In C++, a vector is a dynamic array that can resize itself automatically when elements are added or removed, and it is declared using the `std::vector` template.
Here's an example of vector declaration in C++:
#include <vector>
std::vector<int> myVector; // Declares a vector of integers
What is a Vector in C++?
A vector in C++ is a sequence container representing an array that can change in size. Unlike regular arrays, vectors can grow or shrink dynamically as elements are added or removed. This flexibility makes them an essential tool in C++ programming. Moreover, vectors come with a variety of built-in functions that allow for easy manipulation of data, providing utility far beyond that of static arrays.
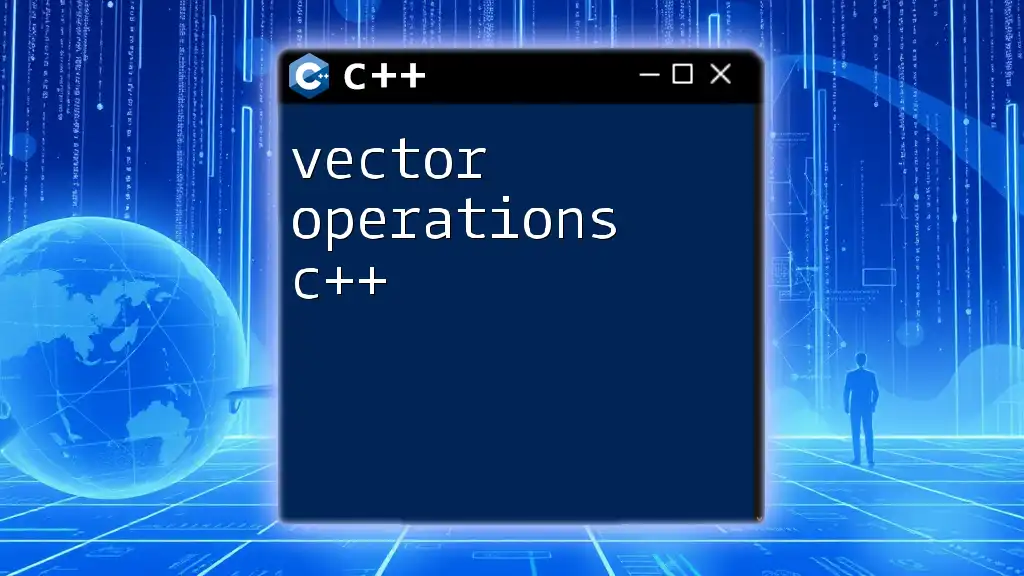
How to Declare a Vector in C++
Understanding the Syntax
Declaring a vector in C++ involves using the `std::vector` template class, which is part of the Standard Template Library (STL). It is crucial to include the header file `<vector>` at the beginning of your program, as this enables you to access the vector functionalities.
Basic Vector Declaration
To declare a vector, simply specify its type. For instance, if you want a vector that holds integers, you would write:
#include <vector>
std::vector<int> myVector; // Declares an empty vector of integers
In this code, `std::vector<int>` defines a vector named '`myVector`' that specifically stores integer types. The `std::` prefix denotes that the vector is part of the standard library.
