C++ is a versatile programming language widely used for system/software development, game development, and performance-critical applications due to its efficiency and control over system resources.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Simple C++ program to display a message
return 0;
}
What is C++?
C++ is an extension of the C programming language that incorporates both procedural and object-oriented programming paradigms. Developed by Bjarne Stroustrup in the early 1980s, C++ was designed to add features like classes and objects to the existing C language while maintaining high efficiency and performance. This flexibility makes it a powerful tool for various applications.
A Brief History of C++
Since its inception, C++ has undergone significant evolution, adapting to meet the needs of modern software development. From its early days as a systems programming language, C++ has expanded into multiple domains, making it a fundamental language in the tech industry.
Key Features of C++
Object-oriented programming (OOP) is at the heart of C++, allowing developers to create objects that combine data and methods. Other key features include:
- High performance: C++ provides low-level memory control, allowing developers to optimize application performance, a critical factor in many software solutions.
- Rich standard library: C++ boasts a comprehensive standard library that facilitates tasks such as file handling, data manipulation, and more, streamlining the development process for programmers.
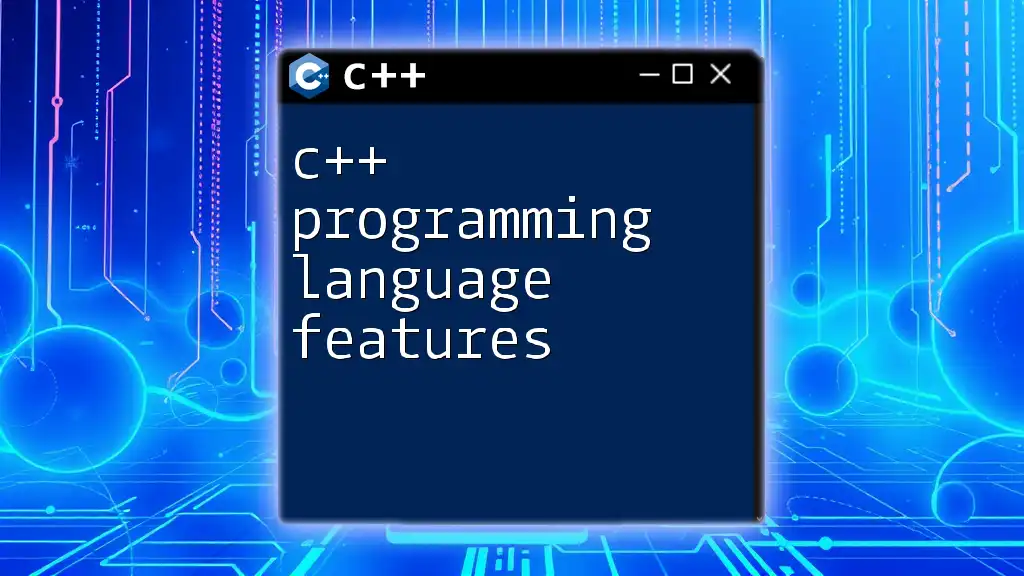
Software Development
General Software Development
C++ is widely used for general software development, making it a versatile option for various applications including operating systems, desktop applications, and even some web development components. Its ability to interact directly with hardware gives it a huge advantage in systems programming.
Example: A simple "Hello World" program in C++ demonstrates the syntax and structure that developers will encounter:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Game Development
The gaming industry frequently relies on C++ due to its performance capabilities and extensive libraries. Many popular game engines such as Unity and Unreal Engine are built on C++, enabling rapid development of graphics-intensive applications.
