In C++, a vector of structs allows you to store and manage a dynamic array of structured data efficiently using the Standard Template Library (STL). Here's an example:
#include <iostream>
#include <vector>
struct Person {
std::string name;
int age;
};
int main() {
std::vector<Person> people = {{"Alice", 30}, {"Bob", 25}};
for (const auto& person : people) {
std::cout << person.name << " is " << person.age << " years old." << std::endl;
}
return 0;
}
Understanding Structs in C++
What is a Struct?
In C++, a struct (short for structure) is a user-defined data type that allows you to group related variables (known as members) under a single name. Structs are particularly useful for organizing complex data models. By using structs, you can maintain the relationship between data points, making your code more readable and maintainable.
Example of a Simple Struct
Consider the following example of a struct defined for a person:
struct Person {
std::string name;
int age;
};
In this example, the `Person` struct has two members: `name` and `age`. Each `Person` object can now encapsulate both a name and an age, making it clear that these two data points are related. Using structs helps keep your code organized and expressive, reducing the likelihood of errors.
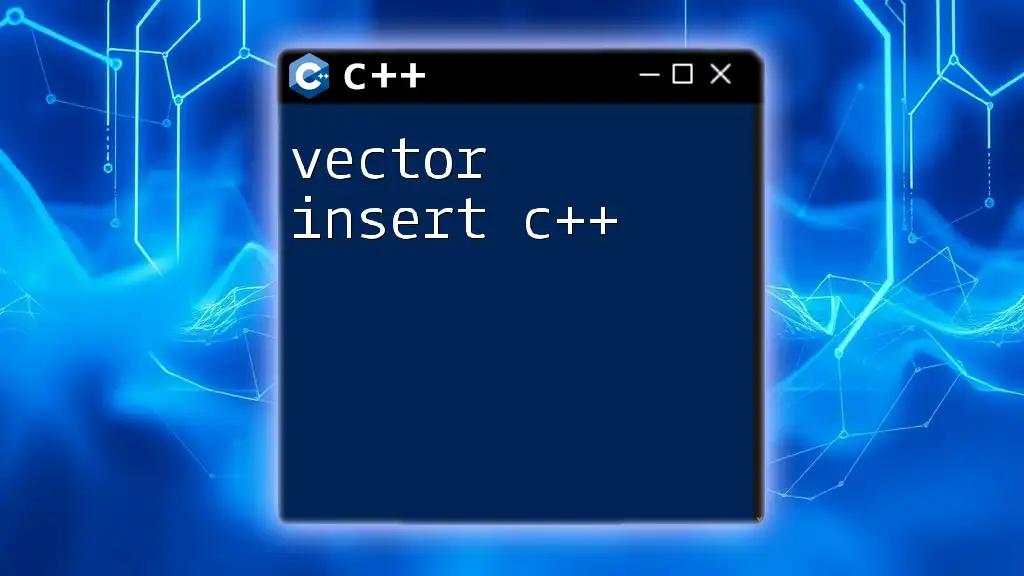
Introduction to Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array that can grow and shrink in size automatically. It is part of the Standard Template Library (STL) and leverages templates to provide type safety and performance. Vectors offer several advantages over traditional arrays, including the ability to resize on the fly and access built-in functions for manipulating data.
Why Use Vectors?
Vectors are highly convenient due to their ability to manage memory automatically. Unlike arrays, which have a fixed size, vectors' dynamic sizing makes them suitable for a wide range of applications. Additionally, vectors provide built-in methods that simplify complex tasks, such as sorting and searching, thereby enhancing performance and developer productivity.

Combining Vectors and Structs
Creating a Vector of Structs
To define a vector that holds structs, you can do so with the following syntax:
std::vector<Person> people;
In this case, `people` is a vector that can store multiple `Person` structs. The vector will automatically handle the memory allocation for you, allowing you to focus on your data management instead of low-level memory handling.
Adding Elements to a Vector of Structs
There are different methods for adding elements to your vector. The most common is using the `push_back()` method:
people.push_back({"Alice", 30});
people.push_back({"Bob", 25});
The `push_back()` method appends a new struct to the end of the vector, making it easy to build your collection dynamically. This feature is especially useful when you don’t know the number of elements you'll need in advance.
