The "expected unqualified-id" error in C++ typically occurs when a compiler encounters code syntax that does not conform to the expected identifiers or valid declarations, often due to missing or misplaced punctuation or keywords.
Here’s a code snippet that illustrates a common scenario leading to this error:
#include <iostream>
int main() {
int 1a = 5; // error: expected unqualified-id before numeric constant
std::cout << "Value: " << 1a << std::endl;
return 0;
}
What Does "Expected Unqualified-Id" Mean?
The "expected unqualified-id" error in C++ indicates that the compiler was expecting an identifier (like a variable name or function name), but it instead encountered something unexpected. This error frequently arises when there are syntax issues or when identifiers are improperly defined or invoked.
Why Does It Happen?
Understanding the root causes of this error can help you avoid it in the future. The error can stem from several common mistakes, including:
- Missing or incorrect function return types
- Incorrectly declared classes and structures
- Improper usage of namespaces
- Typographical errors or misplaced syntax
By recognizing these scenarios, you can strategically troubleshoot and resolve this frustrating error.
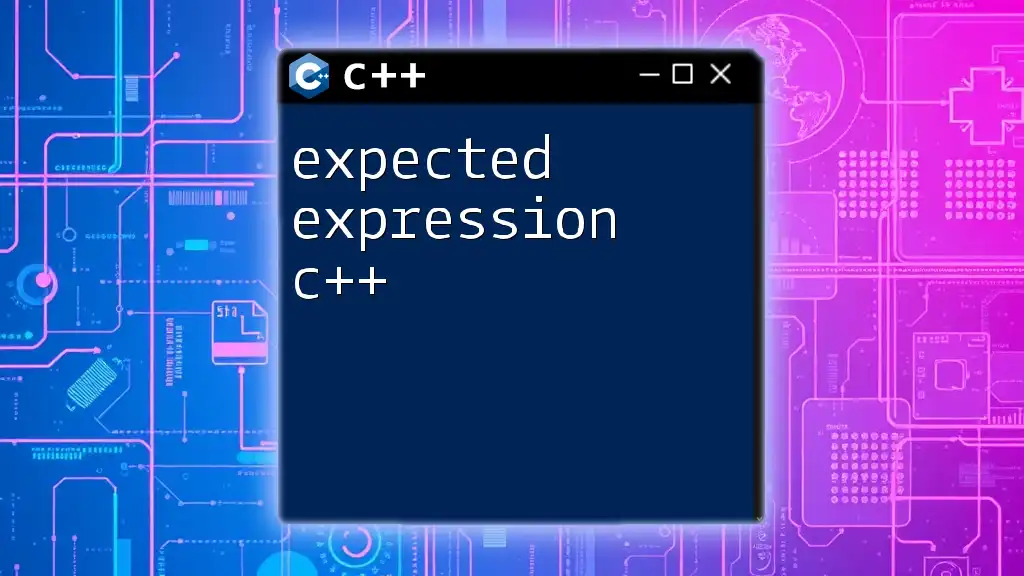
Common Scenarios Leading to the Error
Function Declarations and Definitions
Incorrect declarations or definitions of functions are a frequent source of the "expected unqualified-id" error. For instance, failing to specify a return type can confuse the compiler.
Example 1: Missing a return type in a function definition
int main() {
std::cout << "Hello, World!";
// Error: expected unqualified-id
}
In the above example, the function `main()` is missing a return type declaration. Correcting it to `int main()` will fix the error.
Class and Struct Definitions
Using improper syntax when declaring classes or structs can also lead to this error.
Example 2: Incorrect inheritance declaration
class B {};
class A : B { // Error: expected unqualified-id
};
In this example, the class `A` tries to inherit from `B` without using the proper syntax (`class` or `struct`). The correct declaration would be:
class A : public B {
};
Namespace and Scopes
Misusing namespaces or failing to comply with C++ scoping rules often results in "expected unqualified-id" errors.
