The "cpp aerospace" topic focuses on using C++ programming to develop applications and simulations within the aerospace industry, enhancing efficiency and control in various aerospace systems.
Here's a simple example of how to define a class for a spacecraft in C++:
class Spacecraft {
public:
void launch() {
std::cout << "Launching spacecraft!" << std::endl;
}
void land() {
std::cout << "Landing spacecraft!" << std::endl;
}
};
Key Features of C++
C++, the powerful programming language, brings several key features to the table that make it particularly well-suited for aerospace applications.
Object-Oriented Programming (OOP)
OOP is a programming paradigm centered around objects that combine data and functionality. In the context of aerospace, this approach allows for creating modular and reusable code. Key OOP concepts—like inheritance, encapsulation, and polymorphism—permit engineers to develop complex systems more effectively.
For instance, consider an Aircraft class that might serve as a base class for different aircraft types:
class Aircraft {
public:
virtual void fly() = 0; // Pure virtual function
};
class FighterJet : public Aircraft {
public:
void fly() override {
// Fighter jet specific flight code
}
};
This structure promotes clean code management, essential in the aerospace field where reliability is paramount.
Performance and Efficiency
C++ is renowned for its performance and efficiency, factors crucial in aerospace applications where processing speed and resource management can have significant ramifications. The language facilitates low-level memory management, allowing developers to optimize applications for speed without sacrificing control.
For instance, C++ enables developers to manage memory using pointers and dynamic allocation:
int* arr = new int[100]; // Dynamic memory allocation
// Operations
delete[] arr; // Be sure to free the allocated memory
This capability allows for fine-tuning performance-critical systems like flight controllers and simulations.
Standard Template Library (STL)
The STL is a powerful library that provides generic classes and functions. With numerous ready-made algorithms and data structures, it simplifies complex tasks, making code more efficient and easier to read.
For example, using vectors for managing telemetry data can significantly enhance performance:
#include <vector>
std::vector<int> telemetryData;
// Populate and manipulate telemetry data
This functionality is beneficial in contexts where dynamic data sizes are common, such as real-time data streaming from aircraft systems.

Common Applications of C++ in Aerospace
Flight Simulation
Flight simulators provide a critical training environment for pilots, and C++ plays a vital role in their development. Through C++, developers can simulate realistic flight scenarios which isolate machine performance from human actions.
A simplified example demonstrating a basic flight simulation scenario:
class Aircraft {
public:
void fly() {
// Simplified flight logic
std::cout << "The aircraft is flying!" << std::endl;
}
};
int main() {
Aircraft myPlane;
myPlane.fly(); // Simulate flight
return 0;
}
Such simulation systems become invaluable, helping pilots train in a cost-effective and safe manner.
Control Systems
C++ is integral to avionics and autopilot systems foundational for modern aircraft. These systems require precise, real-time computations and reliability, attributes that C++ provides effectively. An example of a basic control loop implemented in C++:
void controlLoop() {
while (true) {
// Real-time control logic, such as updating flight path or altitude
updateFlightPath();
}
}
Data Processing and Analysis
Another area where C++ excels in aerospace is processing and analyzing telemetry data. Its efficiency can manage and parse large volumes of data seamlessly. A simple code snippet for parsing telemetry info might look like this:
std::string telemetryData = "Altitude: 30000";
std::string altitude = telemetryData.substr(10); // Basic parsing
std::cout << "Current Altitude: " << altitude << std::endl;
Using C++, developers can design robust data handling systems that provide critical insights during test flights and operational phases.