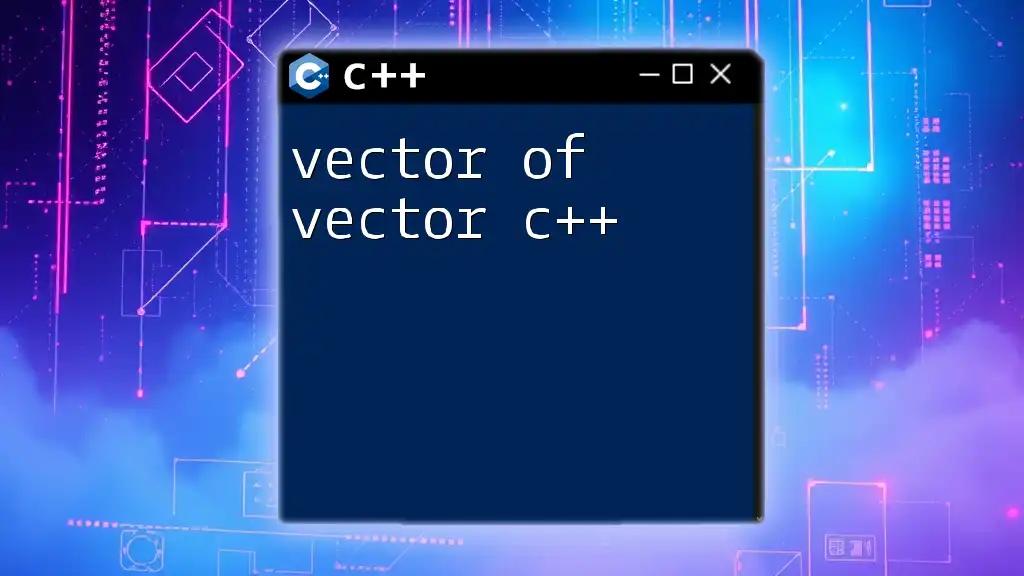
Accessing and Modifying Vector of Structs
Accessing Elements
You can easily access elements in a vector of structs, often through range-based loops:
for(const auto& person : people) {
std::cout << person.name << " is " << person.age << " years old.\n";
}
In this example, we're iterating through the vector and printing the name and age of each `Person`. Using a range-based loop makes the code cleaner and eliminates common pitfalls associated with traditional indexing.
Modifying Elements
Altering the attributes of a struct within a vector is straightforward:
people[0].age = 31;
Here, we access the first element in the `people` vector and update Alice's age. This feature allows for dynamic changes to your data models, enhancing the flexibility of your applications.
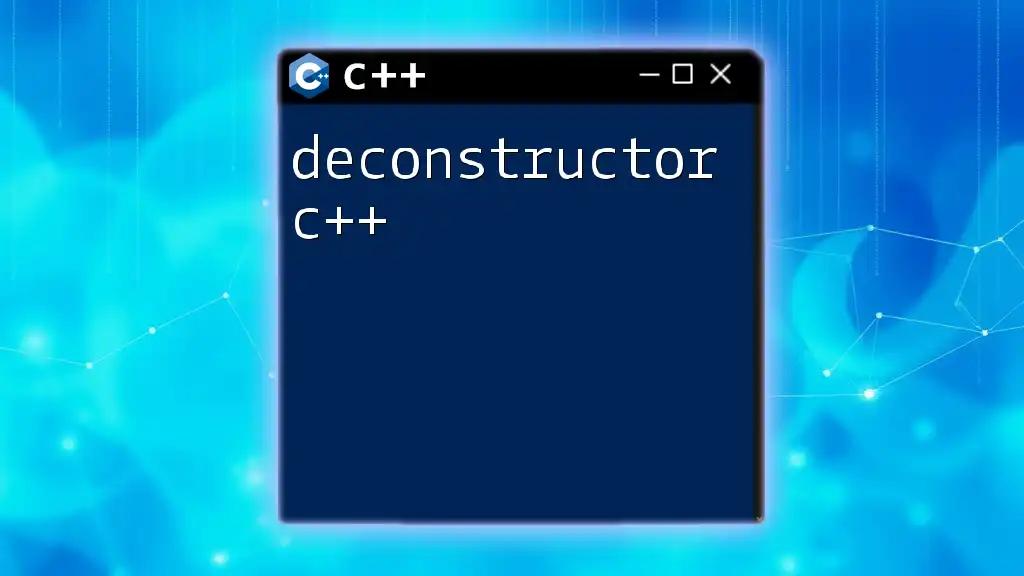
Searching through a Vector of Structs
Finding Elements
You can search for specific structs in the vector using algorithms. For example, with `std::find_if`, you can locate a person by their name:
auto it = std::find_if(people.begin(), people.end(), [](const Person& p) {
return p.name == "Alice";
});
In this snippet, a lambda function is used to define the search condition, and the result is an iterator pointing to the found element, if any.
