In C++, vectors are dynamic arrays that can resize themselves automatically when elements are added or removed, providing a convenient way to store and manage collections of data.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
numbers.push_back(6); // Adding an element
for (int num : numbers) {
std::cout << num << " "; // Output: 1 2 3 4 5 6
}
return 0;
}
Understanding Vectors
What is a Vector?
In C++, a vector is a sequence container that encapsulates dynamic size arrays. Unlike traditional arrays, which have a fixed size defined at compile time, vectors can grow or shrink in size. This flexibility makes them an essential component of the C++ Standard Library.
When you think of vectors, envision them as high-level containers that manage memory for you while providing all the functionality of arrays. They allow you to store a sequence of elements, and most importantly, you can perform various operations without worrying about the underlying memory management.
Benefits of Using Vectors
Using vectors comes with several clear advantages:
-
Automatic Memory Management: Vectors manage their memory automatically. Unlike dynamic arrays, which require manual allocation and deallocation, vectors handle this for you.
-
Support for a Variety of Data Types: Vectors can store data of any type, including primitive types and user-defined objects.
-
Built-in Functions and Features: Vectors come with a wealth of built-in functions, such as adding, removing, resizing, and iterating over elements, making them very easy to use and powerful.
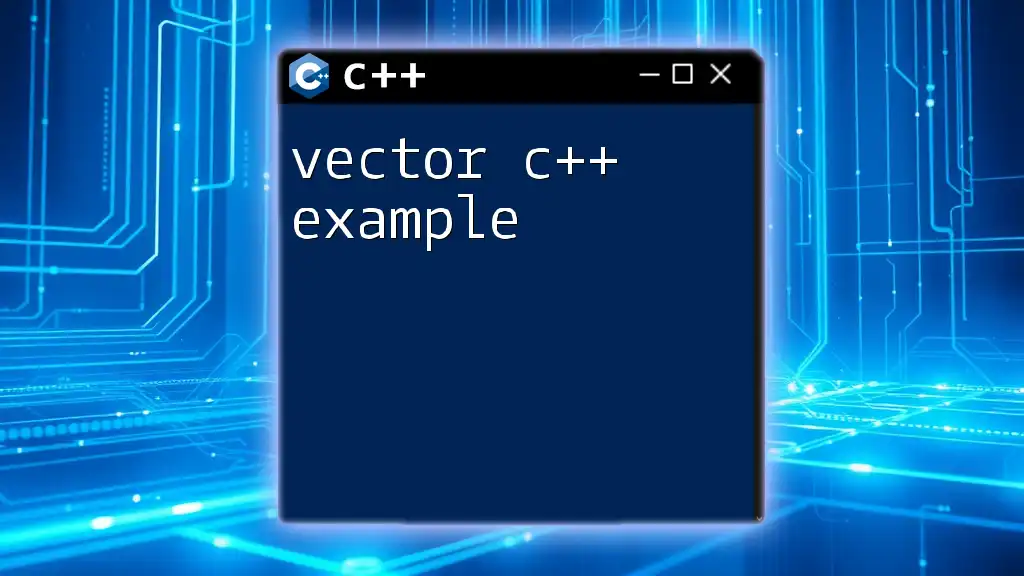
Getting Started with Vectors
Including Necessary Headers
To utilize vectors in your C++ code, you must include the vector header:
#include <vector>
This inclusion provides you access to all vector functionalities, allowing you to define and manipulate vectors seamlessly.
Declaring and Initializing Vectors
Declaring and initializing vectors in C++ is intuitive. You can declare a vector using the following syntax:
std::vector<type> vectorName;
Different ways to initialize vectors:
-
Default Initialization: This creates a vector without any elements.
std::vector<int> numbers; // default initialization
-
Initialization with Size: You can create a vector of a specified size that initializes with default values for that data type.
std::vector<int> numbersWithSize(10); // creates a vector of size 10
-
Initializer List: You can initialize a vector with specific values directly.
std::vector<int> initializedNumbers = {1, 2, 3, 4, 5}; // creates a vector with these values
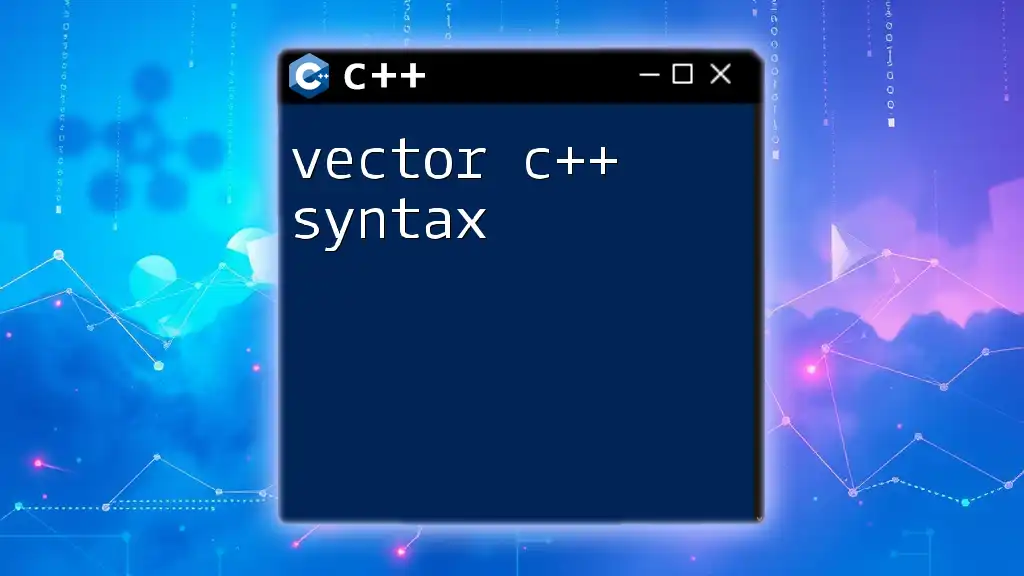
Basic Operations with Vectors
Accessing Elements
Vectors allow you to access their elements using the `.at()` function or the subscript operator `[]`.
