A runtime error in C++ occurs when the program is executed, typically due to illegal operations or resource issues, such as dividing by zero or accessing invalid memory.
#include <iostream>
using namespace std;
int main() {
int a = 5, b = 0;
cout << "Result: " << (a / b) << endl; // This line will cause a runtime error (division by zero)
return 0;
}
Understanding Runtime Errors
What is a Runtime Error?
A runtime error occurs when a program is executed, as opposed to during the compilation phase. Unlike compile-time errors, which prevent your code from compiling at all, a runtime error allows your code to compile successfully but causes it to fail during execution. This type of error can occur for various reasons such as improper input, invalid operations, or memory issues. Understanding runtime errors is crucial for maintaining the stability and reliability of your C++ applications.
Common Causes of Runtime Errors
Several common causes lead to runtime errors in C++:
-
Division by Zero: This happens when you attempt to divide a number by zero, which is mathematically undefined and leads to an error during execution.
#include <iostream> int main() { int x = 10, y = 0; std::cout << x / y; // This will cause a runtime error return 0; }
-
Null Pointer Dereference: Attempting to dereference a null pointer leads to undefined behavior. When you try to access memory at address zero, you are likely to cause a crash.
#include <iostream> int main() { int* ptr = nullptr; std::cout << *ptr; // This will cause a null pointer dereference return 0; }
-
Stack Overflow: This typically occurs due to excessively deep recursion that exceeds the stack space available to your program.
#include <iostream> void recursiveFunction() { recursiveFunction(); // This will cause a stack overflow } int main() { recursiveFunction(); return 0; }
-
Out-of-Bounds Array Access: Accessing an array index that does not exist causes undefined behavior and potential crashes.
#include <iostream> int main() { int arr[5] = {1, 2, 3, 4, 5}; std::cout << arr[10]; // Out-of-bounds access return 0; }
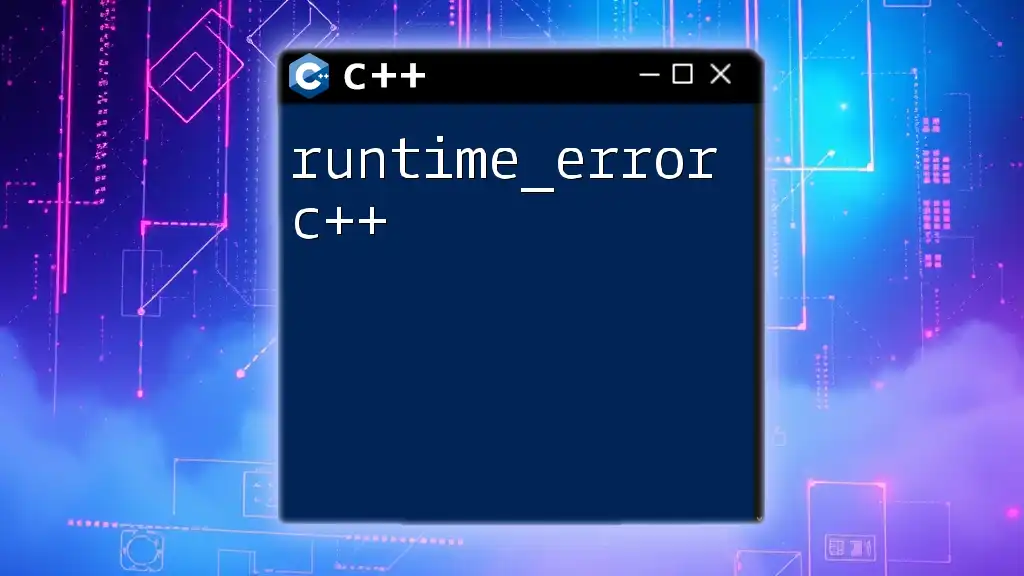
Detecting Runtime Errors
Debugging Techniques
Debugging is an essential skill when dealing with runtime errors. Utilizing debugging tools can help you identify where things are going wrong. Popular tools like gdb or the Visual Studio Debugger allow you to step through your code, examine variables, and analyze the state of your application when an error occurs.