Example of Search Function
You might want to encapsulate the search logic in a reusable function:
Person* findPersonByName(const std::vector<Person>& vec, const std::string& name) {
auto it = std::find_if(vec.begin(), vec.end(), [&name](const Person& p) {
return p.name == name;
});
return it != vec.end() ? &(*it) : nullptr;
}
This function allows you to retrieve a pointer to a `Person` struct based on the name provided. If the name doesn’t exist in the vector, it returns `nullptr`. Such a function enhances code reusability and modularity.

Sorting a Vector of Structs
Sorting Example
Triangles can easily be sorted, for instance, to organize people by age:
std::sort(people.begin(), people.end(), [](const Person& a, const Person& b) {
return a.age < b.age;
});
In this code, we leverage the `std::sort` function along with a lambda to define our sorting criteria. After sorting, the vector will be arranged in ascending order based on the `age` attribute.
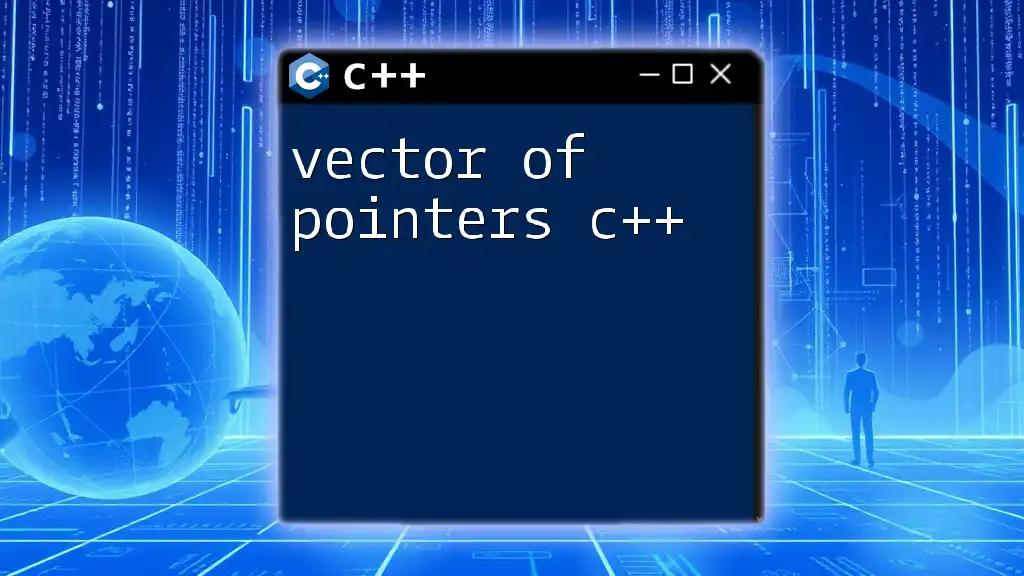
Conclusion
Using a vector of structs in C++ is a powerful approach for organizing complex data. This design pattern enhances your code's clarity, allowing for efficient memory usage and easy data manipulation. Practicing these concepts will not only improve your coding skills but will also bolster your confidence in using C++ effectively.
