In C++, the `erase` function is used to remove elements from a vector at a specified position or within a specified range, effectively reducing its size.
Here's a code snippet demonstrating how to use `erase`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin() + 2); // Removes the element at index 2 (value 3)
for (int num : vec) {
std::cout << num << " "; // Output: 1 2 4 5
}
return 0;
}
Understanding C++ Vectors
What are Vectors?
Vectors in C++ are dynamic arrays that can resize themselves automatically when elements are added or removed. Unlike traditional arrays, vectors manage their own memory, making them a more flexible option for storing collections of data. This adaptability comes with the added bonus of built-in methods that simplify many tasks, such as inserting and erasing elements.
Basic Operations with Vectors
Before diving into the `erase` function specifically, it's essential to familiarize yourself with some basic operations related to vectors.
- Creating Vectors: Vectors can be created using the `std::vector` class from the `<vector>` header.
#include <vector>
std::vector<int> vec; // Creates an empty vector of integers
- Adding Elements: You can use the `push_back` method to add elements to the end of a vector.
vec.push_back(1); // Adds 1 to the vector
- Accessing Elements: Elements in a vector can be accessed directly using their index, similar to arrays.
int firstElement = vec[0]; // Accesses the first element
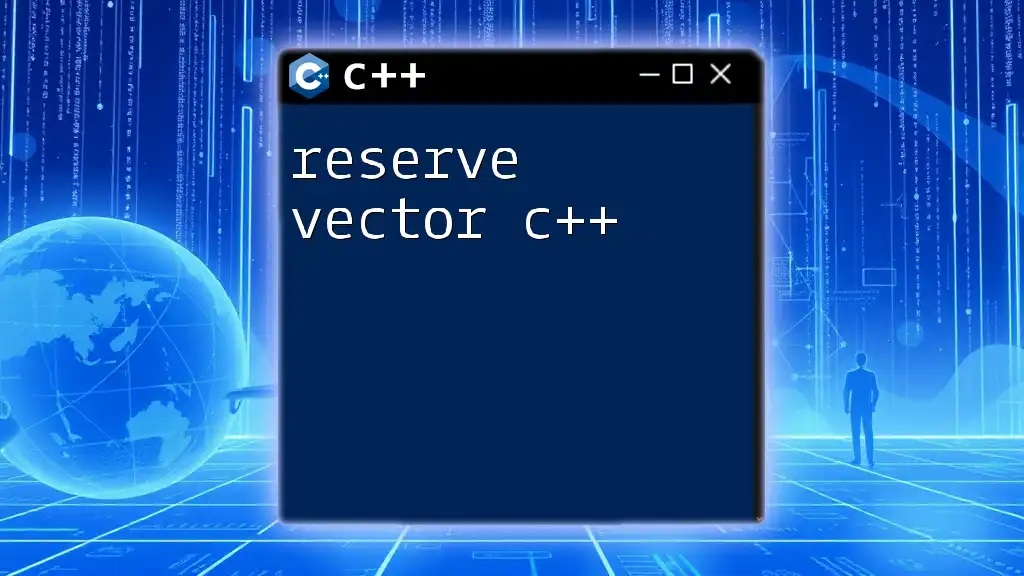
The `erase` Function in C++
Definition of the `erase` Function
The `erase` function in C++ is a member function of the `std::vector` class that allows you to remove one or more elements from a vector. The syntax for the `erase` function is as follows:
iterator erase(iterator position);
iterator erase(iterator first, iterator last);
When to Use the `erase` Function
Understanding when to use the `erase` function is crucial for effective vector management. You should consider using `erase` when:
- You need to remove specific elements based on their position or value.
- You want to maintain a clean and organized vector without unnecessary elements.
- You aim to optimize memory usage by releasing allocated space for unused elements.
Keep in mind that while `erase` is a powerful function, it can impact performance since it may involve shifting other elements in the memory.