-
Using `.at(index)`: This method provides safe access, meaning it checks the index and throws an exception if it's out of bounds.
std::cout << initializedNumbers.at(0); // outputs: 1
-
Using `[]` operator: This provides direct access to the element but does not perform bounds checking.
std::cout << initializedNumbers[0]; // outputs: 1
Modifying Elements
To change the value of a specific element, simply access it and assign a new value:
initializedNumbers[0] = 10; // changes the first element to 10
Adding and Removing Elements
Vectors allow easy modification of their contents:
-
Adding Elements: Use `push_back()` to append elements to the end of the vector.
initializedNumbers.push_back(6); // added 6 to the end
-
Removing Elements: `pop_back()` removes the last element of the vector.
initializedNumbers.pop_back(); // removes the last element
Getting Size and Capacity
Understanding the difference between size and capacity is crucial when working with vectors.
- Size: The number of elements currently stored in the vector. Use `.size()` to get this value.
std::cout << "Size: " << initializedNumbers.size(); // outputs: Size: 5
- Capacity: The amount of memory allocated for the vector, which may be greater than or equal to the size. This can be accessed using `.capacity()`.
std::cout << "Capacity: " << initializedNumbers.capacity(); // outputs the allocated capacity
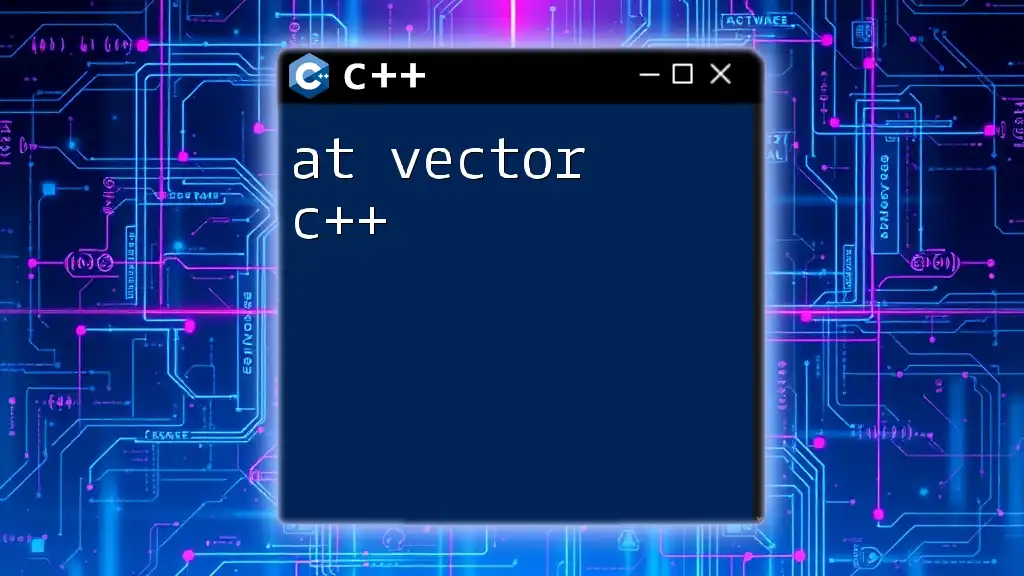
Advanced Vector Operations
Inserting and Erasing Elements
Vectors allow insertion and removal of elements at specific indices:
-
Inserting Elements: Use `insert()` to add an element at a specified position.
initializedNumbers.insert(initializedNumbers.begin() + 1, 10); // inserts 10 at index 1
-
Removing Elements: Use `erase()` to remove an element at a specified index.
initializedNumbers.erase(initializedNumbers.begin() + 1); // erases element at index 1
Sorting Vectors
Sorting is a common operation that enables organized data management. The C++ Standard Library provides the `std::sort()` function, which utilizes the `<algorithm>` header.
