C++ in Unreal Engine is a powerful programming tool that allows developers to create game mechanics and functionality, enhancing the interactive experience of their projects.
Here’s a simple example of how to define a class in Unreal Engine using C++:
#include "GameFramework/Actor.h"
#include "MyActor.generated.h"
UCLASS()
class MYPROJECT_API AMyActor : public AActor
{
GENERATED_BODY()
public:
AMyActor();
protected:
virtual void BeginPlay() override;
public:
virtual void Tick(float DeltaTime) override;
};
Overview of Unreal Engine
Unreal Engine is a powerful and versatile game development platform known for its impressive graphics capabilities and robust toolset. From creating high-fidelity games to architectural visualizations, Unreal provides developers with a wide array of features to bring their creative visions to life. C++ in Unreal Engine plays a critical role by enabling developers to optimize performance and create complex gameplay mechanics.
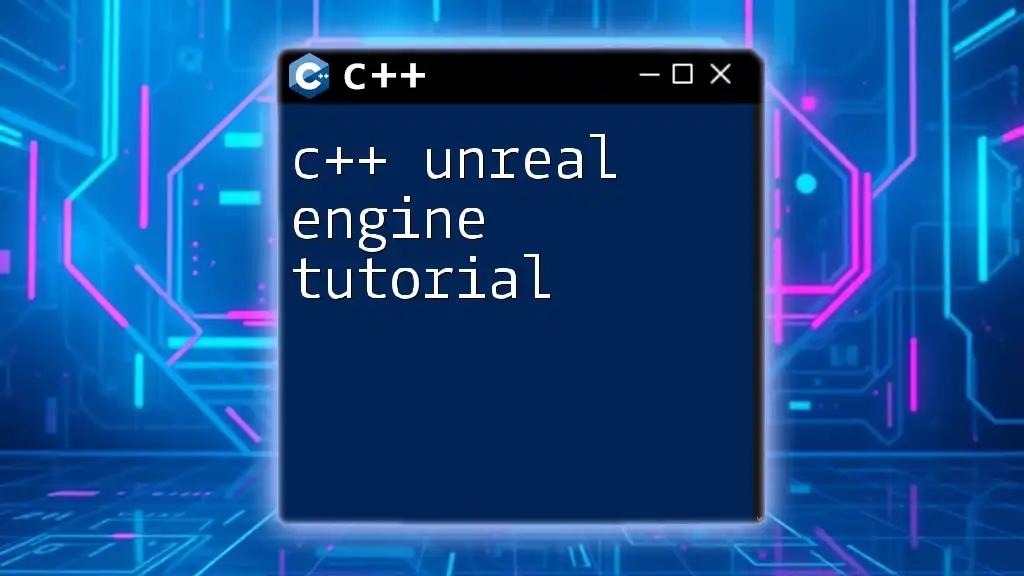
Why Choose C++ for Unreal Engine?
Choosing C++ for Unreal Engine offers significant benefits for developers looking for enhanced performance and flexibility. Unlike BluePrints, which are designed for rapid prototyping and visual programming, C++ allows for greater control over system resources and the ability to implement low-level optimizations.
Performance Benefits
C++ compiles to native code, providing superior performance compared to interpreted languages. This is particularly important in game development, where frame rates and responsiveness can make or break the player experience.
Control and Flexibility
With C++, developers have the ability to leverage advanced programming concepts, such as memory management and complex algorithms, giving them the power to create intricate gameplay systems that are not constrained by the limitations of a visual interface.
Comparison with Blueprints
While Blueprints are user-friendly and ideal for non-programmers or rapid game design, C++ shines in scenarios where performance is critical. Understanding how to use both in tandem can greatly enhance a project's success.
