The `and` keyword in C++ is a logical operator that serves as an alternative to the `&&` operator, used to perform logical conjunction in boolean expressions.
Here's an example of its usage in a code snippet:
#include <iostream>
int main() {
bool a = true;
bool b = false;
if (a and b) {
std::cout << "Both are true!" << std::endl;
} else {
std::cout << "At least one is false!" << std::endl;
}
return 0;
}
What is the `and` Keyword?
The `and` keyword in C++ is an alternative representation of the logical AND operator (`&&`). Its purpose is to evaluate two Boolean expressions, returning true only when both expressions are true. While the traditional `&&` operator is widely recognized, the `and` keyword enhances readability and offer an alternative for those seeking clearer code comprehension.
Why Use `and` Instead of `&&`?
Choosing `and` over `&&` can significantly improve code readability, especially for newcomers. Because `and` spells out the operation in plain language, it allows for easier understanding of the logic behind the conditions being checked. Additionally, it maintains consistency with other logical operators in C++, such as `or` and `not`.
For example, instead of writing:
if (a && b) {
// do something
}
you could write:
if (a and b) {
// do something
}
This choice can make code more accessible, especially in educational settings or collaborative projects where clarity is paramount.
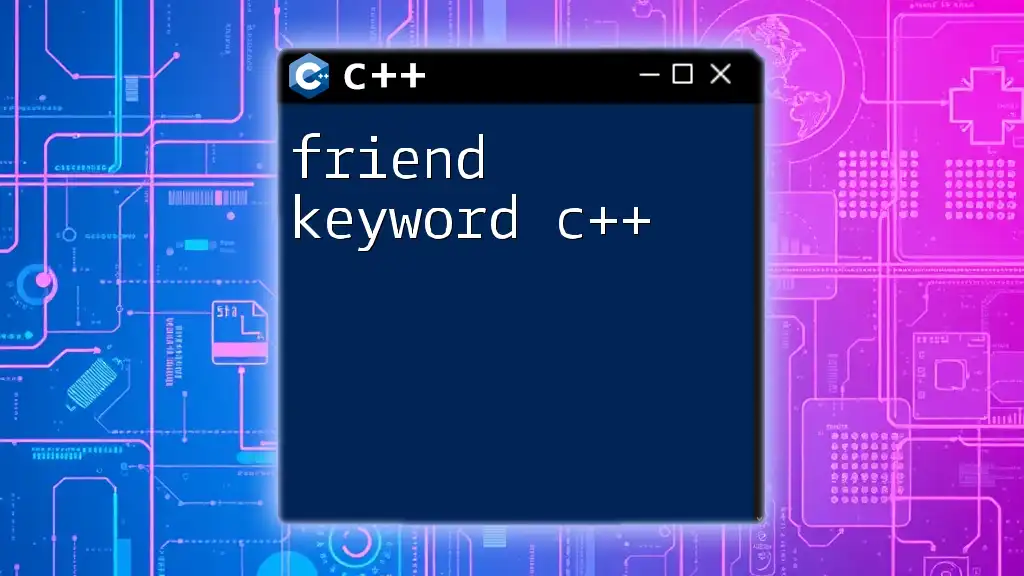
How to Use the `and` Keyword
Syntax of the `and` Keyword
The syntax for using the `and` keyword is straightforward. You can use it in conditional statements or expressions where logical evaluation is required. The structure typically involves two Boolean expressions:
expression1 and expression2
This evaluates to true if both `expression1` and `expression2` are true; otherwise, it returns false.
Examples of `and` in Practice
Example 1: Simple Boolean Operations
Consider this simple example where we check two Boolean variables:
#include <iostream>
using namespace std;
int main() {
bool a = true;
bool b = false;
if (a and b) {
cout << "Both are true.";
} else {
cout << "At least one is false.";
}
return 0;
}
In this code, the `if` statement checks whether both `a` and `b` are true. Since `b` is false, the output will be "At least one is false." This illustrates how the `and` keyword operates in a straightforward logical context.
Example 2: Combined Conditions
When checking multiple conditions, `and` proves useful:
#include <iostream>
using namespace std;
int main() {
int x = 10;
int y = 20;
if (x < 15 and y > 15) {
cout << "Both conditions are true.";
}
return 0;
}
This snippet verifies if `x` is less than 15 and `y` is greater than 15. Since both conditions are met, this block will execute, and the output will be "Both conditions are true."
