Object slicing in C++ occurs when a derived class object is assigned to a base class object, leading to the loss of the derived class's specific attributes and methods.
Here’s a code snippet illustrating object slicing:
#include <iostream>
class Base {
public:
int baseValue;
virtual void show() { std::cout << "Base Value: " << baseValue << std::endl; }
};
class Derived : public Base {
public:
int derivedValue;
void show() override { std::cout << "Base Value: " << baseValue << ", Derived Value: " << derivedValue << std::endl; }
};
int main() {
Derived d;
d.baseValue = 1;
d.derivedValue = 2;
Base b = d; // Object slicing occurs here
b.show(); // Output: Base Value: 1
return 0;
}
Understanding Object Slicing
What is Object Slicing?
Object slicing in C++ refers to a phenomenon that occurs when an object of a derived class is assigned to a variable of a base class type. During this assignment, the derived class-specific attributes and methods are "sliced off," leaving only the base class attributes. This often leads to unintended data loss and unexpected behavior in programs.
The Mechanism of Object Slicing
When you assign a derived class object to a base class object, the following happens:
- Memory Allocation: C++ allocates memory for the base class instance.
- Data Copying: The compiler copies only the parts of the derived object that match the base object, effectively discarding the derived class's members.
This can be particularly problematic when dealing with polymorphism, as the unique features of a derived class are lost.
To illustrate this, consider the following hierarchy:
class Base {
public:
int baseValue;
};
class Derived : public Base {
public:
int derivedValue;
};
If we create an instance of `Derived` and assign it to a variable of type `Base`, `derivedValue` will be lost during the assignment.
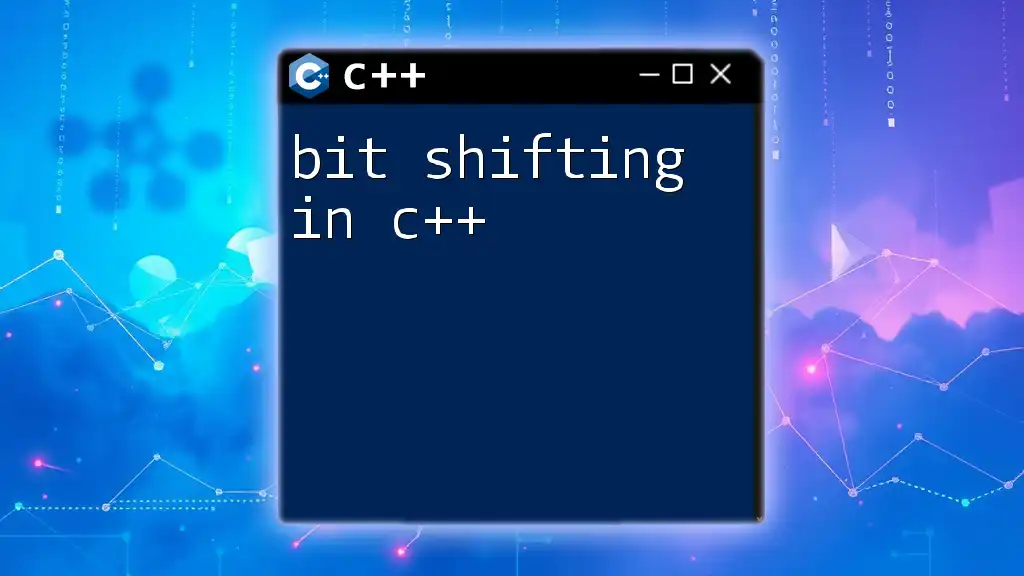
Why Object Slicing is a Problem
Loss of Data
Object slicing leads to loss of data because any derived class-specific information is discarded.
Example:
Derived d;
d.baseValue = 10;
d.derivedValue = 20;
Base b = d; // Object slicing occurs here
After this assignment, `b.baseValue` will have the value `10`, but `derivedValue` is no longer accessible since `b` is of type `Base`.
Efforts to Avoid Slicing
To mitigate the problems associated with object slicing, developers need to implement common strategies that preserve the polymorphic behavior of their classes.
