A C++ 2D graphics engine enables developers to create and manipulate 2D visual representations, often utilizing libraries such as SDL or SFML for rendering graphics.
Here's a simple example using the SFML library to create a window and draw a circle:
#include <SFML/Graphics.hpp>
int main() {
sf::RenderWindow window(sf::VideoMode(800, 600), "2D Graphics Engine Example");
sf::CircleShape shape(50);
shape.setFillColor(sf::Color::Green);
shape.setPosition(375, 275);
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(shape);
window.display();
}
return 0;
}
What is a 2D Graphics Engine?
A 2D graphics engine is a software system designed to facilitate the creation of two-dimensional graphics. It provides developers with the necessary tools to render graphics, manipulate objects, and manage various game components across a scene. In game development, a 2D graphics engine acts as the backbone, ensuring that all visual elements come together smoothly and efficiently.
Understanding how a graphics engine works is crucial for any game developer wishing to create visually appealing and performant games. Unlike 3D graphics engines, which deal with rendering three-dimensional objects and perspectives, 2D engines focus on flat images and sprites, allowing developers to create engaging gameplay that emphasizes storytelling and interaction.
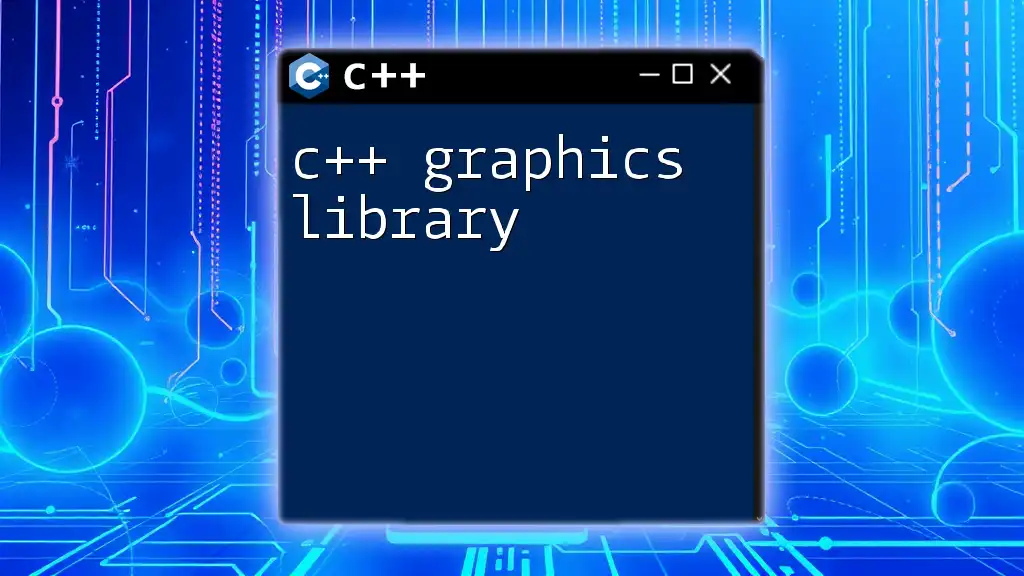
Why Choose C++ for Graphics Engines?
C++ is a go-to language for many game developers, primarily due to its performance capabilities and flexibility. Here are a few reasons why creating a C++ 2D graphics engine is advantageous:
- Performance: C++ provides high-performance execution, which is essential for rendering graphics smoothly and efficiently, especially for games with many concurrent visual elements.
- Control Over Memory Management: With C++, developers can manage memory efficiently, leading to better optimization and performance tuning of graphics-heavy applications.
- Community Support: C++ has a large community of game developers, meaning there are plenty of libraries, frameworks, and resources readily available.
