C++ programming is a powerful, high-level language that enables developers to create efficient software by utilizing object-oriented features, strong type checking, and system-level access.
Here’s a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Getting Started with C++ Coding
Setting Up Your Development Environment
Installing a C++ Compiler
Before diving into C++ programming, the first step is to install a C++ compiler. Common options include GCC (GNU Compiler Collection), Clang, and MSVC (Microsoft Visual C++). Each compiler has its distinct advantages and may suit different development environments. For example, GCC is widely used on Linux, Clang is known for its modern features and diagnostics, and MSVC is tailored for Windows users.
To install GCC, run the following commands on your terminal (for Linux):
sudo apt update
sudo apt install build-essential
For Windows users, you can download MinGW, which includes GCC:
- Download MinGW from the official website.
- Follow the installation instructions, ensuring you check the box for installing the C++ compiler.
Choosing an IDE
Selecting the right Integrated Development Environment (IDE) can significantly enhance your coding experience. Popular IDEs for C++ programming include Code::Blocks, Visual Studio, and CLion. Each IDE provides different features:
- Code::Blocks: Lightweight and user-friendly, ideal for beginners.
- Visual Studio: Comprehensive IDE packed with advanced features and debugging tools.
- CLion: JetBrains' powerful IDE that offers a range of features for advanced C++ development.
Creating Your First C++ Project
Once your IDE and compiler are set up, you're ready to create your first C++ project. Open your IDE, initiate a new project, and select “Console Application.” A simple example of a “Hello, World!” program can help you test the setup:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This simple program includes the required library, uses the standard namespace, and outputs the classic message to the console.
Writing Basic C++ Syntax
Understanding C++ Structure
C++ programs are structured into functions, with the `main` function serving as the entry point. Every executable C++ program must contain a `main` function.
Variables and Data Types
In C++, variables are used to store data. The language supports various data types such as:
- `int`: for integers.
- `float`: for floating-point numbers.
- `char`: for character data.
- `string`: for string data (standard library).
Declaring and using variables is straightforward. Here’s an example of declaring different data types:
int age = 25; // integer type
float height = 5.9; // floating-point type
char initial = 'A'; // character type
These simple declarations enable you to use the variables in subsequent calculations or print statements.
Control Structures in C++
Conditional Statements
Conditional statements allow your program to make decisions based on specific conditions. The if-else construct is fundamental in C++. Here’s how it works:
if (age >= 18) {
cout << "Adult";
} else {
cout << "Minor";
}
This code snippet checks if `age` is 18 or above and outputs whether the person is an adult or minor.
Loops
Loops enable repetitive execution of code blocks. The for loop and while loop are two of the most common types.
For Loop:
for (int i = 0; i < 5; i++) {
cout << i << " ";
}
In this snippet, the loop iterates from 0 to 4, printing each value.
While Loop:
The while loop continues execution as long as the specified condition holds true. Here’s a quick example:
int count = 0;
while (count < 5) {
cout << count << " ";
count++;
}
This loop performs the same task as the previous for loop but uses a different structure.
