The error "expected a declaration" in C++ typically occurs when the compiler encounters a statement or expression where it expects a variable declaration or function definition, often due to missing semicolons or incorrect syntax.
Here's an example that may trigger this error:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl // Missing semicolon here
return 0;
}
What is a Declaration in C++?
Definition of a Declaration
In C++, a declaration is a statement that introduces a name into the program and specifies its type. Essentially, declarations allow the compiler to know the types and structures you will be using so that it can perform type checking. For instance, a function declaration lets the compiler know what the function's name is, what parameters it takes, and what its return type is.
It's important to note the distinction between declarations and definitions. A declaration merely states what something is (e.g., a variable or a function), while a definition also allocates storage and initializes the variable or provides the implementation of the function.
Importance of Declarations
Declarations play a crucial role in C++ because they facilitate type checking during compilation, helping prevent type-related errors. By declaring a variable or a function, you inform the compiler of the entity’s existence before it is used, which is essential for efficient memory management and code clarity.
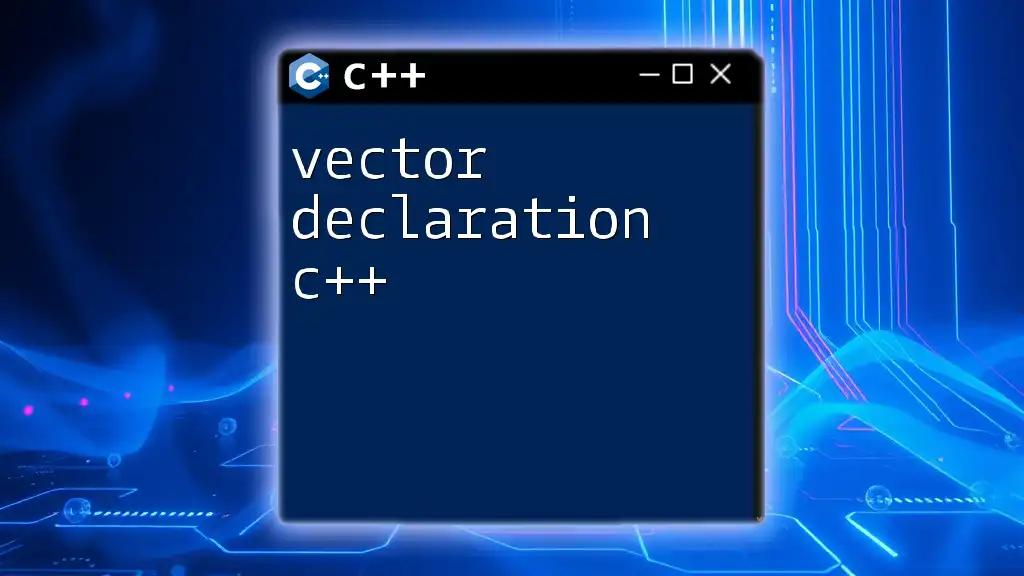
Understanding the Error Message: "Expected a Declaration"
Overview of the Error Message
The error message "expected a declaration" occurs when the C++ compiler anticipates a declaration but encounters something other than a valid declaration. This message can be alarming for developers, especially for those new to the language, as it may not accurately specify where the issue is, leading to frustration.
Common Causes of the Error
Missing Semicolons
One of the most common culprits of the "expected a declaration" error is the absence of a semicolon at the end of a statement. In C++, semicolons are crucial as they denote the end of a statement. For example, consider the following code snippet:
class MyClass // No semicolon here
{
int x;
}
In this snippet, the lack of a semicolon after the class declaration will lead to the "expected a declaration" error.
Incorrect Use of Scope
C++ has specific rules regarding scope, which dictate where variables and functions exist and can be used. Using scope incorrectly can lead to confusion and errors. For example:
int main() {
int a = 5;
if (a == 5)
int b = 10; // Incorrect scope for declaration
}
Here, declaring `b` inside the `if` statement leads to an invalid declaration because the compiler expects it to be a standalone statement. Variables must be declared at the beginning of a block.
Misplaced Code Blocks
The arrangement of code blocks can greatly affect the program's function and lead to compilation errors. Consider the following example:
void myFunction() {
// Some code
} // Correctly placed function
int myVar = 10 // Missing semicolon here
void anotherFunction() {
// Some code
}
In this case, the missing semicolon after the variable declaration `myVar` will result in the "expected a declaration" error.
Redundant or Incorrect Keywords
Using keywords incorrectly also leads to confusion for the compiler. A common mistake occurs when return statements appear before function declarations or definitions. For instance:
return int myFunction(); // Incorrect
This snippet incorrectly uses the `return` keyword, causing the compiler to expect a valid declaration, resulting in the error message.
Unresolved Identifiers
Another scenario that can trigger the "expected a declaration" error is the use of unresolved identifiers—where the C++ compiler cannot find an identifier that has been referenced. For example:
foo(); // If foo is not declared or defined anywhere
Here, if `foo` hasn't been previously declared or defined, the compiler doesn't know how to interpret this call, leading to an error.

