A C++ macro is a preprocessor directive that defines a piece of code that can be reused throughout the program, allowing for more concise and readable code.
#define SQUARE(x) ((x) * (x))
int main() {
int result = SQUARE(5); // result will be 25
return 0;
}
What are Macros in C++?
A cpp macro is a preprocessor directive that facilitates code reuse and can optimize software development. Macros allow developers to define constants and functions that can be used throughout their programs without the overhead of function calls. They serve as a powerful tool, particularly in scenarios requiring multiple constants or repeated computations.

Why Use C++ Macros?
Using C++ macros can lead to significant improvements in code efficiency and maintainability. Some advantages include:
-
Code Reusability: By defining macros, repetitive code can be replaced with a single directive, reducing the risk of mistakes and improving maintainability.
-
Performance: Macros are resolved during preprocessing, often leading to faster code since there is no function call overhead.
-
Conditional Compilation: The ability to compile certain parts of the code while excluding others enhances flexibility in code management.

Understanding Macro Definitions
What is a C++ Macro Definition?
A macro definition uses the `#define` directive to associate a name with a code fragment. This association allows for easy changes and consistent usage throughout the code.
Types of C++ Macros
Object-like Macros: These resemble constants and do not take parameters. When defined, every occurrence of the macro name will be replaced by its definition.
Example:
#define PI 3.14
This definition allows you to replace `PI` with `3.14` throughout your code, enabling easier modifications.
Function-like Macros: These are more complex as they take parameters. The macro name acts as a function, allowing for dynamic behavior based on the inputs.
Example:
#define SQUARE(x) ((x) * (x))
This macro will compute the square of any passed argument when called, essentially performing inline computation.
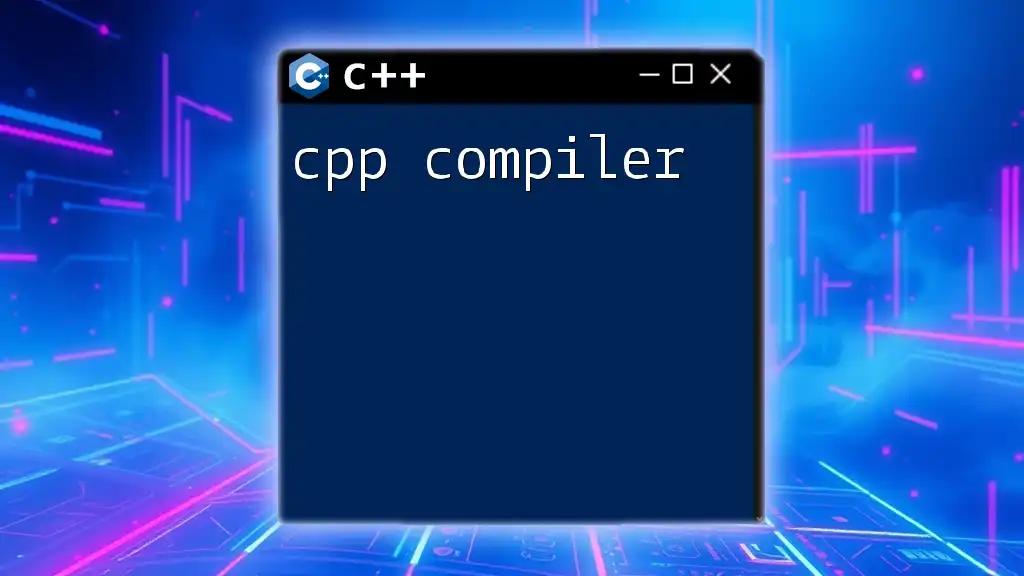
How to Create and Use C++ Macros
Creating C++ Macros
Creating a cpp macro involves using the `#define` directive followed by the macro name and its definition. Best practices suggest using uppercase letters to name macros for easy identification.
Example:
#define MAX_LENGTH 100
Examples of C++ Macro Usage
Macros can simplify coding tasks significantly. Here’s how different macros can be used effectively.
Simple Object-like Macro Example
You can easily define a constant value:
#define PI 3.14
Now, instead of repeatedly using `3.14`, simply use `PI`.