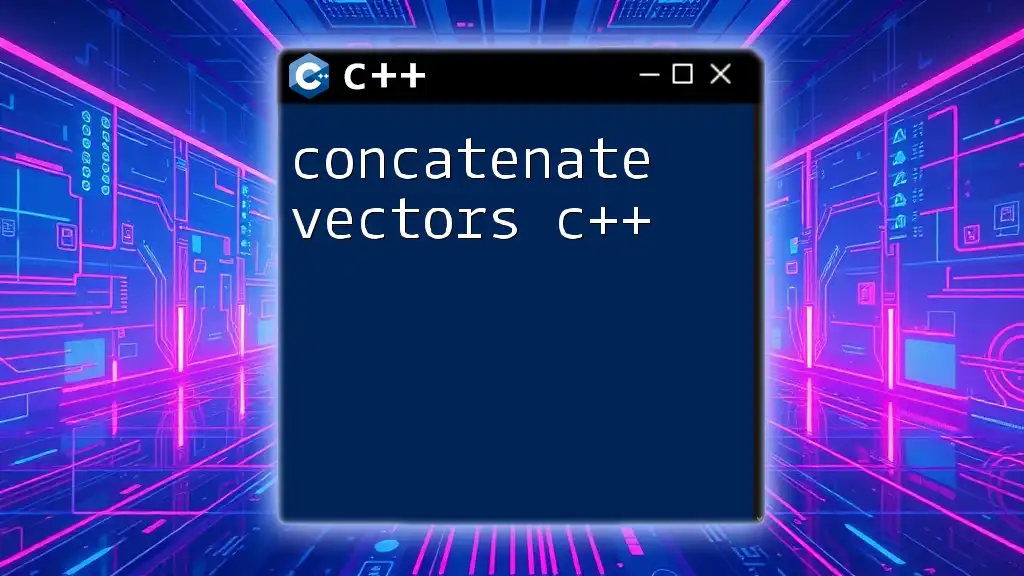
Syntax and Parameters
Function Overloads
Erasing a Single Element
To remove an individual element, you can call `erase` with an iterator pointing to the element you want to remove. Here’s an illustrative example:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
vec.erase(vec.begin() + 2); // Removes the third element (3)
for (const auto& value : vec) std::cout << value << " ";
return 0;
}
In this example, the third element (3) is removed. The elements following it shift left to fill the gap created by the removal.
Erasing Multiple Elements
You can also remove multiple elements at once by passing a range defined by two iterators. Here’s how it works:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5, 6, 7};
vec.erase(vec.begin() + 2, vec.begin() + 5); // Removes elements 3, 4, and 5
for (const auto& value : vec) std::cout << value << " ";
return 0;
}
This code snippet removes a range of elements from the vector. After execution, the output will display the remaining elements.
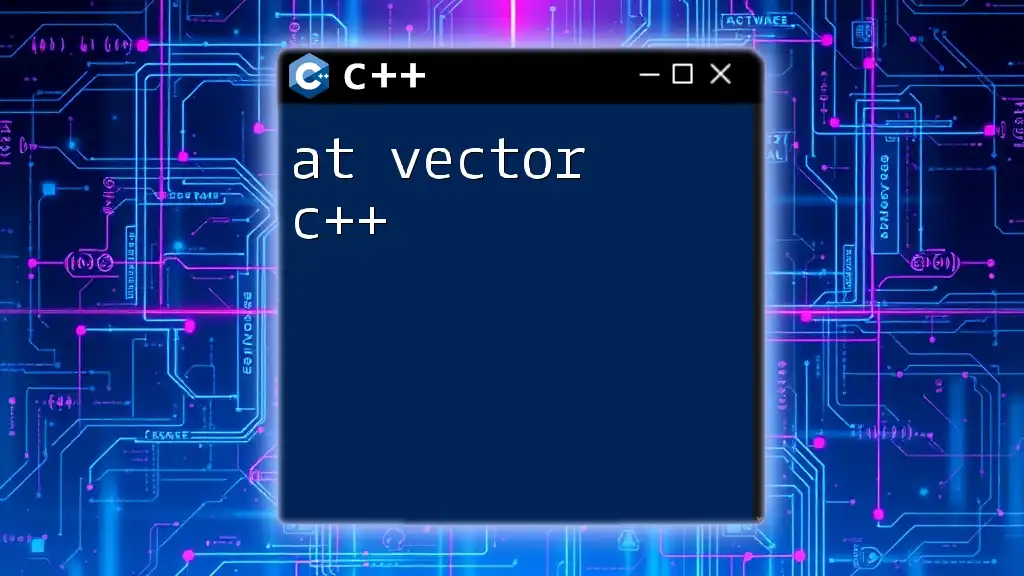
Understanding Iterators
What Are Iterators?
