Type erasure in C++ is a programming technique that allows for hiding the specific type of an object while still providing a common interface for interacting with it, enabling polymorphism without the need for inheritance.
Here's a simple code snippet demonstrating type erasure using `std::function`:
#include <iostream>
#include <functional>
class AnyFunction {
public:
template<typename F>
AnyFunction(F f) : func(std::make_shared<FunctionModel<F>>(f)) {}
void operator()() const { func->call(); }
private:
struct Concept {
virtual void call() const = 0;
virtual ~Concept() = default;
};
template<typename F>
struct FunctionModel : Concept {
FunctionModel(F f) : f_(f) {}
void call() const override { f_(); }
F f_;
};
std::shared_ptr<const Concept> func;
};
int main() {
AnyFunction func = [] { std::cout << "Hello, Type Erasure!" << std::endl; };
func(); // Outputs: Hello, Type Erasure!
return 0;
}
What is Type Erasure?
Type erasure is a powerful concept in C++ that allows developers to abstract away specific type information while maintaining functionality. This enables the creation of highly flexible and reusable code. Instead of relying on templates or inheritance, type erasure provides a way of hiding specific types behind a common interface, enabling different implementations to be used interchangeably.
Why Use Type Erasure?
The primary motivations for utilizing type erasure in C++ include:
- Flexibility: It allows for a wide range of types to be treated uniformly, making it easier to write generic code.
- Abstraction: Developers can work with different types without worrying about their underlying structure, leading to cleaner and more maintainable code.
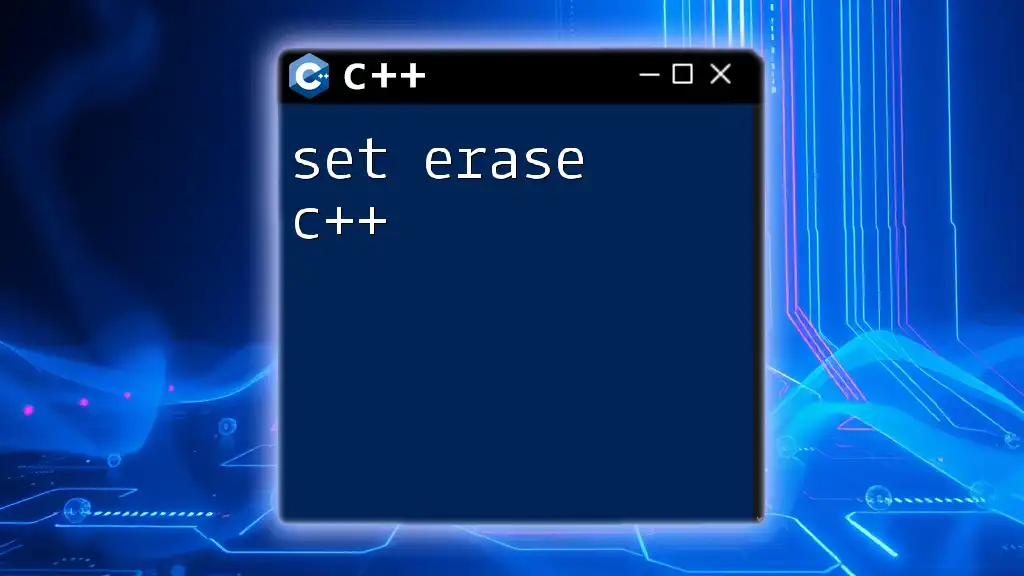
Understanding the Concepts of Type Erasure
C++ Type Erasure Basics
To grasp the significance of type erasure in C++, it is essential to understand templates and polymorphism.
- Templates provide a way to create functions and classes that can operate with any data type. While powerful, using templates can sometimes lead to code bloat if not handled properly.
- Polymorphism, particularly through inheritance, allows classes to be treated as their base class types. However, this approach can lead to rigid architectures if not designed carefully.
Advantages of Type Erasure
One of the key advantages of type erasure is its ability to simplify interfaces. Instead of requiring clients of a class to know about all possible types it can handle, a type-erased interface can present a single API for diverse implementations.