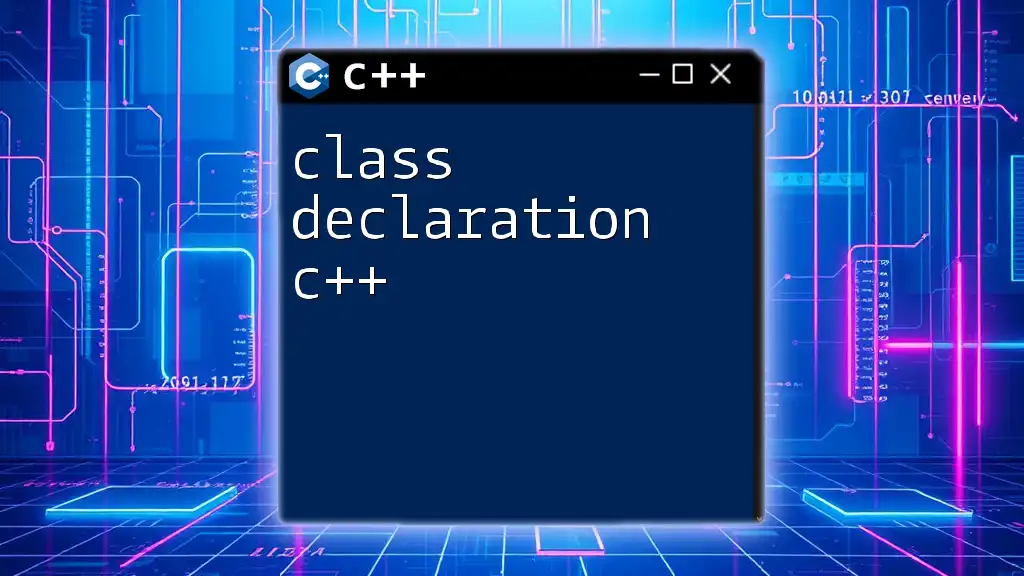
Initializing Vector in C++
Methods to Initialize a Vector
Vectors can be initialized in multiple ways, allowing for flexibility based on your requirements.
Default Initialization
If you want to create an empty vector without specifying a size or initial elements, you can do so with:
std::vector<int> myVector; // Default initializes an empty vector
This creates a vector without any elements inside it.
Initializing with Size
You may also initialize a vector with a defined size. This will create a vector that contains a specified number of elements, all initialized to the default value (like 0 for integers):
std::vector<int> myVector(10); // Initializes a vector with 10 elements
In this case, `myVector` will have ten integers, all set to zero.
Initializing with Values
If you want to create a vector with predetermined values, you can use an initializer list:
std::vector<int> myVector = {1, 2, 3, 4, 5}; // Initializes with specific values
The braces `{}` allow you to specify the contents directly, populating the vector with the given integers.
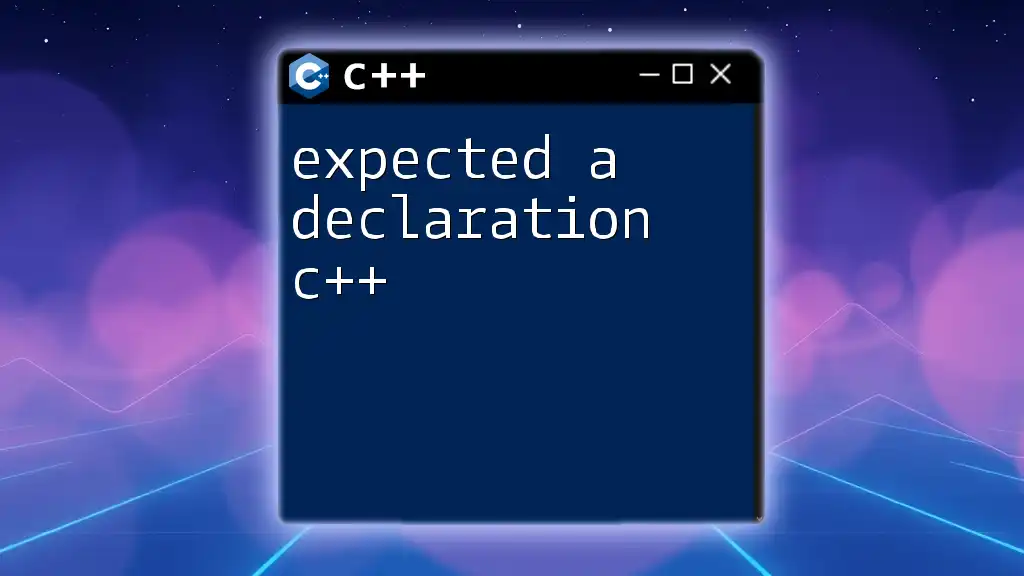
Creating a Vector in C++
Creating a Vector of Different Types
One of the strengths of C++ vectors is their ability to hold various types of data. For example:
std::vector<double> doubleVector; // Vector of doubles
std::vector<std::string> stringVector; // Vector of strings
This demonstrates how vectors can store different types, making them versatile data structures suitable for various applications.