Function-like Macro Example
With a function-like macro, you can define reusable calculations:
#define SQUARE(x) ((x) * (x))
You can use it as follows:
int area = SQUARE(5); // This will expand to ((5) * (5))
Practical Scenario: Using Macros in Real Projects
Imagine a scenario where you need to compute the area of a circle multiple times.
#include <iostream>
#define PI 3.14
#define CIRCLE_AREA(radius) (PI * SQUARE(radius))
int main() {
double radius = 5.0;
double area = CIRCLE_AREA(radius);
std::cout << "Area of circle: " << area << std::endl;
return 0;
}
In this example, the `CIRCLE_AREA` macro combines the use of both object-like and function-like macros to compute the area efficiently.
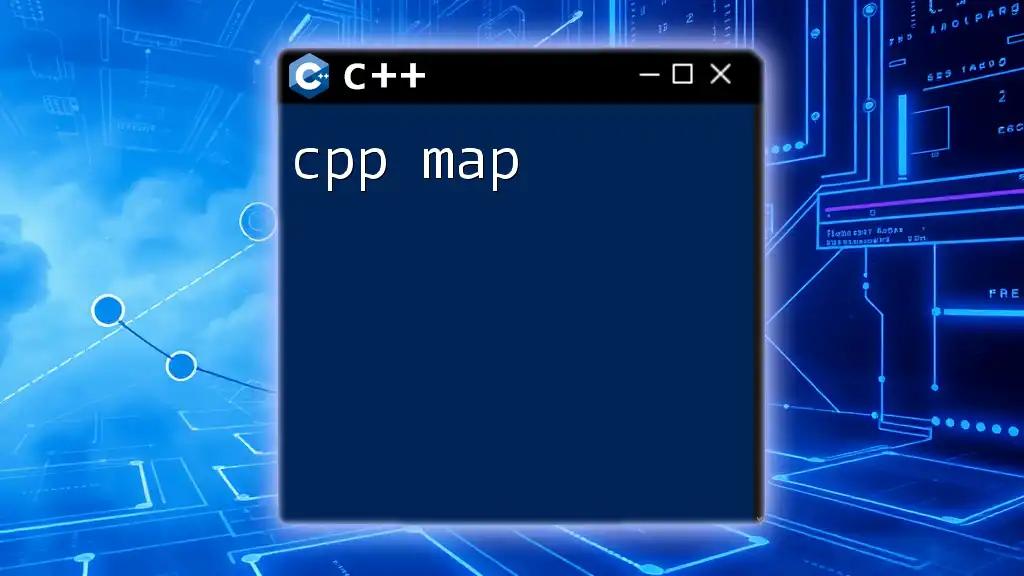
Advanced C++ Macro Techniques
Debugging and Log Macros
Creating debugging macros can help provide clarity during development. For example, you can define a logging macro:
#define LOG(msg) std::cout << "Log: " << msg << std::endl
You can use it to log messages throughout your code:
LOG("This is a debug message.");
Variadic Macros
Variadic macros allow for a variable number of arguments:
#define DEBUG_LOG(...) std::cout << __VA_ARGS__ << std::endl
You can call it with different messages:
DEBUG_LOG("Value of x:", x);
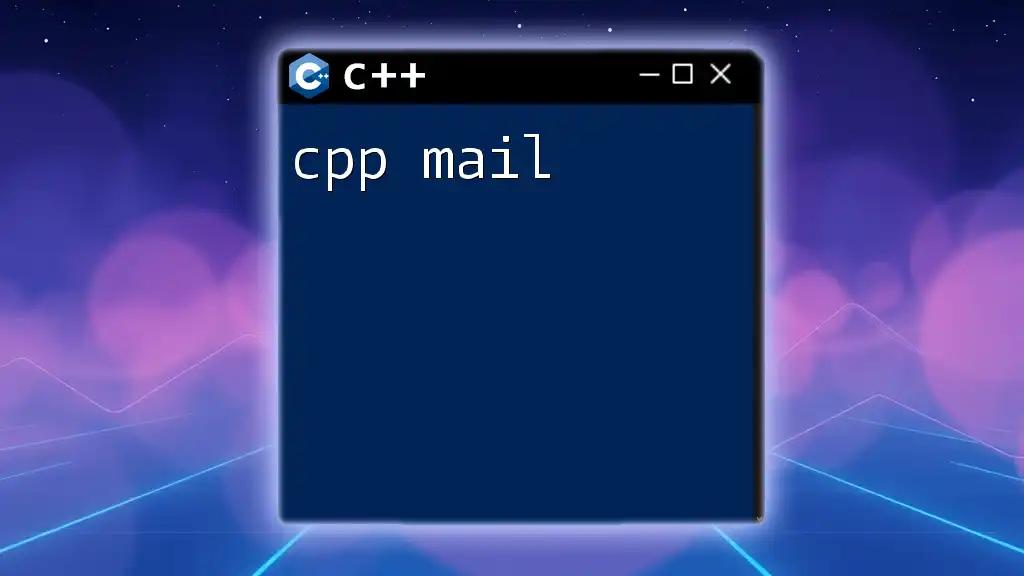
C++ Preprocessor Directives and Macros
Understanding the C++ Preprocessor
The C++ preprocessor is a crucial component that processes source code before compilation. During this phase, macros are expanded, and conditional compilation directives are evaluated. This makes macros powerful tools within the code as they can alter which parts of the code are included during compilation.
