The `volatile` keyword in C++ indicates that a variable may be changed unexpectedly, preventing the compiler from optimizing its access, which is crucial in scenarios like hardware access and multithreading.
Here's a code snippet demonstrating the use of the `volatile` keyword:
#include <iostream>
volatile int flag = 0; // This variable can be modified outside of the current thread
void updateFlag() {
flag = 1; // Simulating changing the flag from another thread or hardware interrupt
}
int main() {
while (flag == 0) {
// Wait for flag to become 1
}
std::cout << "Flag has been updated!" << std::endl;
return 0;
}
What is the Volatile Keyword in C++?
The volatile keyword in C++ is a type qualifier used to indicate that a variable's value may be changed unexpectedly. This means that the compiler cannot optimize accesses to the variable as it would with ordinary variables. The primary function of the volatile keyword is to inform the compiler that the variable may be modified by something outside the control of the current code, such as hardware or another thread.

Why Use the Volatile Keyword in C++?
When compiling, the C++ compiler applies various optimizations that can lead to assumptions about how variables are used. For instance, it may cache the value of a variable in a register, leading to unexpected behavior when that value is modified elsewhere. Here’s where the volatile keyword becomes critical.
The volatile keyword tells the compiler that it cannot make such assumptions and that it should always fetch the variable’s value from memory instead of relying on a cached value. This can prevent bugs in situations where the variable’s value changes asynchronously.
Important Scenarios for Using Volatile
- Hardware Access: In embedded systems, variables that hold state information for hardware devices are often declared as volatile because their values can change independently of the program’s execution flow.
- Multithreading: When multiple threads share a variable, marking it as volatile ensures that one thread always reads the most current value, preventing stale reads.

Mechanics of the Volatile Keyword in C++
Understanding how the volatile keyword works requires a grasp of the C++ compilation process. Without the volatile qualifier, the compiler is free to optimize the code, which could lead to errors if the variable is changed while the program is running.
When the volatile keyword is applied:
- The compiler generates code to always access the variable from memory every time it is read or written to.
- This distinct mechanism helps ensure that the program reflects the variable's latest state, irrespective of any optimizations during compilation.

