C++ language keywords are reserved words that have a special meaning in the programming language and cannot be used as identifiers, playing a crucial role in defining the structure and functionality of the code.
Here's a simple example using some C++ keywords:
#include <iostream>
using namespace std;
int main() {
int number = 10; // 'int' is a keyword to declare an integer variable
if (number > 0) { // 'if' is a keyword to create a conditional statement
cout << "Positive Number"; // 'cout' is used for output
}
return 0; // 'return' is used to exit the program with a status code
}
Understanding C++ Keywords
What are C++ Keywords?
C++ keywords are reserved words that have a predefined meaning within the C++ programming language. These keywords are integral to the syntax and functionality of C++, guiding the compiler in executing various operations. Unlike identifiers (such as variable names), which can be defined by the programmer, keywords are immutable; they can only serve their intended purpose.
Characteristics of C++ Keywords
C++ keywords possess distinct characteristics:
-
Case Sensitivity: C++ keywords are case-sensitive, meaning `int` and `Int` are treated differently. Keywords must always be used in lowercase to be recognized by the compiler.
-
Pre-defined Meaning: Each keyword has a specified role within the language. For example, `if` is used for conditional execution, while `class` is essential for object-oriented programming.
-
Reserved for Specific Functions: Keywords cannot be used as identifiers or variable names. Attempting to do so would result in a compilation error, ensuring consistency and reliability in code interpretation.
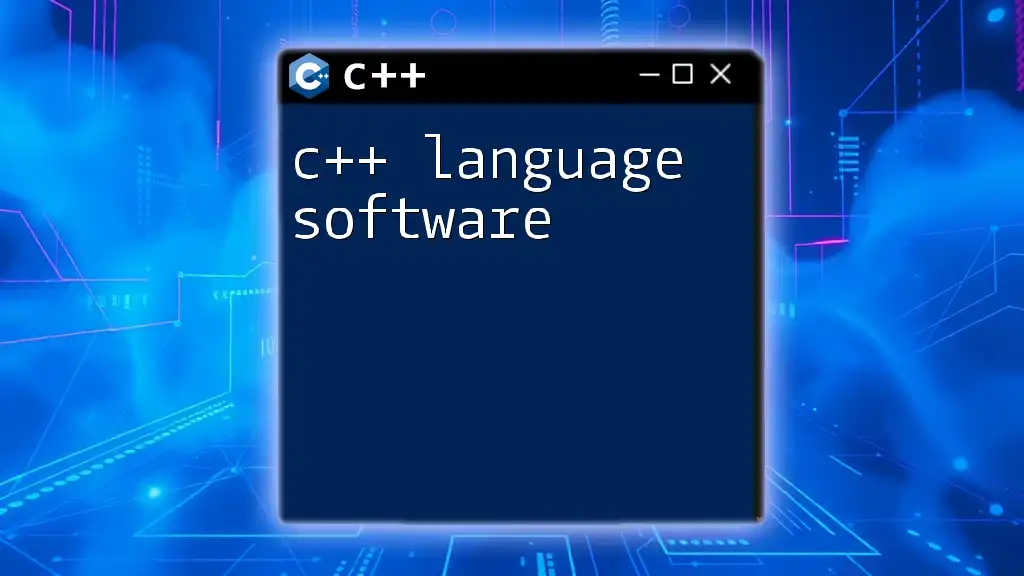
Comprehensive List of C++ Keywords
Core Keywords in C++
Understanding the core keywords is fundamental when learning C++. Here are some essential categories:
Control Flow Keywords: These keywords direct the flow of logic in a program.
- `if`: Evaluates a condition and executes code if true.
- `else`: Executes an alternative block of code when the `if` condition is false.
- `switch`: Allows multi-way branching based on the value of a variable.
Example:
int x = 10;
if (x > 5) {
// Code executes here
} else {
// Code executes here
}
Data Type Keywords: These keywords define the data types used in a program.
- `int`: Represents integer values.
- `char`: Represents single characters.
- `float`, `double`: Represent floating-point numbers for various precision levels.
Example:
int age = 30;
char initial = 'A';
float height = 5.9;
Class and Object Keywords: Essential for object-oriented programming.
- `class`: Declares a new class.
- `struct`: Similar to classes but defaults to public access.
- `public`, `private`, `protected`: Define access control for class members.