Developers can delineate game logic clearly, as shown in this basic game logic snippet:
class Player {
public:
void jump() {
// Logic for jumping
}
};
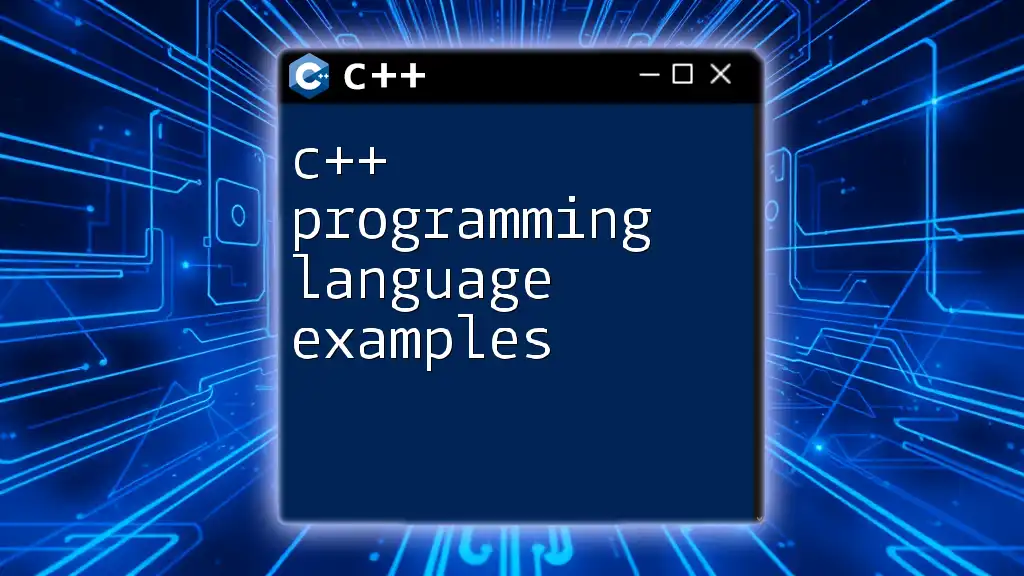
Systems Programming
Operating Systems
C++ plays a crucial role in developing operating systems. Its ability to manage resources efficiently makes it ideal for system-level software. Key operating systems such as Windows and various Linux distributions have components written in C++.
Example: A simple utility program for file management might look like this:
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ofstream myfile;
myfile.open("example.txt");
myfile << "Writing to a file.\n";
myfile.close();
return 0;
}
Embedded Programming
Embedded systems, which are designed to perform dedicated functions within larger systems, often utilize C++. From microcontrollers to IoT devices, C++ allows fine control over hardware, making it a staple in this segment.
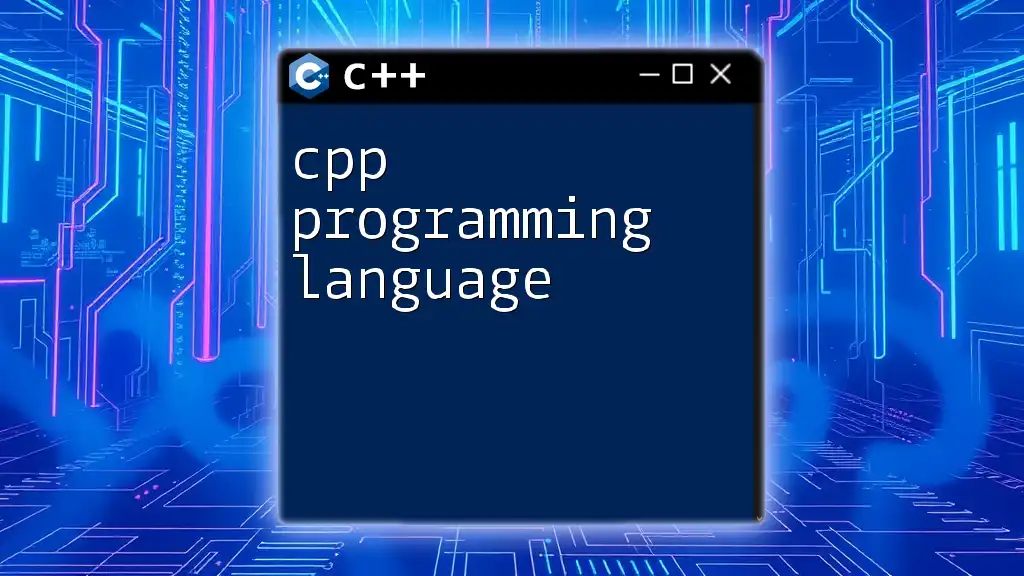
Performance-Critical Applications
High-Performance Computing (HPC)
C++ shines in performance-critical applications, particularly in fields demanding intensive computational capabilities like scientific simulations and financial modeling. Its proximity to hardware allows for optimizations that are essential for systems that run complex calculations efficiently.