Syntax for Declaring a Volatile Variable
Declaring a volatile variable is straightforward. You simply add the `volatile` qualifier before the variable type. Here’s a basic example:
volatile int counter;
In this snippet, `counter` is declared as a volatile int. This tells the compiler that the value of `counter` may change at any moment, and it should not optimize reads and writes to this variable.
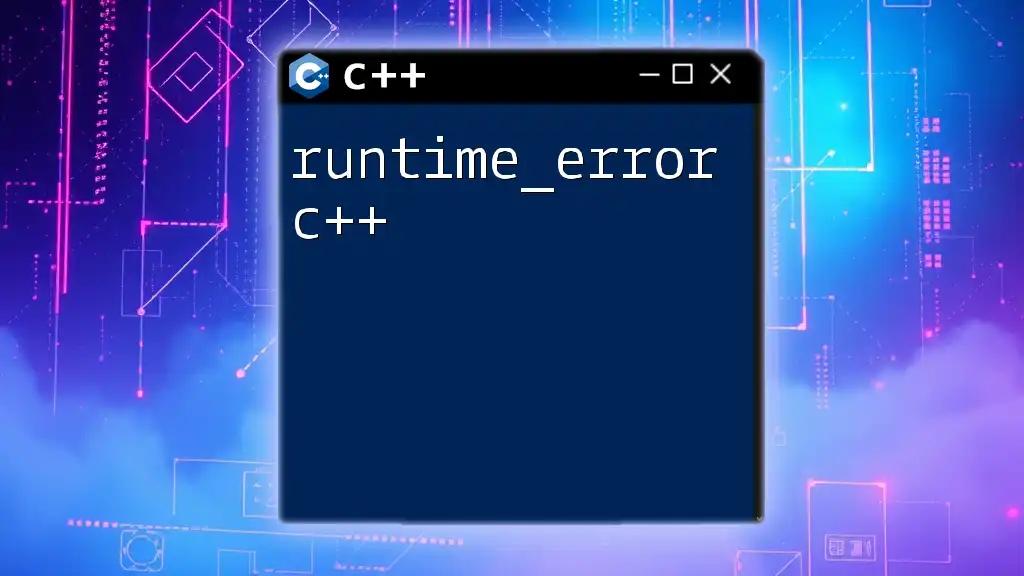
Common Use Cases for Volatile Variables
Typical Applications of Volatile in C++
The volatile keyword is predominantly used in scenarios where variable values are altered outside the normal sequence of operations.
- Hardware Interaction: When interfacing with hardware, registers may change state asynchronously, and using volatile ensures that these changes are recognized by the CPU.
- Inter-thread Communication: When variables share state across threads, declaring them as volatile retains the correct value even amidst competing operations from other threads.
Example Scenarios
-
Interaction with Hardware Registers:
When dealing with memory-mapped hardware registers, declaring the registers as volatile is crucial. For example:
volatile int *hardwareRegister = (int *)0x40021000;
This designation prevents compiler optimization that could cause unintended access issues.
-
Shared Memory Between Threads:
In a multithreading environment, a flag variable changed by one thread should be marked as volatile to ensure that other threads see the latest change:
volatile bool flag = false; void interrupt_handler() { flag = true; // set flag in interrupt context }
Differences Between Volatile and Other Keywords
Volatile vs. Const Keyword
While `volatile` tells the compiler that a variable can be changed unexpectedly, `const` signifies that a variable's value should not be changed after it is initialized. For example:
const int maxValue = 100; // cannot change maxValue
volatile int sharedValue; // value may change unexpectedly
Volatile vs. Atomic Variables
Atomic variables provide a higher level of synchronization and safety compared to volatile variables. Using `std::atomic` ensures proper memory ordering and guarantees that reads and writes are executed without interruption:
#include <atomic>
std::atomic<int> atomicCounter;
While `volatile` is useful for preventing compiler optimizations, it does not guarantee thread safety.
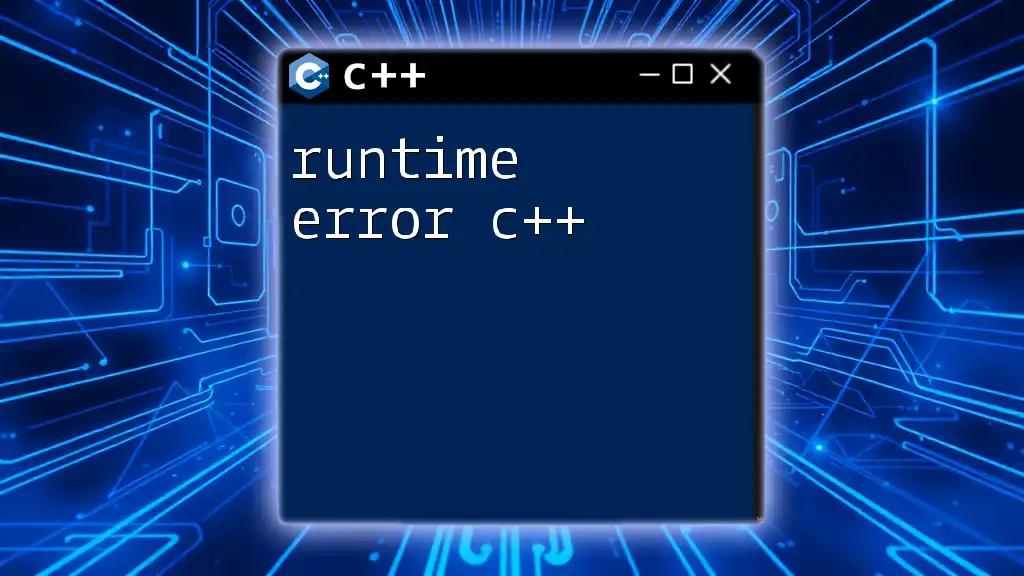
Best Practices for Using Volatile in C++
When using the volatile keyword in C++, consider the following best practices:
- Use sparingly: Only use volatile for variables that are genuinely shared between threads or accessed by hardware interrupts. Overusing volatile can result in code that is hard to understand and maintain.
- Understand its limitations: Remember that volatile does not ensure safety in multithreaded environments. Always consider using proper synchronization mechanisms where race conditions might occur.
- Code clarity: Use meaningful variable names to convey the variable's volatile nature and usage, thus enhancing the readability of your code.
Common Pitfalls to Avoid
- Misunderstanding Volatile and Data Races: While volatile informs the compiler not to cache values, it does not prevent data races from occurring in multithreaded applications.
- Performance Implications: Overuse can lead to performance degradation, as each access to a volatile variable will always hit memory.

