C++ micro refers to the practice of using minimal and efficient C++ code to perform tasks quickly, often employing concise commands or functions that illustrate programming concepts effectively.
Here's a simple example using a C++ lambda function to add two numbers:
#include <iostream>
int main() {
auto add = [](int a, int b) { return a + b; };
std::cout << "Result: " << add(3, 5) << std::endl; // Outputs: Result: 8
return 0;
}
Understanding Micro Programming
Micro programming refers to a smaller, more focused approach to programming, particularly in the context of C++. It emphasizes precision, efficiency, and minimal resource usage, making it ideal for systems with limited computing power and memory. Understanding C++ micro is essential in embedded systems, where every byte counts, and performance is critical.
Benefits of C++ Micro
One of the significant advantages of C++ micro lies in its efficiency. Unlike high-level languages that often abstract away details, C++ allows developers to manage memory directly. This capability is vital for optimizing resource usage in microcontrollers or low-power devices.
Another benefit is the flexibility offered by C++. The language supports multiple programming paradigms, including procedural, object-oriented, and generic programming, enabling developers to choose the best approach for their particular C++ micro tasks.
Real-time performance is another aspect where C++ micro excels. In situations where hardware needs to respond to inputs quickly (like sensors in IoT), C++ delivers predictable execution time, minimizing overhead.

Setting Up Your C++ Micro Environment
To start working with C++ micro, you first need to set up a suitable development environment.
Choosing the Right IDE
Various integrated development environments (IDEs) are popular among C++ developers. For effective C++ micro programming, consider IDEs like Visual Studio, Code::Blocks, or CLion, which offer robust debugging capabilities and support for various C++ standards. Your choice should reflect your previous experience and project complexity.
Installing Necessary Libraries and Tools
Having a proper library setup can significantly enhance your productivity in C++ micro programming. Essential libraries include:
- Boost: For advanced data structures and algorithms.
- STL (Standard Template Library): For common data types and algorithms.
- Microcontroller-specific libraries: These can often be found within specific vendor SDKs (e.g., Arduino, STM32).
Installation can typically be handled through package managers or directly downloaded from the library's homepage.
Compilers and Build Tools
Compiling your C++ code is crucial for any micro programming scenario. Popular compilers for C++ micro include GCC, MinGW, and Clang. Each compiler has its strengths, and your choice may depend on platform compatibility or specific project needs.
Build tools such as CMake or Makefile help automate the compilation process, improve workflow efficiency, and manage dependencies within your project.
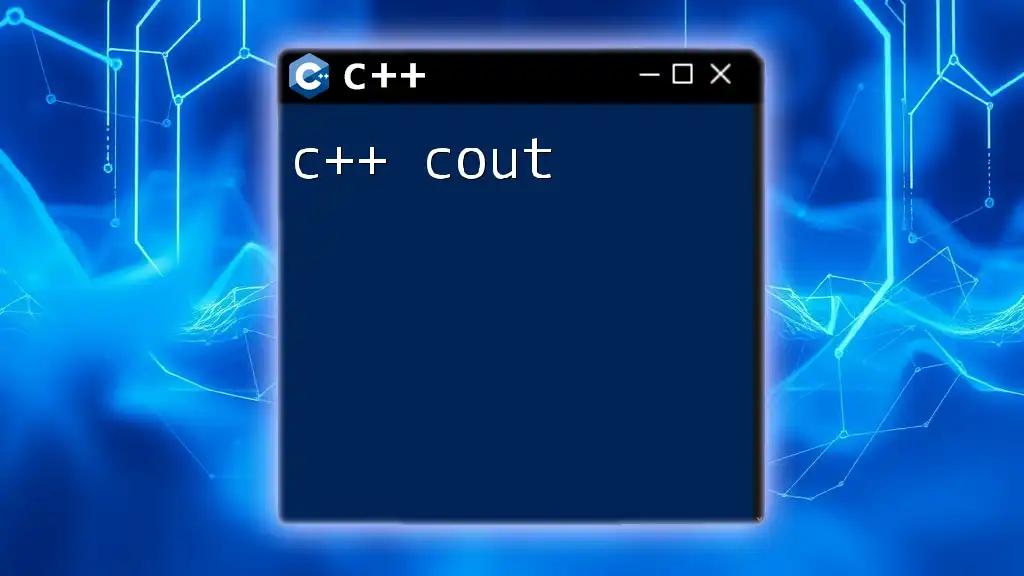
Core Concepts of C++ Micro Programming
Understanding the foundational concepts in C++ micro is key to becoming proficient.
