Yes, Unreal Engine uses C++ as its primary programming language, allowing developers to create high-performance game mechanics and systems.
#include "GameFramework/Actor.h"
class AMyActor : public AActor
{
public:
AMyActor();
virtual void BeginPlay() override;
virtual void Tick(float DeltaTime) override;
};
Understanding Unreal Engine
What is Unreal Engine?
Unreal Engine is a powerful game development platform created by Epic Games. Initially launched in 1998, it has evolved significantly over the years, becoming a go-to choice for both indie creators and large studios. This engine enables developers to create high-quality games, simulations, and virtual experiences across various platforms, including PC, console, and VR. Its expansive capabilities have solidified its reputation in the gaming industry.
Key Features of Unreal Engine
Unreal Engine boasts a wide array of features that contribute to its popularity among developers:
-
High-fidelity graphics: The engine is renowned for its stunning visual capabilities. With real-time rendering of high-quality textures and materials, Unreal Engine empowers developers to create incredibly immersive worlds.
-
Blueprint Visual Scripting: This intuitive system allows developers to design game mechanics without needing extensive programming knowledge. Blueprints serve as a powerful visual scripting alternative but run on C++ under the hood, demonstrating how critical C++ is to the operation of Unreal Engine.
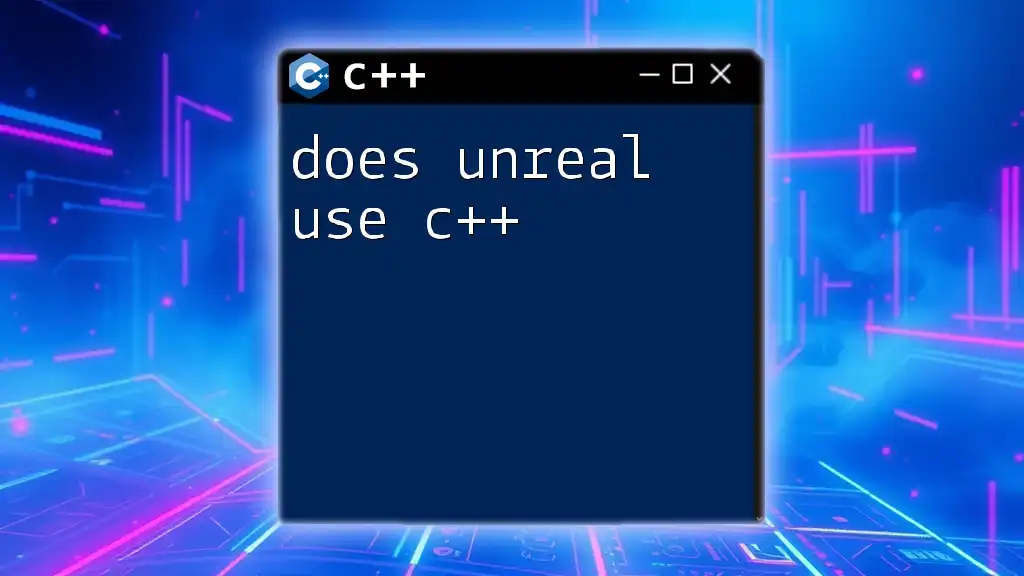
The Role of C++ in Unreal Engine
Why C++?
C++ is a crucial component of Unreal Engine for several reasons:
-
Performance reasons: One of the main advantages of C++ is its high performance. C++ provides developers control over system resources, allowing for better memory management and faster execution speed. This performance edge is essential in game development, where every millisecond counts.
-
Control over system resources: C++ offers low-level access to memory manipulation, which means developers can optimize their games more effectively, tailoring them to deliver the best possible user experience.
C++ as the Core Language
Unreal Engine is fundamentally built using C++. The core components, such as game logic, physics, and rendering systems, are all implemented in C++. This underlying use of C++ explains why so much of the engine’s functionality is accessible when programming in this language.
For example, your game’s characters, environments, and gameplay mechanics are primarily all crafted and controlled via C++ code, allowing for intricate, bespoke systems that tailor the experience to your game's needs.