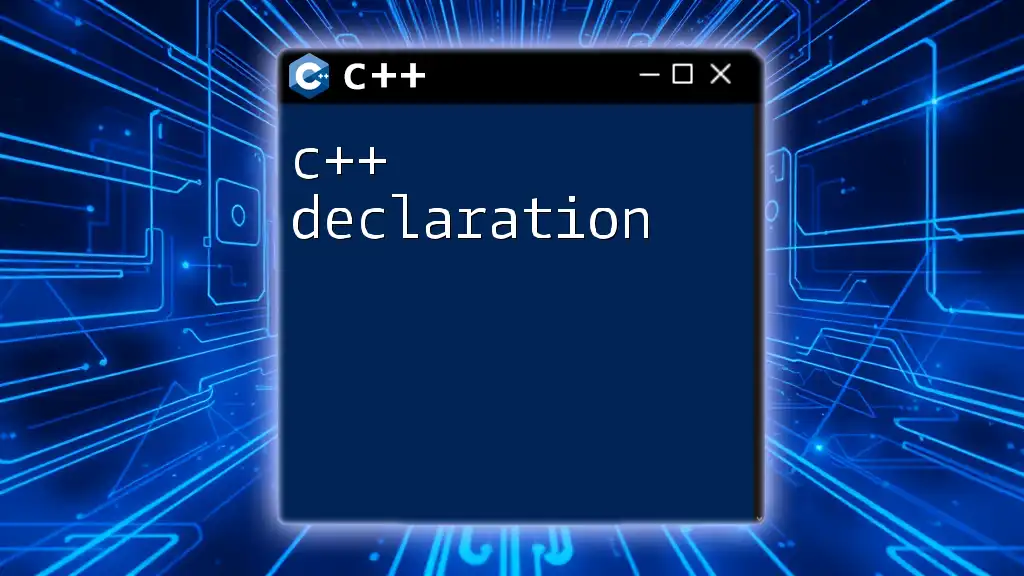
C++ Declaring Vectors with Different Operations
Once you have declared and initialized your vector, you can perform numerous operations on it.
Adding Elements
To add elements to the end of a vector, the `push_back` method is your go-to solution:
myVector.push_back(6); // Adds 6 to the end of the vector
Using `push_back`, you can continue to grow your vector as needed.
Accessing Elements
Accessing elements in a vector is straightforward, just like with arrays. Here’s how you can retrieve the value at a specific index:
int value = myVector[2]; // Accesses the third element
Remember that C++ uses zero-based indexing, meaning the first element is at index 0.
Removing Elements
If you want to remove the last element from the vector, you can do so with:
myVector.pop_back(); // Removes the last element
The `pop_back` function is simple yet effective for managing the size of your vector by removing its last element.
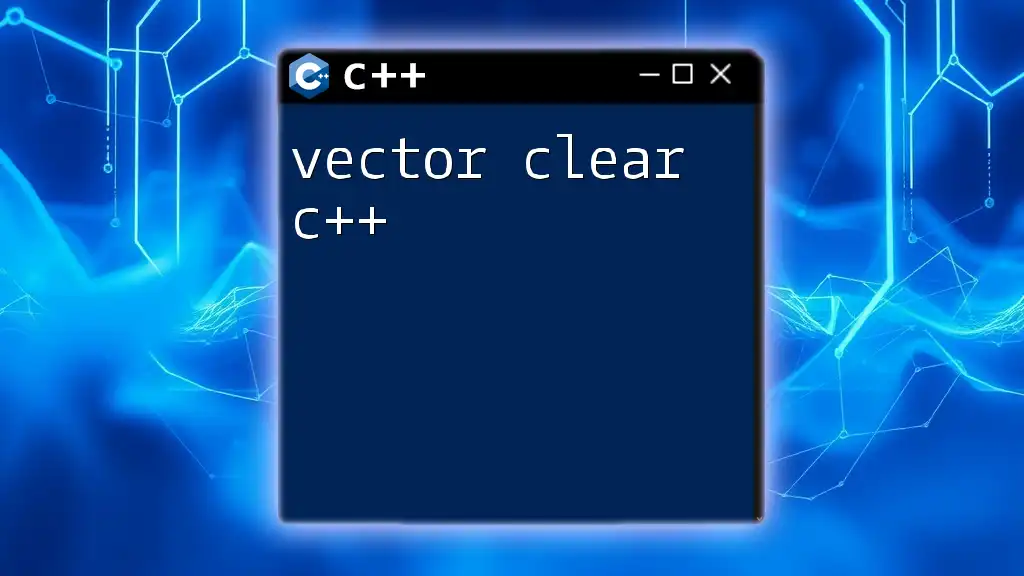
Common Mistakes to Avoid in Vector Declaration
While using vectors, several common pitfalls may arise for novice programmers:
-
Forgetting to include the `<vector>` header file: Always ensure that you have included the necessary libraries before using vectors, as failing to do so will lead to compilation errors.