In C++, iterators act as a generalized pointer for traversing through containers, such as vectors. They allow you to iterate over the elements without exposing the underlying data structure.
Using Iterators with `erase`
The use of iterators with the `erase` function is essential for removing items based on their position. Since vectors use iterators, you can erase elements easily as follows:
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
auto it = vec.begin() + 1; // Points to the second element (2)
vec.erase(it); // Removes the second element (2)
for (const auto& value : vec) std::cout << value << " ";
return 0;
}
In this case, an iterator points to the second element in the vector, which is removed using `erase`.
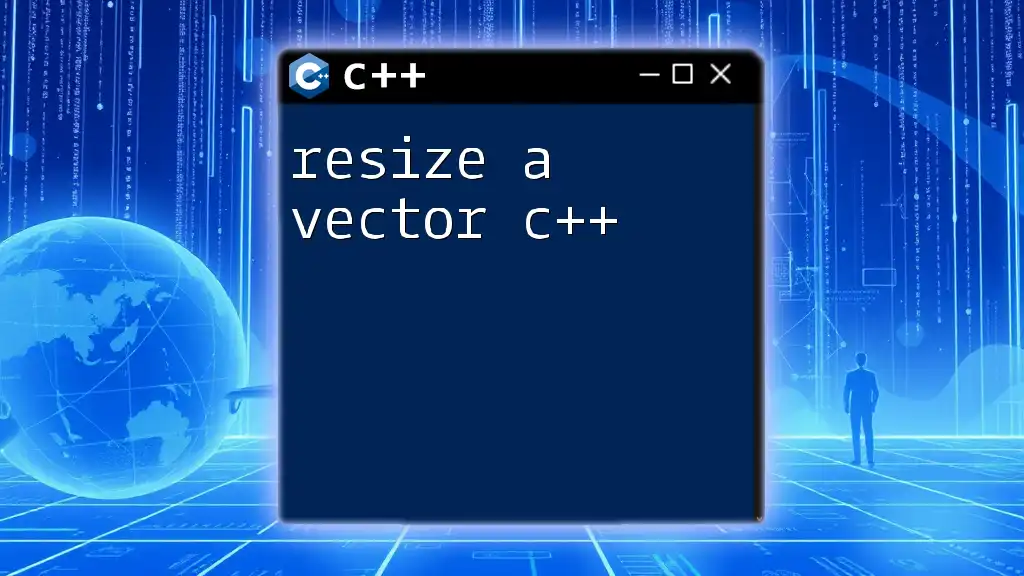
Performance Implications
Complexity of the `erase` Function
The performance of the `erase` function should be carefully considered. Removing a single element from a vector has a time complexity of O(n), where n is the number of elements in the vector. This is due to the need for subsequent elements to be shifted left.
When erasing a range of elements, the performance implication might worsen since all elements that follow the erased range must also be shifted.
Best Practices for Efficient Erase Operations
To minimize performance degradation, consider the following suggestions:
- When removing multiple elements frequently, assess if using a different data structure, like `std::list` or `std::deque`, may be more efficient.
- Whenever possible, bulk remove elements by keeping track of the indices to minimize iteration time.
- Avoid calling `erase` within a loop if it's possible to collect all indices to be removed first and execute the removal in a single operation.