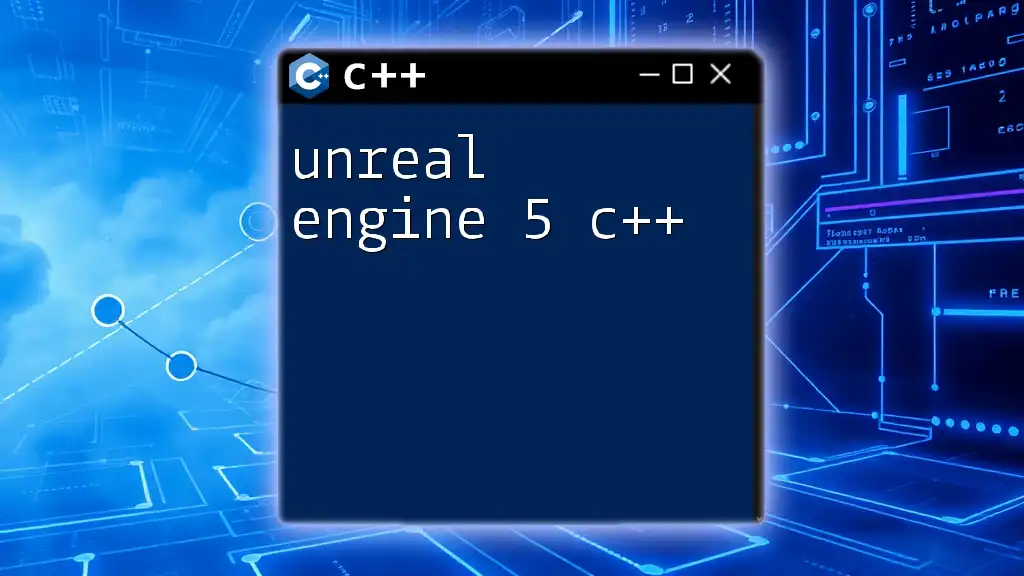
C++ vs. Blueprints
Comparison Overview
When evaluating whether "does Unreal Engine use C++," it's crucial to consider the relationship between C++ and the Blueprint system.
-
C++: Programming in C++ can offer more precision and performance. However, it also requires a deeper understanding of coding principles and potentially has a steeper learning curve.
-
Blueprints: This visual scripting language allows for a more manageable entry point for designers and programmers alike. While Blueprints are less performant than C++, they make rapid prototyping and iterating much easier.
When to Use C++ vs. Blueprints
In practical scenarios, knowing when to use C++ versus Blueprints can maximize efficiency in your game development process.
- Use cases for C++:
- Custom game mechanics that require refined control, such as implementing complex AI behaviors or advanced player movement systems.
- Use cases for Blueprints:
- Rapid prototyping of gameplay ideas, where quick iterations are crucial. For instance, tweaking game mechanics or level design might be more convenient using Blueprints.
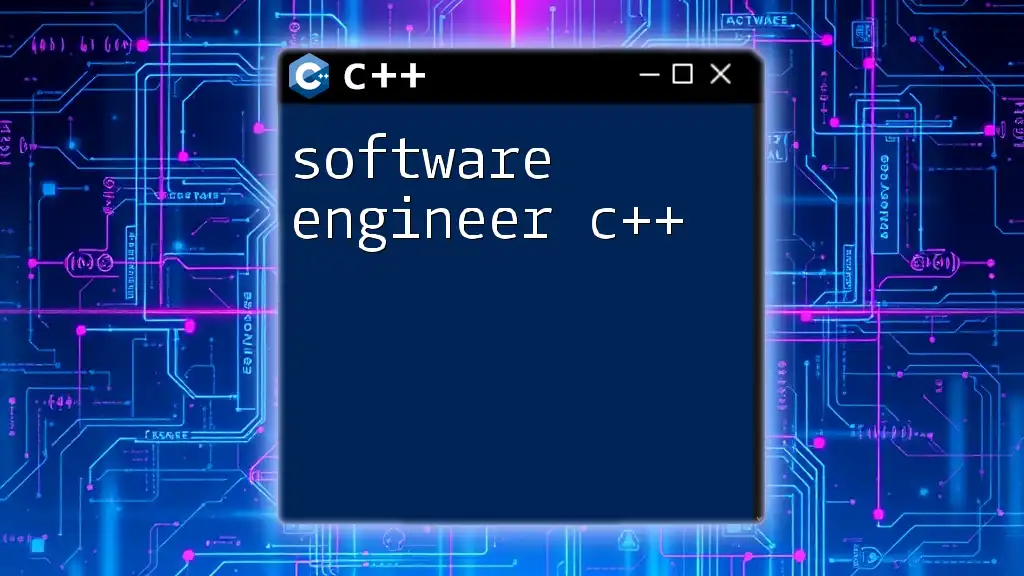
Code Snippets and Examples
Basic C++ Class in Unreal Engine
Creating C++ classes in Unreal is fundamental to developing game features. Here's an example of a simple actor class:
#include "GameFramework/Actor.h"
#include "MyActor.generated.h"
UCLASS()
class MYGAME_API AMyActor : public AActor
{
GENERATED_BODY()
public:
AMyActor();
virtual void BeginPlay() override;
virtual void Tick(float DeltaSeconds) override;
};
In this snippet, we are creating a new actor class called `AMyActor`. The `GENERATED_BODY()` macro facilitates the Unreal Engine's reflection system, ensuring our class integrates smoothly with the engine.