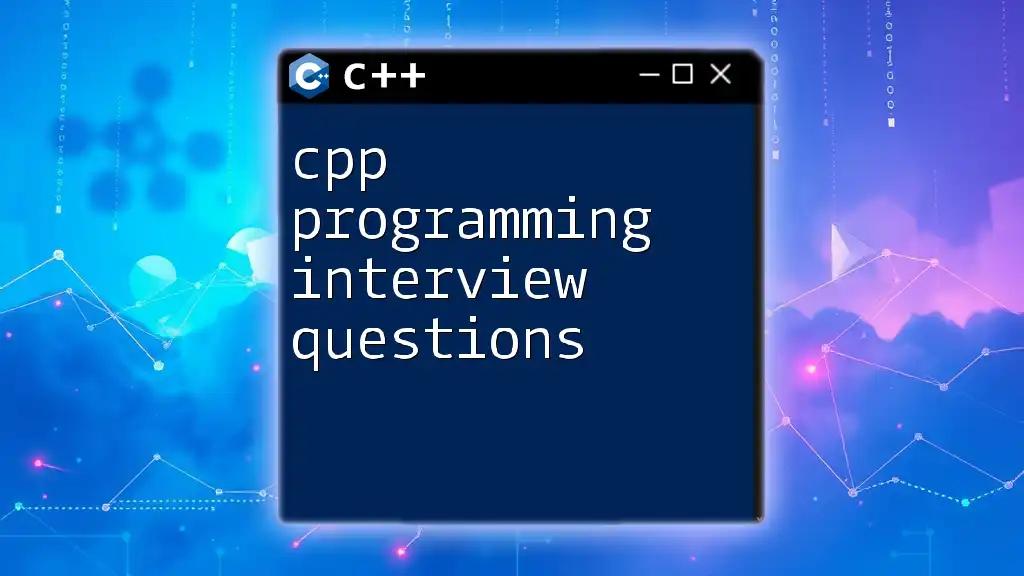
Advanced C++ Concepts
Functions in C++
Functions play a crucial role in code organization and reuse. A function must be defined with a specific return type, a name, parentheses for parameters, and a body encapsulated in curly braces.
Defining and Calling Functions
Here's an example of defining and calling a function in C++:
void greet() {
cout << "Hello, welcome to C++ programming!" << endl;
}
int main() {
greet(); // Calling the function
return 0;
}
Default Arguments and Function Overloading
C++ allows functions to have default arguments, which can be handy in certain scenarios. Here’s an example:
void printInfo(string name, int age = 18) {
cout << name << " is " << age << " years old." << endl;
}
With this function, if you call `printInfo("John")`, it will default `age` to 18.
Function overloading allows you to create multiple functions with the same name but different parameter lists, enhancing code readability and usability.
Object-Oriented Programming in C++
Understanding Classes and Objects
C++ is an object-oriented programming language, which means it is designed around the concept of "objects" that encompass data and methods. To define a class:
class Animal {
public:
void speak() {
cout << "Animal speaks" << endl;
}
};
You can create an object of the class and call its method as follows:
Animal myAnimal;
myAnimal.speak(); // Output: Animal speaks
Encapsulation, Inheritance, and Polymorphism
Encapsulation involves bundling the data and methods that operate on the data within one unit, typically a class. Inheritance allows one class (subclass) to inherit the attributes and methods of another (superclass), facilitating code reuse. Polymorphism enables methods to do different things based on the object that it is acting upon, thus providing a powerful way to create interfaces.
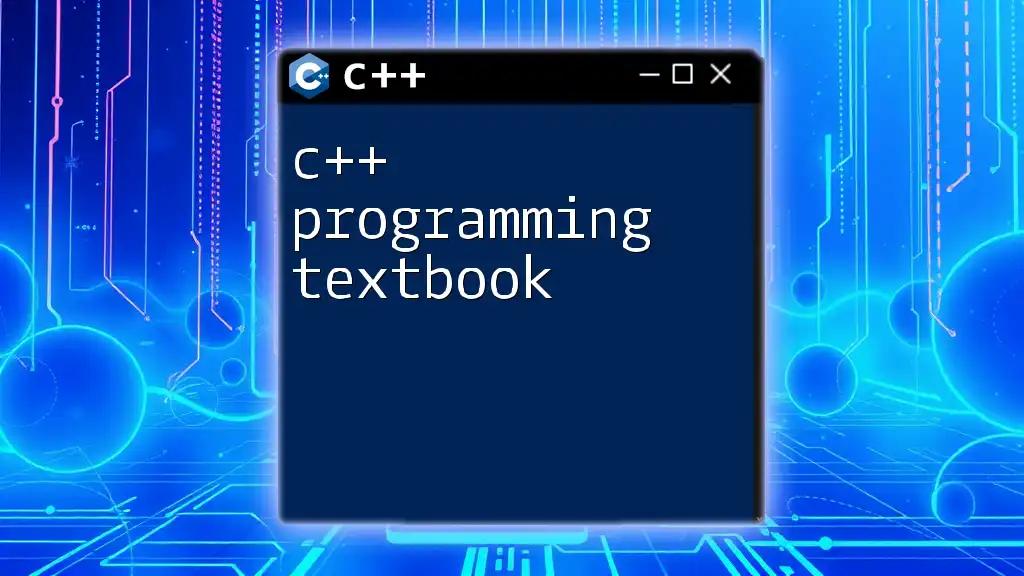
Best Practices in C++ Programming
Code Optimization Techniques
Memory Management
Effective memory management is crucial in C++ programming to ensure that applications run efficiently. Using pointers and references can provide more control over memory usage, but they must be handled cautiously to avoid memory leaks.