Example 3: Incorrect namespace usage
namespace MyNamespace {
void MyFunction() {}
}
MyNamespace::MyFunction; // Error: expected unqualified-id
To correctly invoke the `MyFunction()` from `MyNamespace`, ensure to include parentheses:
MyNamespace::MyFunction(); // Correct usage
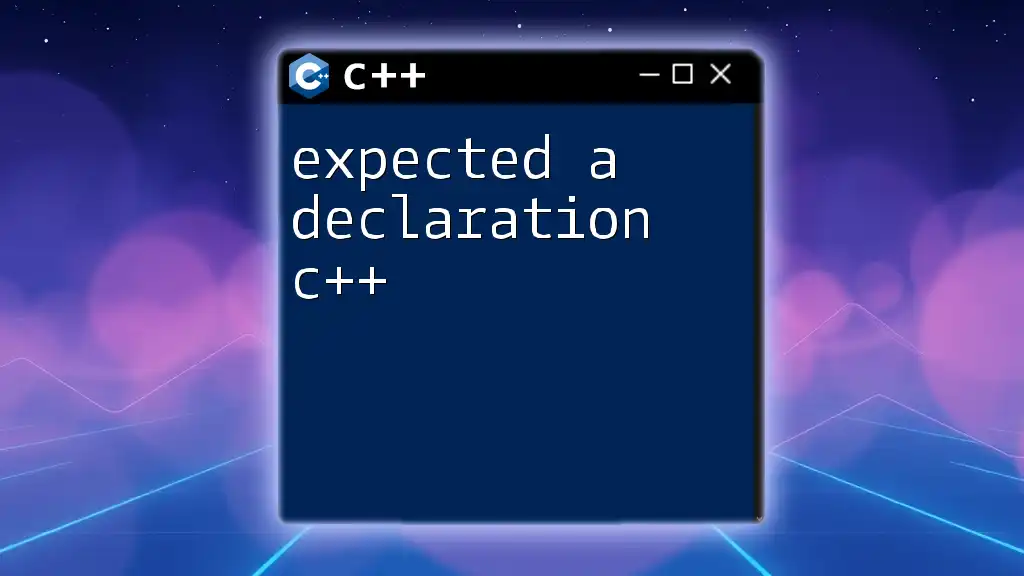
Fixing "Expected Unqualified-Id" Errors
Identifying the Origin of the Error
When you encounter the "expected unqualified-id" error, the first step is to read the error message closely. Modern C++ IDEs and compilers provide contextual hints that help trace the source of the errors.
Consider breaking down your code into smaller sections and compiling them one at a time. This technique helps isolate the problematic code and can significantly simplify the debugging process.
Code Correction Strategies
General Syntax Corrections
To correct this error, pay attention to your syntax. Ensure that all function and variable declarations are accurate and include necessary return types.
Using Proper Scopes
Scoping issues can also be a common pitfall. Make sure to utilize namespaces appropriately and always include parentheses when calling functions. For instance:
MyNamespace::MyFunction(); // Proper invocation
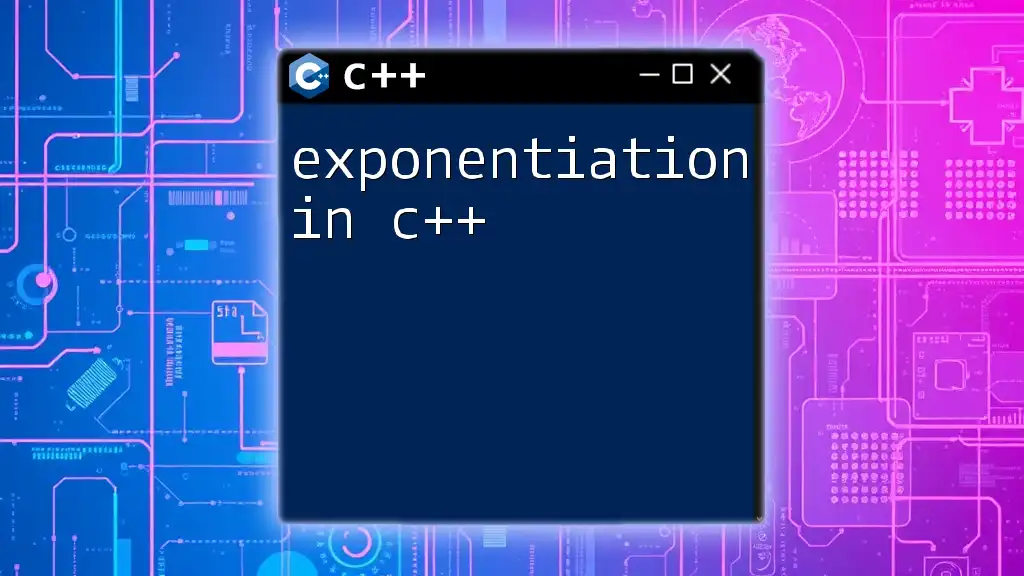
Best Practices to Avoid This Error
Consistent Style Guidelines
Establishing consistent coding standards can minimize the occurrence of such syntax errors. Following a clear style guide allows for easier identification of mistakes and improves overall code readability.
