In C++, a `logic_error` is an exception thrown to indicate that a program has encountered a logical issue, such as an invalid operation that violates the rules of the program's logic.
Here's an example of throwing a `logic_error` when trying to calculate the square root of a negative number:
#include <iostream>
#include <stdexcept>
#include <cmath>
double safeSqrt(double value) {
if (value < 0) {
throw std::logic_error("Cannot calculate the square root of a negative number.");
}
return std::sqrt(value);
}
int main() {
try {
std::cout << safeSqrt(-1) << std::endl;
} catch (const std::logic_error& e) {
std::cerr << "Logic error: " << e.what() << std::endl;
}
return 0;
}
What is a logic_error?
In C++, a `logic_error` is a type of exception class that derives from the `std::exception`. It represents errors in logic that occur during the program’s execution. These errors usually arise from incorrect assumptions made by the programmer, typically in regard to the preconditions or invariants of the code.
Importance in Exception Handling
Handling exceptions properly is crucial for creating robust and manageable applications. Using logic errors allows developers to catch and address issues stemming from logical misjudgments, ensuring that their program fails gracefully rather than crashing unpredictably.
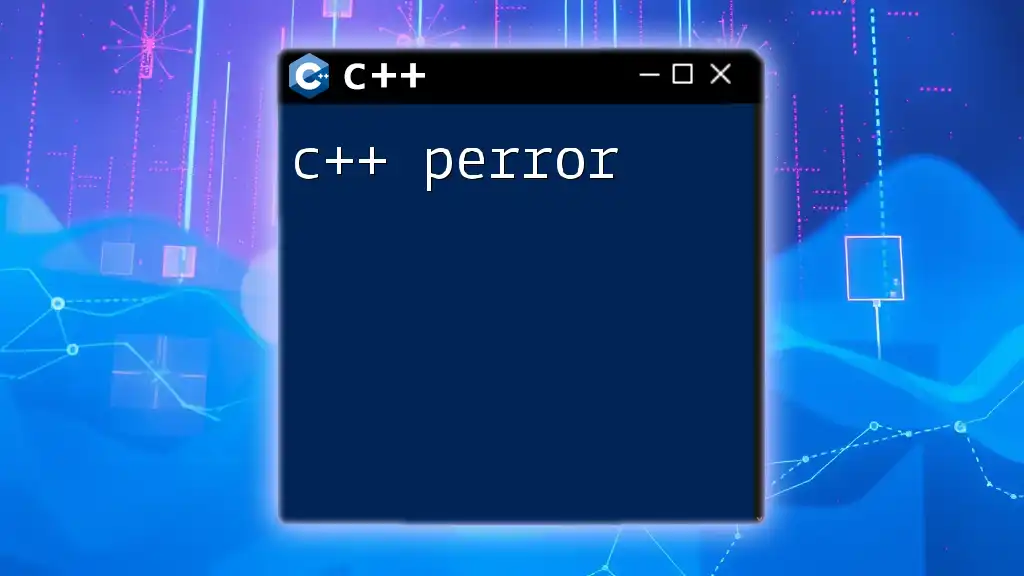
Overview of C++ Exception Handling
Exception handling in C++ is a mechanism that enables developers to respond to exceptional conditions or errors at runtime. C++ categorizes exceptions primarily into two types: logic errors and runtime errors.
- Logic Errors: These occur when there’s a flaw in the program's logic, such as violating constraints or assumptions.
- Runtime Errors: These arise from unforeseen issues during execution, like trying to access out-of-bounds memory.
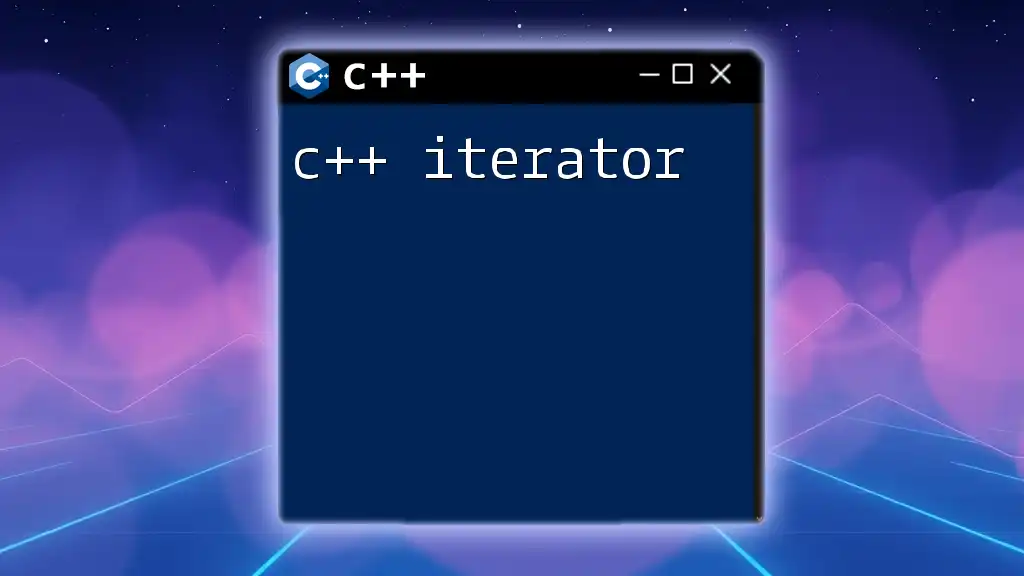
Understanding the logic_error Class
What is the logic_error Class?
