Yes, Unreal Engine extensively uses C++ for game development, allowing developers to create high-performance gameplay mechanics and customize gameplay features.
Here's a simple example of a C++ class in an Unreal project:
#include "GameFramework/Actor.h"
#include "MyActor.generated.h"
UCLASS()
class MYGAME_API AMyActor : public AActor
{
GENERATED_BODY()
public:
AMyActor();
protected:
virtual void BeginPlay() override;
public:
virtual void Tick(float DeltaTime) override;
};
What is Unreal Engine?
Unreal Engine is a powerful and versatile game development platform created by Epic Games. It allows developers to create stunning visuals, immersive environments, and complex gameplay mechanics across various platforms, including PC, consoles, and mobile devices. With features like real-time rendering, physics simulation, and expansive asset libraries, Unreal Engine has become a staple in both AAA game studios and indie projects alike.
The engine's evolution began in 1998 with the release of Unreal, and it has progressed through numerous iterations, culminating in the latest version, Unreal Engine 5, which pushes the boundaries of realism and interactivity in gaming.
Popular Use Cases of Unreal Engine
- Game Development: From colorful indie titles to massive blockbuster franchises, Unreal Engine serves as a foundation for creating immersive gameplay experiences.
- VR and AR Applications: Its capabilities extend beyond traditional gaming, reaching applications in virtual reality and augmented reality, making it a favored choice in architectural visualization, training simulations, and other interactive experiences.
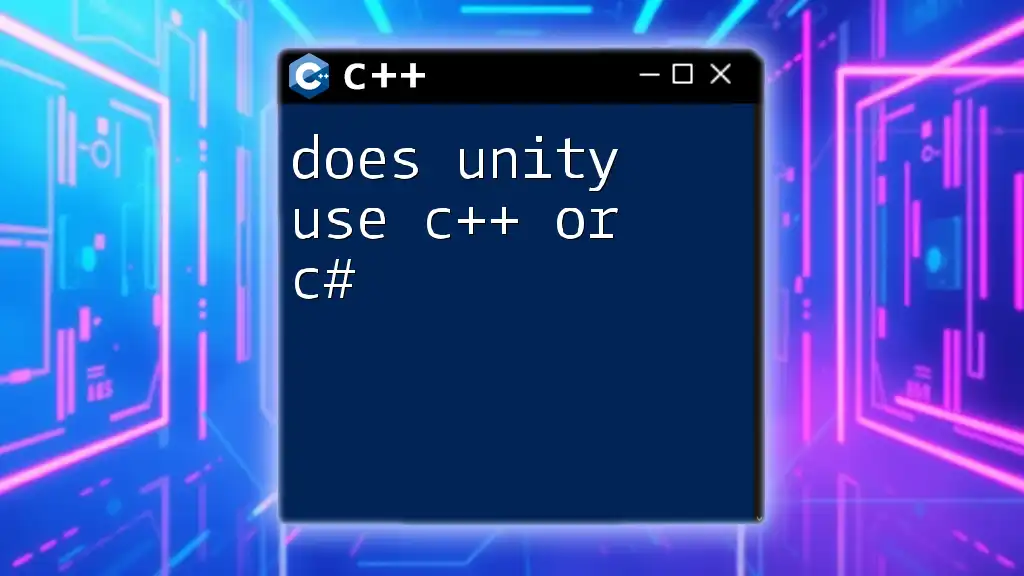
The Role of C++ in Unreal Engine
Why C++?
C++ plays a critical role in Unreal Engine for several reasons:
-
Performance Advantages: C++ allows developers to write low-level code that can interact closely with system resources, providing greater control over memory management and optimizing game performance. This is crucial for rendering graphics efficiently and executing complex gameplay mechanics.
-
Industry Standards: As one of the most widely used programming languages in game development, C++ is familiar to many professionals in the industry. This familiarity helps ensure smooth collaboration among developers working on large projects.
-
Comparison with Other Languages: While Unreal Engine offers Blueprint, a visual scripting language that caters to non-programmers, C++ remains essential for data-driven design and complex systems, offering capabilities that go beyond what Blueprint can achieve.
