The `mutable` keyword in C++ allows members of a class to be modified even if they are part of an object declared as `const`, enabling the flexibility to change the state of specific member variables while keeping the rest of the object's interface immutable.
#include <iostream>
class MyClass {
public:
mutable int mutableValue;
MyClass() : mutableValue(0) {}
void changeValue() const {
mutableValue++; // This is allowed because mutableValue is mutable
}
};
int main() {
const MyClass obj;
obj.changeValue();
std::cout << "Mutable Value: " << obj.mutableValue << std::endl; // Output: 1
return 0;
}
What is the Mutable Keyword in C++?
The mutable keyword in C++ allows you to modify class member variables within const member functions. This keyword is a powerful addition to the C++ language, enabling flexibility in managing the state of objects, particularly when you want certain members to be mutable while keeping others constant.
The primary purpose of the mutable keyword is to indicate that a specific member can be modified regardless of whether the containing object is constant. This contrasts with the const keyword, which enforces read-only access to members of a const object.

When to Use the Mutable Keyword
Using the mutable keyword is beneficial in several scenarios:
-
Modifying Class Members in Const Member Functions: When you want a specific member variable to maintain state even in methods declared as const, the mutable keyword is invaluable. This is particularly useful for counters or caches.
-
Use Cases in Multi-threading: In concurrent programming, a mutable member can help manage shared data where the object's state may alter in a synchronized manner, allowing for efficient resource management.
The benefits of using the mutable keyword include:
-
Increased Flexibility: You can design your classes to encapsulate behavior while still allowing controlled mutation of specific members.
-
Better Control over Member States: It allows you to declare members that can change under certain conditions without affecting the constness of the enclosing instance.

How to Use the Mutable Keyword
The syntax of the mutable keyword is straightforward. You declare a member variable as mutable within a class. Here’s an illustrative example:
class Example {
mutable int counter;
public:
Example() : counter(0) {}
void increment() const {
++counter; // allowed because counter is mutable
}
int getCounter() const { return counter; }
};
Detailed Breakdown of Example Code
Class Declaration: In the example, we define a class named `Example` that contains a mutable integer member named `counter`.
Mutable Int Member: The declaration of `counter` with the mutable keyword indicates that the member can be modified even when accessed through const methods.
Const Member Function: The function `increment()` is marked as `const`, yet it is designed to increment the `counter`. This is permissible because `counter` is declared as mutable, showcasing how mutable members can be modified even in a const context.
Behavior of the Code: When an instance of `Example` is created and `increment()` is called, the `counter` variable can be updated despite the surrounding const-ness of the function, demonstrating the flexibility that the mutable keyword provides.