Developers often profile their code for performance benchmarking:
#include <iostream>
#include <chrono>
void performHeavyComputation() {
// Some intensive calculations
}
int main() {
auto start = std::chrono::high_resolution_clock::now();
performHeavyComputation();
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Time taken: " << std::chrono::duration_cast<std::chrono::microseconds>(end - start).count() << " microseconds." << std::endl;
return 0;
}
Real-Time Systems
In real-time applications, such as those found in telecommunications and automotive software, C++ provides the speed necessary for timely processing and responsiveness. The language's efficiency allows for minimal lag between input and system response, which is crucial in many industrial applications.
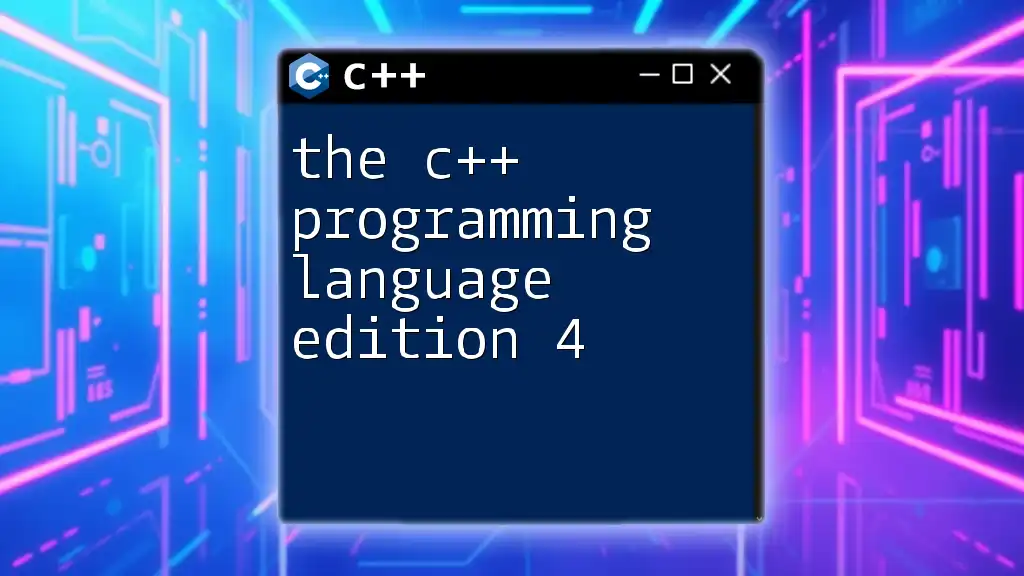
Application in Web Development
Backend Development
C++ is also making strides in web development, especially on the backend. Frameworks such as Node.js and Wt allow developers to build high-performance server-side applications. The efficiency of C++ can significantly enhance the performance of web services.