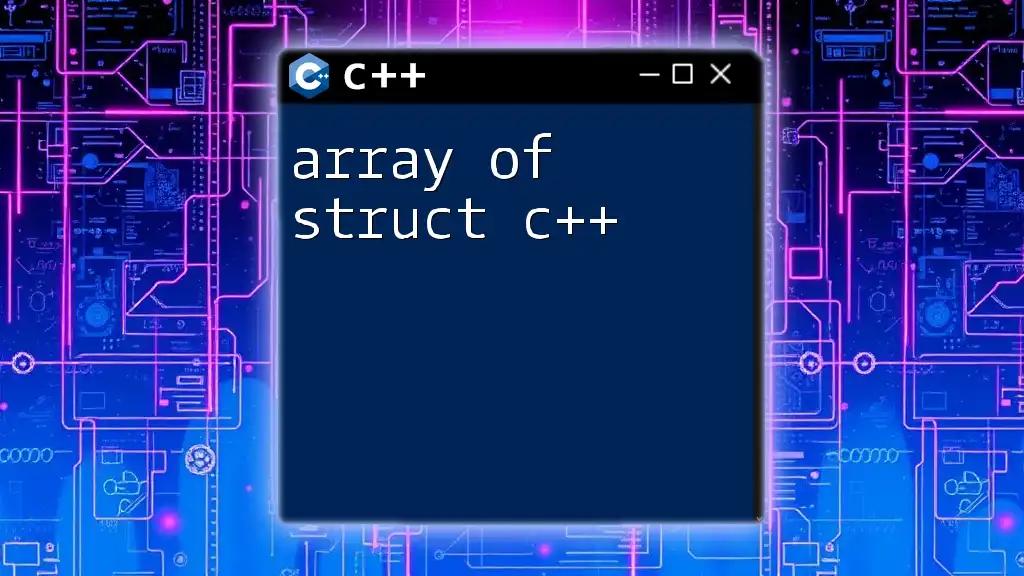
Additional Resources
For those looking to deepen their understanding of C++ vectors and structs, you might find it helpful to explore various websites, online courses, and books that focus on C++ programming. Community forums and coding practice sites can also provide valuable insights and opportunities to collaborate with others.
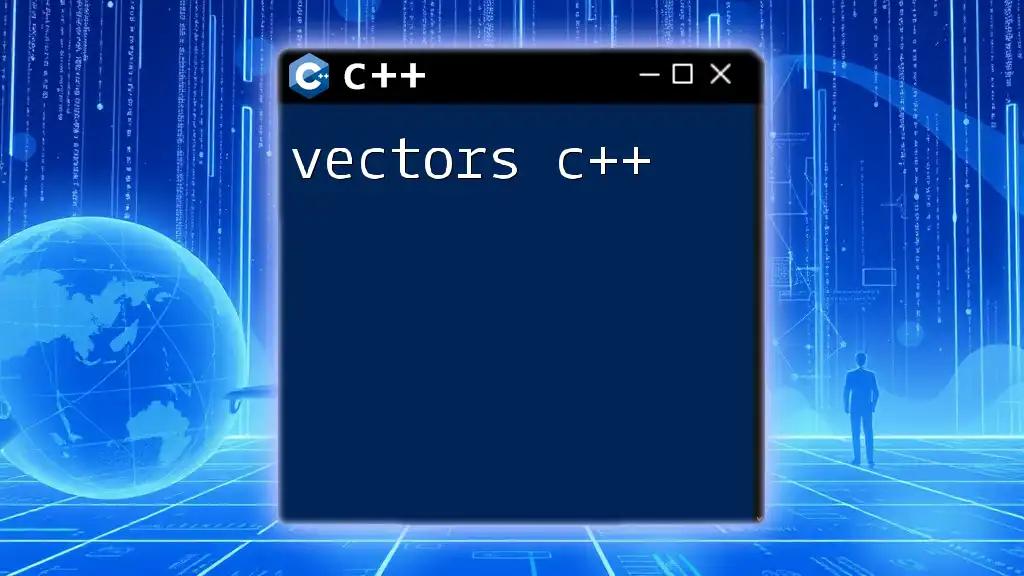
FAQs
What are the advantages of using vectors of structs over arrays?
Using vectors offers memory management, flexibility in size, and easy access to built-in functions, all of which contribute to cleaner and more efficient code.
Can structs hold other vectors or structs?
Yes, structs can contain other vectors or even other structs, allowing for the creation of complex data types that model real-world scenarios effectively.
How are vectors of structs better for OOP in C++?
Vectors of structs can promote better encapsulation and data management, making it easier to design object-oriented applications that require handling multiple related data objects.
Expanding on these concepts through hands-on coding will greatly improve your competency in C++. Happy coding!