Creating a Simple Function in C++
Let’s delve into a simple function to control player movement in C++:
void AMyCharacter::MoveForward(float Value)
{
if (Value != 0.0f)
{
AddMovementInput(GetActorForwardVector(), Value);
}
}
In this example, the method `MoveForward` checks if a value has been provided. If it has a non-zero value, it uses `AddMovementInput` to move the character forward based on the actor's orientation. This level of control is why C++ plays a central role in Unreal Engine's game development process.
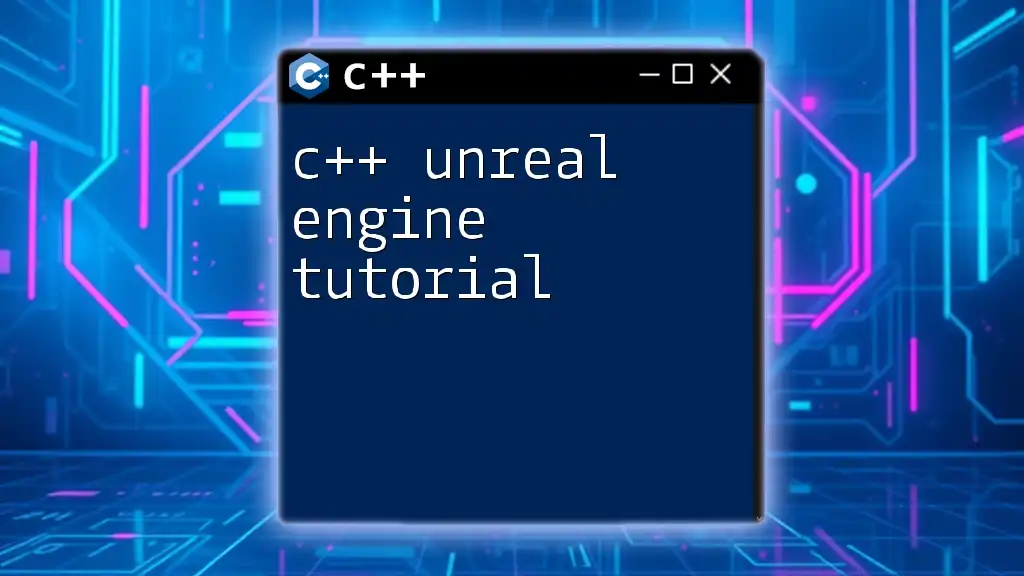
Real-World Usage of C++ in Unreal Engine
Case Studies
Many high-profile games have harnessed the power of Unreal Engine combined with C++. Titles such as Fortnite and Gears of War illustrate the engine's capabilities in delivering complex, high-performance gaming experiences. Developers can see directly how C++ contributes to game development success through these examples.
Community and Open Source Contributions
The Unreal Engine community is vibrant, with many developers actively contributing to an ever-expanding codebase. Open-source plugins, game mechanics, and modifications built using C++ allow others to enhance their projects with innovative features. This collaborative environment shows how C++ is not merely a language but a pivotal element of the Unreal development ecosystem.