-
Improper initialization: Attempting to access elements in an uninitialized vector may result in unexpected behavior or crashes. Always initialize your vector before using it.
-
Misunderstanding zero-based indexing: Remember that the first element has an index of 0, leading to potential out-of-bounds errors if you're not careful.
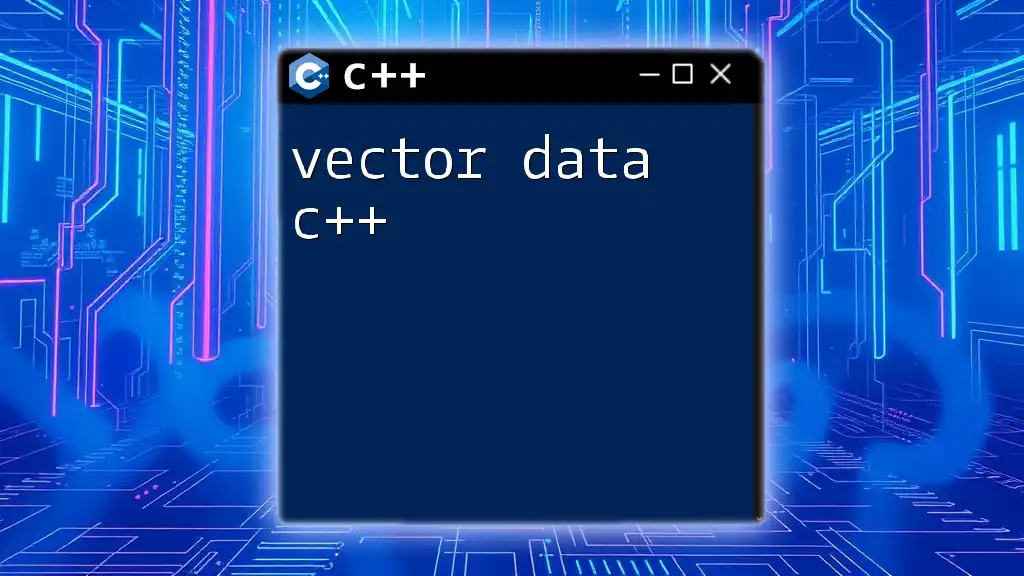
Conclusion
Mastering vector declaration in C++ is fundamental for anyone looking to make the most out of this powerful programming language. Vectors provide a rich set of features that can significantly simplify data management tasks in your applications. It's important to experiment and apply these concepts so that you can leverage their full potential in your programming journey.
