting Style
Adopting a consistent code formatting style helps maintain a clean codebase. Various styles exist, such as K&R and Allman. You can choose any standard but must apply it uniformly throughout your code. Tools like `clang-format` and `AStyle` can automatically format your code to comply with your chosen style.
Grouping Related Code
Modularizing your code by grouping related functions and classes enhances structure and readability. For example:
void printStudentInfo(Student s) {
// Print student details like name, age, etc.
}
void updateStudentInfo(Student &s) {
// Update student information based on user input
}
By organizing your code into logical sections, you create a clearer separation of concerns, making it easier for others to navigate your work.
Memory Management
Utilize Smart Pointers
C++ offers powerful tools like smart pointers, which help manage dynamic memory automatically. Using smart pointers like `std::unique_ptr` and `std::shared_ptr` can eliminate many memory-related bugs. For example:
#include <memory>
std::unique_ptr<int> ptr = std::make_unique<int>(42); // Automatically managed
By avoiding manual memory management, you minimize the risk of memory leaks and dangling pointers.
Avoid Memory Leaks
While smart pointers automate memory management, if you are using raw pointers, it is essential to ensure every `new` statement has a corresponding `delete`. Neglecting this can lead to memory leaks:
int* ptr = new int(5);
// Missing delete statement can lead to memory leak
delete ptr; // Always ensure you free allocated memory
Error Handling
Use Exceptions for Error Handling
Handling errors gracefully is crucial for creating robust applications. C++ provides a powerful exception handling mechanism that should be used instead of relying on return codes. Here's a simple example:
try {
throw std::runtime_error("An error occurred");
} catch (const std::exception& e) {
std::cerr << e.what() << std::endl;
}
Using exceptions allows you to maintain cleaner code and separate error handling from regular logic.
Validate Input
Validating input can prevent your application from encountering undefined behavior due to erroneous data. Always check for valid input before processing it:
if (userInput < 0) {
throw std::invalid_argument("Input must be non-negative");
}
By validating input, you protect your application from unexpected crashes and maintain a good user experience.
Leverage the Standard Library
Use Built-in Containers and Algorithms
The C++ Standard Template Library (STL) offers a range of containers and algorithms that enhance productivity and performance. For instance, using `std::vector` can simplify memory management:
#include <vector>
#include <algorithm>
std::vector<int> nums = {1, 2, 3, 4, 5};
std::sort(nums.begin(), nums.end());
This approach not only saves time but also utilizes well-tested and optimized code provided by the standard library.
Avoid Reinventing the Wheel
When developing in C++, always consider whether existing libraries can help you achieve your goals. For instance, libraries such as Boost and Poco offer additional functionalities that can save you time and effort.
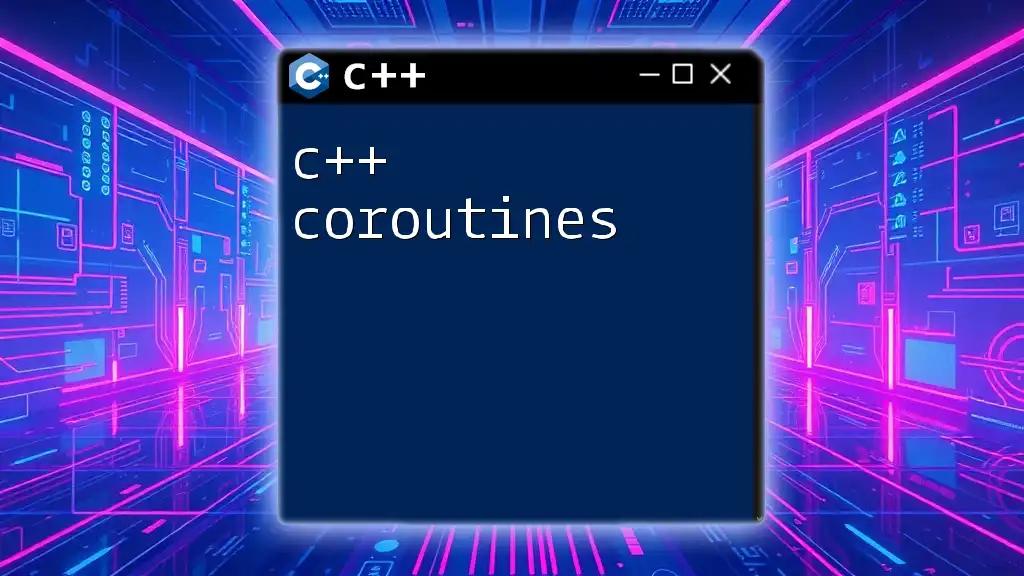
C++ Performance Best Practices
Optimize for Performance
Avoid Unnecessary Copies
Understanding C++'s move semantics and rvalue references is vital for optimizing performance. When passing large objects, prefer to use references to avoid costly copies:
class MyClass {
public:
MyClass(MyClass&& other) noexcept; // Move constructor
};
By embracing move semantics, you ensure that your program is efficient and minimizes resource usage.