Real-World Example: Using Volatile with Interrupts
In real-time systems, interrupt handlers commonly modify shared variables. It is critical to declare these variables as volatile to ensure that the main program logic always sees the most up-to-date value.
volatile bool flag = false;
void interrupt_handler() {
flag = true; // set flag in an interrupt context
}
void main_logic() {
while (!flag) {
// Wait for flag to be set by the interrupt
}
}
In this example, the main thread waits for the flag set by the interrupt handler without risking stale reads.
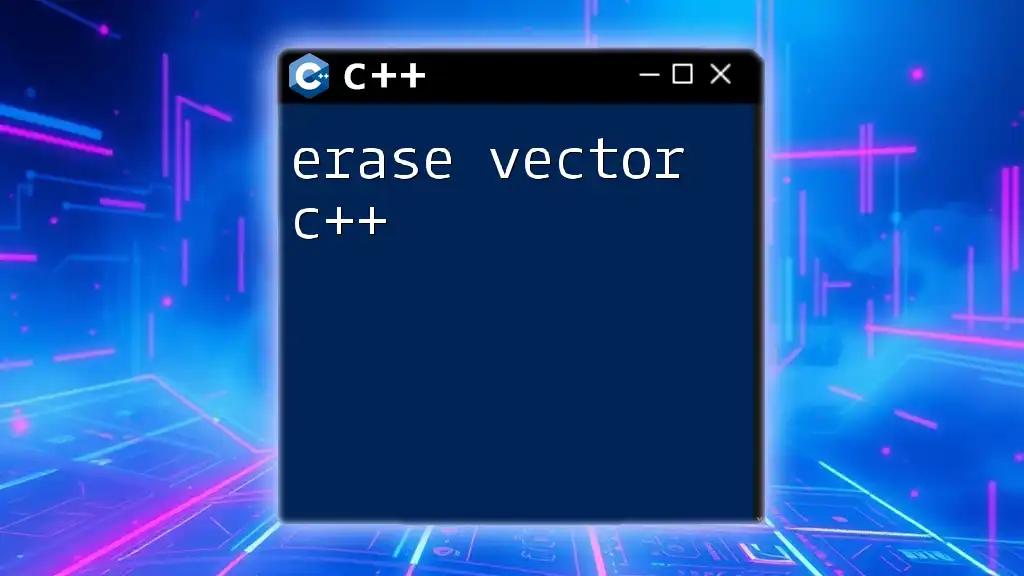
Multithreading Example: Volatile Variables with Thread Communication
Here’s an example of how volatile can be used in a multithreaded scenario:
volatile int shared_value = 0;
void producer() {
shared_value = 42; // produce a value
}
void consumer() {
while (shared_value == 0) {
// Busy wait until a new value is produced
}
// Now shared_value has a real value
}
The consumer checks the variable `shared_value`, which is declared volatile. This ensures that it sees the changes made by the producer thread regardless of any compiler optimizations that might occur.

Recap of the Volatile Keyword’s Importance
The volatile keyword in C++ is an essential tool for correctly dealing with variables that can change unexpectedly, particularly in hardware interfacing and multithreaded programming. By ensuring that variable accesses are not optimized away by the compiler, it helps maintain the integrity of the program's flow. However, it is crucial to use this keyword judiciously and understand its limitations in multithreaded contexts.
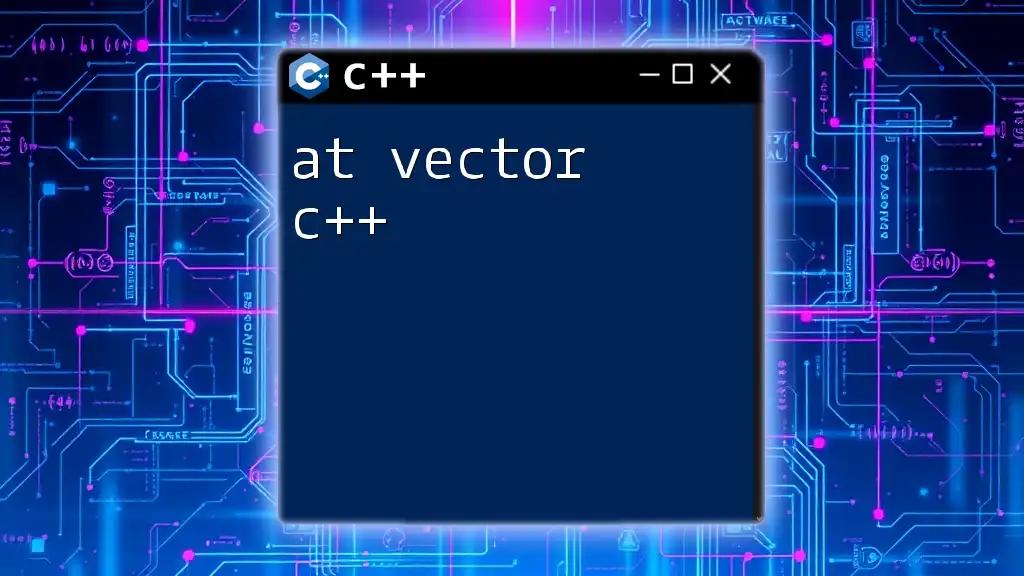
FAQs about the Volatile Keyword in C++
What happens if I do not use volatile?
If you do not use the volatile keyword for variables that are changed outside your program's control, the compiler might optimize out required reads or writes. This can lead to bugs that are difficult to trace.
Can you modify a volatile variable from an interrupt?
Yes, you can modify a volatile variable within an interrupt context. However, declaring it as volatile ensures that other parts of your code see the most current value.
Are volatile variables automatically thread-safe?
No, volatile variables are not automatically thread-safe. Volatile merely prevents optimization but does not provide guarantees against race conditions or synchronized access. Use proper synchronization mechanisms such as mutexes or atomic variables where necessary.