In C++, a vector is a dynamic array that can grow in size and provides various member functions for managing the elements, and it can be declared and initialized as follows:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5}; // Declare and initialize a vector
for(int n : numbers) std::cout << n << " "; // Output the elements
return 0;
}
What are Vectors in C++?
Vectors in C++ are a part of the C++ Standard Template Library (STL) and represent a dynamic array that can change size and type. They are similar to arrays but offer greater flexibility since they can automatically manage memory. The primary advantages of using vectors include:
- Dynamic resizing: Unlike arrays, which have a fixed size, vectors can adjust their size automatically as elements are added or removed.
- Ease of use: STL vectors come with built-in functions that simplify common operations such as insertion, deletion, and traversal.
Overview of C++ Standard Template Library (STL)
The STL is a powerful library that provides a collection of generic classes and functions. Vectors are one of the most frequently used components of the STL due to their versatility. The benefits of utilizing STL containers include:
- Improved code manageability.
- Ready-to-use algorithms and operations to avoid reinventing the wheel.
- Enhanced performance due to optimized implementations.
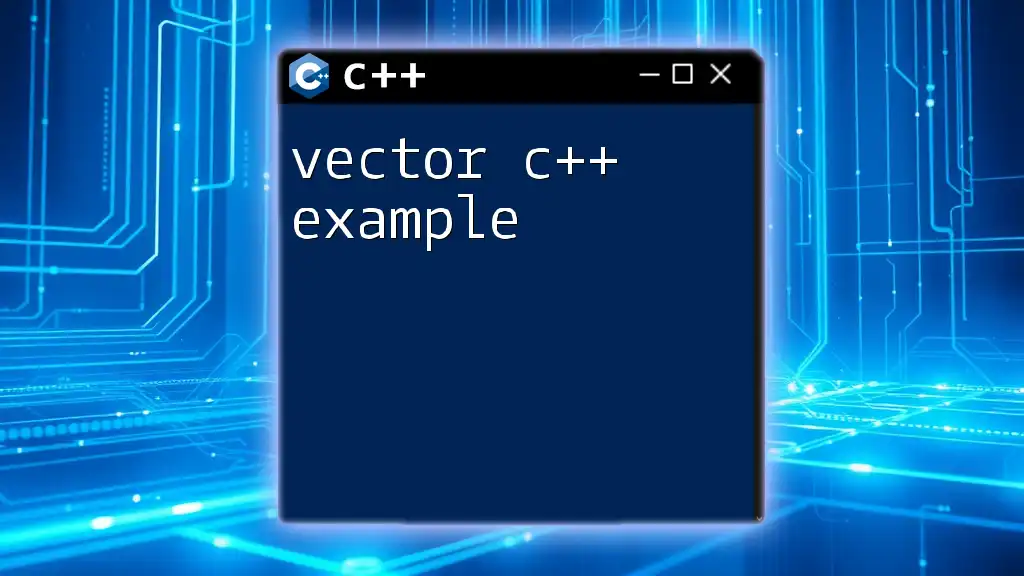
Understanding Vector Syntax in C++
Basic Vector Syntax
To begin working with vectors, you need to include the appropriate header file:
#include <vector>
You can then declare a vector using the following syntax:
std::vector<data_type> vector_name;
To initialize a vector, you can provide initial values as below:
std::vector<int> myVector = {1, 2, 3, 4, 5};
Common Vector Operations
Adding Elements
Using the `push_back()` method allows you to add elements to the end of the vector. Here’s how it works:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector;
myVector.push_back(10);
myVector.push_back(20);
std::cout << "Element at index 0: " << myVector[0] << std::endl; // Output: 10
}
The `push_back()` function dynamically adjusts the size of the vector to accommodate new elements.
Accessing Elements
You can access vector elements using the indexing operator `[]` or the `at()` method. Here’s an example:
std::cout << myVector[0] << std::endl; // Using indexing
std::cout << myVector.at(0) << std::endl; // Using at()
Important Note: While using `at()` adds a layer of safety by checking bounds, the indexing operator does not perform such checks, which can lead to undefined behavior if used incorrectly.
Removing Elements
To remove the last element of a vector, the `pop_back()` method is utilized. Here's how it looks:
myVector.pop_back(); // Removes the last element
After calling `pop_back()`, the vector's size decreases by one.
Inserting Elements
You can insert elements at any position in a vector using the `insert()` function. This is illustrated below:
myVector.insert(myVector.begin() + 1, 15); // Inserts 15 at index 1
Other Useful Methods
Vectors in C++ offer several essential methods:
-
`size()`: Returns the number of elements in the vector.
std::cout << "Size: " << myVector.size() << std::endl;
-
`capacity()`: Returns the total number of elements that the vector can hold before resizing.
std::cout << "Capacity: " << myVector.capacity() << std::endl;
-
`clear()`: Removes all elements from the vector.
myVector.clear();
Each of these methods helps in managing memory and understanding the state of your vector effectively.