int* ptr = new int; // Dynamic memory allocation
delete ptr; // Deallocating memory to prevent leaks
Avoiding Common Pitfalls
C++ can be tricky, especially for beginners. Common pitfalls include using uninitialized variables, neglecting proper memory management, and mismanaging object lifetimes. Always initialize your variables and be mindful of resource allocation and deallocation.
Writing Readable Code
Naming Conventions
Employing clear and consistent naming conventions is essential for readability. Function and variable names should be descriptive and convey their purpose. For instance, instead of naming a variable `x`, use `age`.
Commenting Your Code
Comments are invaluable for maintaining and understanding code. Use comments to explain complex logic and provide context, but strive to keep them concise and relevant. For example:
// This function calculates the area of a circle
float calculateArea(float radius) {
return 3.14 * radius * radius; // Area formula
}
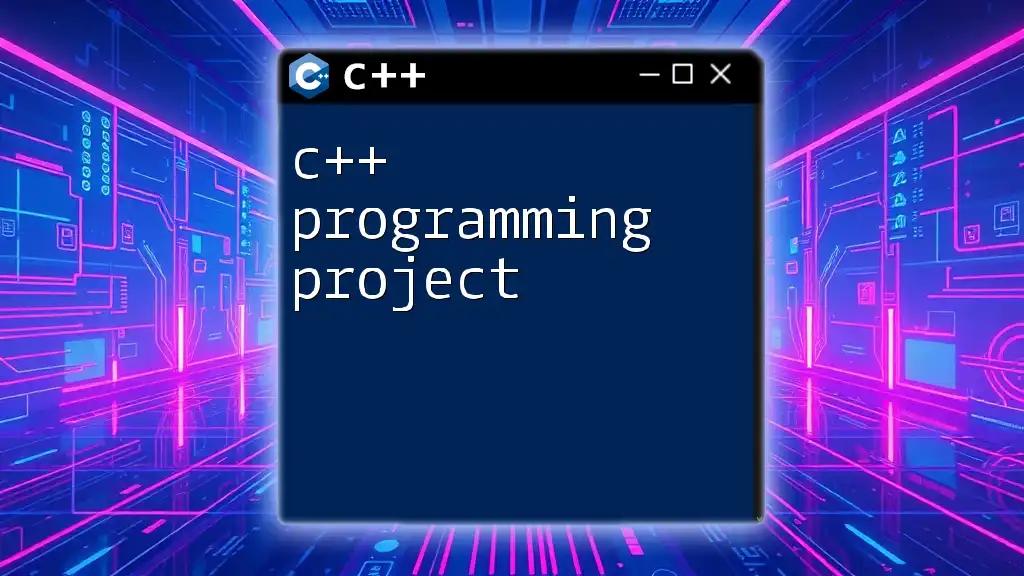
Resources for Further Learning
To enhance your C++ programming skills, consider following online courses and tutorials tailored for both beginners and intermediate developers. Platforms like Coursera, Udemy, and Codecademy offer structured learning paths.
Books like “C++ Primer” by Stanley B. Lippman and “Effective C++” by Scott Meyers are excellent references for deepening your understanding of C++.