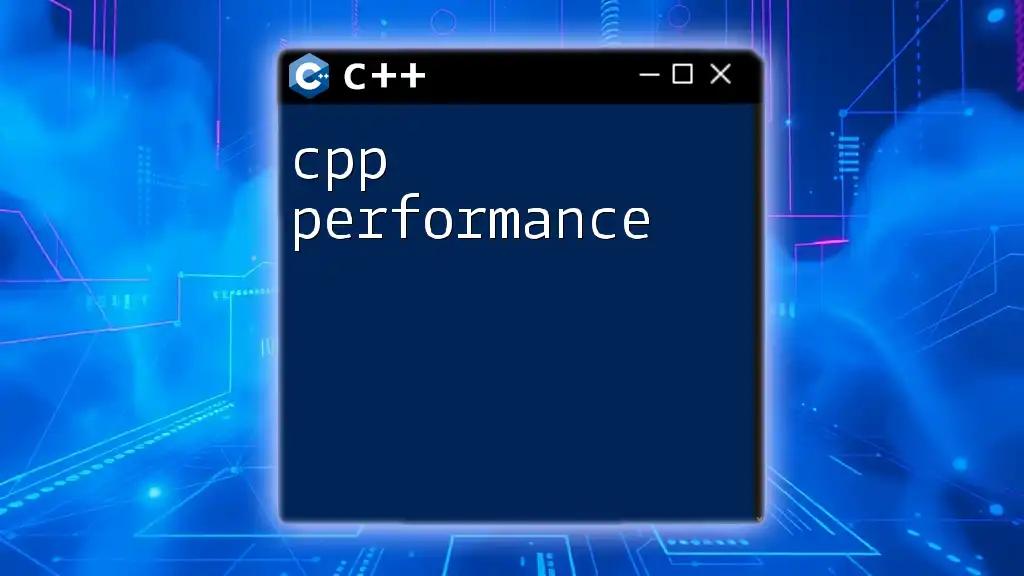
Integrating C++ with Aerospace Standards
DO-178C and C++
Compliance with standards like DO-178C is non-negotiable in the aerospace industry. This document outlines a framework to ensure the safety and reliability of software. While developing with C++, engineers must adhere to these guidelines, encompassing design, implementation, verification, and documentation.
Best Practices for C++ Code in Aerospace
When developing aerospace software using C++, certain best practices should be followed:
- Code Reviews and Testing: Rigorous peer reviews are fundamental to identify potential issues early in the development cycle.
- Use of Version Control Systems: Tools like Git help track revisions, ensuring better management of the codebase and enhancing collaboration among teams.

Challenges of Using C++ in Aerospace Projects
Complexity and Learning Curve
While C++ is powerful, its complexity can present challenges for newcomers. Mastering its nuances requires dedication and practice. New programmers must be prepared to engage deeply with the language and its best practices.
Safety and Reliability
In aerospace applications, safety and reliability are paramount. Implementing effective error handling enhances system robustness. C++ provides mechanisms for exception handling that are crucial in scenarios where software failures could have dire consequences:
try {
// Risky operation, such as interfacing with hardware
} catch (const std::exception& e) {
std::cerr << "An error occurred: " << e.what() << std::endl;
}
Ensuring that aerospace software can gracefully handle exceptions is non-negotiable, solidifying trust in the systems deployed.