The `std::logic_error` class is defined in the `<stdexcept>` header. It serves as the base class for errors that can be detected by the program while it is running, such as invalid input data or violations of class rules.
Why Use logic_error?
Programmers use `logic_error` when they encounter conditions that are logically flawed but do not stem from resource issues. This can include:
- Invalid Function Arguments: Arguments that fail to meet expectations.
- Class Invariants: Rules that an object must satisfy throughout its lifetime.
Understanding when to throw a `logic_error` versus a `runtime_error` can save debug time and lead to better code practices.
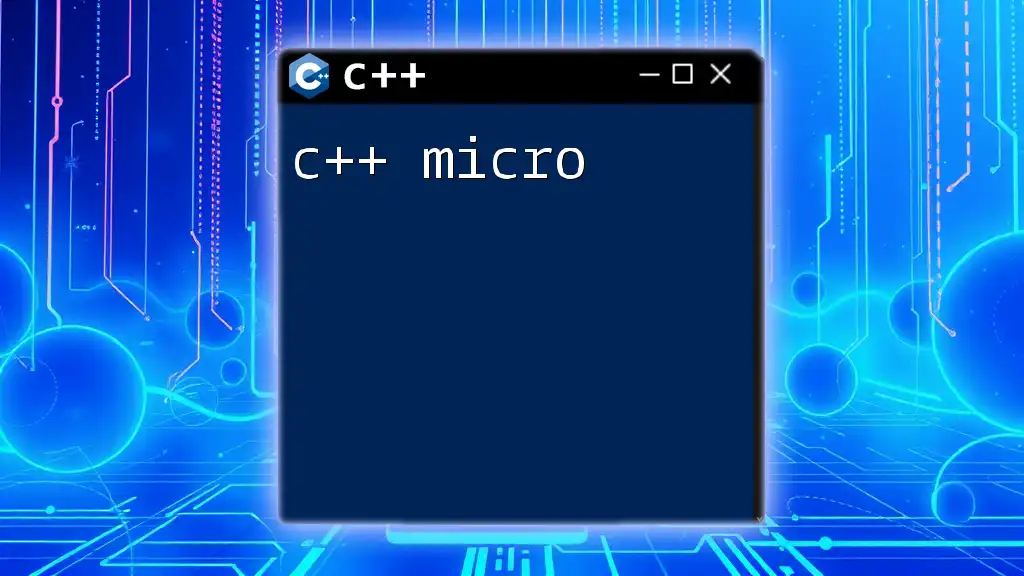
Common Scenarios Leading to logic_error
Typical Use Cases for logic_error
Recognizing when to implement a `logic_error` is pivotal for maintaining code quality. Here are a few common scenarios:
- Invalid Arguments: This can happen when a function receives unacceptable input, such as negative values where only positive numbers are valid.
- Invariance Violations: If an internal state of an object deviates from its defined rules, it is a sign that a logic error has occurred.
Example Scenarios
Invalid Function Arguments
#include <iostream>
#include <stdexcept>
void setAge(int age) {
if (age < 0) {
throw std::logic_error("Age cannot be negative.");
}
// Set age logic here
}
In this example, the `setAge` function throws a `logic_error` if the age provided is negative, indicating a logical flaw in the inputs.
Violating Class Invariants
class Circle {
private:
double radius;
public:
Circle(double r) {
if (r < 0) {
throw std::logic_error("Radius cannot be negative.");
}
radius = r;
}
};
Here, the `Circle` constructor checks the radius before assigning it. Throwing a `logic_error` emphasizes that the radius must conform to logical expectations.