Code Review and Testing
Conducting peer reviews allows others to scrutinize your code before it goes live. Implementing a solid testing process also helps. Testing code snippets individually ensures that each piece functions as intended without introducing errors.
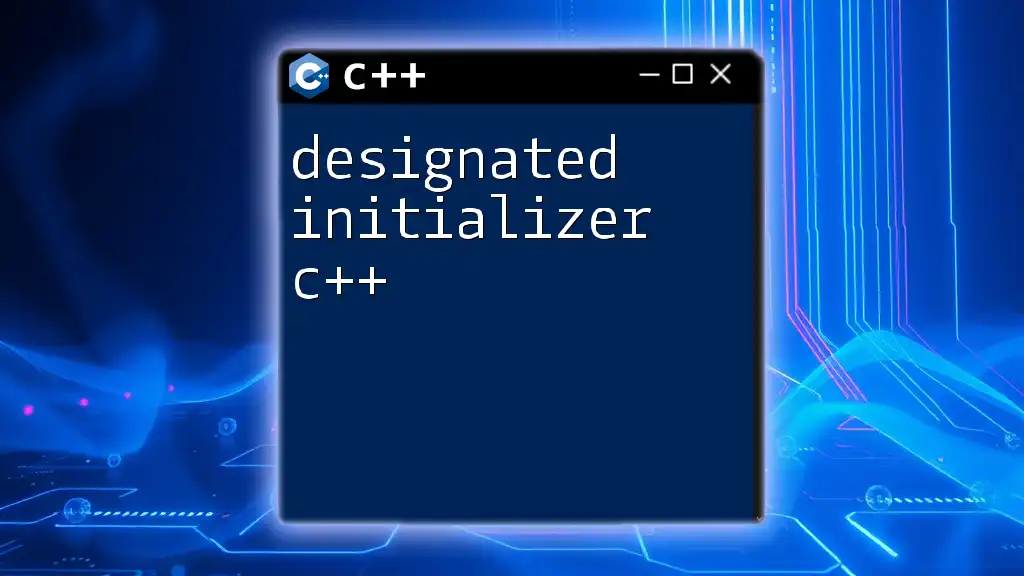
Tools and Resources for Debugging
C++ Compilers and IDEs
Choosing the right tools can greatly influence your coding experience. Compilers like GCC or IDEs like Visual Studio and Code::Blocks offer detailed error messages that pinpoint issues effectively, allowing for quicker resolution of errors like the "expected unqualified-id" message.
Online Forums and Documentation
When you encounter persistent issues, don't hesitate to leverage online resources like documentation or community forums. Platforms like Stack Overflow boast a wealth of knowledge from experienced C++ developers, making them invaluable for troubleshooting.
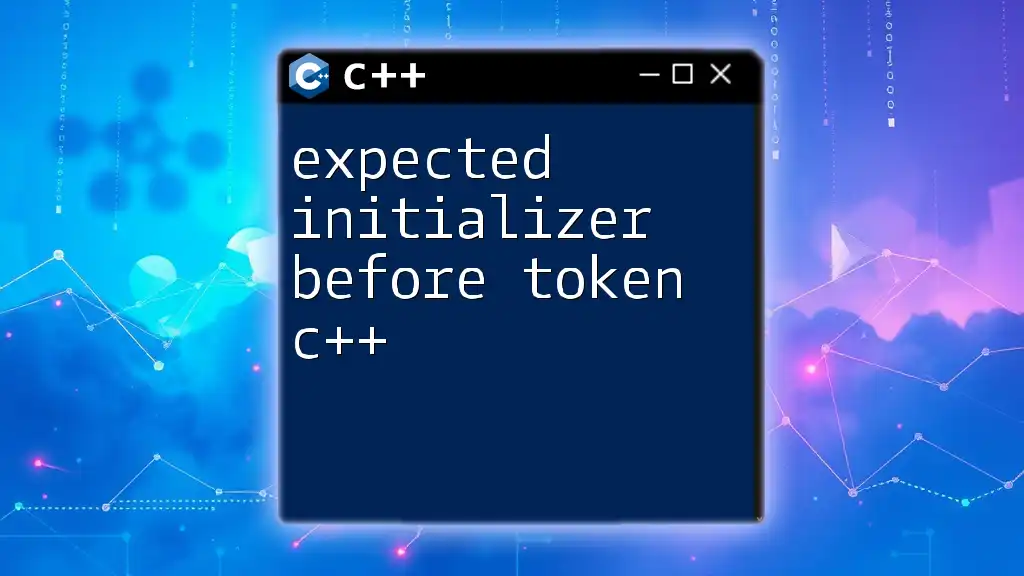
Summary
Mastering the nuances of C++ syntax helps prevent frustrating errors such as "expected unqualified-id." Recognizing common scenarios that lead to this error and implementing best practices can save you valuable time and enhance your coding skills.