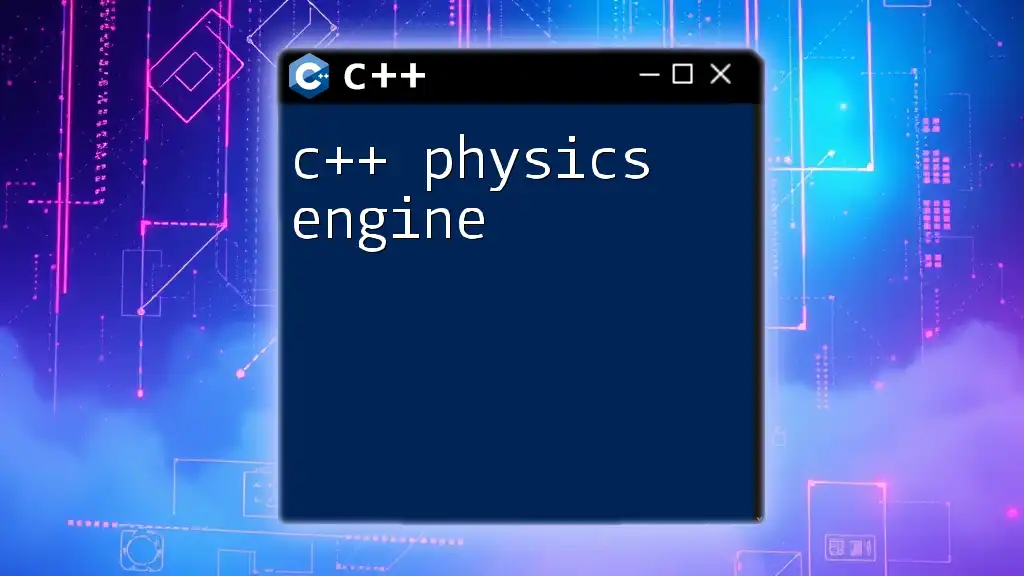
Key Components of a C++ 2D Graphics Engine
Rendering System
The rendering system is one of the most vital components of any graphics engine. It is responsible for displaying graphics on the screen, processing, and drawing all visual elements.
Understanding Rendering Pipelines
Rendering typically follows a predetermined pipeline. This involves fetching graphics data, processing it, and then sending it to the GPU for display. A basic rendering loop can be structured as follows:
while (running) {
update(); // Calculate the new state of the game
render(); // Render the graphics
}
This loop allows for the continuous refreshing of the screen, giving the illusion of motion and interaction.
Graphics API Integration
C++ graphics engines often interface with graphics APIs such as OpenGL or SDL for rendering graphics. For instance, initializing an OpenGL context can be done in a few steps:
// Initialize GLFW
if (!glfwInit()) {
// Initialization failed
}
// Create OpenGL window
GLFWwindow* window = glfwCreateWindow(800, 600, "2D Graphics Engine", NULL, NULL);
glfwMakeContextCurrent(window);
This snippet demonstrates how to set up a basic OpenGL environment, which is essential for any rendering tasks in a C++ 2D graphics engine.
Input Handling
User input management is vital for creating engaging gameplay. Players interact via keyboards, mice, or game controllers, and understanding how to manage these inputs enhances user experience.
Building an Input System
A robust input system can help capture user actions and transform them into in-game actions. For instance, using SDL to capture keyboard input is straightforward:
SDL_Event event;
while (SDL_PollEvent(&event)) {
if (event.type == SDL_QUIT) {
running = false;
}
if (event.type == SDL_KEYDOWN) {
switch (event.key.keysym.sym) {
case SDLK_UP:
// Move object up
break;
case SDLK_DOWN:
// Move object down
break;
}
}
}
With this setup, developers can react to user input, whether it’s for movement, actions, or navigating menus.
Scene Management
Efficient scene management organizes all game objects, ensuring clear relationships and hierarchies.
Implementing Simple Scene Graphs
A scene graph allows for hierarchical organization, making it easier to manage complex scenes with many objects. A basic implementation might include:
class GameObject {
public:
std::vector<GameObject*> children;
void addChild(GameObject* child) {
children.push_back(child);
}
// Other methods for updating and rendering
};
This structure provides a way to manage how objects interact and render based on their relationships within the scene.