Data Types and Memory Management
C++ offers a wide range of data types: from primitive types like `int` and `char` to more complex structures like `struct` and `class`. Understanding these types is fundamental to optimizing memory usage in a constrained environment.
Memory management is another critical aspect. In C++, developers can allocate memory on the heap, allowing for dynamic storage, or use the stack, which is automatically managed. Here is a simple example of dynamic memory allocation:
int* arr = new int[10]; // Allocating memory for an array
delete[] arr; // Freeing the allocated memory
In this example, we allocate memory for an array of integers and remember to deallocate it to prevent memory leaks, crucial in long-running applications.
Control Structures
Conditional Statements
Conditional statements determine which code block to execute based on specific conditions. Here’s a basic example of an `if` statement:
int a = 5;
if (a > 3) {
std::cout << "a is greater than 3" << std::endl;
} else {
std::cout << "a is not greater than 3" << std::endl;
}
Understanding how to effectively use conditional structures is essential for decision-making within your applications.
Loops
Loops, such as `for`, `while`, and `do-while`, are integral in C++ micro programming for iterating through data or processes. Consider this example of a `for` loop that prints numbers from `0` to `9`:
for(int i = 0; i < 10; i++) {
std::cout << "Value: " << i << std::endl;
}
These looping structures allow developers to execute the same block of code multiple times, which is often necessary in micro programming scenarios like sensor readings and data analysis.
Functions and Modularity
Function Definitions and Declarations
Functions are crucial for code organization and reusability in C++ micro. Here's a simple function that prints "Hello, World!":
void helloWorld() {
std::cout << "Hello, World!" << std::endl;
}
By calling `helloWorld()`, you encapsulate the behavior and can reuse it throughout your program without rewriting the code.
Inline Functions
Inline functions can optimize your micro programs further by suggesting to the compiler to insert the function’s body wherever the function is called, eliminating any function call overhead. Here’s an example:
inline int square(int x) { return x * x; }
Using inline functions can be highly beneficial in performance-critical applications, especially if called frequently.
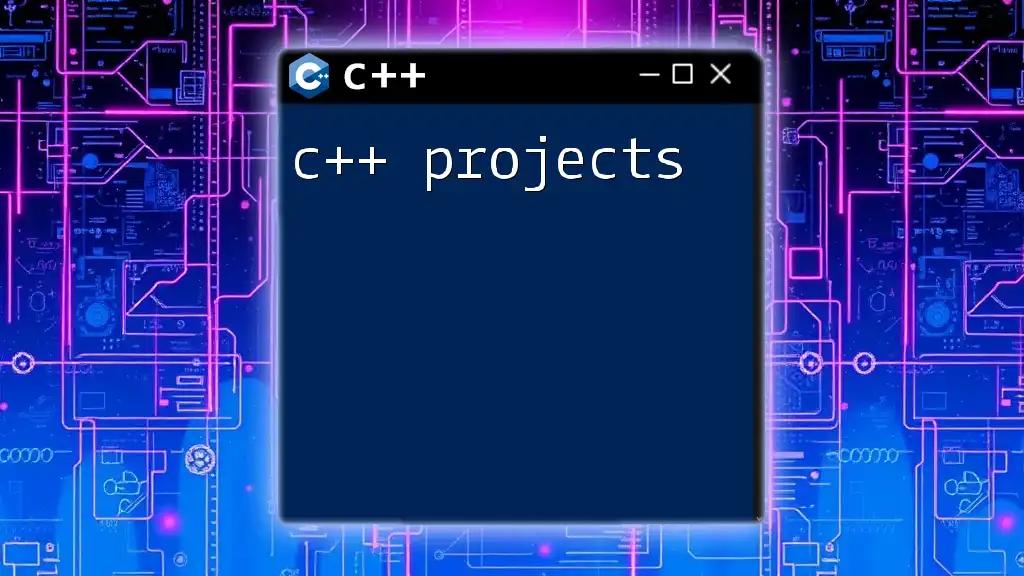
Advanced C++ Micro Techniques
Object-Oriented Programming (OOP) in Micro
OOP principles can simplify the development of complex systems. Encapsulation, inheritance, and polymorphism can all be employed effectively in micro programming. For instance, here's a basic class for a microcontroller:
class MicroController {
public:
void start() { /* start the microcontroller */ }
};
By encapsulating functionality within a class, you promote better organization and data protection within your C++ micro applications.