Code Snippet Example: Basic gdb Commands
To demonstrate using gdb, you can compile the following simple program and debug it.
#include <iostream>
int main() {
int* ptr = nullptr;
std::cout << *ptr; // This will cause a null pointer dereference
return 0;
}
You can run this in gdb like so:
$ g++ -o my_program my_program.cpp
$ gdb ./my_program
In gdb, use commands like `run` to execute your program, and when it crashes, use `backtrace` to trace where the null pointer dereference occurred.
Error Messages Explained
Being able to interpret compiler or runtime error messages is vital for effective debugging. Each error message is crafted to give you context about what's gone wrong. For instance, when an out-of-bounds access occurs, the error message may refer to an invalid index, which hints you to check your indexing logic.
Code Snippet Example: Common Runtime Error Message in C++
#include <iostream>
int main() {
int arr[5] = {1, 2, 3, 4, 5};
std::cout << arr[10]; // Out-of-bounds access
return 0;
}
When executing this code, the system may throw an error about accessing a non-existent index. Reading and interpreting these messages can significantly reduce the time spent debugging.
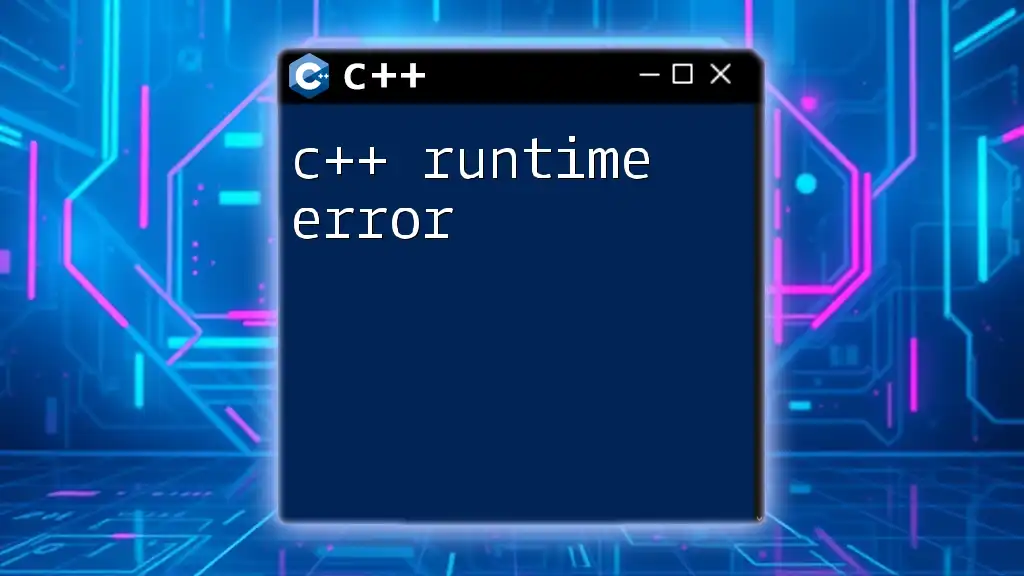
Common Runtime Errors in C++
Division by Zero
Division by zero is one of the most common runtime errors. If you do not check the denominator before performing a division, your program may crash or exhibit undefined behavior.
Prevention Strategy: Always validate your inputs before processing them.
Code Snippet Example: Handling Division by Zero
#include <iostream>
int main() {
int x = 10, y = 0;
if (y != 0) {
std::cout << x / y;
} else {
std::cout << "Error: Division by zero!";
}
return 0;
}
Accessing Out-of-Bounds Arrays
Out-of-bounds array access occurs when your code attempts to access memory outside the allocated range for an array. This can lead to unpredictable behavior and crashes.
Prevention Technique: Always check the size of your array before accessing it.
Code Snippet Example: Safe Array Access
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
if (vec.size() > 10) {
std::cout << vec[10];
} else {
std::cout << "Accessing an out-of-bounds index!";
}
return 0;
}
Null Pointer Dereferences
A null pointer dereference occurs when a program attempts to access or modify the data at the address indicated by a null pointer, leading to runtime errors.
Prevention Strategy: Before dereferencing a pointer, check if it is null.