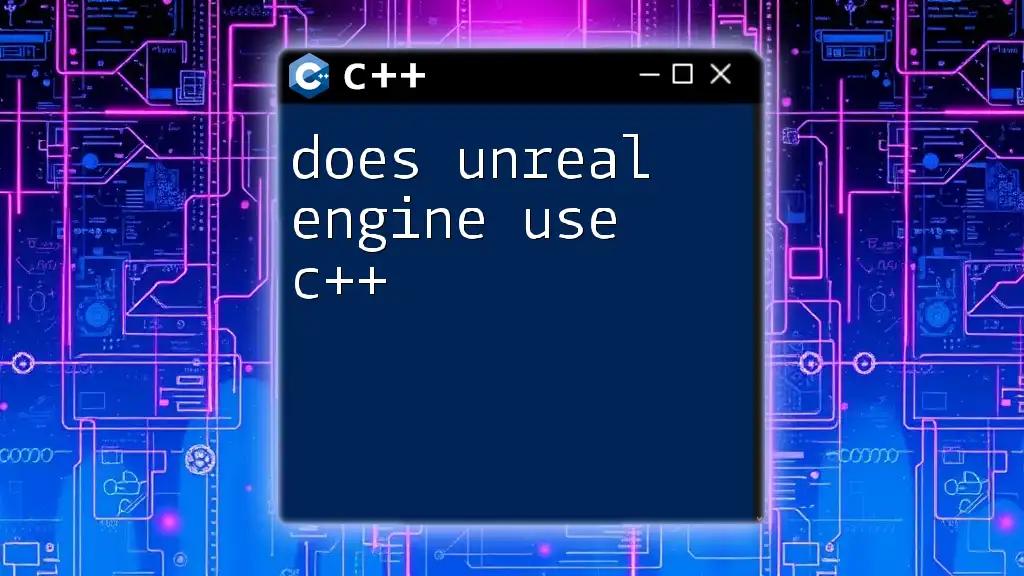
Getting Started with C++ in Unreal Engine
Setting Up Your Development Environment
First, you need to install Unreal Engine from the Epic Games Launcher. Make sure to have Visual Studio (with the C++ workload) installed on your system. Proper configuration will ensure a smooth development experience.
Creating Your First C++ Project
To create a new project, open the Unreal Engine editor and select "New Project." Choose the C++ template option and follow the prompts. You can choose from various templates depending on the type of game you're developing.
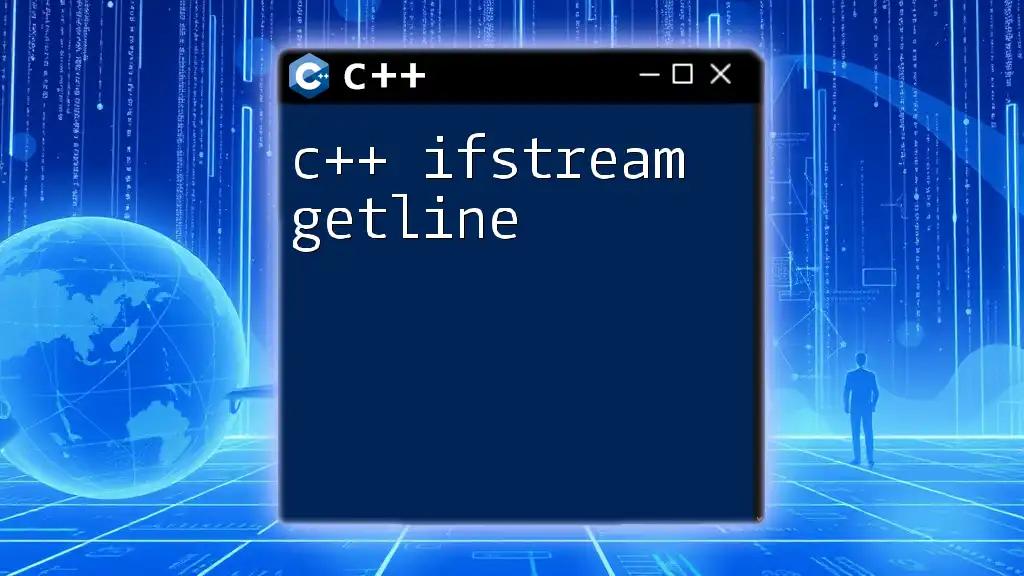
Understanding the C++ Class Structure in Unreal Engine
Basic C++ Concepts
At its core, C++ programming is based on the principles of object-oriented programming. Understanding concepts like classes, objects, inheritance, and polymorphism is crucial when working with Unreal Engine.
Unreal Engine’s Class Hierarchy
Unreal Engine employs a unique class hierarchy that emphasizes components. The UObject class is the base class for most objects within the engine, while AActor is a special type of UObject that can be placed in a level. Components are reusable pieces of functionality that can be added to actors, allowing for a modular approach to game design.
