The `const` keyword in C++ is used to define variables as immutable, meaning their values cannot be changed after initialization.
const int MAX_LENGTH = 100;
// MAX_LENGTH = 200; // This will result in a compilation error
Understanding the Basics of `const`
What Does `const` Mean?
The `const` keyword in C++ is a powerful modifier that indicates that the value of a variable will not change after it has been initialized. In other words, declaring a variable with `const` enforces immutability. This allows developers to prevent accidental changes to data, which can lead to bugs and unpredictable behavior in programs.
By using `const`, we can:
- Improve code readability by making the intention of our variables clear.
- Enhance code safety by allowing the compiler to provide checks that catch unintended changes.
Applying the `const` Keyword
Declaring a constant variable is simple and clear. The syntax for using `const` is as follows:
const int maxUsers = 100; // maxUsers is a constant
In this example, `maxUsers` is set to 100 and cannot be altered later in the code. If you try to assign a new value to `maxUsers`, the compiler will throw an error, helping catch potential mistakes early.

The Scope and Lifetime of `const`
Local vs Global Constants
Constants in C++ can be defined at various scopes:
- Local Constants: These are declared within a function and are accessible only within that function's scope.
void exampleFunction() {
const int localConst = 20; // Accessible only within this function
}
- Global Constants: These are declared outside of any function and can be accessed by any function in the same file or file included in main.
const int globalConst = 50; // Accessible from anywhere in the same file
Understanding the scope of constants helps reduce variable shadowing and avoids confusion during variable usage in larger codebases.
Constant Expressions
Constant expressions are values that are known at compile time. When using `const`, they become essential when you want to initialize a variable, especially for indexing arrays or setting sizes.
const int size = 10;
int arr[size]; // size is a constant expression
The compiler knows `size` will never change, which can optimize performance.

Using `const` with Pointers
Pointers to Constants
Declaring pointers to constants allows you to retrieve constant values without modifying them. This is useful for passing large structures or arrays without the overhead of copying.
const int *ptr = &localConst; // Pointer to a constant integer
With this pointer, you can read the value of `localConst`, but you cannot modify it through `ptr`.
Constant Pointers
Conversely, constant pointers are pointers that cannot be redirected to point somewhere else after initialization, although the value pointed to can usually still be modified.
int var = 30;
int *const ptrConst = &var; // Constant pointer
Here, `ptrConst` must always point to `var`, but the value of `var` can still be changed.
Constant Pointers to Constants
Finally, a constant pointer to a constant means neither the pointer nor the value it points to can be changed.
const int *const ptrToConst = &localConst; // Constant pointer to a constant integer
This ensures maximum protection of data in cases where it is crucial to maintain value integrity.
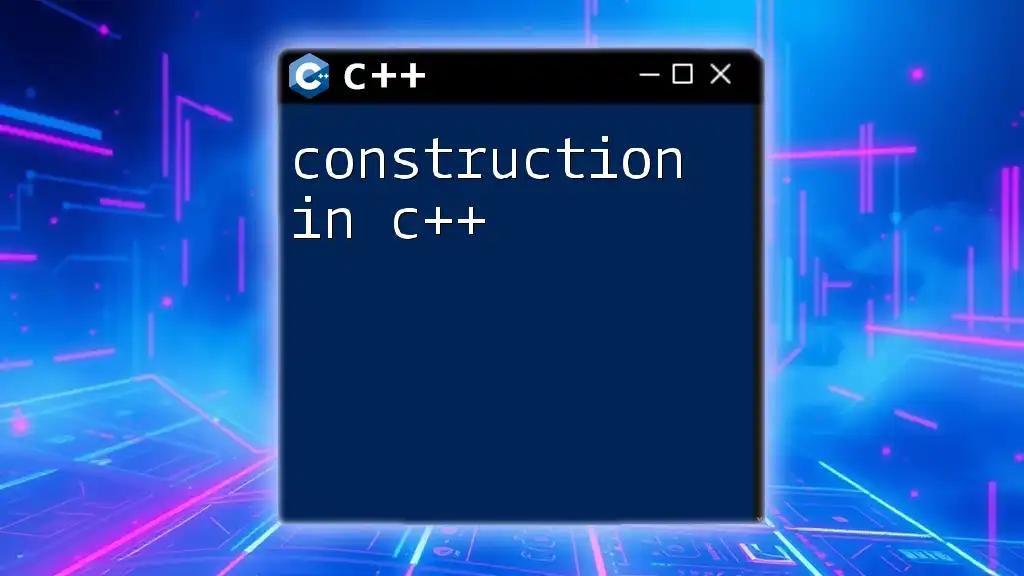
Using `const` with Member Functions
Const Member Functions
In C++, member functions can also be declared with the `const` keyword. When this is done, it indicates that the function will not modify any member variables of the class.
class MyClass {
public:
void display() const {
// This function cannot modify any member variables
}
};
Using `const` this way helps in maintaining class invariants and keeps your code clean, explicitly stating that certain methods are read-only.
Importance of Const in Class Design
When designing classes, leveraging `const` helps encapsulate and protect the internal state. It's particularly critical in methods that overload operators, as it can affect the behavior and usage of your classes significantly.

`const` and Function Parameters
Passing by Value vs Passing by Reference
When you have functions that take parameters, using `const` can greatly enhance safety and performance. For instance, passing large objects by const reference avoids unnecessary copies.
void process(const std::vector<int>& vec) {
// vec cannot be modified within this function
}
With this code snippet, you ensure that `process` cannot alter the original vector while still allowing for efficient access to its data.
Avoiding Unintended Side Effects
Using `const` with function parameters helps to avoid side effects. If you have a function that takes a parameter by reference, marking it as `const` ensures that the caller's data remains unchanged, which can prevent bugs that are hard to track down.

Best Practices for Using the `const` Keyword
When to Use `const`
In general, you should use `const` in the following situations:
- Whenever you define a variable that should not change.
- For function parameters that should not be modified.
- In class member functions that do not alter the class state.
Common Pitfalls to Avoid
Be cautious when employing `const`. Certain subtleties can lead to confusion:
- Const-Correctness: Ensure that both the function declaration and definition match in constness.
- Pointer Confusion: Remember the difference between pointers to constants and constant pointers to maintain clarity in your code.
Understanding these nuances not only improves your own coding practices but also aids those who will read or maintain your code in the future.
Code Examples of Effective `const` Usage
Here’s an example showcasing effective use of `const` that balances clarity and efficiency:
class Circle {
private:
const double radius;
public:
Circle(double r) : radius(r) {}
double area() const { // This function will not change any members
return 3.14159 * radius * radius;
}
};
void calculateArea(const Circle& circle) {
cout << "Area: " << circle.area() << endl; // Safe from modification
}
In this example, both the `radius` variable and the `area()` function ensure data integrity, and `calculateArea` efficiently uses const reference to avoid copies.

Conclusion
In summary, the `const keyword in C++` is an essential tool for developers looking to write robust, clear, and maintainable code. By enforcing immutability where necessary, you not only safeguard your code from unintended modifications but also enhance its overall quality. Emphasizing `const` in your programming practices can lead to better-designed applications and fewer hard-to-trace bugs. As you delve deeper into C++ programming, continued exploration of const will undoubtedly yield substantial benefits in your coding journey.