A C++ runtime error occurs when a program is executed but encounters an unexpected condition, resulting in abnormal termination, often due to issues like dereferencing a null pointer or array index out of bounds.
Here's an example code snippet that demonstrates a common runtime error caused by dividing by zero:
#include <iostream>
int main() {
int a = 5;
int b = 0; // This will cause a runtime error
std::cout << "Result: " << a / b << std::endl; // Division by zero
return 0;
}
Understanding C++ Runtime Errors
What are Runtime Errors?
A runtime error in C++ occurs during the execution of a program, as opposed to compile-time errors that present themselves during the compilation of the code. Runtime errors are significant because they can cause a program to behave unexpectedly or terminate prematurely. For any C++ programmer, understanding runtime errors is crucial to writing robust and error-free code.
Common Causes of Runtime Errors
Memory Management Issues
One of the most frequent sources of runtime errors in C++ arises from improper memory management. This includes buffer overflows, which occur when a program writes more data to a block of memory than it can hold, and memory leaks, where allocated memory is not properly released, leading to decreased performance over time.
Code Example: A memory leak using `new` and `delete`.
#include <iostream>
void memoryLeakExample() {
int* array = new int[10]; // dynamically allocating memory
// Forgetting to delete leads to a memory leak
// delete[] array; // Uncommenting this line fixes the leak
}
Division by Zero
This is a straightforward yet common error that occurs when a program attempts to divide a number by zero. This leads to undefined behavior and can crash the program.
Code Example: A divide-by-zero scenario.
#include <iostream>
int main() {
int numerator = 10;
int denominator = 0;
// Attempting to divide by zero
// int result = numerator / denominator; // Uncommenting this line leads to a runtime error
std::cout << "Result: " << result << std::endl;
return 0;
}
Null Pointer Dereferencing
Dereferencing a null pointer results in a runtime error since the program is trying to access memory that is not allocated. This can cause segmentation faults and crashes.
Code Example: Accessing a member of a null object.
#include <iostream>
class MyClass {
public:
void display() {
std::cout << "Hello, World!" << std::endl;
}
};
int main() {
MyClass* obj = nullptr;
// Attempting to access a method of a null pointer
// obj->display(); // Uncommenting this line leads to a runtime error
return 0;
}
Array Out-of-Bounds Access
Accessing elements outside the defined limits of an array can lead to unpredictable behavior and crashes. This occurs because the program may access memory that is not intended for the array.
Code Example: Out-of-bounds access illustration.
#include <iostream>
int main() {
int arr[5] = {1, 2, 3, 4, 5};
// Accessing out of bounds
// std::cout << arr[10]; // Uncommenting this line leads to a runtime error
return 0;
}
Less Common Runtime Errors
Invalid Cast
Dynamic casting in C++ can lead to runtime errors if the cast is invalid. This happens when the program tries to convert an object of one type into another incompatible type.
Code Example: Demonstrating an invalid cast.
#include <iostream>
#include <exception>
class Base {};
class Derived : public Base {};
int main() {
Base* basePtr = new Base();
// Invalid cast from Base to Derived
// Derived* derivedPtr = dynamic_cast<Derived*>(basePtr); // Uncommenting this line leads to a runtime error
delete basePtr;
return 0;
}
Exceptions and Exception Handling
Proper exception handling is imperative to avoid runtime errors. Using `try`, `catch`, and `throw` allows programmers to manage errors gracefully and maintain control of the flow of the program.
Code Example: Using `try`, `catch`, and `throw`.
#include <iostream>
#include <stdexcept>
void riskyFunction() {
throw std::runtime_error("An error occurred!");
}
int main() {
try {
riskyFunction();
} catch (const std::runtime_error& e) {
std::cout << "Caught exception: " << e.what() << std::endl;
}
return 0;
}
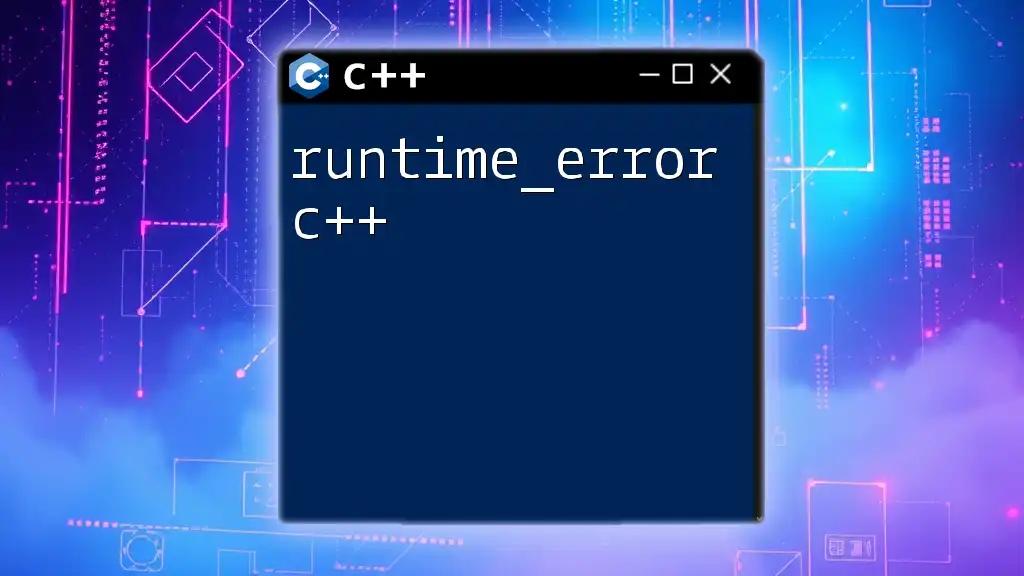
Diagnosing Runtime Errors
Debugging Techniques
Using Debuggers
Debuggers are a programmer's best friend when it comes to diagnosing runtime errors. With tools like GDB or the Visual Studio debugger, developers can step through their code line by line, monitor variable states, and spot where the error occurs. Setting breakpoints can also help identify the flow of the program leading up to the error.