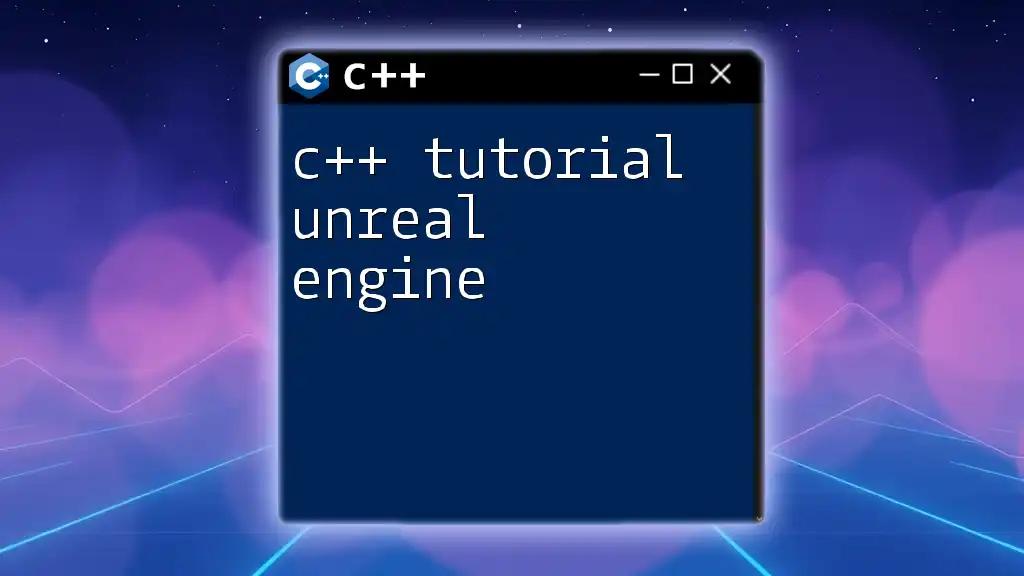
Writing Your First C++ Class
Creating a New C++ Class
Creating a new class in Unreal Engine is straightforward. Once your project is opened, right-click on the Content Browser, navigate to C++ Class, and select Actor to derive from AActor.
Defining Class Properties and Methods
In your new class, start by defining class properties and methods. Use the UPROPERTY() macro to make variables accessible within the editor and to Blueprints.
Here’s an example of a basic class:
class AMyActor : public AActor {
GENERATED_BODY()
public:
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "MyActor")
float MyFloatValue;
void MyFunction() {
// Custom functionality goes here
}
};
In this example, `MyFloatValue` is a public property that can be set in the editor, making it easily adjustable during game development.
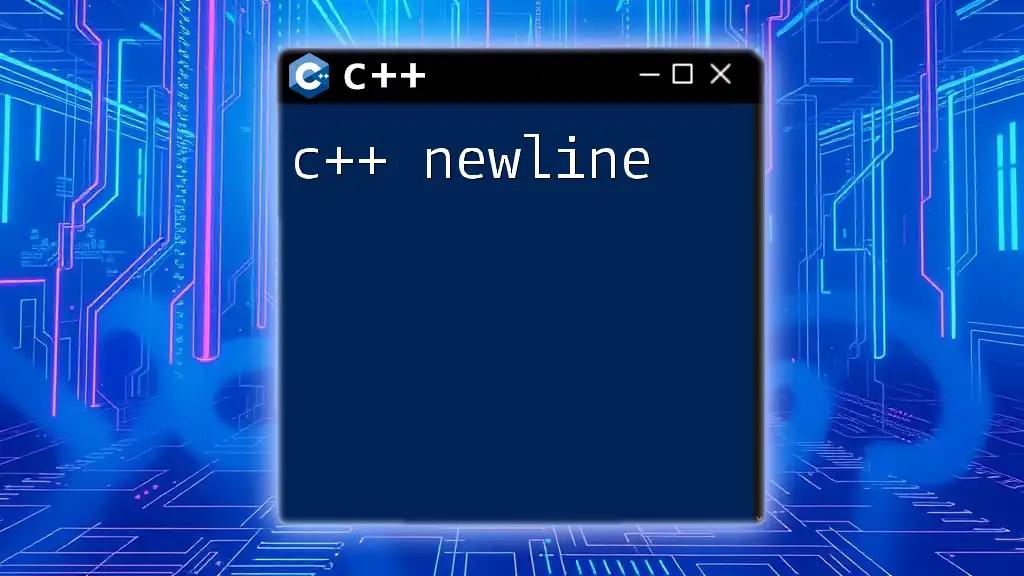
Integrating C++ with Unreal Engine’s Systems
Understanding Unreal Engine's Reflection System
Unreal Engine has a built-in reflection system that allows for runtime type information. This is particularly useful when working with Blueprints, as it enables you to create classes and functions that are visible and usable within the Blueprint editor.