Moreover, type erasure enhances code reusability. By abstracting away concrete types, developers can create more generic components that can be reused across different parts of an application.
Disadvantages of Type Erasure
However, type erasure is not without its drawbacks. There are performance overheads associated with using dynamic dispatch, which can lead to slower execution times compared to static polymorphism. It's crucial to weigh these trade-offs when considering type erasure.
Additionally, using type erasure means losing static type information. This can complicate error handling and debugging, as developers may not have compile-time type checks available within type-erased contexts.
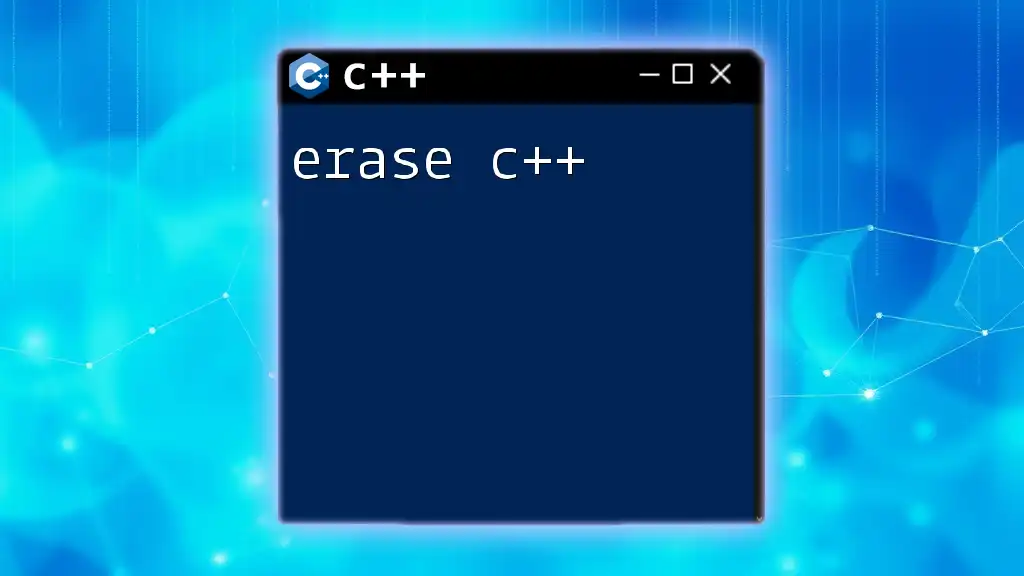
Implementing Type Erasure in C++
Basic Usage of std::function
C++ provides several built-in features to support type erasure, with `std::function` being a standout.
`std::function` is a versatile template class that can store, copy, and invoke any callable target—functions, lambda expressions, or function objects—while erasing the actual type of the callable. Here's how it works:
#include <iostream>
#include <functional>
void invoke(std::function<void()> func) {
func();
}
int main() {
invoke([] { std::cout << "Hello from a lambda!" << std::endl; });
return 0;
}
In this example, we define a function `invoke` that accepts a `std::function<void()>` type. You can pass any callable that matches this signature, demonstrating the flexibility of type erasure.
Custom Type Erasure Implementations
While `std::function` is incredibly useful, understanding how to implement type erasure manually can deepen your knowledge. Below is a simple type-erased wrapper class:
#include <iostream>
#include <memory>
class Any {
public:
template<typename T>
Any(T value) : m_value(new Holder<T>(value)) {}
void execute() const {
m_value->execute();
}
private:
struct Concept {
virtual void execute() const = 0;
virtual ~Concept() {};
};
template<typename T>
struct Holder : Concept {
Holder(T value) : m_value(value) {}
void execute() const override {
m_value();
}
T m_value;
};
std::shared_ptr<Concept> m_value;
};
int main() {
Any anyLambda([] { std::cout << "Executing a Lambda!" << std::endl; });
anyLambda.execute();
return 0;
}
In this code, we create a class `Any` that can hold any callable type. It achieves this through a nested `Concept` interface, which defines a pure virtual function `execute()`. The `Holder` template class inherits from `Concept` and implements the `execute()` method for specific types.