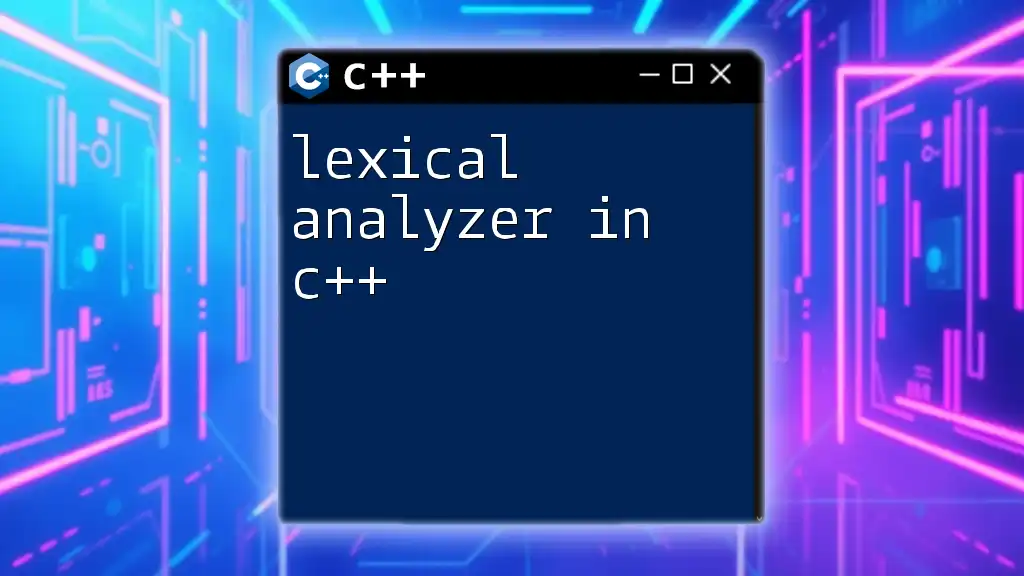
Conclusion
Learning the intricacies of C++ programming is a continual journey. Emphasizing understanding and addressing errors like "expected unqualified-id" is vital for any programmer aiming for proficiency. Whether through further education or practice, improving your skills will bolster your confidence in coding.
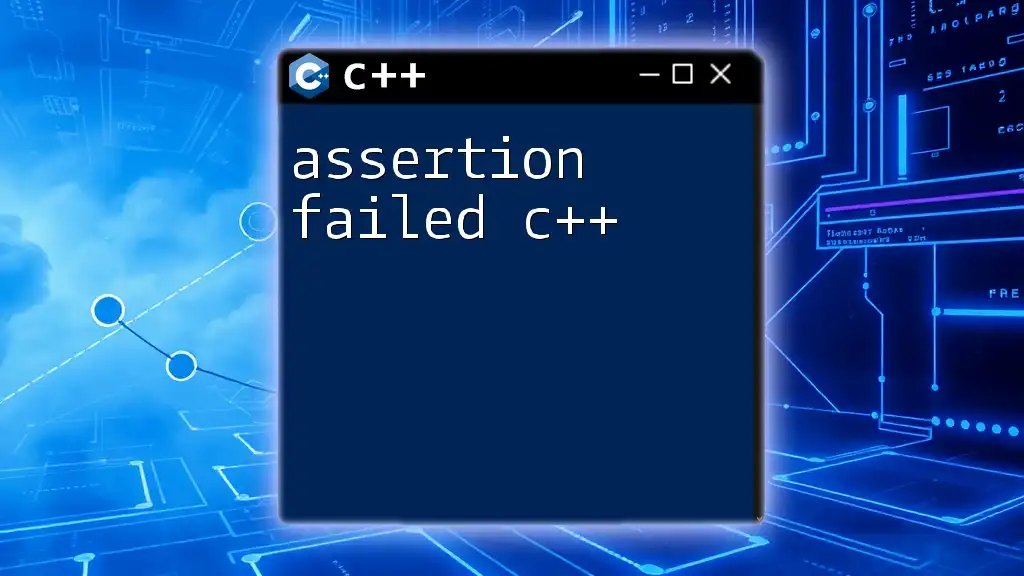
Additional Resources
Access reliable resources to deepen your C++ knowledge, including textbooks, online courses, and tutorials. Joining community support forums also opens up avenues for assistance and continued learning. Embrace these opportunities to enhance your programming expertise!