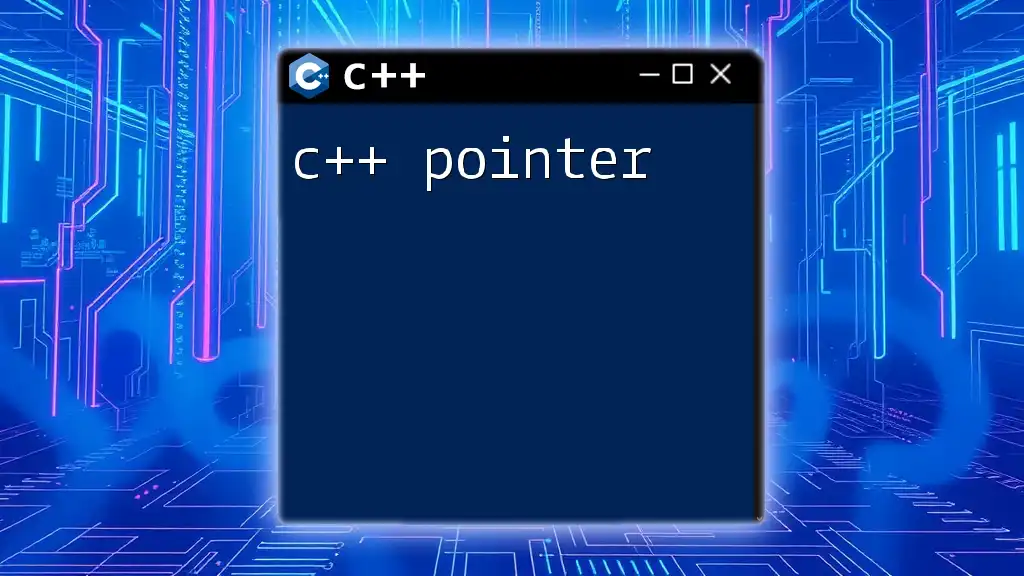
How to Use logic_error in Your Code
Catching and Handling logic_error
Using `logic_error` effectively involves catching it in a try-catch block to handle the issue gracefully without crashing the program.
try {
setAge(-5);
} catch (const std::logic_error& e) {
std::cerr << "Logic error: " << e.what() << '\n';
}
In the code above, if `setAge` throws a `logic_error`, the catch block will display the error message without crashing the program, enhancing user experience and debuggability.
Creating Custom Exception Messages
When creating `logic_errors`, it’s essential to provide clear and informative messages. A meaningful message can greatly aid in debugging and understanding the root cause of the problem. Ensure your messages:
- Clearly state the issue.
- Provide guidance on how to correct the problem.
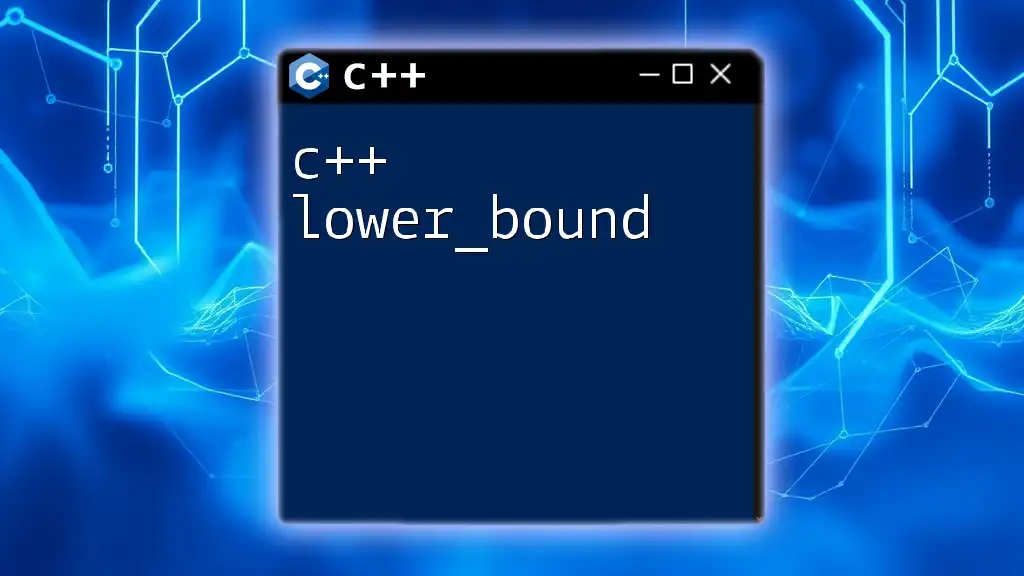
Best Practices for Using logic_error
When to Throw a logic_error
To effectively use `logic_error`, consider throwing it when:
- A function is invoked with improper or unexpected arguments.
- Internal states or invariants of classes are breached.
Avoiding Common Pitfalls
It's essential not to confuse `logic_error` with other exceptions. Logic errors are about incorrect assumptions made within the program. Misusing or mishandling them can lead to misunderstandings about program behavior and debugging frustrations.