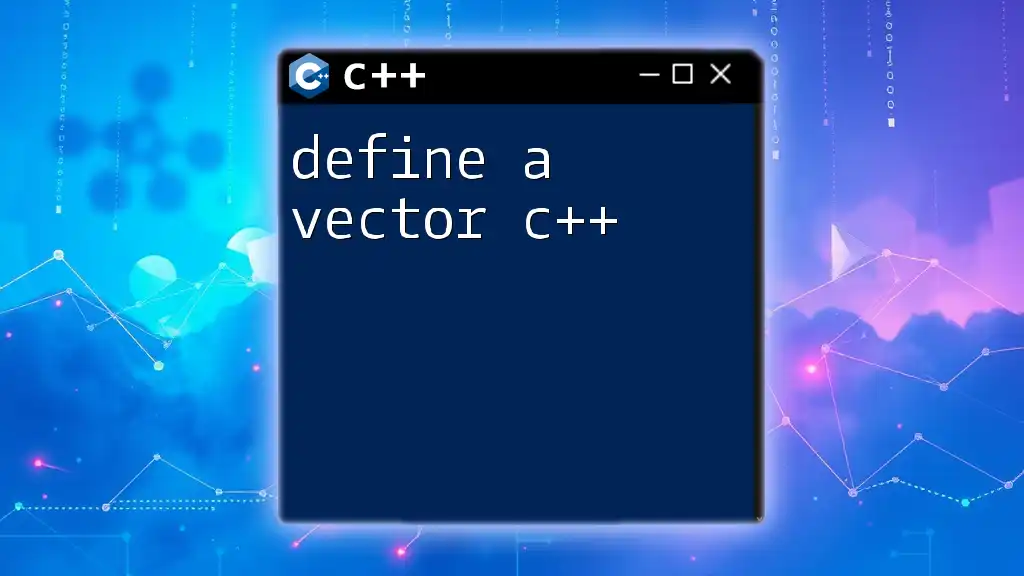
Common Pitfalls and Troubleshooting
Issues with Invalidated Iterators
One of the critical aspects of using the `erase` function is that it invalidates all iterators pointing to or beyond the erased element. This invalidation can lead to undefined behavior if not handled properly. To manage this, it's crucial to adjust any iterators or consider re-fetching them post-erase operation.
Debugging Erase Operations
Errors commonly encountered when using `erase` include:
- Accessing an invalidated iterator causes runtime errors.
- Attempting to erase from an empty vector can lead to segmentation faults.
To troubleshoot, ensure your logic accounts for iterator extents, and make use of debugging tools or simple print statements to trace your program's flow.
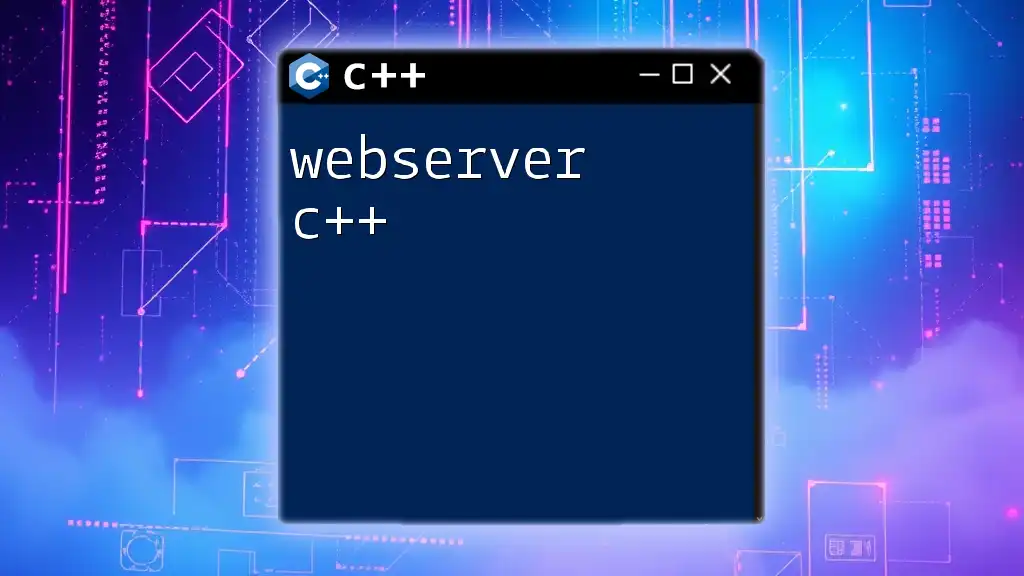
Conclusion
The `erase` function is a fundamental tool for managing C++ vectors. Understanding its syntax, performance implications, and common pitfalls allows for more effective and efficient coding practices. By mastering the use of the `erase` function, you can optimize your vector operations and enhance the overall performance of your C++ applications.