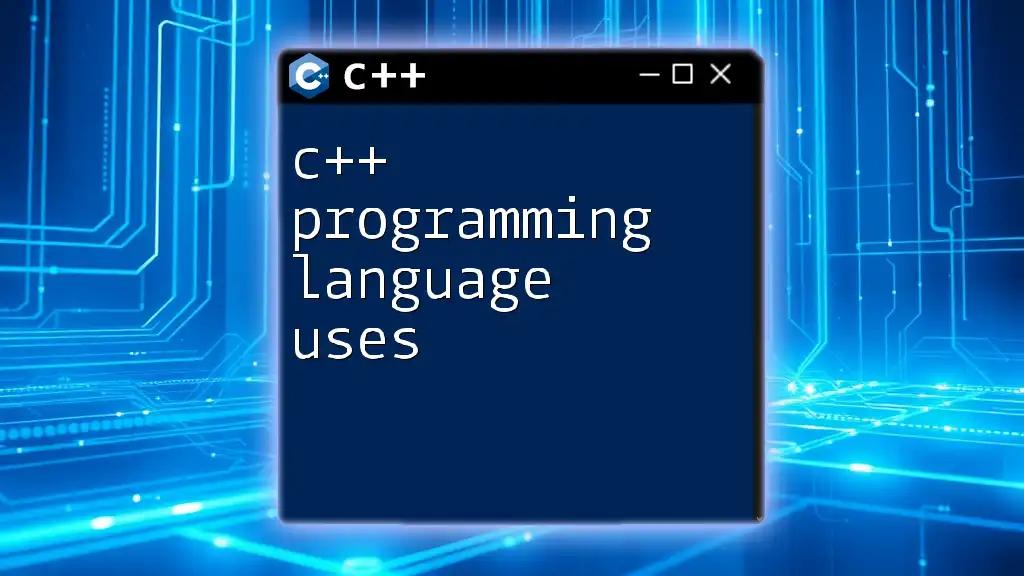
Conclusion
C++ programming is a powerful skill that opens doors to numerous opportunities in software development. By mastering its syntax, control structures, and advanced concepts, you can develop robust applications across various domains. Continuous learning and practice are key to becoming a proficient C++ programmer.
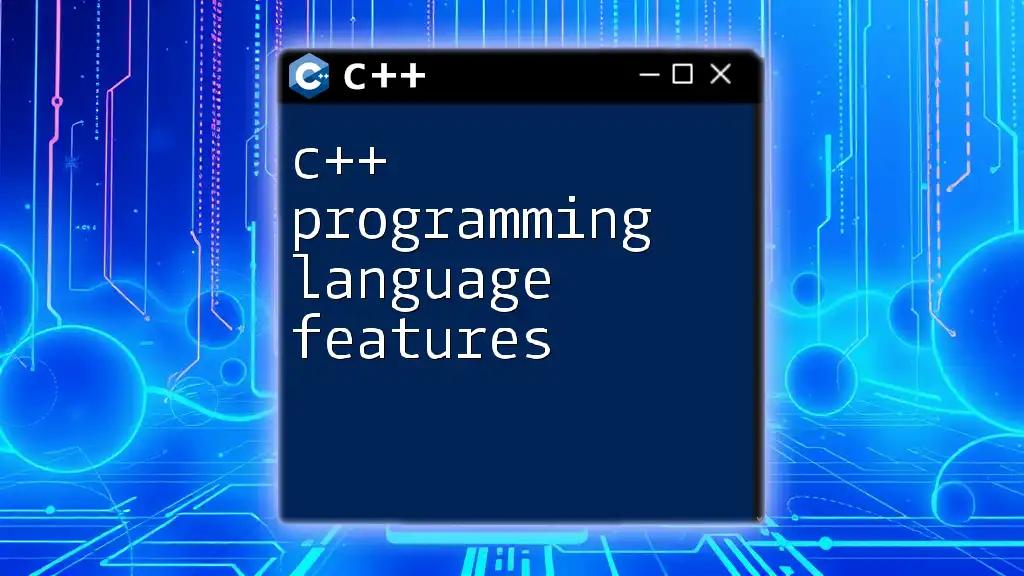
Call to Action
Join our C++ coding community! Engage with peers and gain access to additional resources to accelerate your learning journey in C++ programming. Whether you are starting from scratch or looking to improve your skills, we're here to support you every step of the way.