Memory Management with Mutable
Using the mutable keyword can have implications on memory usage. Mutable members allow for precise control over which data can be modified without altering the overall state of the object. This can lead to efficient memory management, especially in scenarios where objects are frequently passing through const interfaces.
When a mutable member is involved, memory can be optimized by maintaining essential data without complicating the object's const-correctness. It facilitates easier management of member states in contexts where you need to maintain an internal mechanism (like a counter) without exposing it to external modification.
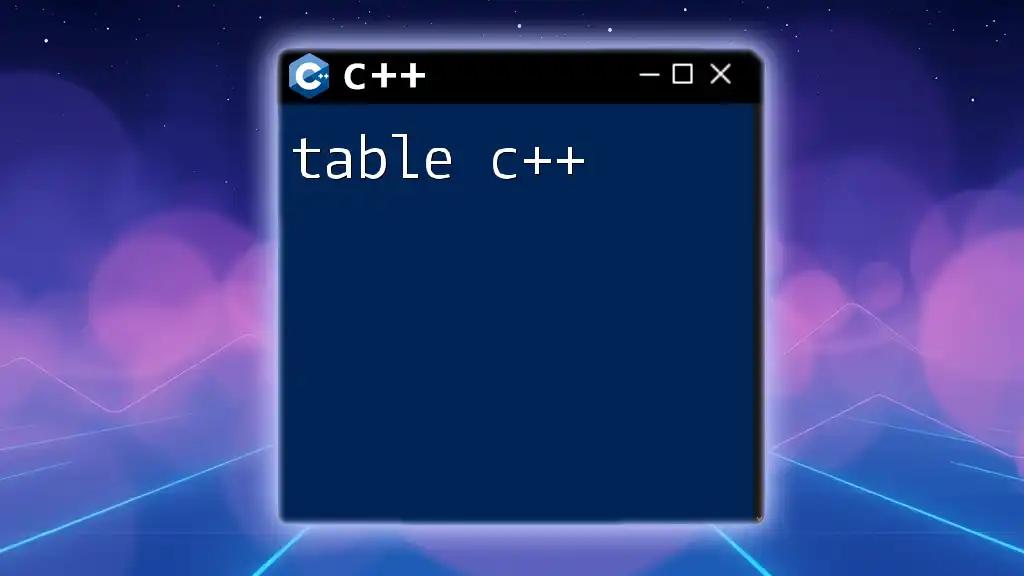
Best Practices for Using Mutable in C++
When using the mutable keyword in C++, follow these best practices to ensure its effective application:
-
Clear Intent: Always document the purpose of a mutable member within your classes. This clarity helps maintain the code and avoids confusion for future developers.
-
Keep It Minimal: Limit the use of mutable members to cases where absolutely necessary. Overuse can lead to complex code and obscure the intended const-correctness of other class members.
-
Thread Safety Considerations: If mutable members are shared across threads, ensure appropriate locking mechanisms are in place to prevent data races.
-
Alternatives: Consider using other design patterns or data structures that do not impose const constraints, especially in multi-threaded environments, where clarity may be more beneficial than mutability.
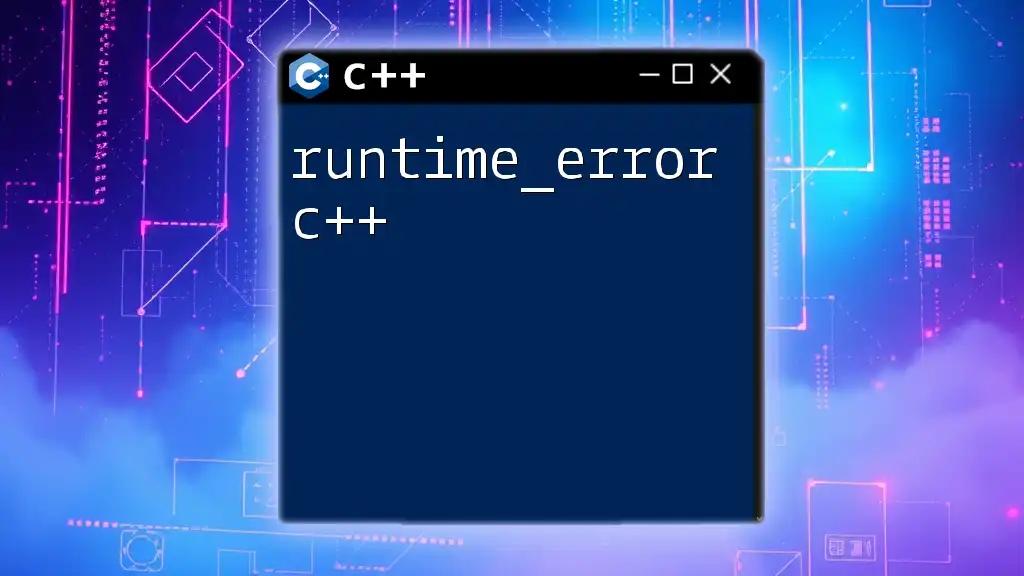
Real-World Applications of Mutable
The mutable keyword finds its place in several practical applications, such as:
-
Caching Mechanisms: In classes that represent complex calculations, mutable members can be used for caching results. This minimizes the need for recalculating values, thereby enhancing performance.
-
Lazy Initialization: By utilizing mutable, you can delay object initialization until it's first accessed, improving the overhead during object creation.
Case studies from established C++ projects often showcase the power of mutable in cases where performance is critical, such as in graphics rendering engines and system-level libraries.

Mutable vs Constexpr
Understanding the difference between mutable and constexpr is essential. The constexpr keyword declares that a variable’s value can be computed at compile time. Unlike mutable, which emphasizes runtime behavior, constexpr focuses on immutable values that should never change.
When making choices between mutable and constexpr, consider:
-
Use mutable when you need to modify a member variable in const contexts without affecting other member variables.
-
Use constexpr when you want a member to be evaluated and set at compile time, enhancing performance and predictability.