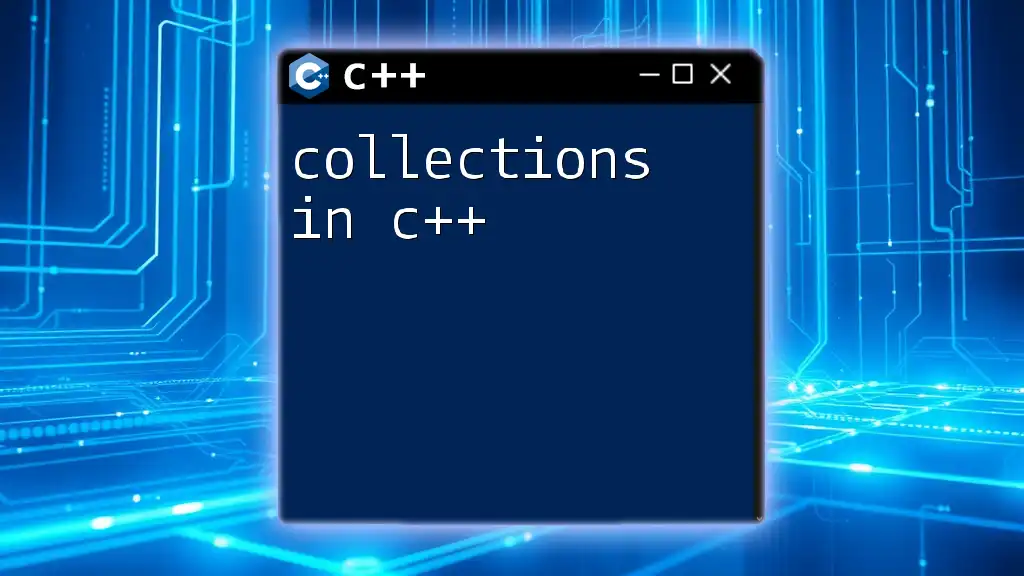
How to Avoid Object Slicing
Use of Pointers or References
One of the most effective strategies to avoid object slicing is to use pointers or references to objects instead of directly assigning them. This way, the program retains access to the derived class members.
Example:
Base* b = new Derived();
b->baseValue = 10;
// We can still use Derived class functionality through the pointer.
In this case, since `b` is a pointer to `Base`, there is no slicing, and we can still access the derived class functionalities.
Smart Pointers and Object Management
With the advent of C++11, smart pointers like `std::shared_ptr` and `std::unique_ptr` provide a modern approach to manage memory while avoiding object slicing.
Example using `std::shared_ptr`:
#include <memory>
class Base {
public:
virtual void display() { std::cout << "Base class\n"; }
};
class Derived : public Base {
public:
void display() override { std::cout << "Derived class\n"; }
};
std::shared_ptr<Base> b = std::make_shared<Derived>();
b->display(); // Outputs: "Derived class"
This method not only helps avoid slicing but also manages the lifecycle of the objects efficiently.
Design Patterns to Prevent Slicing
Implementing certain design patterns can effectively prevent object slicing. For instance, the Factory Pattern allows you to create derived instances without exposing their full complexity.
Example demonstrating the Factory Pattern:
class Base {
public:
virtual void sayHello() = 0;
};
class Derived : public Base {
public:
void sayHello() override { std::cout << "Hello from Derived!\n"; }
};
std::unique_ptr<Base> createDerived() {
return std::make_unique<Derived>();
}
int main() {
std::unique_ptr<Base> obj = createDerived();
obj->sayHello(); // Outputs: "Hello from Derived!"
}
Using a factory function helps encapsulate the creation of derived classes and ensures that object slicing does not occur.
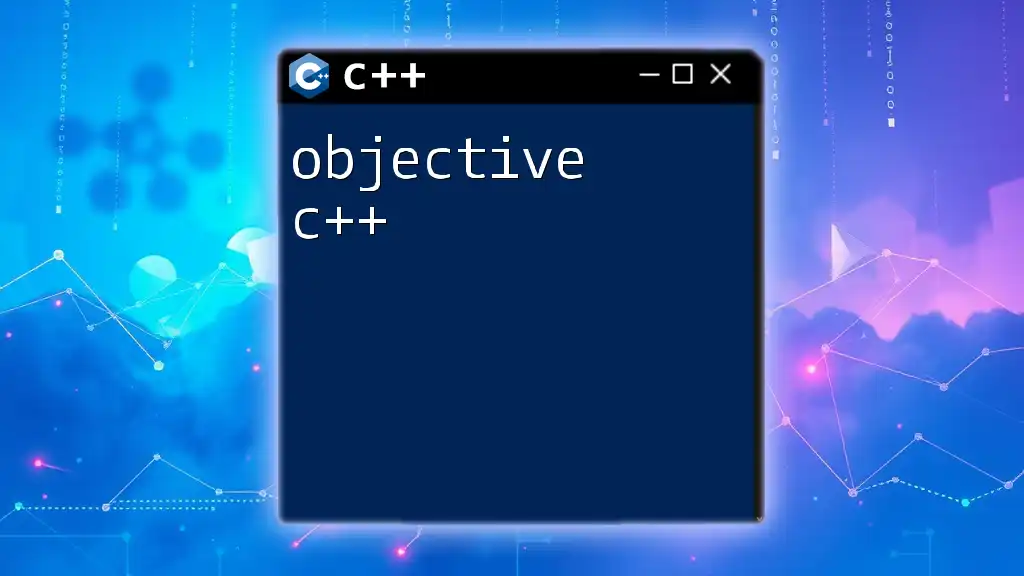
Handling Object Slicing in Your Code
Debugging Object Slicing Issues
Detecting object slicing can sometimes be tricky. Pay close attention to compiler warnings. If your derived class has members that do not appear to be accessing properly, check if they are being sliced due to incorrect type assignment.
You can also utilize tools like Valgrind or other memory management tools to track object lifetimes and check for anomalies caused by slicing.