Conditional Compilation with Macros
Macros can be used to include or exclude code segments through conditional compilation. This is particularly useful for platform-specific code.
Example:
#ifdef DEBUG
#define LOG(msg) std::cout << "Debug: " << msg << std::endl
#else
#define LOG(msg) // No operation
#endif
In the above example, if `DEBUG` is defined, logging will occur; otherwise, it will do nothing.
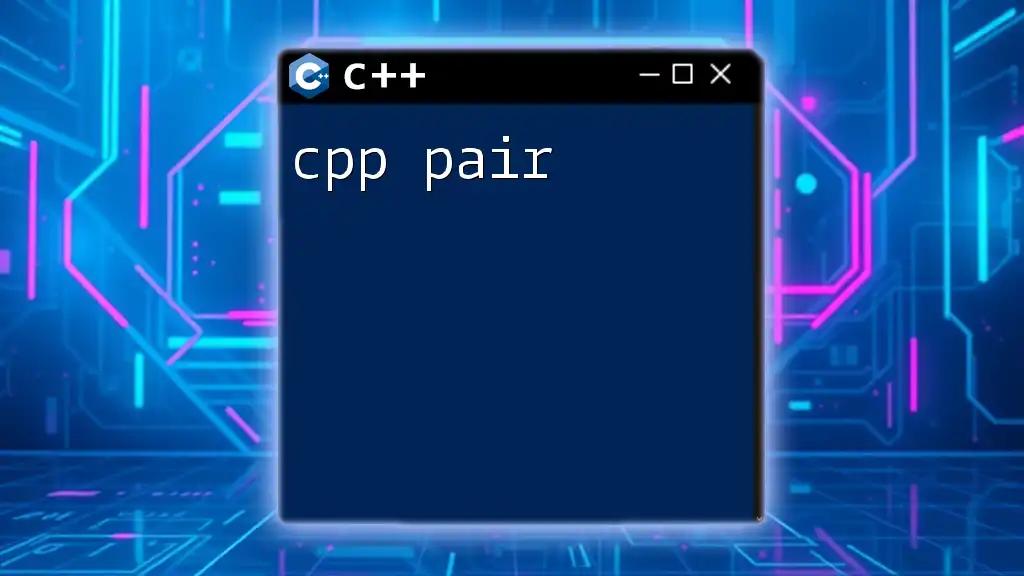
Common Pitfalls and Best Practices
Using macros can lead to complexities. Here are common issues:
-
Debugging Challenges: Macros don’t respect scope, making them difficult to debug compared to functions.
-
Unexpected Side Effects: Due to their in-line substitution nature, they can produce unexpected results if not careful with parentheses.
Best Practices for Using C++ Macros
-
Favor Inline Functions: Whenever possible, consider using inline functions instead of macros for type safety and scope control.
-
Naming Conventions: Use a prefix for macros (e.g., `MYLIB_MAX`), which helps avoid naming conflicts.