C++ as Unreal's Core Language
C++ serves as the backbone of Unreal Engine. Its architecture is designed to leverage the language's strengths, allowing developers to write robust systems that are integral to the game's performance and functionality. C++ code typically handles low-level engine programming as well as high-level gameplay logic, making it indispensable for comprehensive game development.
How C++ Integrates with Unreal Engine
Unreal Engine utilizes a unique build system, detailed in the Unreal Build Tool (UBT), to compile C++ code. Understanding how this integration works is vital for any developer working with Unreal Engine.
- Binding C++ with Unreal's Environment: C++ classes are utilized as base components for game elements, and Unreal provides a specific compilation process that produces optimized executables.
- Reflection and Serialization: Unreal’s architecture features reflection capabilities, allowing properties to be edited in the editor and serialized for saving and loading game states— valuable for both game designers and developers.
The Unreal Object Model
In Unreal Engine, two crucial concepts are UObjects and AActors.
-
UObjects: These are foundational building blocks for creating gameplay elements. Each UObject can have properties and methods, and developers can create their own UObjects by subclassing the UObject class.
-
AActors: These represent objects that can be placed in the game world; they include components and define aspects such as behavior and rendering.
An example of creating a simple UObject might resemble:
#include "MyObject.h"
#include "CoreMinimal.h"
UMyObject::UMyObject()
{
// Constructor logic here
}
This illustrates declaring a custom UObject class that can then be used within Unreal.
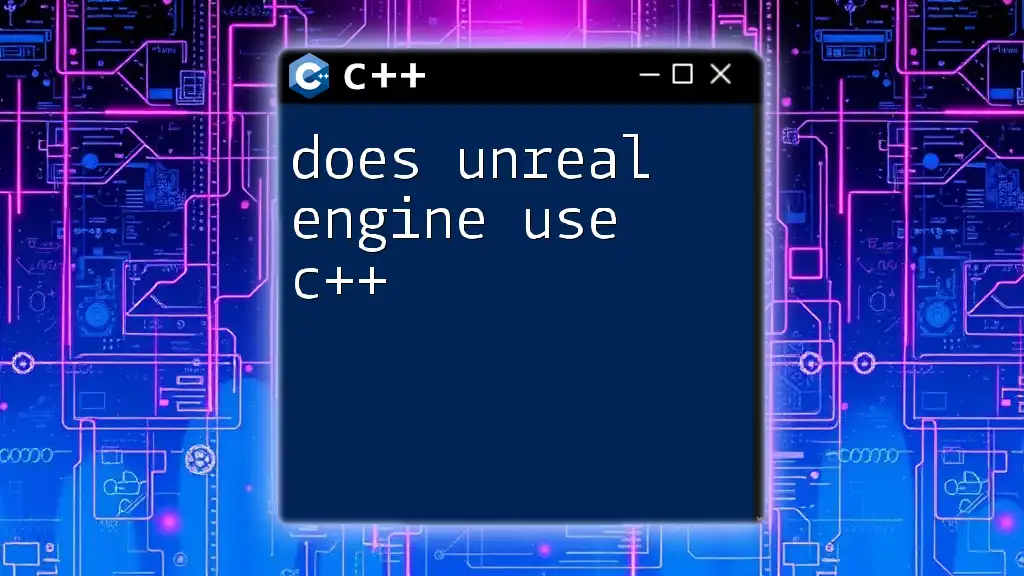
Getting Started with C++ in Unreal Engine
Setting Up Your Environment
To effectively start using C++ in Unreal Engine, you'll need to prepare your development environment:
- Install Unreal Engine: Download it via the Epic Games Launcher and choose a version that suits your projects.
- Set Up Visual Studio or Xcode: If you're on Windows, Visual Studio is the go-to IDE. For macOS, Xcode is required. Ensure that you include C++ development tools during installation.
- Configuring Your First C++ Project: Launch Unreal Editor, create a new project, and select the C++ option.
Creating Your First C++ Class
When you're ready to dive into coding, creating a new C++ class is your next step.
Walkthrough: Creating a New C++ Class in Unreal
- Open Unreal Engine and create or open a project.