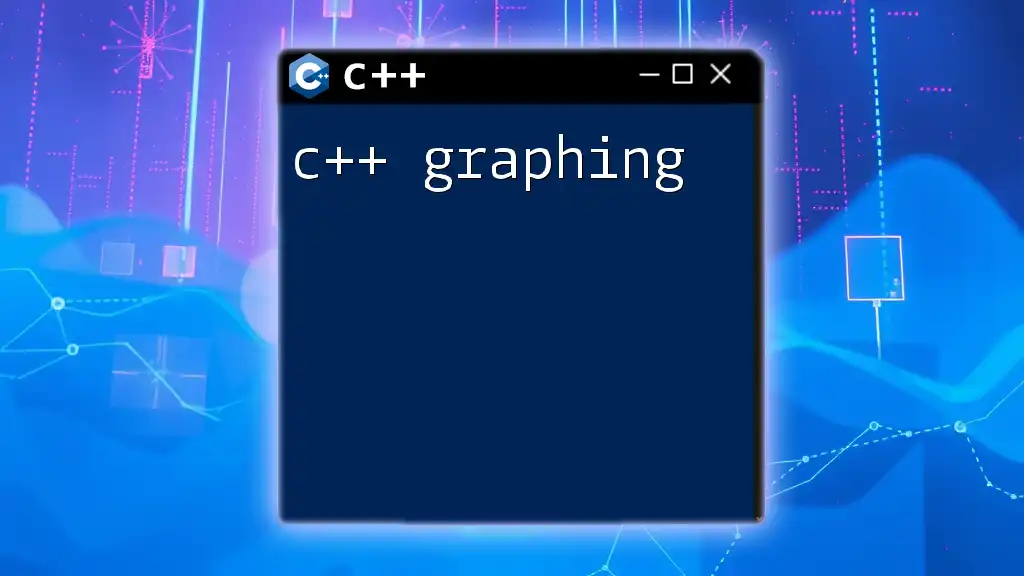
Core Functionalities of a C++ 2D Engine
Sprites and Animation
Sprites are the lifeblood of 2D graphics engines. They represent objects that move around the screen and can be animated for added effects.
Understanding Sprites
Sprites are typically images displayed on the screen. Loading and displaying a sprite in your engine might look like this:
SDL_Texture* loadTexture(const char* path) {
SDL_Texture* texture = IMG_LoadTexture(renderer, path);
return texture;
}
This simple function allows developers to load textures from an image file, paving the way for sprite usage in any game.
Animation Techniques in 2D
Animation can be frame-based or skeletal. A basic implementation of frame-based animation could resemble the following snippet:
class Animation {
public:
std::vector<SDL_Texture*> frames;
int currentFrame;
int frameDelay;
void update() {
// Update frame based on time and delay
if (/* condition met for frame change */) {
currentFrame = (currentFrame + 1) % frames.size();
}
}
};
This logic controls frame progression based on time, enabling seamless animation transitions in games.
Physics and Collision Detection
Implementing physics in a game offers a realistic feeling, allowing developers to simulate object interactions.
Basic Collision Detection Techniques
One of the simplest collision detection methods is the Axis-Aligned Bounding Box (AABB) technique. The logic for collision detection can be easily structured as follows:
bool checkCollision(const SDL_Rect& a, const SDL_Rect& b) {
return SDL_HasIntersection(&a, &b);
}
This straightforward function checks if two rectangles overlap, ensuring you can detect and handle collisions in your game.