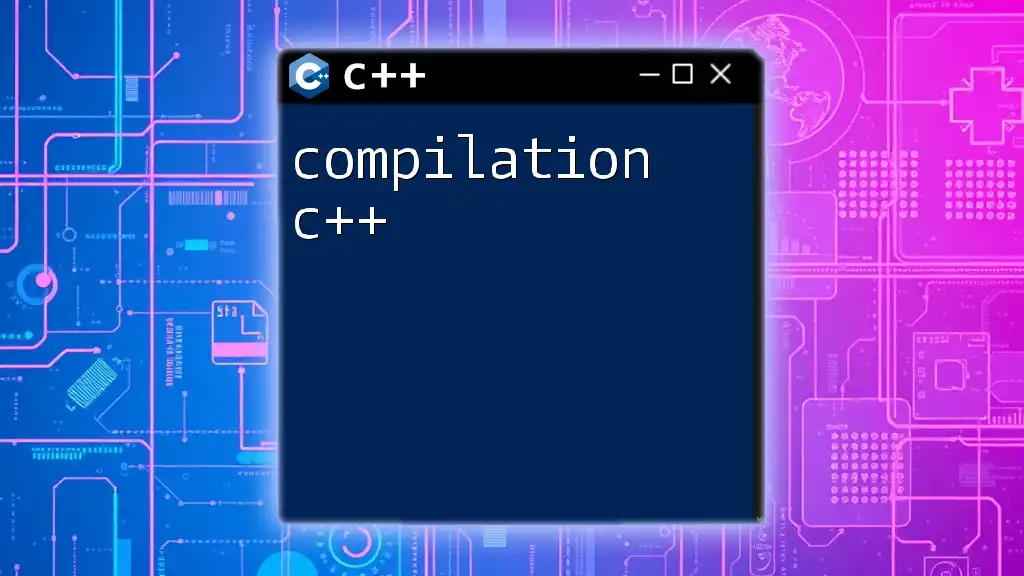
Additional Resources
To deepen your understanding of vectors in C++, consider these learning resources:
- Tutorials on advanced vector operations on platforms like Codecademy or Coursera.
- Recommended C++ programming textbooks, such as "C++ Primer" by Stanley Lippman, which provide deeper insights into the STL and vectors.
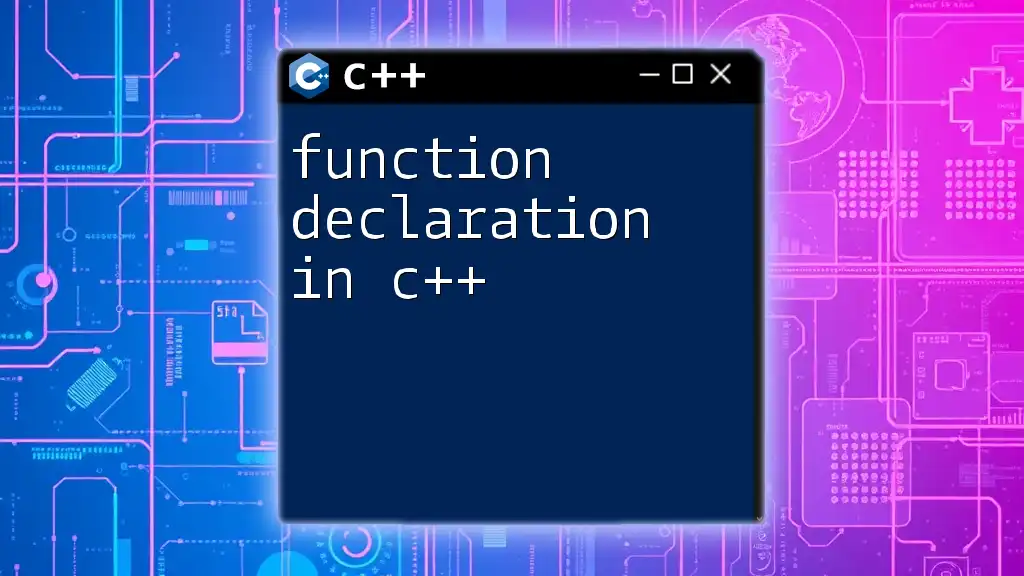
FAQs
-
What is the maximum size of a vector in C++? Generally, the size of a vector is only limited by the available memory on your machine, subject to system constraints.
-
Can a vector hold objects of non-POD types? Yes, vectors can hold objects of classes and structs, provided they adhere to the necessary rules of object copying and assignment.
-
How is vector memory managed in C++? Vectors automatically manage their memory, allocating more space when needed. However, it's essential to be mindful of the performance implications of frequent resizing.