Example: Here's a snippet showcasing a basic backend application using the Wt framework:
#include <Wt/WApplication.h>
#include <Wt/WText.h>
class HelloWorld : public Wt::WApplication {
public:
HelloWorld(const Wt::WEnvironment& env) : Wt::WApplication(env) {
setTitle("Hello World App");
root()->addWidget(std::make_unique<Wt::WText>("Hello, World!"));
}
};
Wt::WApplication *createApplication(const Wt::WEnvironment& env) {
return new HelloWorld(env);
}
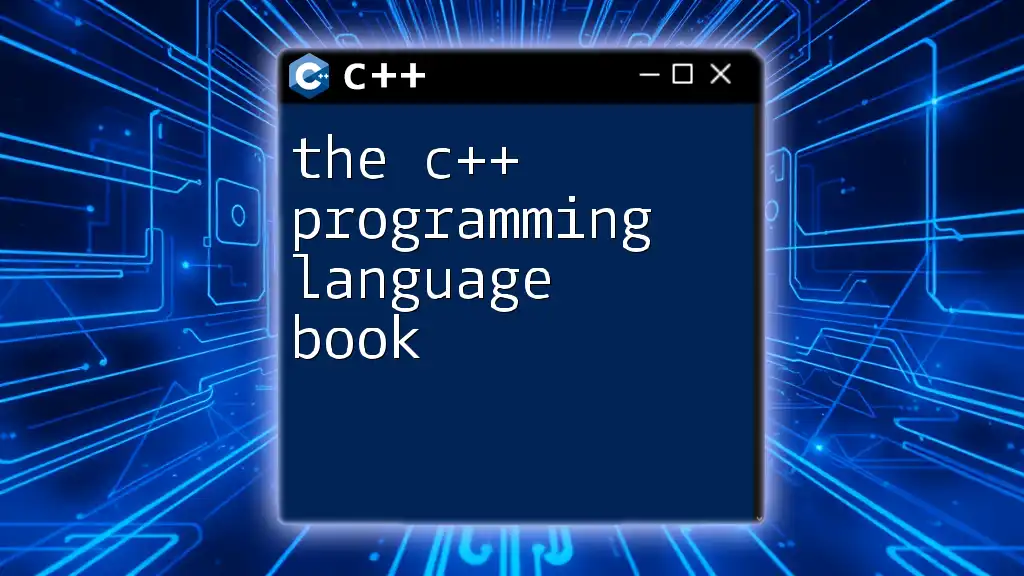
Financial Sector
Banking and Financial Services
C++ is heavily utilized in the banking and financial sectors due to its ability to process vast amounts of data swiftly. It’s employed in algorithmic trading systems where split-second decisions can result in substantial financial gains or losses.
C++ enables the development of powerful algorithms that analyze market trends and execute trades automatically.
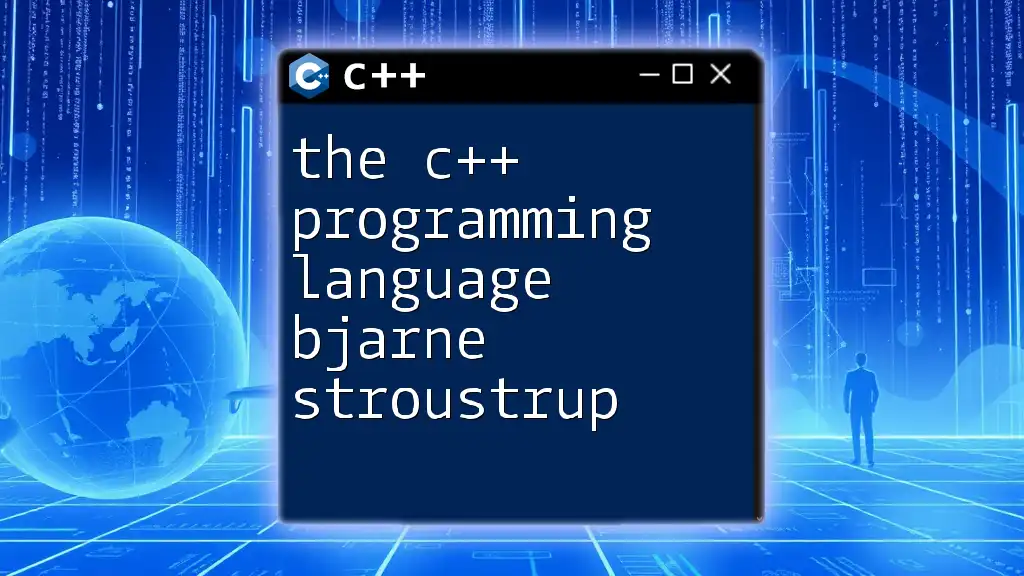
GUI Applications
Graphical User Interfaces
C++ is often favored for developing graphical user interfaces (GUIs) through libraries like Qt and wxWidgets. These libraries provide developers with tools to create rich, interactive applications swiftly.