Inline Functions
Using inline functions when appropriate can reduce function call overhead in performance-critical code. It hints to the compiler that the function should be expanded in place:
inline int add(int a, int b) { return a + b; }
This can lead to faster execution times, particularly in small, frequently called functions.
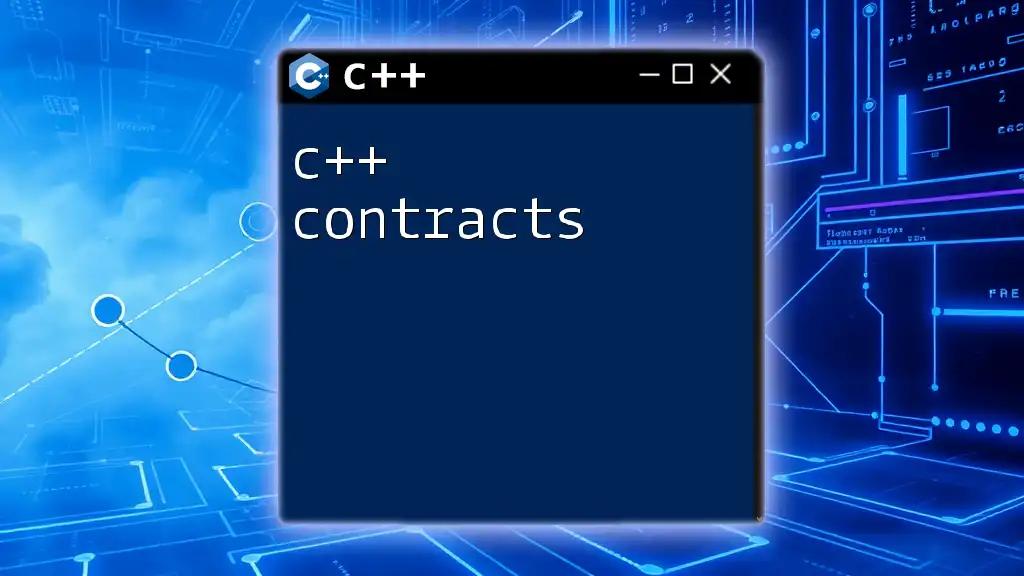
Testing and Debugging
Unit Testing and Debugging Practices
Write Unit Tests
Unit testing is an indispensable part of software development. Implementing tests for your code ensures its correctness and helps catch bugs early. Frameworks like Google Test make it easy to write and run unit tests:
#include <gtest/gtest.h>
TEST(MyTestSuite, MyTest) {
EXPECT_EQ(add(2, 3), 5); // Assert that add function works correctly
}
By frequently running your tests, you can maintain confidence in your code's correctness.
Use Debugging Tools
Debugging tools such as GDB and Valgrind can help you diagnose issues in your code. Learning to leverage these tools effectively can save you countless hours when tracking down elusive bugs or memory errors.
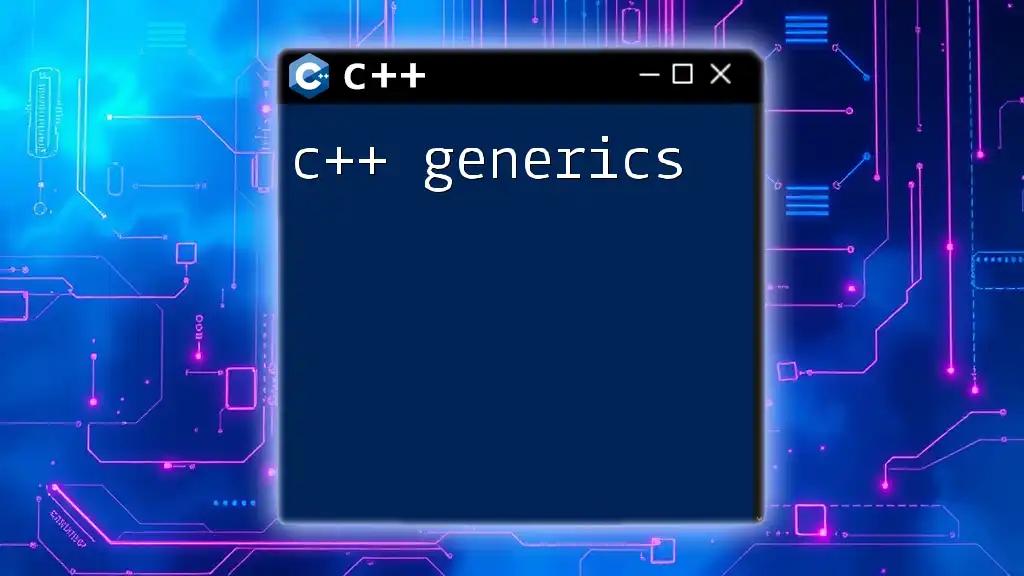
Conclusion
Implementing C++ good practices is a critical step for any developer aiming to write high-quality, maintainable, and efficient code. By focusing on readability, consistency, memory management, error handling, leveraging the standard library, optimizing performance, and employing robust testing strategies, you can create software that stands the test of time.