Working with Unreal Engine Input System
Implementing player input effectively is crucial for gameplay. In your class, you can set up inputs via the Project Settings under Input. Here's a basic demonstration:
void AMyActor::SetupPlayerInputComponent(UInputComponent* PlayerInputComponent) {
Super::SetupPlayerInputComponent(PlayerInputComponent);
PlayerInputComponent->BindAction("Jump", IE_Pressed, this, &AMyActor::Jump);
}
This example binds an action to a player input and integrates it into the game’s control system.
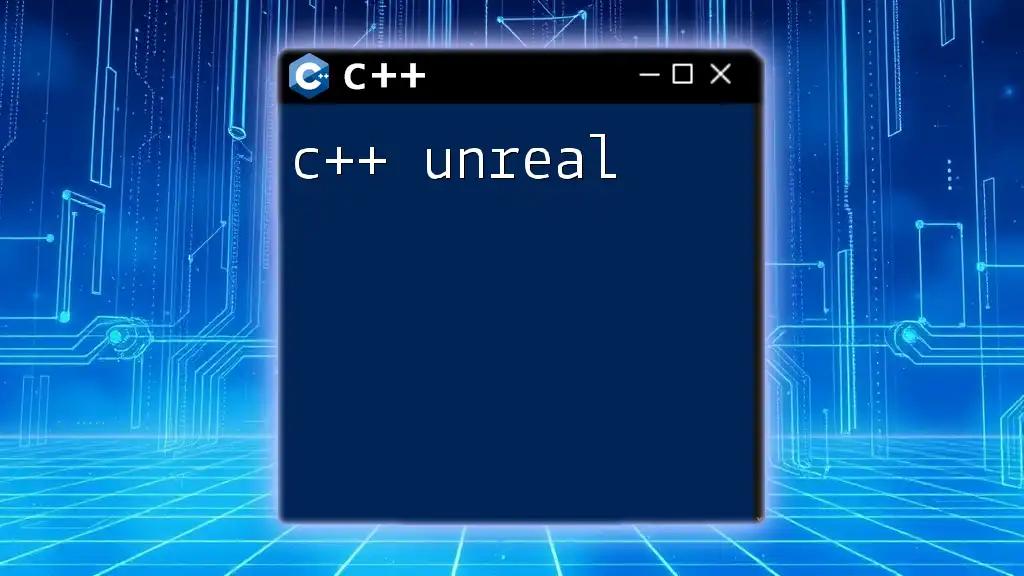
Gameplay Programming with C++
Basic Game Loop and Tick Function
The game loop is where the game's ongoing operations occur. In Unreal Engine, you can override the Tick function to implement per-frame updates.
Here’s how you can start implementing your `Tick` function:
void AMyActor::Tick(float DeltaTime) {
Super::Tick(DeltaTime);
// Insert code that updates the actor every frame
}
Any custom game logic you want executed every frame can be inserted here.
Creating Interactions and Event Systems
Interactivity is one of the cornerstones of gameplay. Unreal Engine allows you to define custom interactions using delegates and events.
Here’s an example of using an event:
void AMyActor::BeginPlay() {
Super::BeginPlay();
OnClicked.AddDynamic(this, &AMyActor::HandleClicked);
}
In this code snippet, you set up an event handler for when the actor is clicked, enhancing player interactions significantly.