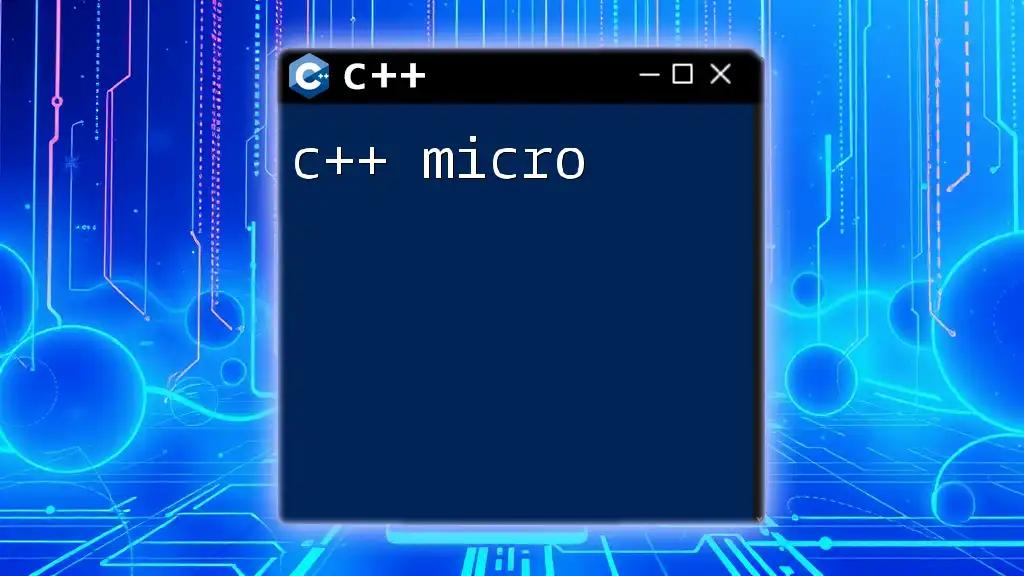
Conclusion
C++ macros serve as a potent tool for optimizing code and promoting reusability. They simplify repetitive tasks and streamline certain programming practices. However, caution is necessary to avoid debugging challenges and side effects. Understanding C++ macros will enhance your coding efficiency, making your software development experience more productive.
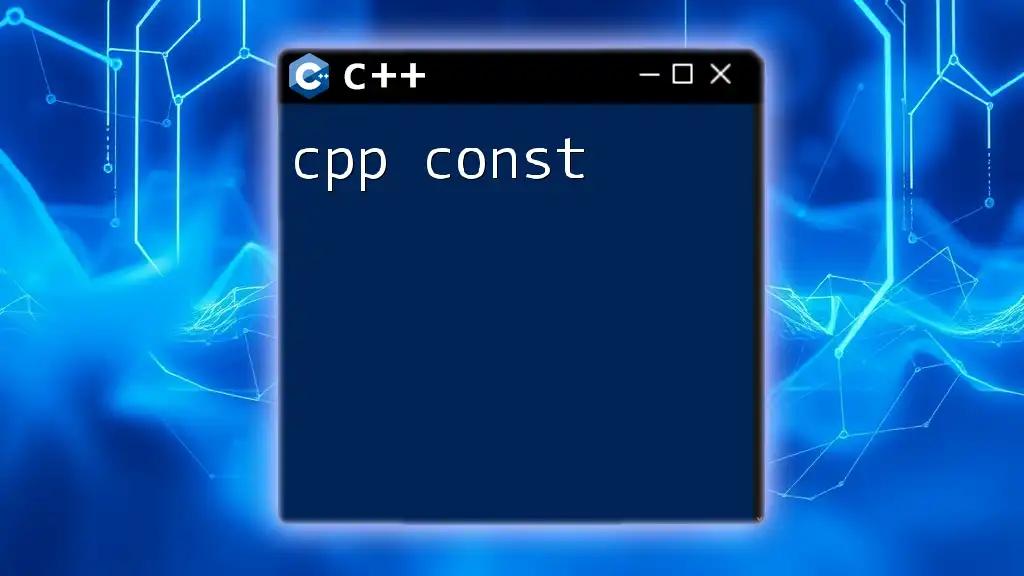
Additional Resources
For further exploration of C++ macros, consider delving into recommended books and online courses tailored to different expertise levels. Engaging with community forums can also expand your understanding and provide support as you navigate your programming journey.