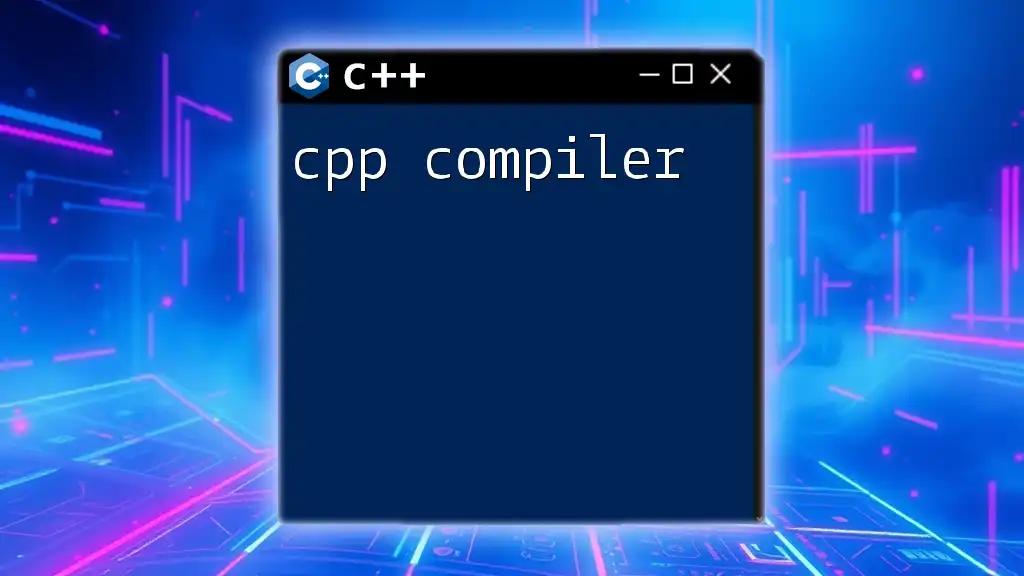
Future Trends in C++ for Aerospace
Adoption of C++20 and Beyond
As technology evolves, so too does C++. The adoption of C++20 introduces several new features that can enhance aerospace applications, such as ranges and coroutines, that can streamline data processing and asynchronous tasks.
Emerging Technologies
In addition, the incorporation of emerging technologies like AI and machine learning within C++ frameworks is reshaping aerospace innovation. C++ offers the performance needed to run these advanced algorithms, proving invaluable in autonomous flight systems and operational optimization.
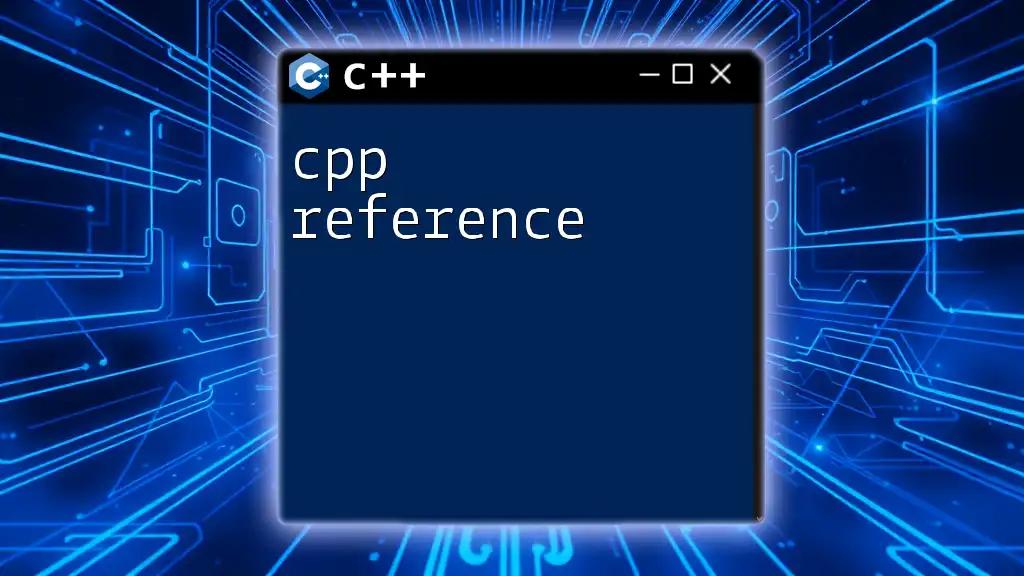
Conclusion
C++ in the aerospace sector is not merely a toolkit; it stands as a cornerstone of innovative development, ensuring that aircraft operate safely and efficiently. From flight simulations to avionics, the language's capabilities align perfectly with the industry's demanding needs. Engaging with C++ offers budding engineers an unparalleled opportunity to champion the future of aerospace technology. Now is the time to learn and excel in C++ aerospace, setting the stage for a rewarding career in this vital and evolving field.

Additional Resources
Seek further learning through recommended books, online courses, and communities dedicated to C++ and aerospace. Engaging with others in the field can provide invaluable insights and foster growth in your understanding and application of C++.