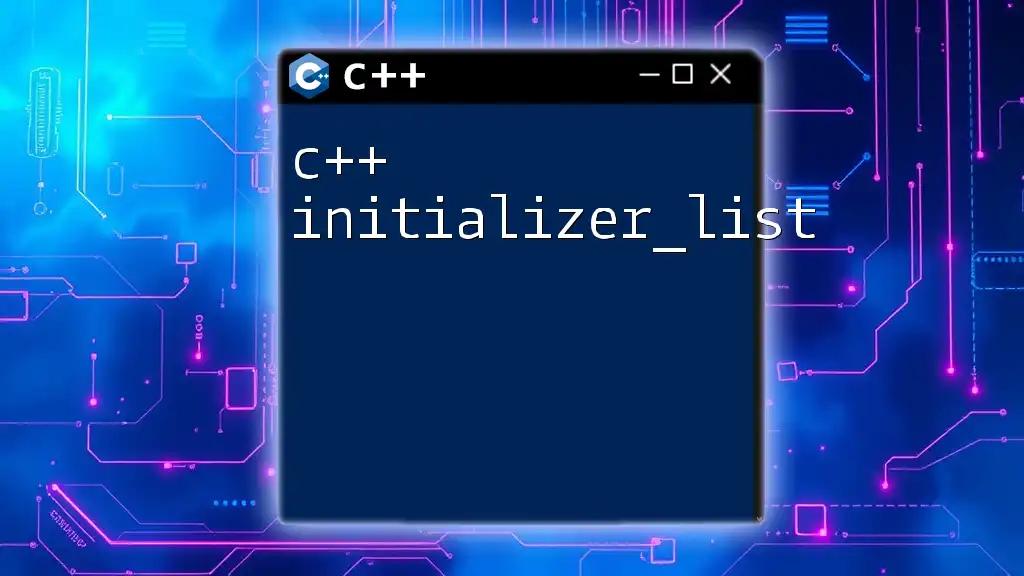
Debugging and Optimizing C++ in Unreal Engine
Best Practices for Debugging C++ Code
Debugging C++ code in Unreal Engine can be accomplished using Visual Studio’s powerful debugging tools. Setting breakpoints and inspecting variables can significantly speed up the debugging process. Log messages can also be incredibly helpful for understanding what's happening at runtime.
Performance Optimization Techniques
Optimization is key in game development, especially when working with intensive game mechanics. Profiling your code can help identify bottlenecks. Unreal Engine provides tools such as the Profiler and Memory Insights to aid in this process.
Consider implementing efficient memory management practices, such as using object pooling for frequently spawned actors, to enhance your game's performance.
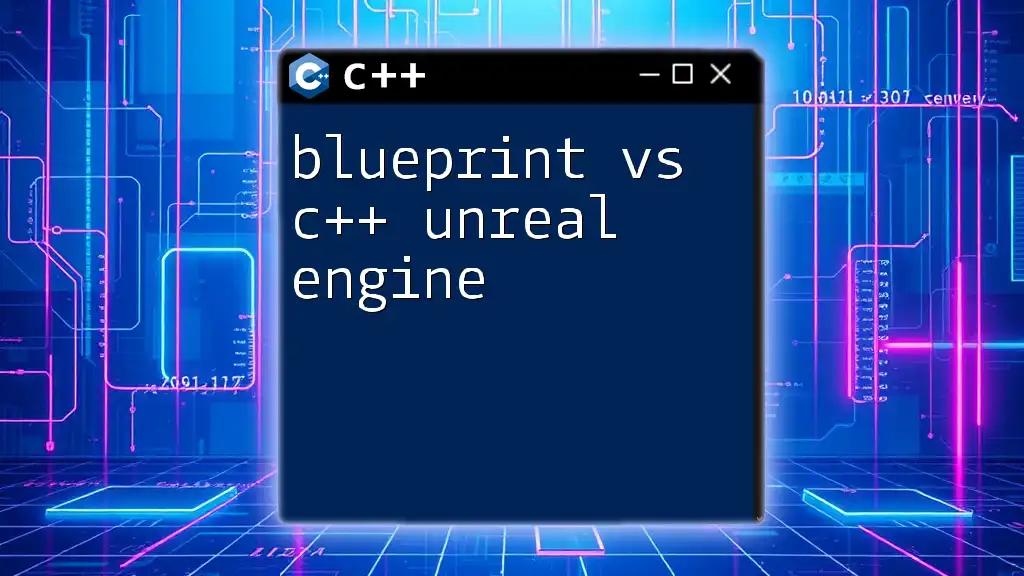
Common Challenges When Using C++ in Unreal Engine
Navigating the Learning Curve
Transitioning from Blueprints to C++ can be a steep learning curve for many developers. It's essential to grasp the fundamentals of C++ first before diving into Unreal-specific implementations. Seeking out resources, such as tutorials or community forums, can enlighten your C++ journey.
Interfacing with Blueprints
C++ and Blueprints work best in conjunction. You can easily call C++ functions from Blueprints by marking them with the `UFUNCTION(BlueprintCallable)` macro, enabling seamless interaction between the two systems.
UFUNCTION(BlueprintCallable, Category = "MyActor")
void MyBlueprintFunction();
This will expose your C++ function to the Blueprint editor, allowing designers access to your code's functionality.