Logging
Integrating logging into a program is highly beneficial for diagnosing issues. Logs can provide insight into the program's state and behavior right before a runtime error occurs.
Code Example: Simple logging solution in C++.
#include <iostream>
#include <fstream>
#include <string>
void logMessage(const std::string& message) {
std::ofstream logFile("log.txt", std::ios_base::app);
logFile << message << std::endl;
}
int main() {
logMessage("Program started");
// Simulating an error
try {
throw std::runtime_error("An error occurred!");
} catch (const std::runtime_error& e) {
logMessage("Error: " + std::string(e.what()));
}
logMessage("Program ended");
return 0;
}
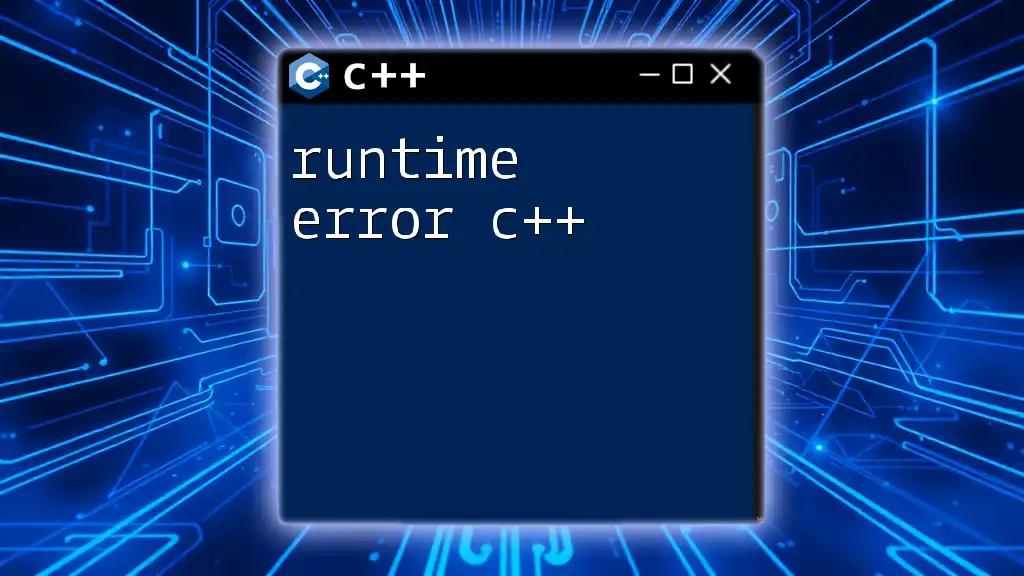
Preventing Runtime Errors
Best Practices in Coding
Input Validation
Failing to validate user input can lead to runtime errors. Always ensure that user inputs match expected formats and types before proceeding with any operations.
Code Example: Validating integer input.
#include <iostream>
#include <limits>
int main() {
int number;
std::cout << "Enter an integer: ";
while (true) {
if (std::cin >> number) {
break; // valid input
} else {
std::cout << "Invalid input. Please enter an integer: ";
std::cin.clear(); // clear error flag
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // discard invalid input
}
}
std::cout << "You entered: " << number << std::endl;
return 0;
}
Utilizing Smart Pointers
Smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, automatically manage memory for you, reducing the chances of memory leaks and dangling pointers.
Code Example: Using `std::unique_ptr`.
#include <iostream>
#include <memory>
class MyClass {
public:
void display() {
std::cout << "Hello from MyClass!" << std::endl;
}
};
int main() {
std::unique_ptr<MyClass> myPtr(new MyClass());
myPtr->display();
// Automatically deallocated when going out of scope
return 0;
}
Effective Exception Handling
Implementing effective exception handling techniques can help catch runtime errors early, allowing developers to react appropriately without crashing the program.
Code Example: Custom exception classes.