Templates and Generic Programming
C++ templates allow developers to create functions or classes that operate with any data type. This capability is particularly useful in writing general-purpose software. For example:
template<typename T>
T add(T a, T b) { return a + b; }
This generic function can add integers, floats, or even complex numbers, depending on what gets passed to it.
Exception Handling
Proper error management is essential for robust applications. C++ provides exception handling mechanisms to catch and respond to runtime errors effectively. Here’s an example using try-catch blocks:
try {
// code that may throw an exception
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
Being able to handle exceptions gracefully is critical, especially when dealing with hardware components that may behave unpredictably.
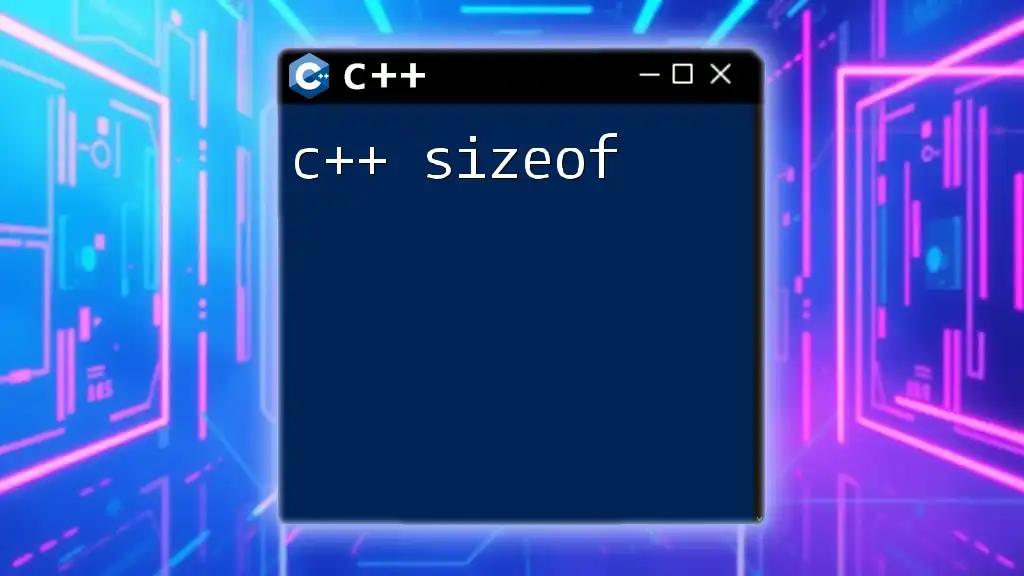
Best Practices for C++ Micro Programming
Code Optimization Techniques
To ensure efficient performance in C++ micro, it’s crucial to write optimized code. Focus on minimizing memory usage, choosing the right algorithms, and carefully managing your data structures. Regular profiling can help identify bottlenecks in your code.
Managing Complexity
Complexity can be managed through proper code organization, consistent naming conventions, and modular programming practices. Regular documentation is essential, as it aids future developers and even yourself in recalling the code's intent.
Testing and Debugging
Testing frameworks like Google Test can help establish a comprehensive testing environment, ensuring your C++ micro programs behave as expected. Debugging techniques, including using breakpoints and inspect variables, can simplify the development process and catch potential issues early.

Real-World Applications of C++ Micro
Embedded Systems
One of the most significant areas for C++ micro programming is in embedded systems. These systems, such as home appliances or industrial controllers, require efficiency and reliability. C++ offers the necessary tools and libraries to develop software for these unique computing environments.
IoT and Smart Devices
The Internet of Things (IoT) represents another exciting frontier for C++ micro programming. Smart devices often rely on C++ due to its efficiency and performance characteristics. By leveraging C++, developers can create powerful applications that interact with sensors and user interfaces seamlessly.