- Ensure that exceptions convey meaningful feedback.
- Consider the user's perspective and provide relevant instructions in the error messages.
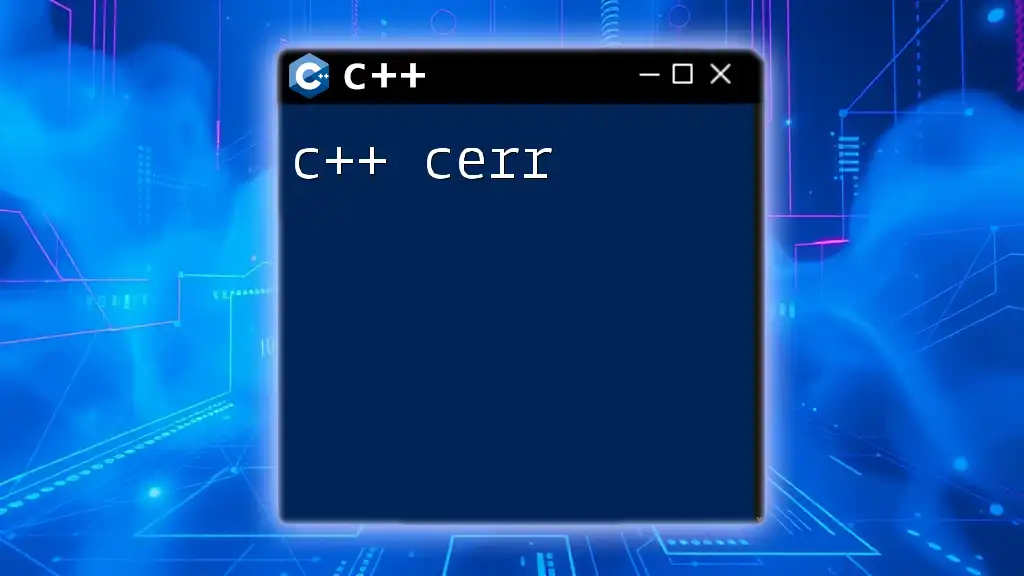
Conclusion
Understanding C++ logic_error is crucial for any developer looking to write robust applications. Proper use of `std::logic_error` can lead to cleaner code and more manageable debugging processes. By throwing logical errors where applicable and gracefully handling them, developers can maintain high code quality and improve user experiences.
By practicing effective exception handling techniques and understanding the relevance of logic errors, you can enhance your proficiency in C++ programming.
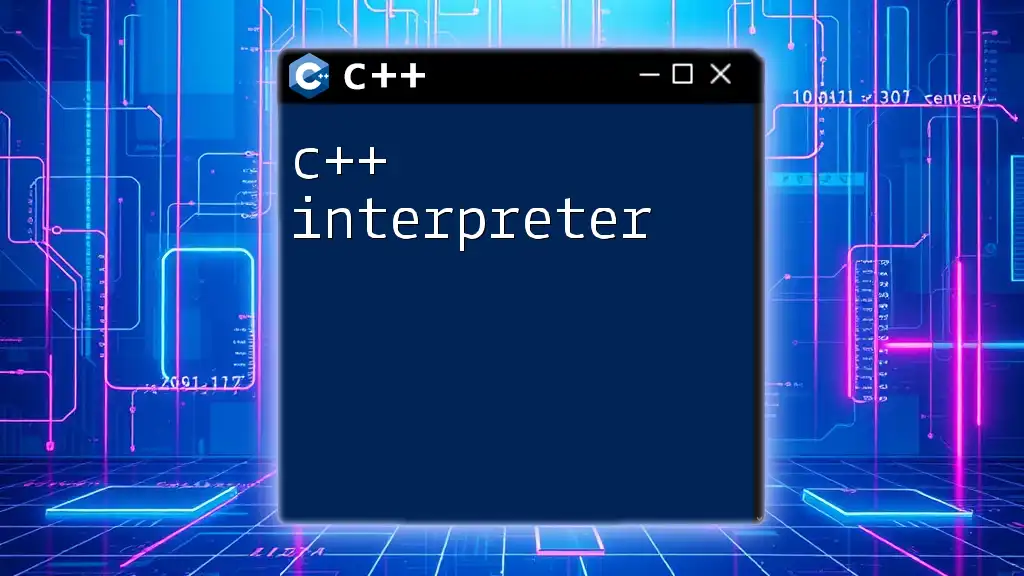
Additional Resources
- Reference the official C++ documentation on `<stdexcept>` for more detailed information.
- Explore books and online courses focused on advanced C++ techniques to deepen your understanding of exceptions and error handling strategies.