#include <iostream>
#include <exception>
class MyException : public std::exception {
public:
const char* what() const noexcept override {
return "My custom exception occurred!";
}
};
int main() {
try {
throw MyException();
} catch (const MyException& e) {
std::cout << e.what() << std::endl;
}
return 0;
}
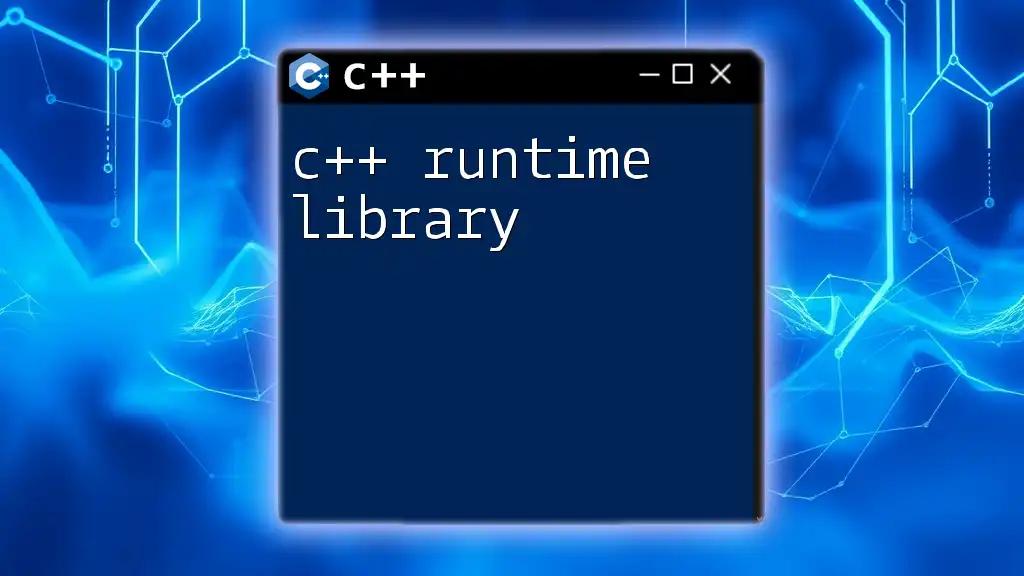
Runtime Error Troubleshooting
Handling Common Runtime Errors
Analyzing Core Dumps
When a program crashes, it may generate a core dump, which contains the memory image of the process at the time of the crash. Analyzing core dumps can provide insights into where in the code the error occurred. Tools like GDB can load core files to facilitate analysis.
Using Static Code Analysis Tools
Static code analysis tools help identify issues in the code before execution, thus preventing runtime errors. They can detect memory leaks, uninitialized variables, and other potential pitfalls. Popular tools include Clang Static Analyzer and cppcheck.
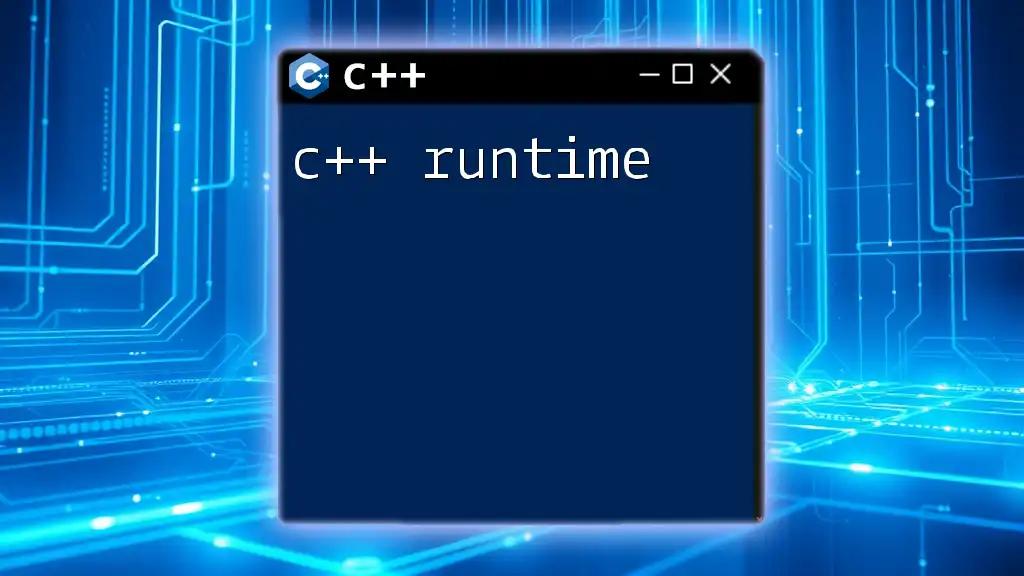
Conclusion
Understanding and preventing C++ runtime errors is essential for any programmer aiming to create efficient and reliable applications. By incorporating best coding practices, employing debugging techniques, and utilizing tools adeptly, developers can significantly mitigate runtime errors. Always remember: a proactive approach to error prevention is far more effective than troubleshooting after the fact. Embrace these concepts and apply them in your future C++ projects to enhance your programming skills.