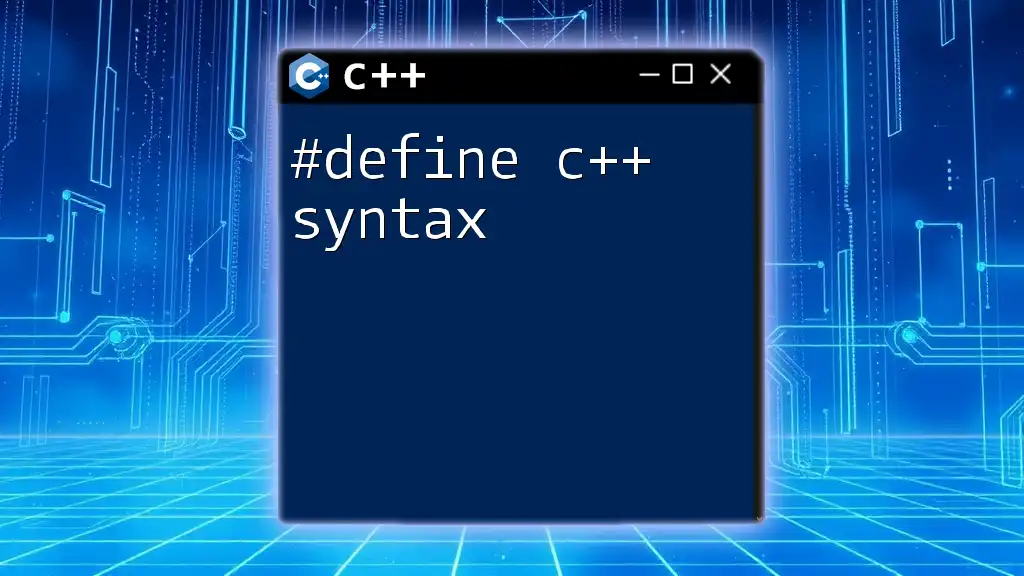
Using A Vector in C++
Vector Examples in C++
Sample Program: Basic Vector Operations
Let’s construct a simple program to demonstrate some of the basic operations with vectors:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
myVector.push_back(6);
std::cout << "Size after push_back: " << myVector.size() << std::endl;
for (int i = 0; i < myVector.size(); i++) {
std::cout << myVector[i] << " "; // Output: 1 2 3 4 5 6
}
}
In this example, we initialize a vector, add an element, and print its size along with all the elements.
C++ STL Vector Example: Iterating through a Vector
There are multiple ways to iterate through a vector:
Using a traditional for loop:
for(int i = 0; i < myVector.size(); i++) {
std::cout << myVector[i] << " ";
}
Using a range-based for loop:
for (auto &val : myVector) {
std::cout << val << " ";
}
Both methods will yield the same output, but the range-based loop offers cleaner syntax.
Advanced Vector Syntax and Features
Nested Vectors
Vectors can also hold other vectors, allowing for the creation of multi-dimensional arrays, such as matrices. Here’s how to declare a nested vector:
std::vector<std::vector<int>> matrix = {{1, 2}, {3, 4}};
Each inner vector can be treated like a single vector, enabling complex data structures.
Pass By Reference vs. Value
When passing vectors to functions, it's crucial to understand the difference between passing by reference and by value. Passing by value creates a copy:
void modifyVector(std::vector<int> vec) {
vec.push_back(10);
}
In contrast, passing by reference doesn’t create a copy, making your function more efficient:
void modifyVector(std::vector<int>& vec) {
vec.push_back(10);
}
This distinction impacts performance and memory usage.
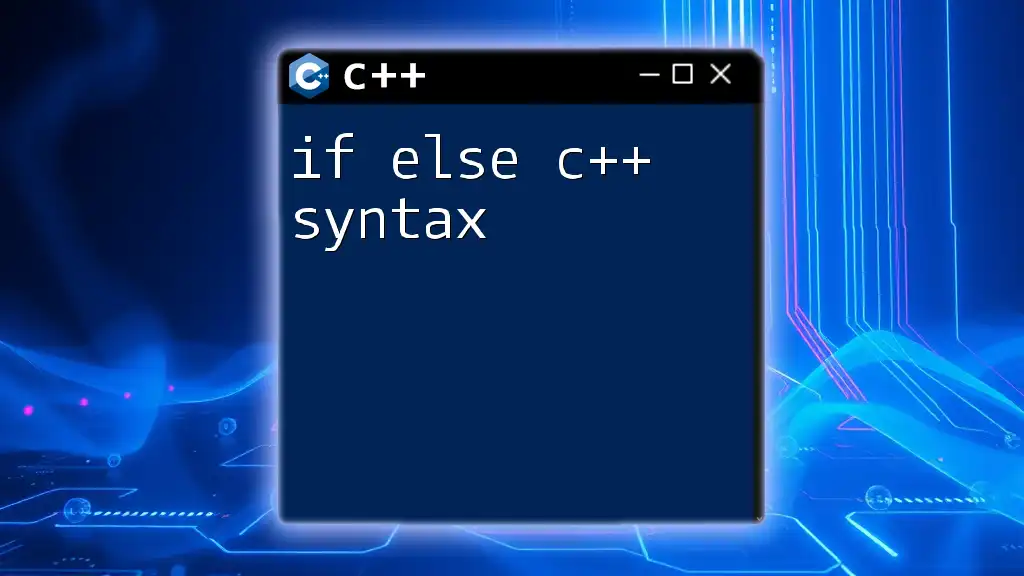
Best Practices for Using C++ Vectors
Performance Considerations
When considering performance, it’s often better to choose vectors over other data structures for dynamic data because they provide faster access and better memory locality.