#include <algorithm>
// Sort the vector in ascending order
std::sort(initializedNumbers.begin(), initializedNumbers.end());
Iterating through Vectors
Iterating over the elements in a vector is straightforward. You can use different methods:
- Simple For Loop:
for (size_t i = 0; i < initializedNumbers.size(); i++) {
std::cout << initializedNumbers[i] << " ";
}
- Range-Based For Loop: This method is more modern and concise.
for (const auto& num : initializedNumbers) {
std::cout << num << " ";
}
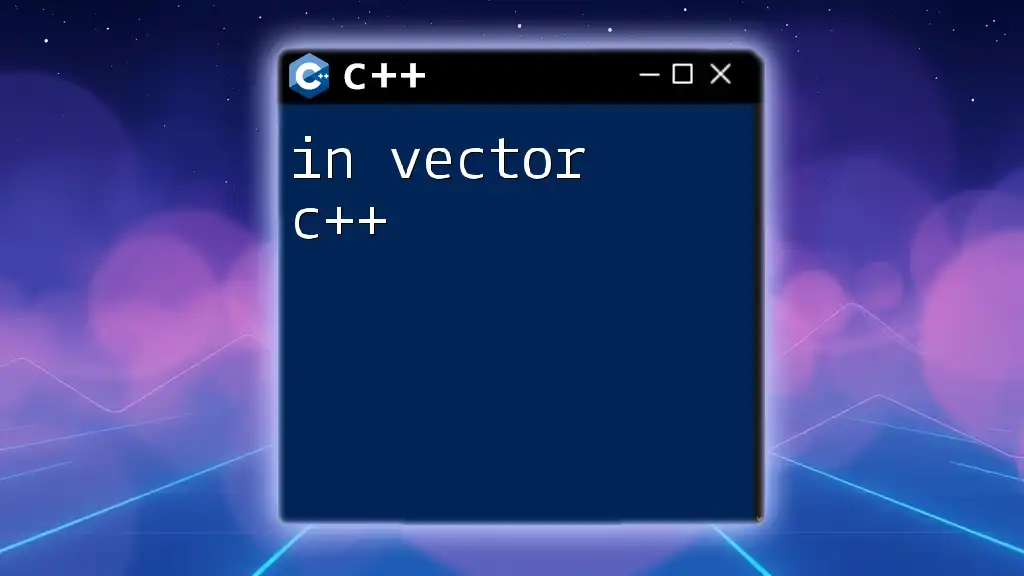
Common Use Cases for Vectors
Storing Collections of Data
Vectors are ideal for storing collections of related data. For example, you can use a vector to keep track of student grades:
std::vector<double> studentGrades = {88.5, 92.0, 79.5};
Dynamic Data Handling
Vectors excel in scenarios where you do not know the number of items in advance. For example, you can use a vector to store user-entered numbers until a certain condition is met. This might look like:
int num;
std::vector<int> userInputs;
while (std::cin >> num && num != -1) {
userInputs.push_back(num); // Store numbers until -1 is entered
}
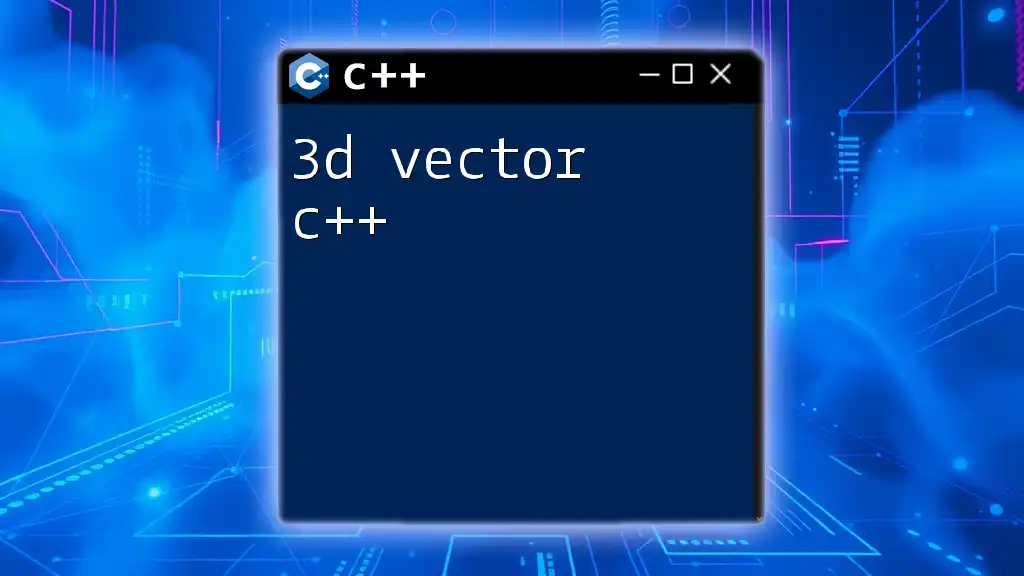
Conclusion
Vectors in C++ offer a dynamic, efficient, and user-friendly alternative to traditional arrays. Their automatic memory management, support for diverse data types, and rich set of functions make them invaluable for modern C++ programming. By understanding the fundamentals of vectors, from declaration through advanced operations, you can harness their power to write more flexible and efficient code.
As you continue your journey with C++, I encourage you to practice using vectors. Explore more advanced topics related to the C++ Standard Library and consider deepening your knowledge through courses and resources available online. Happy coding!