- Navigate to the “File” menu, select “New C++ Class” and choose a parent class (like Actor).
- Name your class and click "Create Class".
The code snippet below depicts a basic Actor class:
#include "MyActor.h"
#include "GameFramework/Actor.h"
// Constructor
AMyActor::AMyActor()
{
PrimaryActorTick.bCanEverTick = true;
}
// Called every frame
void AMyActor::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
}
// Example of something happening on BeginPlay
void AMyActor::BeginPlay()
{
Super::BeginPlay();
}
Compiling and Running Your C++ Code
Once your class is defined, compiling your code within Unreal Engine is essential. You can do this from the editor or via your chosen IDE.
If you encounter compilation errors, common issues may involve missing headers or incorrect method signatures. Checking the output log in Unreal Engine can provide valuable hints on what needs fixing.
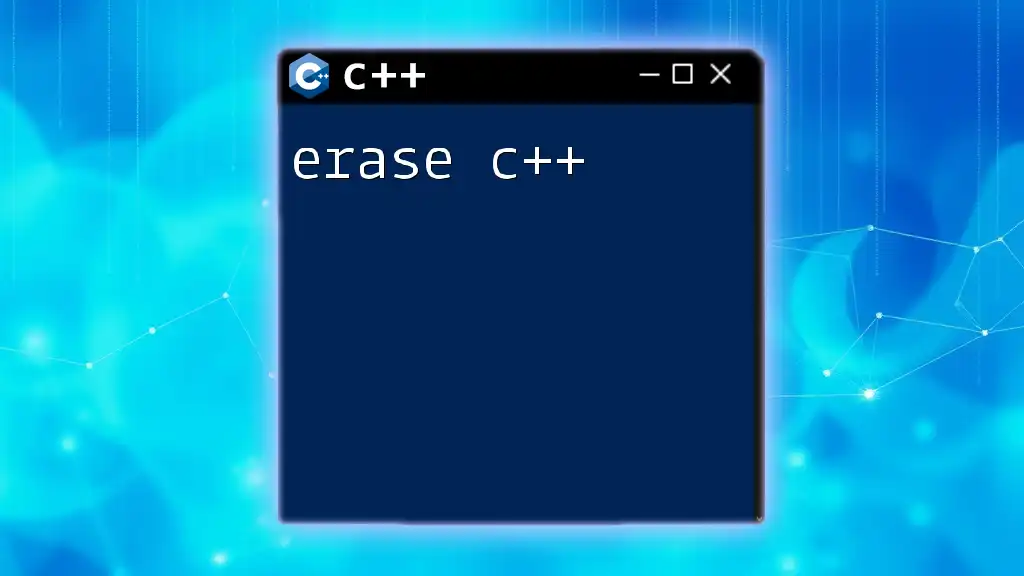
C++ vs Blueprint in Unreal Engine
Overview of Blueprint
Blueprint is Unreal Engine's visual scripting system. It offers an alternative to traditional coding, making it accessible for designers and developers who prefer a more visual approach. Blueprint enables rapid prototyping, speeds up development, and allows for easier debugging.
When to Use C++ vs Blueprints
While Blueprints are immensely useful, certain scenarios necessitate the robust features of C++. Consider using C++ in the following cases:
- Performance-Critical Systems: If your gameplay mechanics require low latency, like running AI algorithms, C++ provides the necessary performance edge.
- Complex Gameplay Logic: For intricate mechanics or when working with large codebases, C++ can offer clearer structure and maintainability compared to a visual graph.
Real-world projects often leverage both: using C++ for foundational systems and Blueprints for rapid iteration on gameplay design.