Example:
class Person {
public:
string name;
int age;
};
Additional Keywords
Storage Class Keywords: Control the lifespan and visibility of variables.
- `auto`: Automatically deduces the type of a variable.
- `static`: Preserves the variable's value across function calls.
- `extern`: Indicates that a variable is defined elsewhere.
Example:
static int count = 0; // count retains its value between function calls
Exception Handling Keywords: Key for managing errors and exceptional circumstances.
- `try`: Begins a block of code that may throw exceptions.
- `catch`: Defines the response to an exception.
- `throw`: Used to raise an exception.
Example:
try {
// Code that may throw an exception
} catch (const exception& e) {
// Handle exception
}
Miscellaneous Keywords: These keywords provide additional functionalities.
- `namespace`: Used to organize code entities and avoid naming conflicts.
- `using`: Simplifies code by allowing access to namespace members.
- `typedef`: Creates new names for existing data types.
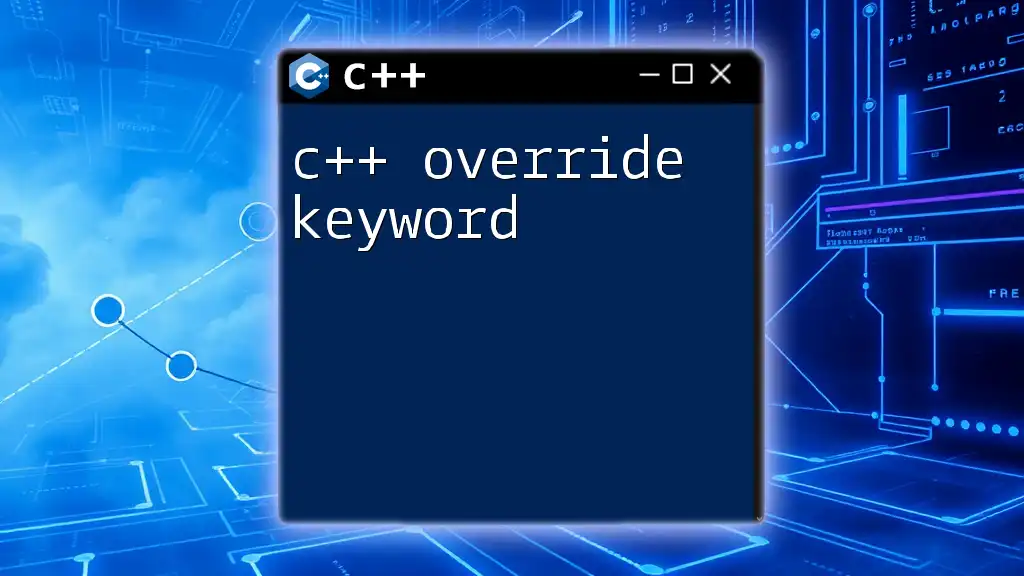
The Role of Keywords in C++
How to Use Keywords Effectively
Understanding and effectively using keywords is vital for writing clean, efficient C++ code. Familiarity with the purposes and constraints of each keyword helps in structuring your programs logically. Follow best practices, such as:
- Use appropriate keywords to enhance code readability.
- Stick to conventions and be mindful of keyword placement in your code.
Common Mistakes with C++ Keywords
Beginners often make mistakes when using keywords, which can hinder compilation and functionality.
- Misusing keywords: For example, trying to use `if` as a variable name will result in a compilation error.
Example:
int if = 5; // This will cause a compilation error
- Ignoring case sensitivity: Using `int` as `Int` leads to undefined behavior.