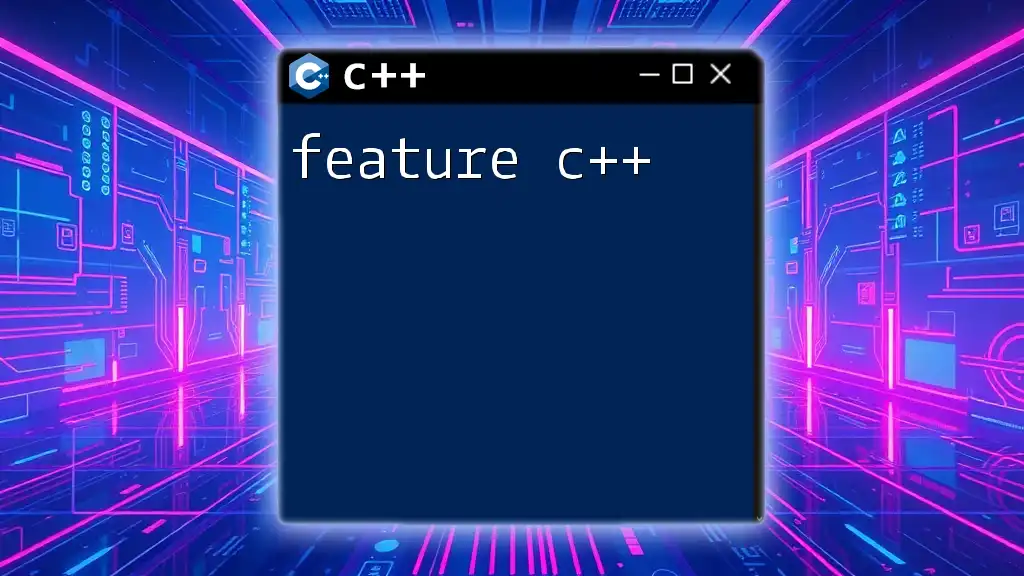
Real-World Applications of Type Erasure
Using Type Erasure in Libraries
Many standard C++ libraries utilize type erasure to provide generic functionality. The `std::function`, `std::variant`, and `std::any` templates are prime examples of this concept. They allow developers to manage different types with a unified interface, fostering versatility in codebases.
Type Erasure in Frameworks
Several popular frameworks leverage type erasure to enable dynamic functionality. For instance, libraries like Boost and Qt use it extensively, allowing developers to create highly flexible and maintainable systems. By abstracting type details, developers can create APIs that adapt easily to changes.
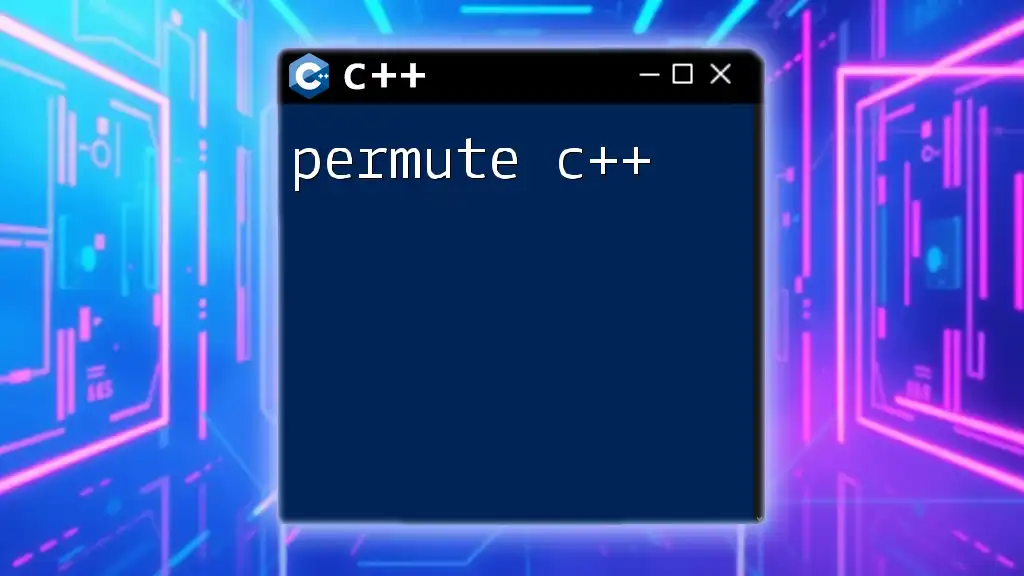
Comparing Type Erasure and Alternative Approaches
Type Erasure vs. Inheritance
Compared to inheritance, type erasure offers greater flexibility. It allows for more granular designs, where implementation details are hidden behind interfaces. However, inheritance provides stronger compile-time type checking, which is valuable in many scenarios.