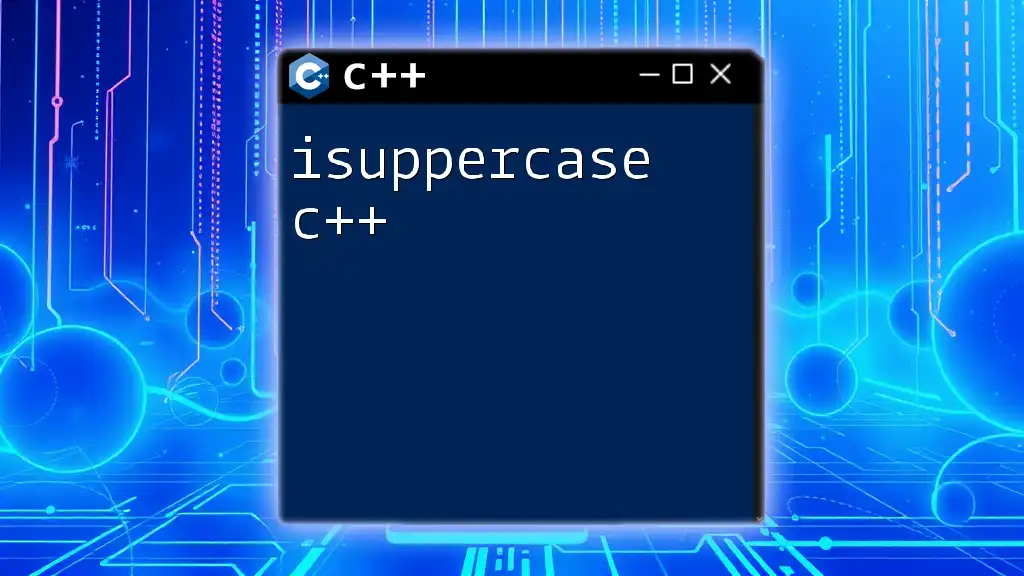
Advanced C++ Concepts in Unreal Engine
Inheritance and Polymorphism
Unreal Engine fully embraces object-oriented programming, allowing developers to create complex hierarchies. C++ enables inheritance and polymorphism, letting developers create derived classes that inherit properties and methods from base classes.
An illustrative code example:
class ABaseCharacter : public ACharacter
{
public:
virtual void Move();
};
class APlayerCharacter : public ABaseCharacter
{
public:
void Move() override
{
// Custom player movement logic
}
};
Templates and Generics
C++ supports templates, facilitating the creation of functions or classes that work with any data type. This is particularly useful for creating reusable game logic, as code can adapt to various input types without redundancy.
Error Handling in C++
Robust error handling is vital for maintaining gameplay stability. Using try-catch blocks, developers can gracefully manage exceptions while keeping an eye on the Unreal Engine's log for error messages.
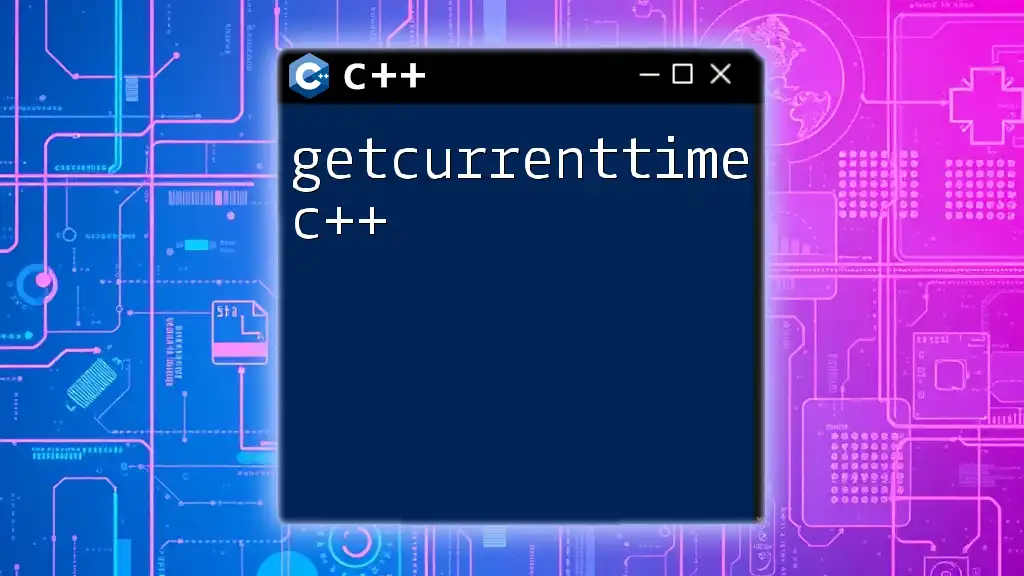
Best Practices for C++ Development in Unreal
Maintaining clean and organized code is essential in C++ development:
- Code Organization and Project Structure: Always maintain a consistent folder structure and naming conventions for your classes and assets.