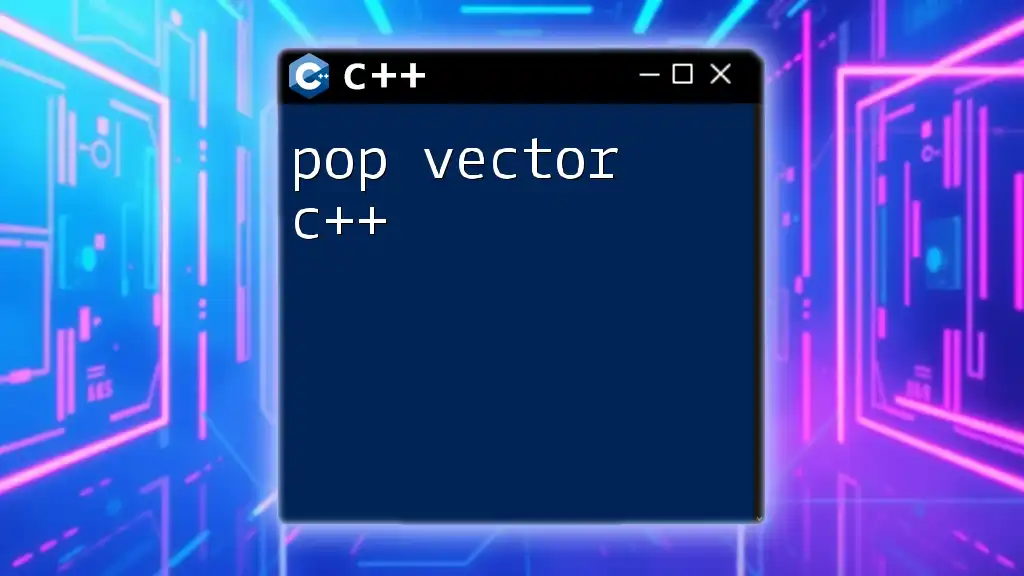
Additional Resources
For those looking to dive deeper into C++ vectors and related topics, consider checking out the official C++ documentation on vectors, as well as video tutorials that explore advanced memory management strategies.
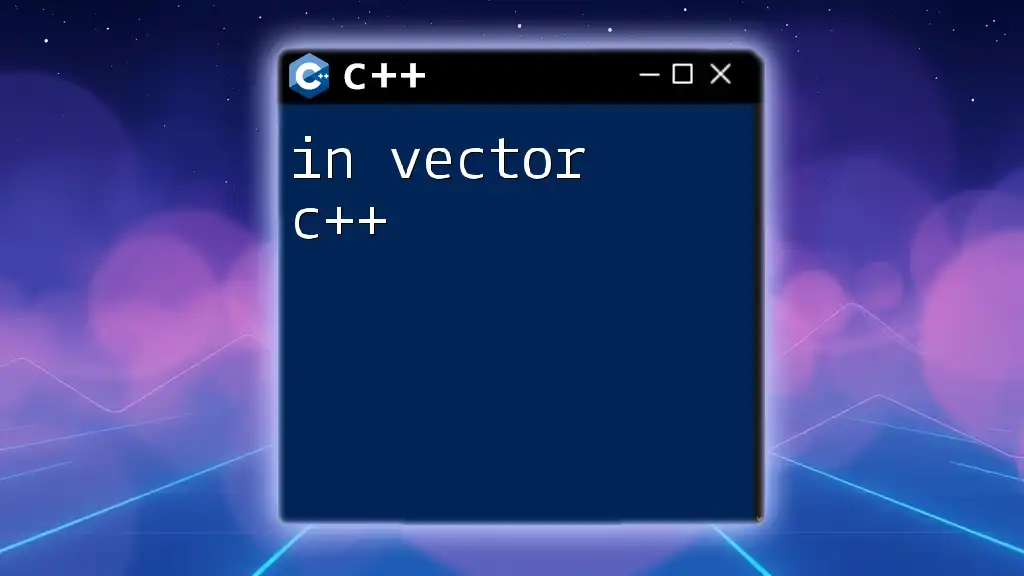
Call to Action
We invite you to share your experiences with the `erase` function or raise any questions you might have about vector manipulation in C++. Your insights could help foster a learning community, so don’t hesitate to engage with us!