Code Snippet Example: Null Pointer Protection
#include <iostream>
int main() {
int* ptr = nullptr;
if (ptr) {
std::cout << *ptr;
} else {
std::cout << "Error: Attempt to dereference a null pointer!";
}
return 0;
}
Stack Overflow
Stack overflow, often the result of uncontrolled recursion, can crash your program when calls exceed the stack limit.
Prevention Strategy: Limit the recursion depth, or use iterative approaches whenever possible.
Code Snippet Example: Recursive Function Causing Stack Overflow
#include <iostream>
void recursiveFunction() {
recursiveFunction(); // This will cause a stack overflow
}
int main() {
recursiveFunction();
return 0;
}
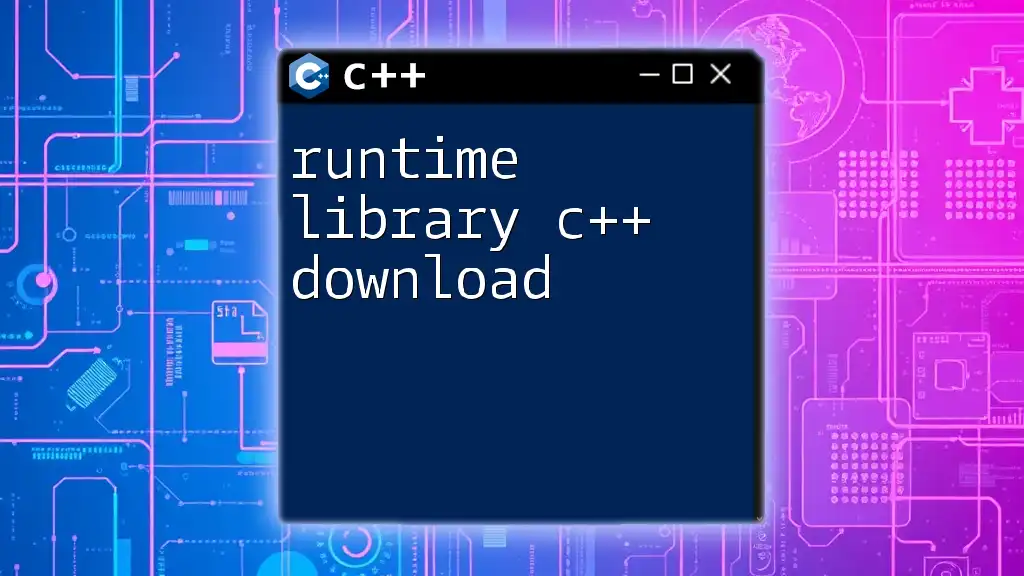
Best Practices to Avoid Runtime Errors
Input Validation
Inspecting and validating inputs prevents a wide array of runtime errors. By ensuring that inputs conform to expected formats and ranges, you can catch errors before they lead to crashes.
Using Exception Handling
Utilizing exception handling mechanisms such as try-catch blocks can effectively manage runtime errors without crashing your entire application. Exception handling allows for graceful recovery and error reporting.
Code Snippet Example: Using Try-Catch for Error Handling
#include <iostream>
#include <stdexcept>
int main() {
try {
throw std::runtime_error("An error occurred.");
} catch (const std::runtime_error& e) {
std::cout << "Caught: " << e.what() << std::endl;
}
return 0;
}
Memory Management
Proper memory management is crucial in C++. Failing to delete dynamically allocated memory can lead to memory leaks, while mismanaging pointers can lead to dangling references. Always ensure you allocate and deallocate memory appropriately.