- Using Comments and Documentation Effectively: Well-documented code helps others understand your work and simplifies future modifications.
- Collaborating in a Team Environment: Ensure that everyone adheres to coding standards and performs regular code reviews to foster a productive team dynamic.
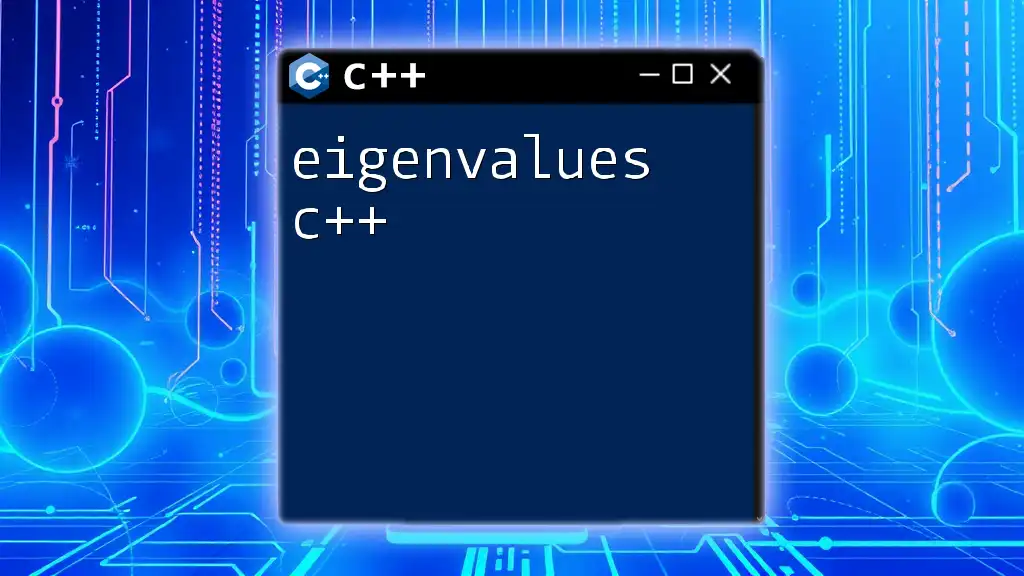
Resources for Learning C++ in Unreal Engine
Aspiring developers can access a wealth of resources for learning C++ in Unreal Engine:
- Books: Titles like “Programming in Unreal” and “Learning C++ by Making Games” provide structured learning paths.
- Online Courses: Platforms like Udemy and Coursera offer C++ courses specifically tailored to Unreal Engine.
- Official Unreal Engine Documentation: The Epic Games website hosts comprehensive documentation, covering every aspect of Unreal Engine and C++ usage.
- Community Forums and Support Channels: Engaging with the Unreal Engine community on platforms like Stack Overflow or the Unreal Engine forums can provide invaluable insights and assistance.