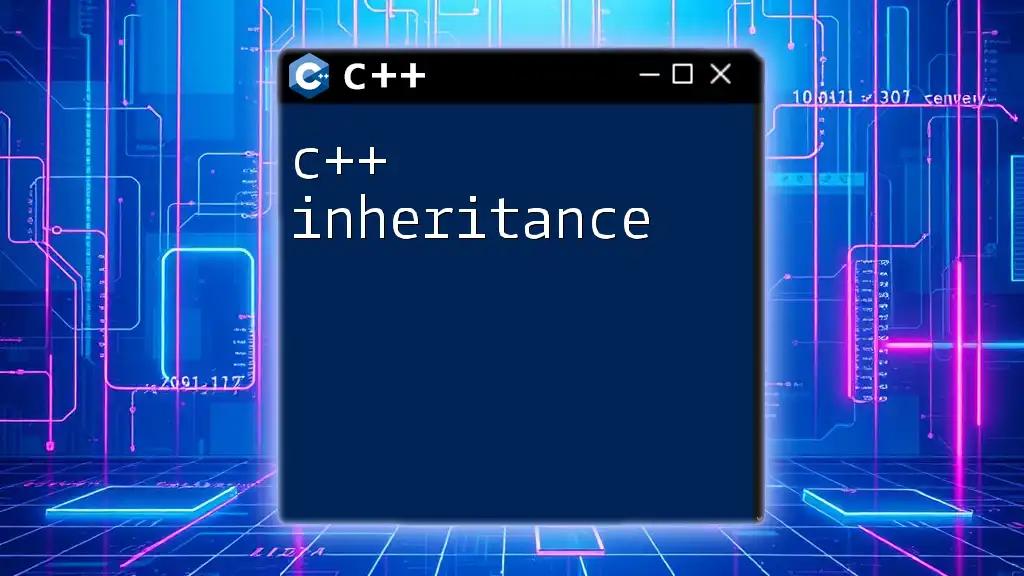
Conclusion
C++ in Unreal Engine represents a powerful combination that allows developers to create high-performance, complex gameplay experiences. Each concept detailed in this guide will help you become proficient in utilizing C++ alongside Unreal Engine's extensive toolset.
In the ever-evolving landscape of game development, mastering C++ not only enhances your skills but also opens up numerous opportunities in the gaming industry. Don’t hesitate to dive deeper into each topic discussed, engage with communities, and experiment with your own projects to unlock the true potential of C++ in Unreal Engine.
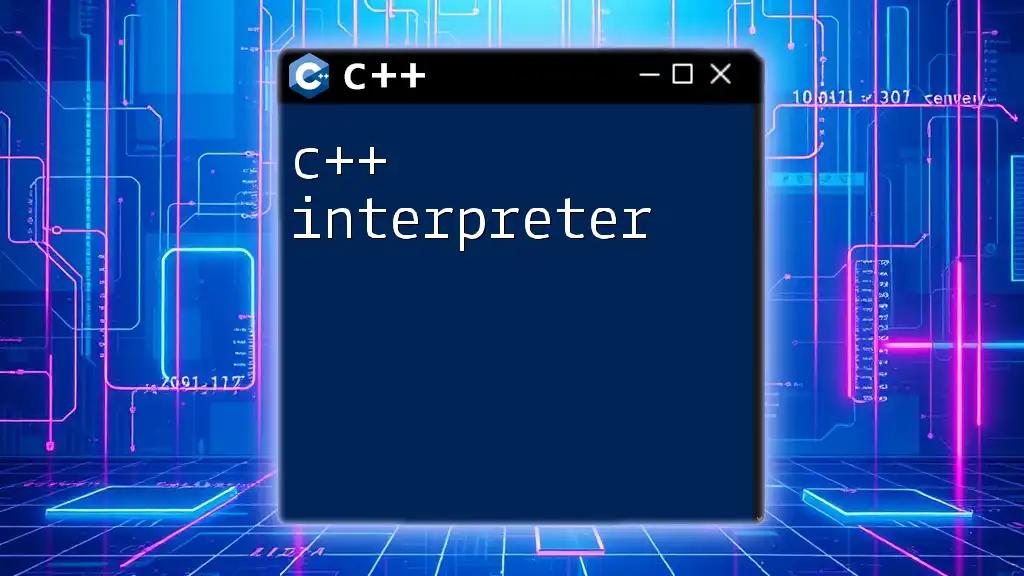
Call to Action
Join our C++ in Unreal Engine community for ongoing support and learning. Signing up will grant you access to exclusive resources, courses, and like-minded individuals aiming to excel in game development. Don’t miss out on future updates and a wealth of knowledge that can help you refine your skills!