How to Fix the "Expected a Declaration" Error
Step-by-Step Troubleshooting
To resolve the "expected a declaration" error, consider the following steps:
Step 1: Check for Typographical Errors
A simple typo can cause significant headaches. Review your code carefully to spot any unintentional mistakes, such as misspellings or misplaced symbols that could disrupt declarations.
Step 2: Ensure Proper Semicolon Use
Always check that semicolons are present at the end of your declarations to signal the completion of statements. This simple habit can save you a lot of time debugging.
Step 3: Review Scoping Rules
Ensure that your variables and functions are declared within the correct scope, adhering to C++'s rules on how and where different types can be declared.
Step 4: Check Identifier Declarations
Make sure that every identifier you use has been declared before its first use. This will prevent errors related to unresolved identifiers and improve the overall clarity of your code.
Code Refactoring
Organizing Your Code
Proper organization of your code is essential to avoid confusion and inadvertent errors. Use comments to clearly mark different sections in your code, which will greatly help both you and anyone else reading your code in the future.
Use Proper Naming Conventions
Naming conventions matter. Using descriptive and clear names for your variables and functions not only makes your code readable but also helps in identifying declarations quickly.
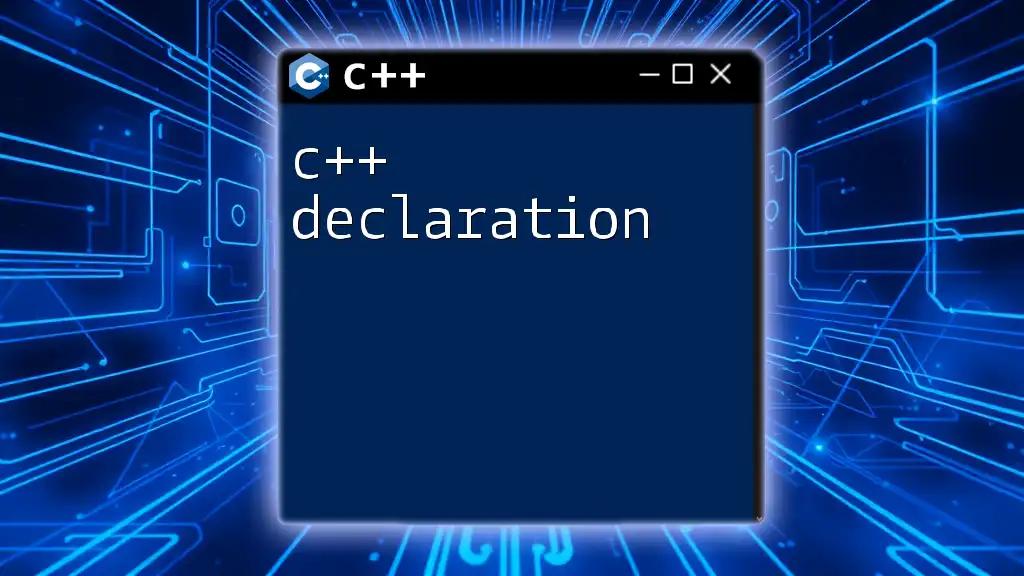
Best Practices to Avoid "Expected a Declaration" Errors
Consistent Formatting
Maintaining proper code formatting enhances readability. Consistent indentation and spacing help to visualize the structure of your code naturally, which can help identify parts that may lead to declaration errors.
Familiarize with C++ Syntax Rules
Being well-acquainted with the syntax rules of C++ is vital. Investing time in understanding C++'s language specifications can save you from many common pitfalls associated with declaration errors.
Utilize IDE Features
Integrating an Integrated Development Environment (IDE) that offers syntax highlighting and error detection features can be invaluable. Such tools often underline errors in real-time, allowing you to catch mistakes as you code.

Conclusion
Understanding the "expected a declaration c++" error allows developers to handle this common yet frustrating issue effectively. By appreciating the significance of declarations and following best practices in code structure and syntax, you can significantly reduce the occurrence of such errors in your C++ programs. The learning process is continuous, so remain open to practice and gathering feedback on your work to become a more proficient C++ developer.

Additional Resources
Recommended Reading
Delve into books and online courses tailored for C++ enthusiasts looking to deepen their understanding. Topics might include advanced C++ techniques, error handling, and best practices in programming.
Community Assistance
Don’t hesitate to leverage the vast array of forums and communities available. Engaging with other developers can provide insights and solution strategies for overcoming declaration-related issues and other challenges.