Example: A simple GUI application using Qt could follow this structure:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.resize(200, 100);
button.show();
return app.exec();
}
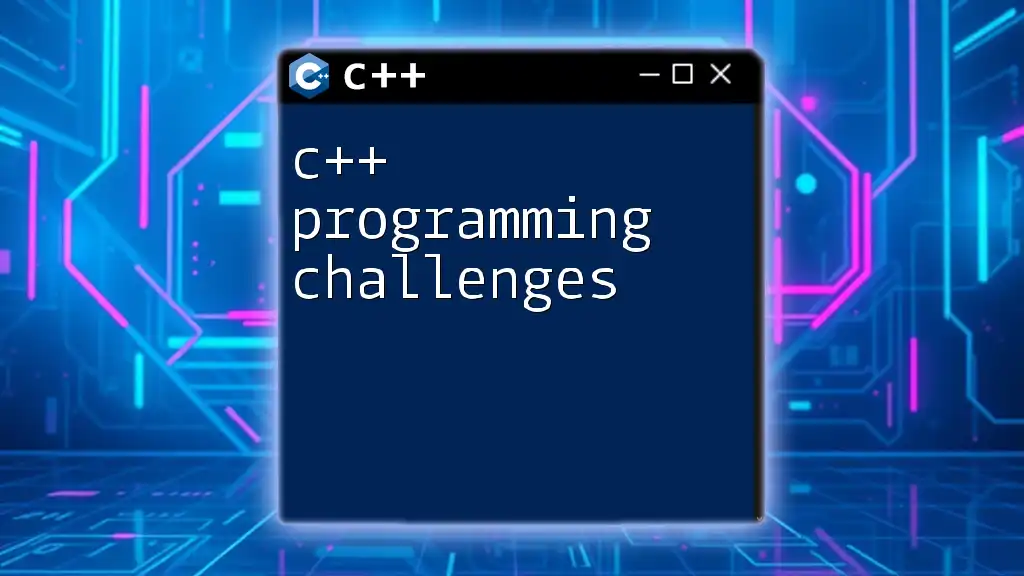
Scientific and Engineering Applications
Simulation and Modelling
In scientific fields, C++ is a key player in simulations and modeling tasks. Libraries like ROOT for data analysis in physics and OpenCV for computer vision applications exemplify C++’s significance in scientific computing.
Computer-Aided Design (CAD)
Moreover, C++ is crucial in developing Computer-Aided Design (CAD) software. Applications that facilitate design and engineering processes heavily rely on the capabilities offered by C++.
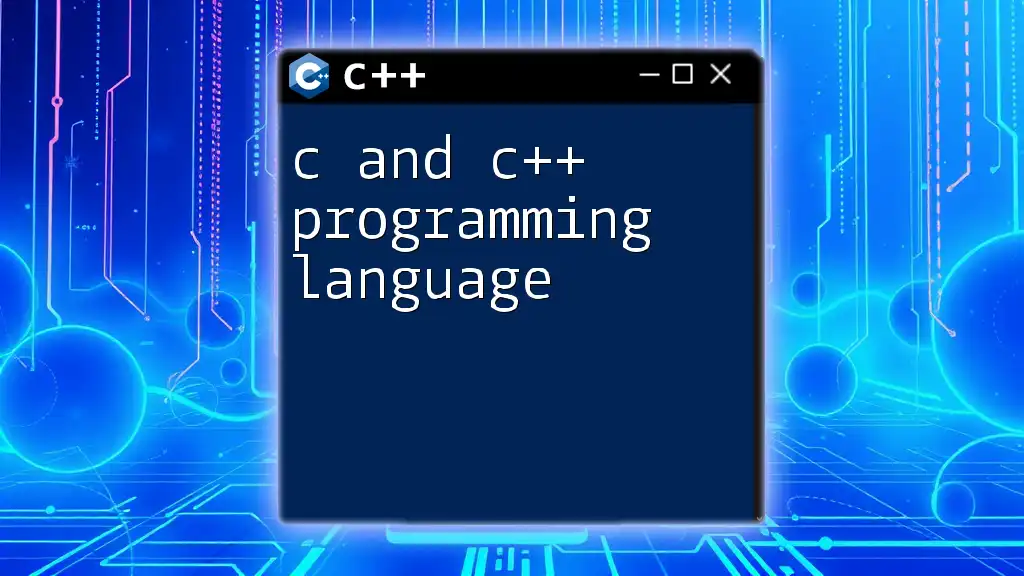
Conclusion
Understanding the C++ programming language uses can significantly inform your path as a developer. Its versatility, performance, and efficiency make it indispensable in software development, gaming, systems programming, and various other fields. If you’re eager to harness its power for your projects, consider exploring dedicated courses to deepen your knowledge and skills.