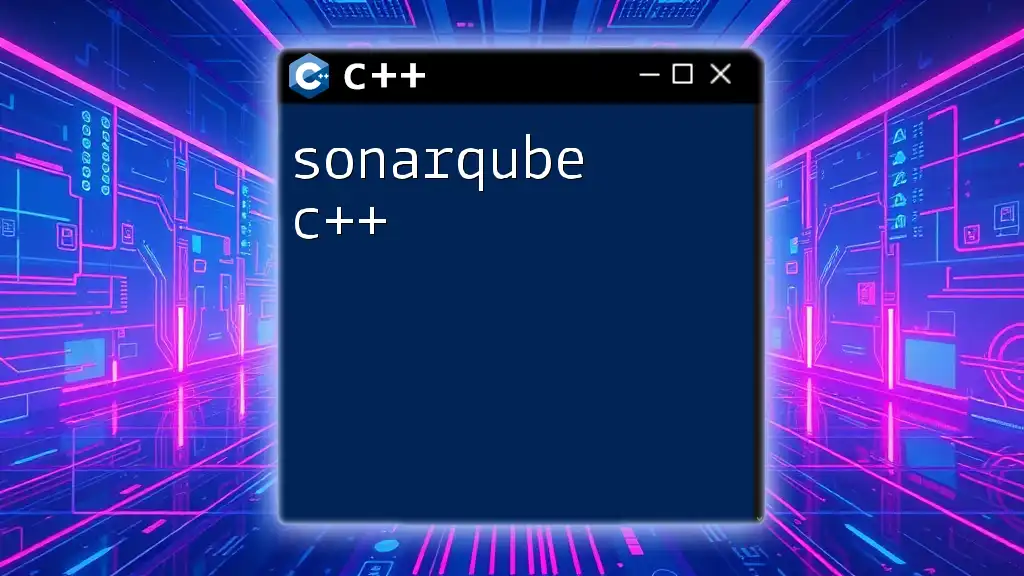
Conclusion
Understanding does Unreal use C++ is essential for anyone looking to make a mark in game development with Unreal Engine. The combination of C++'s performance and Unreal's capabilities provides a powerful toolkit for creating extraordinary games. As you delve into C++, remember to embrace both the challenges and the rewards of mastering this robust language.
Engage in continuous learning, practice coding, and be sure to reach out to our company for additional guidance and support as you embark on your development journey.
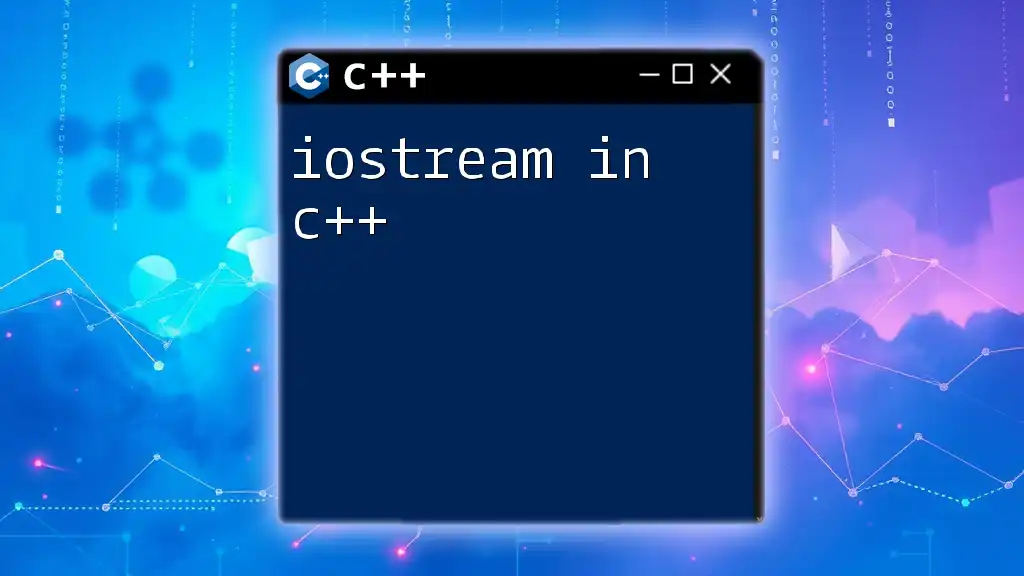
Frequently Asked Questions (FAQs)
Is C++ necessary for Unreal Engine?
While it's possible to create games using only Blueprint, C++ is essential for performance-critical elements and complex gameplay systems.
Can you create a complete game using only Blueprints?
Yes, you can create a complete game using Blueprints. However, for maximum efficiency and performance, many developers find a hybrid approach—combining C++ and Blueprints—most effective.
What are the performance impacts of using C++ in Unreal?
C++ generally offers better performance than Blueprint, especially in compute-intensive tasks. However, the performance gains depend on proper implementation, as inefficient C++ code may negate these advantages.