Frequently Asked Questions (FAQs)
Can a mutable member be static?
Yes, a mutable member can be declared static, but it will not be associated with any instance of the class. Instead, it will have a single shared state across all instances of the class.
What happens if you try to modify a non-mutable member in a const function?
C++ will generate a compilation error. Attempting to modify any non-mutable member within a const function violates const-correctness.
Are there any performance implications of using mutable?
Using mutable may introduce complexity, especially if accessed in multi-threaded scenarios. Therefore, while mutable itself may not degrade performance, the way it's managed in your program can affect overall performance and maintainability.
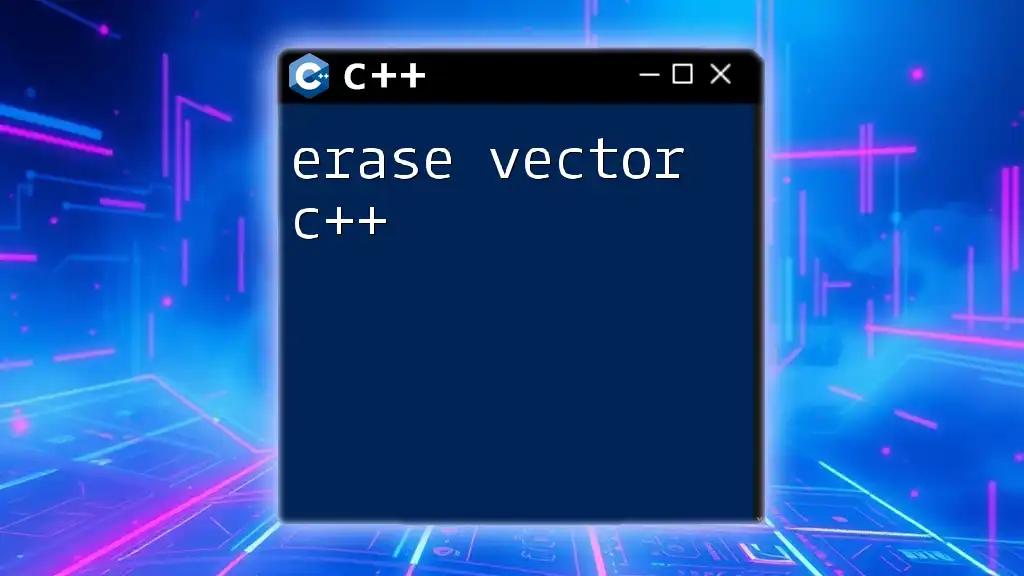
Conclusion
The mutable keyword in C++ is a powerful tool for managing state while maintaining const-correctness. Understanding its application and implications can greatly enhance the design and efficiency of your code. Use it wisely to provide flexibility in specific contexts while ensuring clarity and maintainability in your development practices. Embrace the mutable keyword and enhance your C++ programming skills!
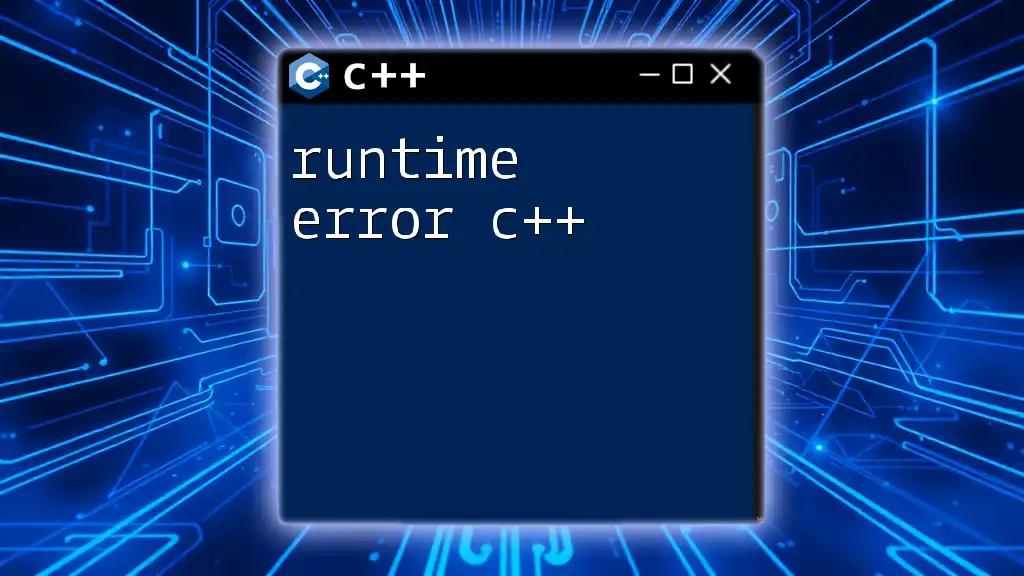
Additional Resources
Ensure to dive deeper into the official C++ documentation regarding the mutable keyword, and explore various C++ tutorials that will broaden your understanding of this and other keywords, enriching your overall development journey.