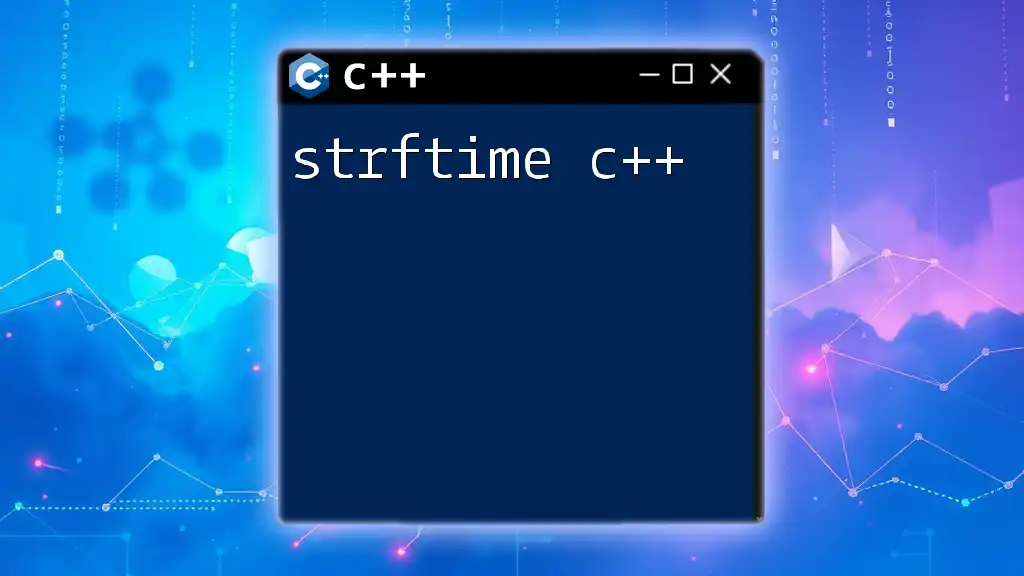
Conclusion
Understanding and handling runtime errors is essential for effective C++ programming. By familiarizing yourself with common causes, debugging techniques, and best practices, you will significantly enhance the stability and robustness of your applications. Practicing real-world coding scenarios will further solidify your ability to tackle runtime errors.
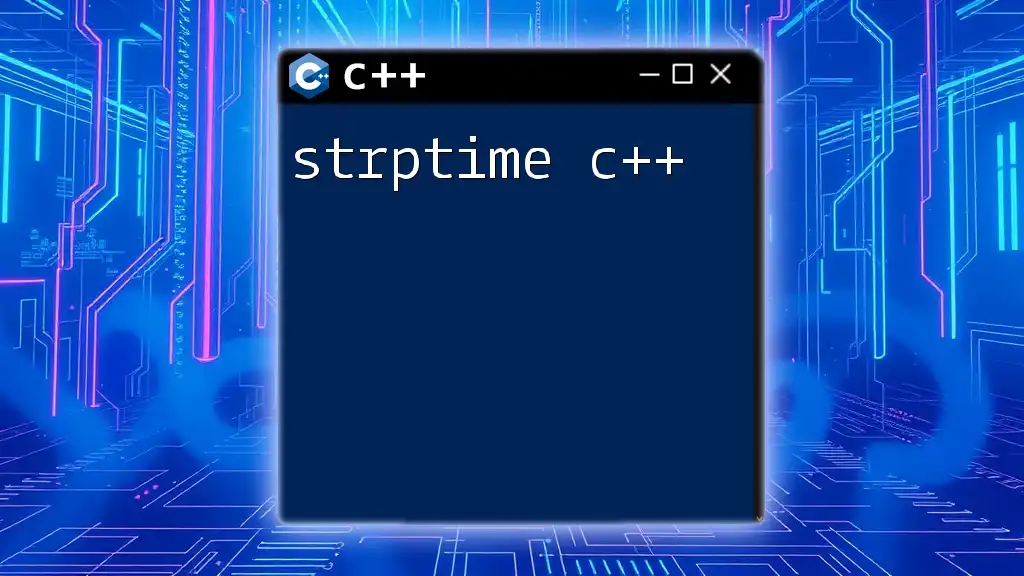
Additional Resources
For further learning, consult the official [C++ documentation](https://en.cppreference.com/w/), explore powerful C++ books, or take online courses that specialize in error handling and debugging. Engaging in community forums can also provide support and creative solutions to common pitfalls you might encounter.
Final Thoughts
By mastering the intricacies of runtime errors, you will not only improve your coding skills but also position yourself as a more effective developer. Remember that experimentations, along with failures, can significantly contribute to your learning journey in the C++ world.