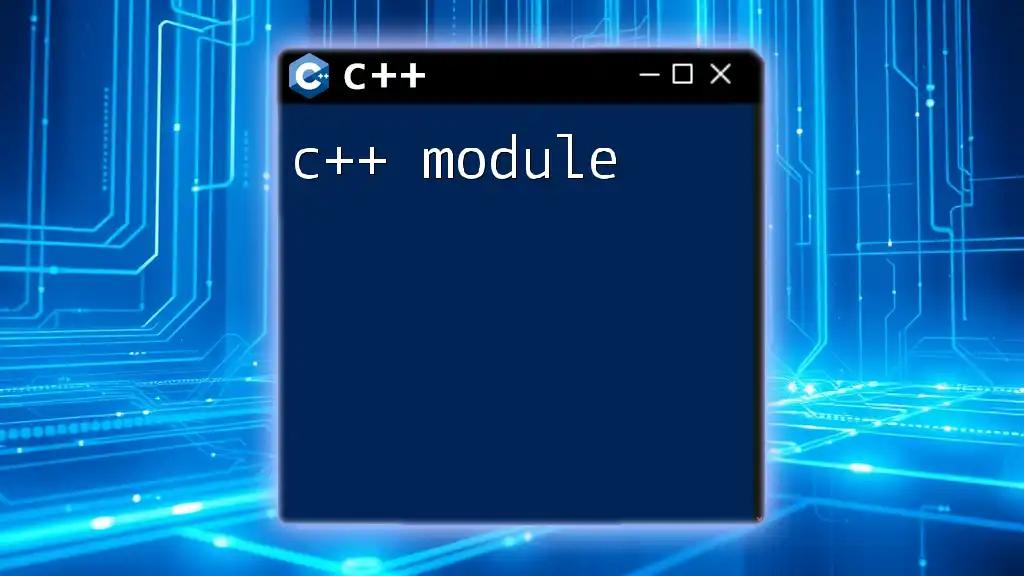
Conclusion
Mastering C++ micro programming opens up various opportunities in high-performance computing environments. From embedded systems to IoT devices, understanding these concepts will empower you to create efficient, robust programs. The skills you cultivate through practice and exploration of this domain will yield lasting benefits throughout your programming career.
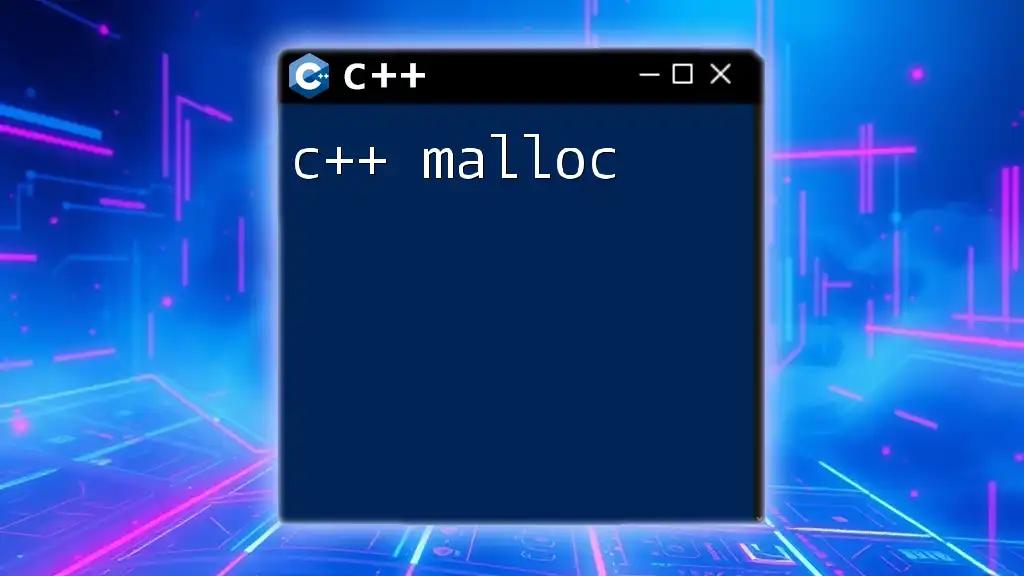
Additional Resources
In addition to this guide, numerous books and online courses delve deeper into C++ micro programming. Engage in community forums, such as Reddit or Stack Overflow, to connect with other developers and enhance your knowledge further. The world of C++ micro programming awaits you!