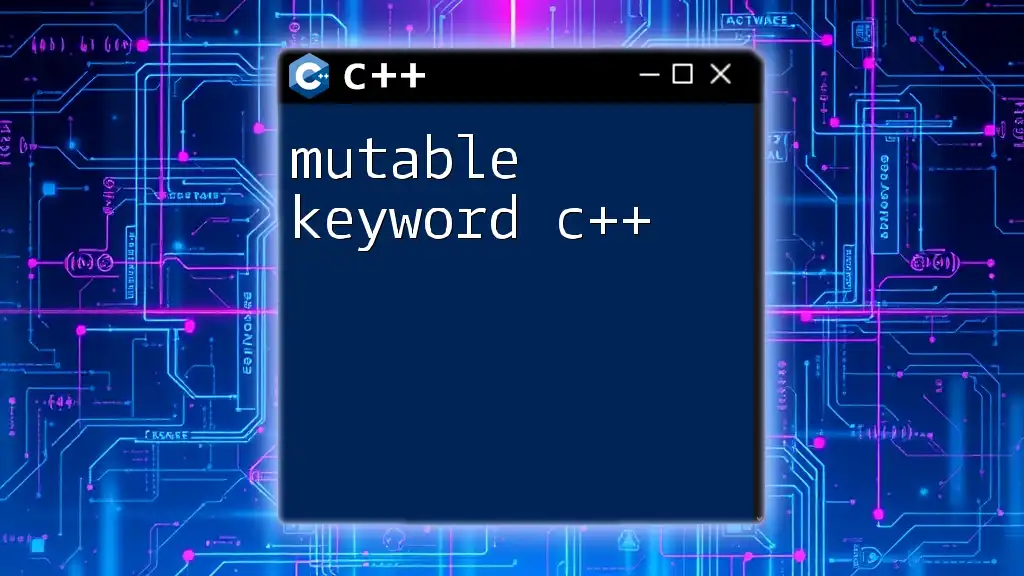
Alternatives to the `and` Keyword
Comparison with Other Operators
The `and` keyword is functionally equivalent to the `&&` operator, but it introduces a more readable format. Consider this comparison:
Using the `&&` operator:
if (a && b) {
// do something
}
Vs. using the `and` keyword:
if (a and b) {
// do something
}
Both expressions function identically; however, the `and` keyword can make the intent clearer, particularly in complex logical conditions.
Additionally, the C++ language offers other alternative keywords such as `or` for the logical OR (`||`) operator and `not` for the logical NOT (`!`) operator. Using consistent keywords for logical expressions can help streamline your code style.
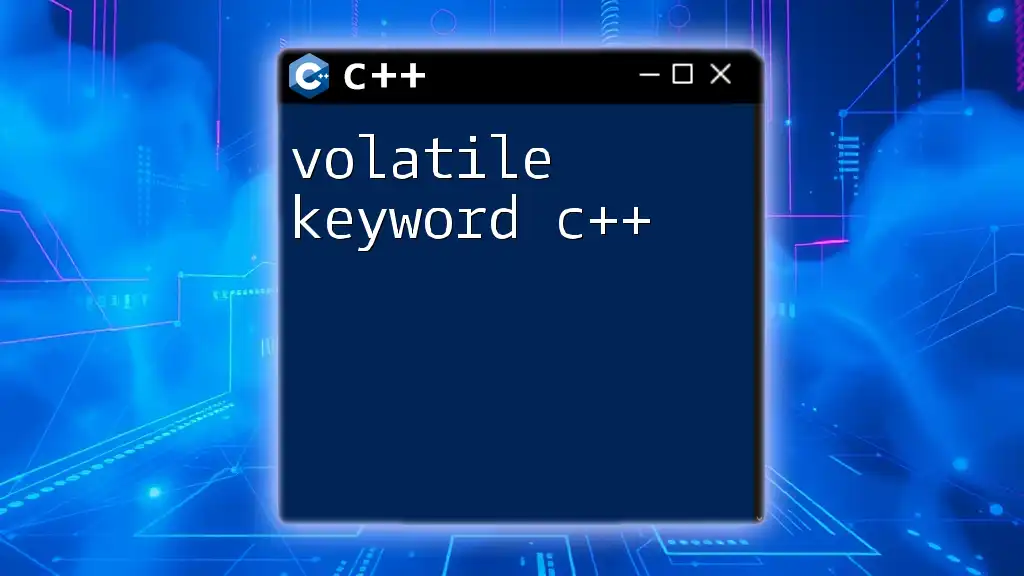
Best Practices for Using the `and` Keyword
When to Use `and`
Opt to use the `and` keyword in scenarios where you believe it will improve clarity, especially when collaborating with less experienced programmers. When teaching or sharing code, opting for `and` can help make logical expressions more intuitive and easier to follow.
Common Pitfalls
While the `and` keyword increases readability, some common mistakes programmers may make include:
- Misunderstanding how operator precedence works in conjunction with `and`. Always remember that parentheses can eliminate ambiguity.
- Assuming that the keywords will be universally understood; not every programmer prefers the alternative symbols. Clarify your coding style in team settings to maintain consistency.