Type Erasure vs. Variadic Templates
While variadic templates can also create flexible APIs, they statically enforce types at compile time. Type erasure, in contrast, allows different types to be handled dynamically, at runtime. This can be particularly beneficial in situations where the set of types cannot be known at compile time.
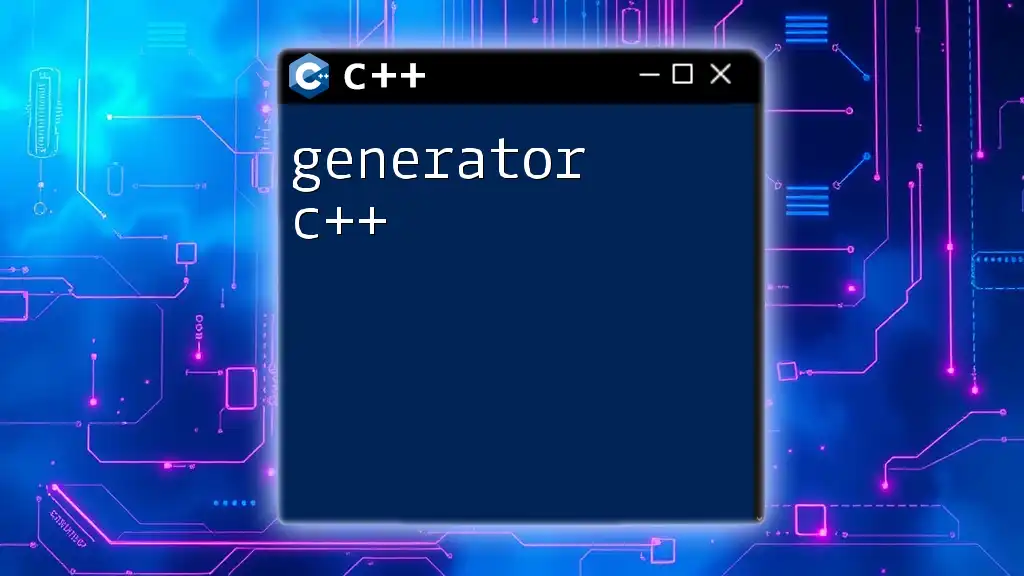
Best Practices for Using Type Erasure in C++
When to Use and When to Avoid
Type erasure shines in scenarios requiring high flexibility, such as heterogeneous containers or callback systems. However, developers should avoid it in performance-critical code or where static type guarantees are necessary.
Code Organization
When working with type erasure, it's essential to organize your code effectively. Start with a well-defined interface, implement concrete types that adhere to this interface, and leverage polymorphic behavior wisely. Keeping the responsibility of the type-erased components clear can lead to code that is both maintainable and easy to understand.