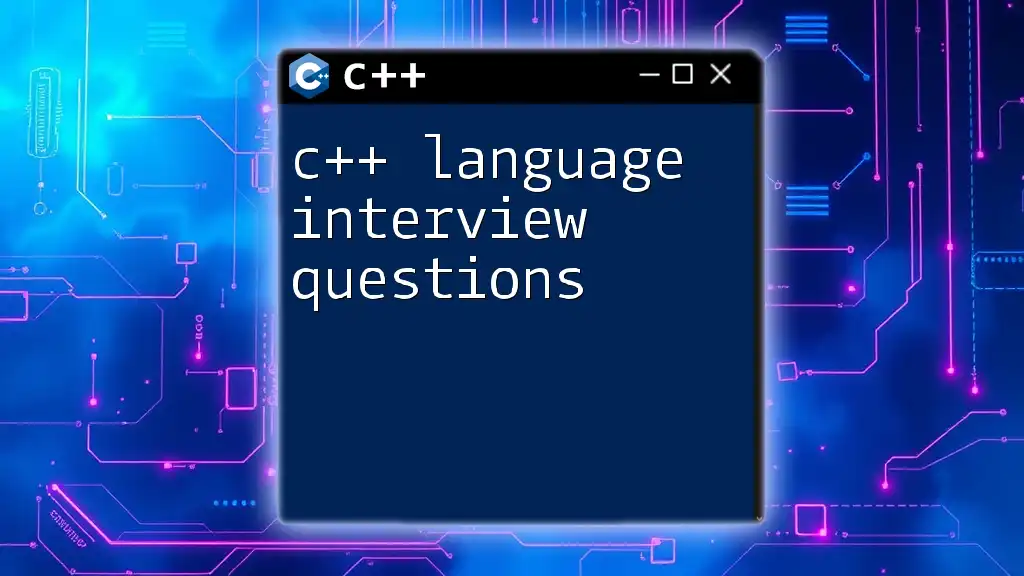
Practical Examples Using C++ Keywords
Creating a Simple C++ Program
A simple program demonstrating several C++ keywords:
#include <iostream>
using namespace std;
class Vehicle {
public:
void start() {
cout << "Vehicle started." << endl;
}
};
int main() {
Vehicle car; // 'Vehicle' is a class keyword
car.start(); // Accessing method using an object
return 0; // Exiting the program
}
In this example, keywords like `class`, `public`, and `return` illustrate their roles in defining a class and managing program execution.
Real-World Applications of C++ Keywords
Understanding C++ keywords is crucial in real-world programming. For instance, control flow keywords enable complex decision-making processes in software applications, while data type keywords ensure efficient memory management. Knowledge of keywords aids in debugging, optimizing performance, and enhancing code maintainability.
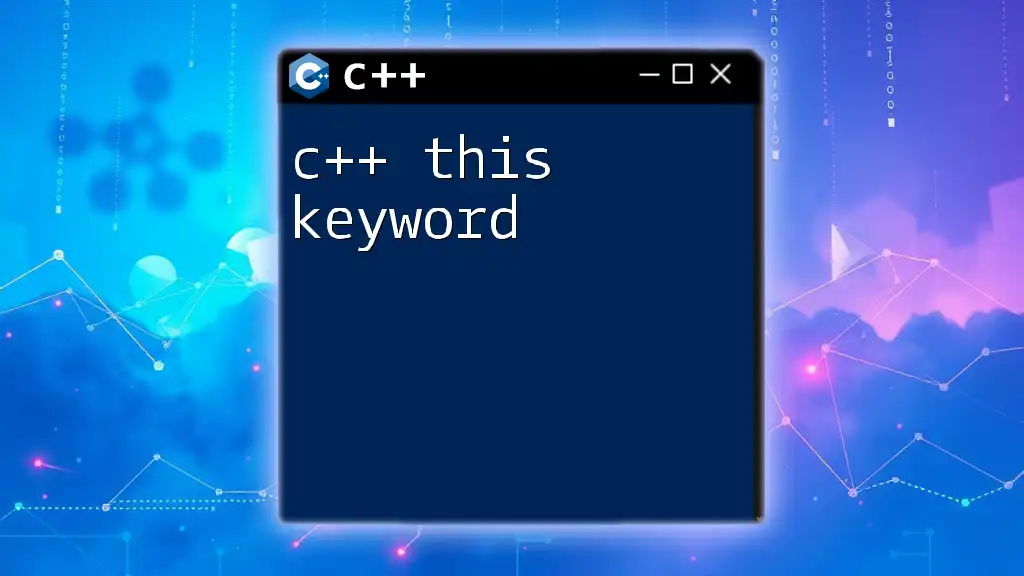
Conclusion
C++ language keywords are fundamental to programming within the C++ ecosystem. Their predefined roles provide structure and enhance code functionality. By mastering these keywords, programmers can write more efficient, readable code and effectively troubleshoot issues. Understanding keywords not only bolsters your C++ skills but also prepares you for advanced concepts in programming.
In conclusion, continue practicing with C++ keywords, explore their functionalities in-depth, and refer to additional resources as you develop your coding prowess.