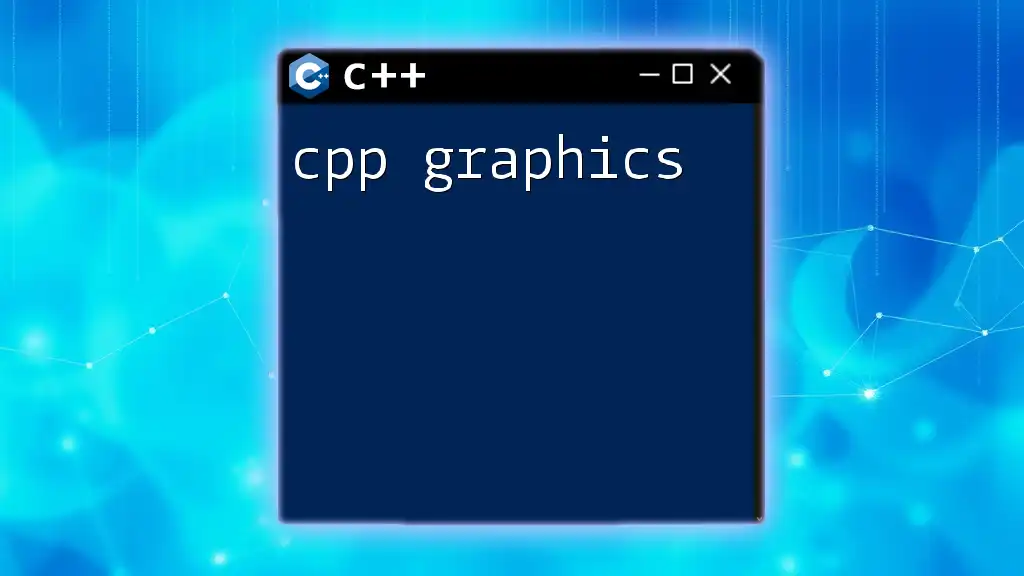
Building Your First C++ 2D Game
Setting Up Your Development Environment
Before diving into building a game, you need to set up your development environment. Popular IDEs, such as Visual Studio or Code::Blocks, work well for C++ game development. Additionally, libraries like SDL or SFML provide the needed tools to build games.
Game Architecture Overview
Understanding the architecture of your game is crucial. The game loop is the heart of this architecture, taking care of continuous updates and rendering.
Understanding Game Loops and States
A typical game loop continuously updates game logic and renders graphics. Here’s a basic skeleton of a game loop:
void gameLoop() {
while (running) {
handleInput();
updateGameState();
renderGraphics();
}
}
Implementing a Simple 2D Game
To create something playable, you can start with a simple game like a platformer. Implementing a basic game could follow these steps:
- Game Setup: Initialize libraries, set up the window, and prepare assets.
- Manage Game State: Use a system to transition between the main menu, gameplay, and pause states.
Code Snippet: Initial Game Setup Code
void initialize() {
// Initialize SDL
if (SDL_Init(SDL_INIT_VIDEO) < 0) {
// Handle error
}
// Create a window and a renderer
SDL_Window* window = SDL_CreateWindow("Simple 2D Game", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, 800, 600, 0);
SDL_Renderer* renderer = SDL_CreateRenderer(window, -1, 0);
}
Handling Game Events and States
Managing game states ensures players have a smooth experience transitioning between menus, gameplay, and other functional areas. This can be managed through state machines or by encapsulating logic into different classes.
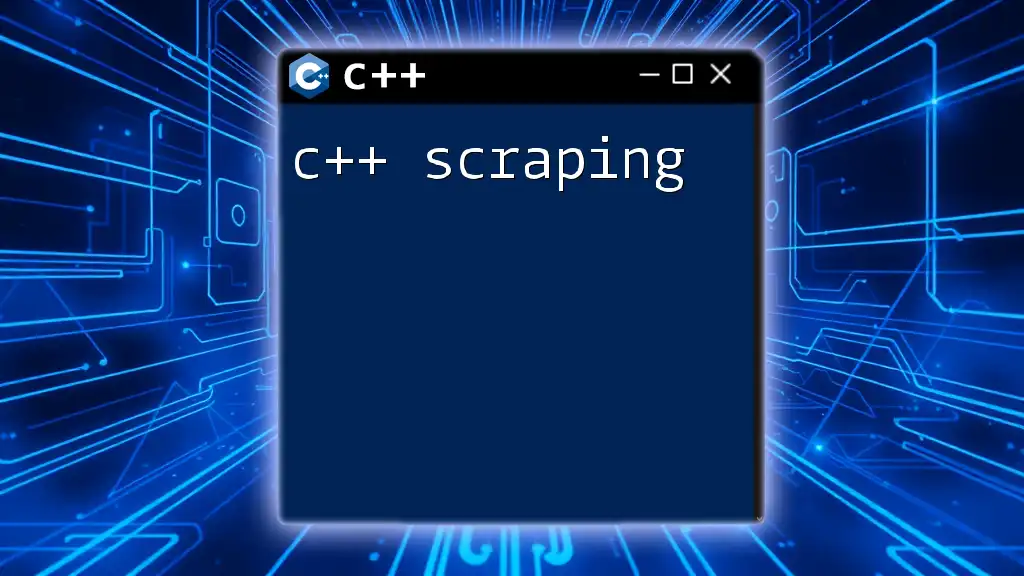
Advanced Features in C++ 2D Graphics Engines
Particle Systems
Particle systems are essential for creating dynamic visual effects that enhance gameplay by simulating phenomena like explosions or weather effects.