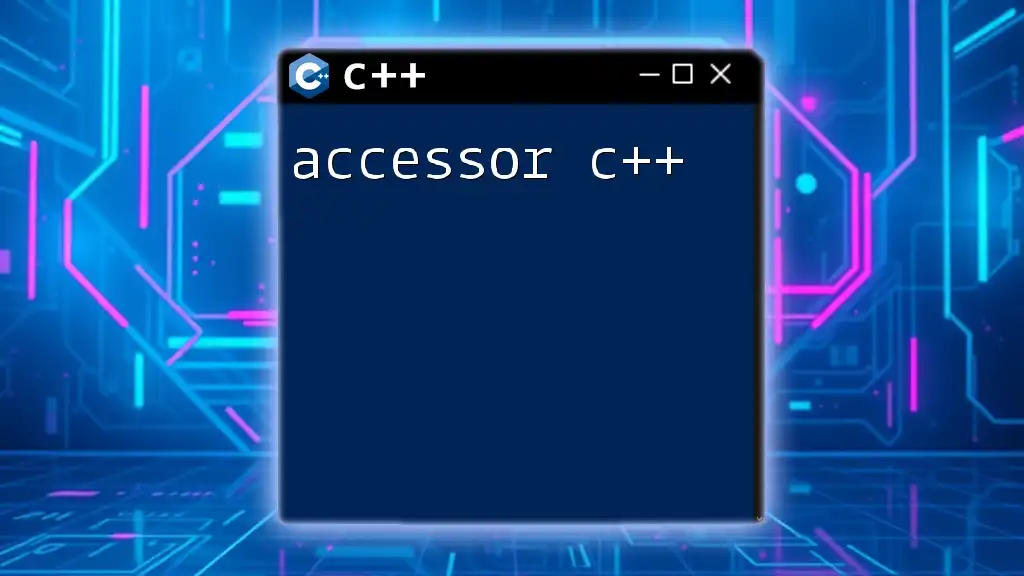
Conclusion
In summary, the `and` keyword in C++ provides a valuable alternative to the traditional `&&` operator, enhancing code readability and aiding comprehension, especially for beginners. By leveraging this keyword, you can write clearer, more intuitive code that more effectively communicates intent. We encourage you to practice using `and` in your own projects, potentially improving your programming style and clarity.
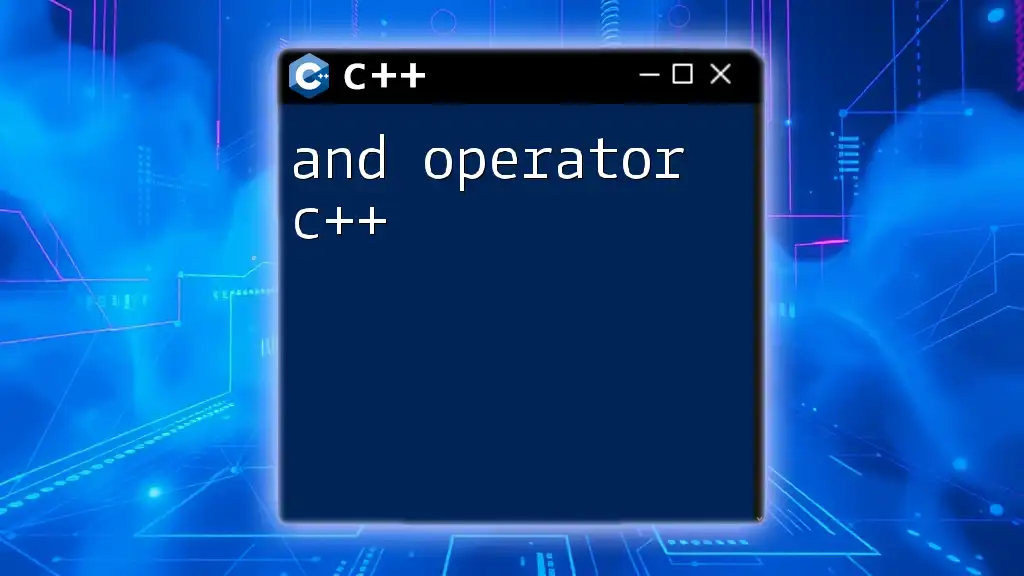
Additional Resources
For those interested in furthering their knowledge about C++ keywords, consider exploring the available textbooks, articles, and online resources. Engaging with online communities can also provide you with insights and assistance as you deepen your understanding of C++.
C++ Keyword Cheat Sheet
Don't forget to access our downloadable resource summarizing C++ keywords, including `and`, `or`, and `not`. It’s a handy guide for quick reference.
By committing to usage of the `and` keyword and improving your familiarity with C++, you take an essential step in advancing your programming skills. Happy coding!