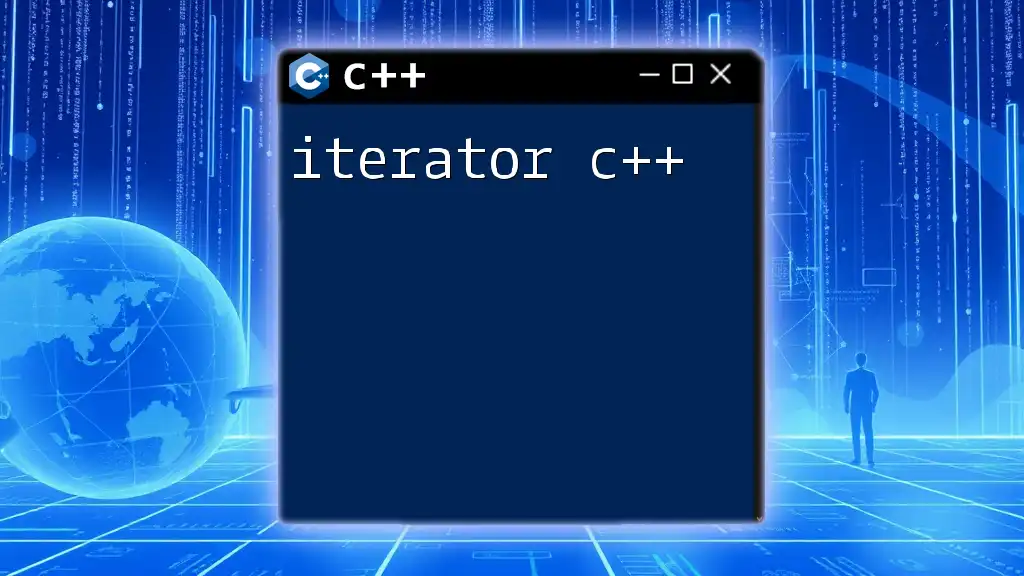
Conclusion
Type erasure in C++ provides a robust mechanism for abstracting type information while maintaining functionality. By implementing this concept effectively, developers can lead their projects toward increased flexibility and reusability. As development paradigms shift toward more generic programming methodologies, type erasure is set to remain a valuable tool in the C++ programmer's toolkit.
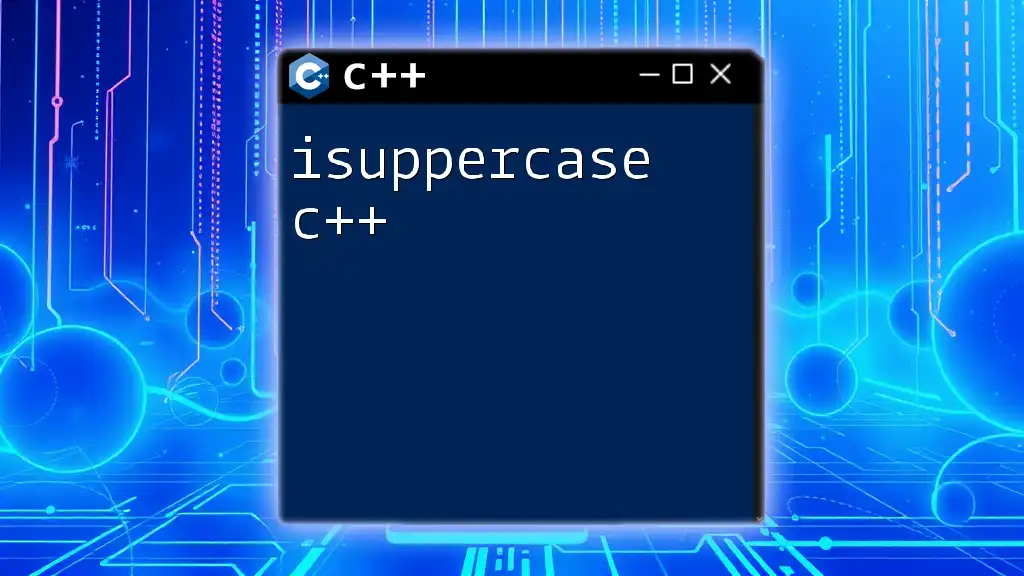
Additional Resources
For those looking to dive deeper into type erasure and its applications in C++, numerous resources are available, such as books, online articles, and courses. Engaging with existing projects that utilize type erasure can also be a beneficial way to see its practical implications and gain firsthand experience.