Common Pitfalls
Avoid accessing vector elements out of bounds, as doing so results in undefined behavior. Always verify the vector’s size before accessing its elements.
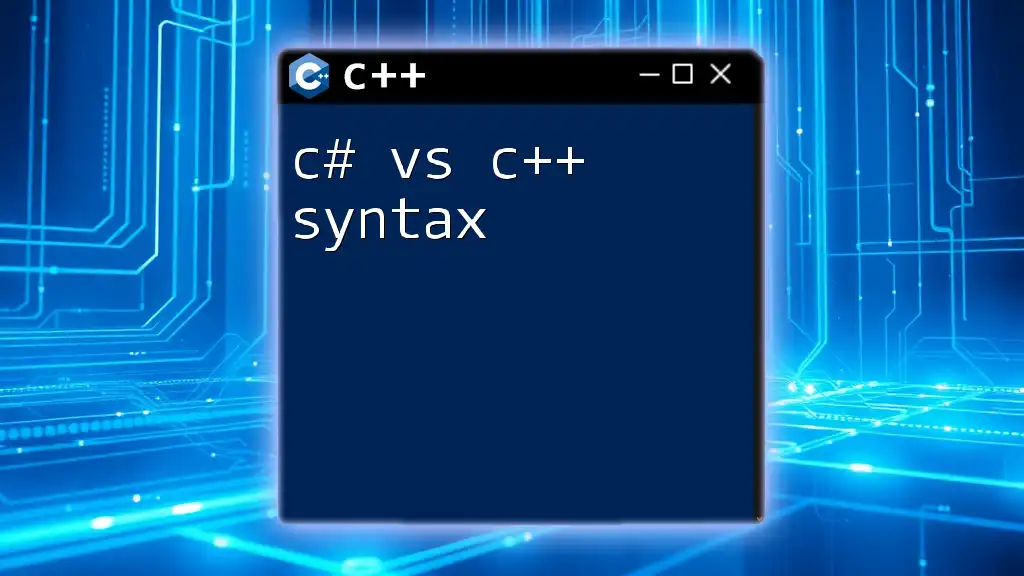
Conclusion
In conclusion, mastering vector C++ syntax is essential for efficient C++ programming. Vectors not only provide an advanced way to handle dynamic arrays, but they also come with numerous built-in features that facilitate complex data management without compromising performance.
Further Resources
To delve deeper into the world of vectors and the STL, consider exploring the official C++ documentation, reputable programming books, or interactive online courses that provide extensive exercises and examples.
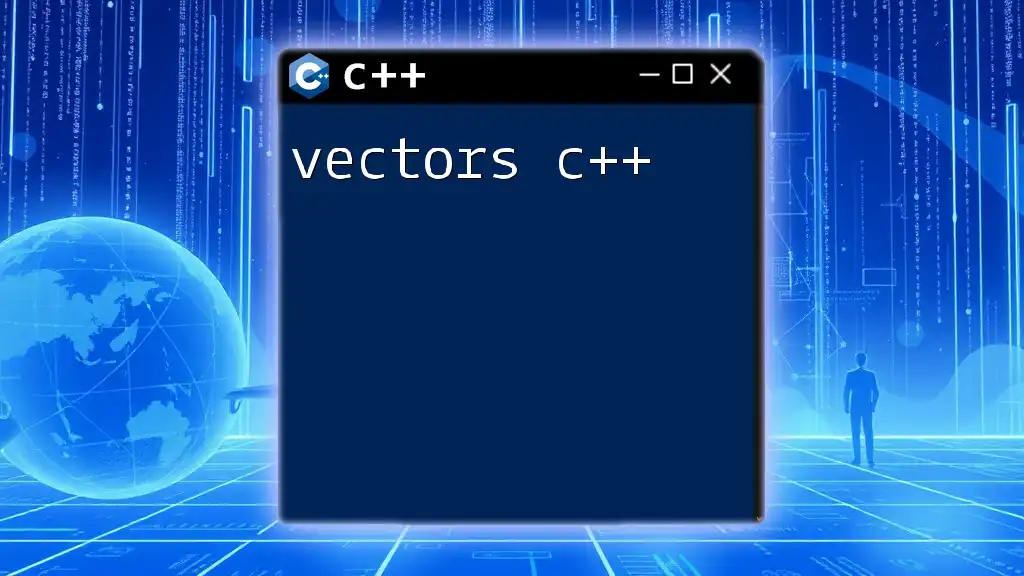
Call to Action
Practice using vectors by creating your implementations and exploring varying data types. Understanding their syntax will greatly enhance your skillset as a C++ programmer.