What are Particle Systems?
A particle system works by generating a large number of tiny visual elements, or particles, that behave under the influence of physics principles like gravity, wind, or friction.
Basic Implementation of a Particle System
Creating a simple particle system might look something like this:
class Particle {
public:
SDL_Point position;
SDL_Color color;
float lifetime;
void update() {
// Update particle position and decay lifetime
}
};
This structure allows you to dynamically generate and update a visual representation of effects in your game.
Sound and Music Integration
Audio plays a crucial role in elevating a gaming experience. Background music and sound effects keep players immersed and engaged.
Importance of Audio in 2D Games
Integrating sound into games enhances emotional responses and provides feedback for in-game actions.
Integrating Audio Libraries
Using libraries like SDL_mixer allows for manageable sound integration. An example of playing background music may look like this:
Mix_OpenAudio(44100, MIX_DEFAULT_FORMAT, 2, 2048);
Mix_Music* music = Mix_LoadMUS("background.mp3");
Mix_PlayMusic(music, -1);
This represents how to initialize audio, load music, and play it in a loop.
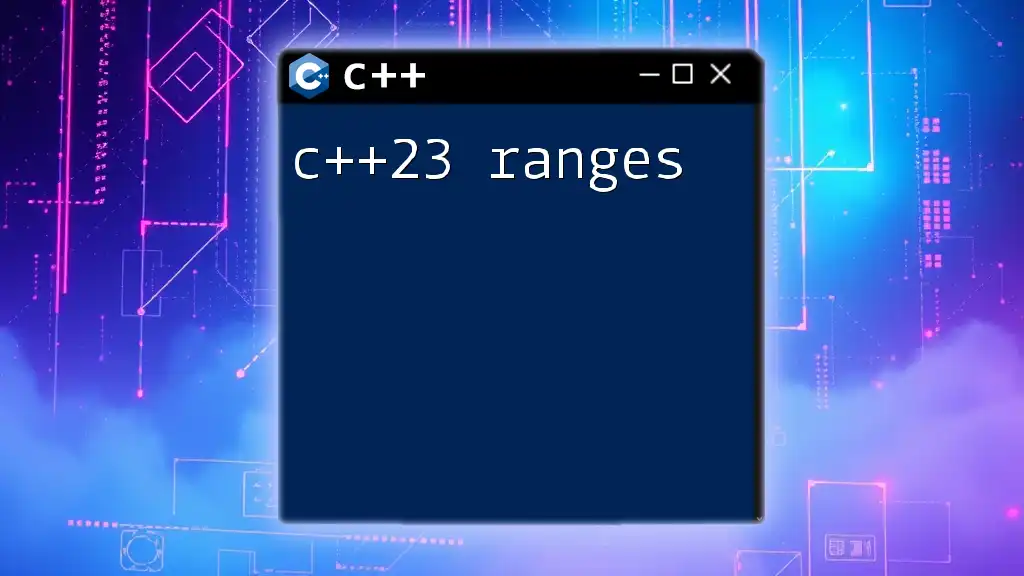
Conclusion
In summary, understanding how to create a C++ 2D graphics engine unlocks the potential for developing immersive and performance-oriented games. From rendering systems to input handling and advanced features like particle systems, the knowledge gained in this article lays a solid foundation for further exploration and game development.
As you continue to learn, be sure to leverage the vast array of resources available, from books to community forums. Don’t hesitate to experiment and build simple projects, as practical application is key to mastering game development. Happy coding!