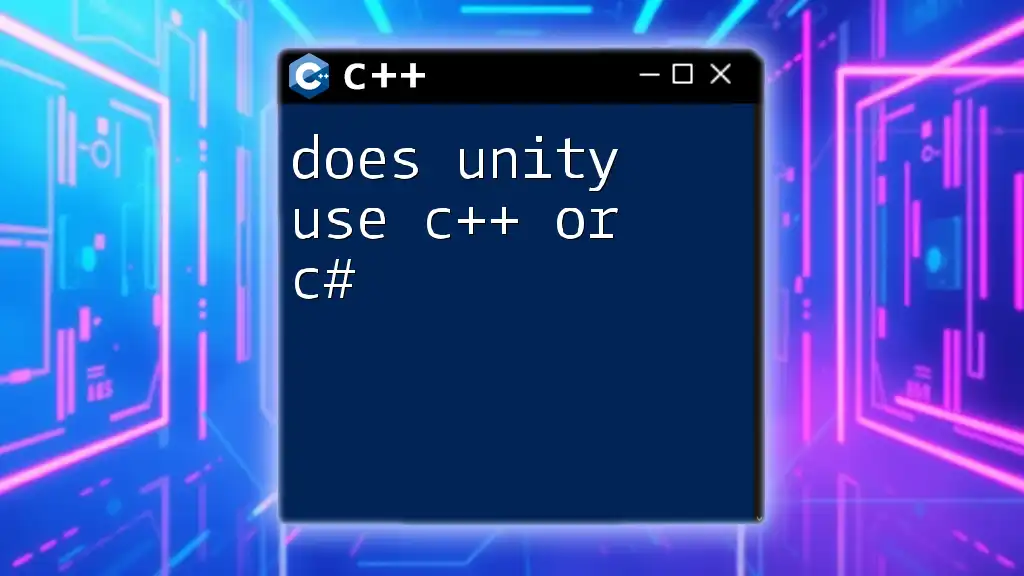
Learning C++ for Unreal Engine
Resources for Beginners
For those looking to embark on the journey of learning C++, various resources are available:
- Online courses: Platforms such as Udemy and Coursera offer courses dedicated to C++ and Unreal Engine.
- Books: Titles like Beginning C++ Through Game Programming provide a comprehensive starting point.
- Video tutorials: YouTube channels like Unreal Engine’s official channel cover a wealth of topics, including C++ scripting.
Common Challenges in Learning C++
Learning C++ can present challenges, including:
- Understanding complex syntax and programming paradigms.
- Navigating Unreal's extensive API and integrating C++ code within the editor.
However, by focusing on practical examples and continually building projects, beginners can overcome these hurdles effectively. Utilizing community forums, such as Unreal Engine's own forums or Stack Overflow, can also provide support when facing specific issues.
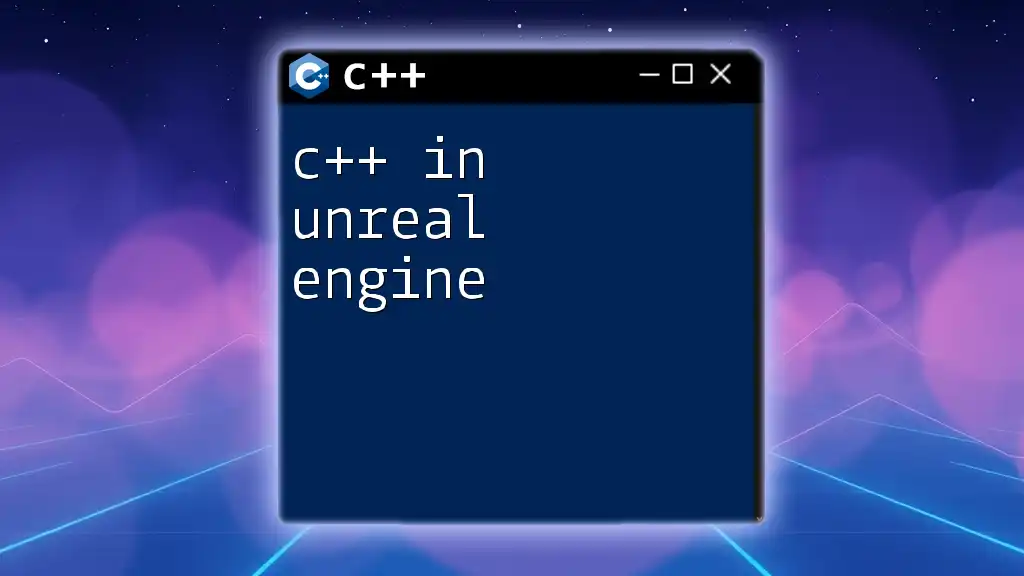
Conclusion
In summary, C++ is an integral part of Unreal Engine, powering not only the engine's core functionalities but also enabling developers to create complex, high-performance games. It stands as a testament to the capabilities of C++ in optimizing game experiences while offering precise control over game mechanics.
As the gaming industry continues to evolve, understanding C++ and its applications within Unreal Engine will become increasingly valuable. Taking the next steps toward learning C++ will open countless opportunities in game development, providing the tools you need to create engaging experiences in your games.