Refactoring to Prevent Slicing
If you identify existing code where slicing is present, consider refactoring to use pointers or references. You can also take the opportunity to revisit class hierarchies, ensuring proper abstract base classes exist to manage polymorphism effectively.
Refactoring Example:
Before:
void process(Base b) {
// Process base class object, risking slicing
}
After:
void process(Base& b) {
// Process base class reference without slicing
}
This simple change helps to preserve the entire object structure without losing derived-specific data.
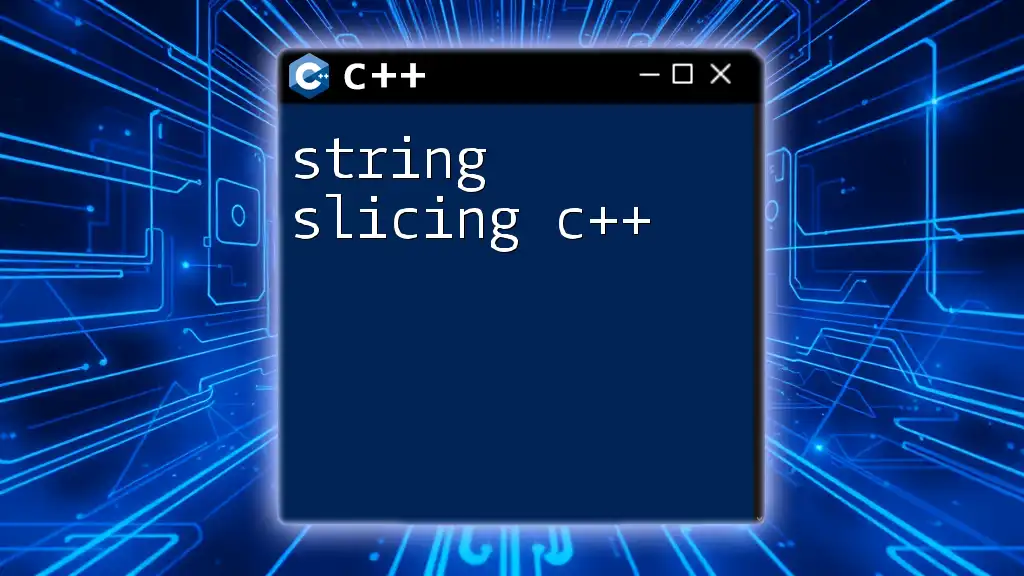
Best Practices to Remember
Key Takeaways
- Always be aware of object slicing when dealing with polymorphism in C++.
- Prefer using pointers or references to avoid slicing issues.
- Utilize smart pointers for memory management alongside preventing object slicing.
- Implement design patterns that encapsulate object creation and management.
Additional Resources
For more in-depth understanding, consider the following resources:
- C++ programming textbooks that cover object-oriented principles.
- Online courses dedicated to C++ best practices.
- Community forums like Stack Overflow for real-world examples and solutions.
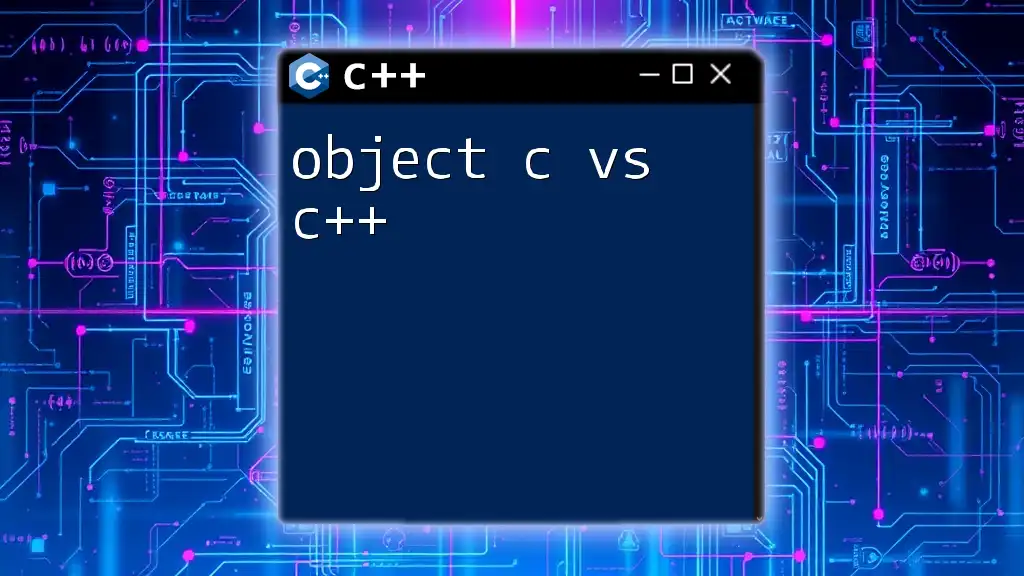
Conclusion
Understanding and preventing object slicing in C++ is crucial for writing effective and maintainable code. By using pointers and references, employing smart pointers, and following design patterns, developers can safeguard their programs against this common pitfall. Apply these strategies in your own projects to enhance your coding skills and ensure your classes work as intended.
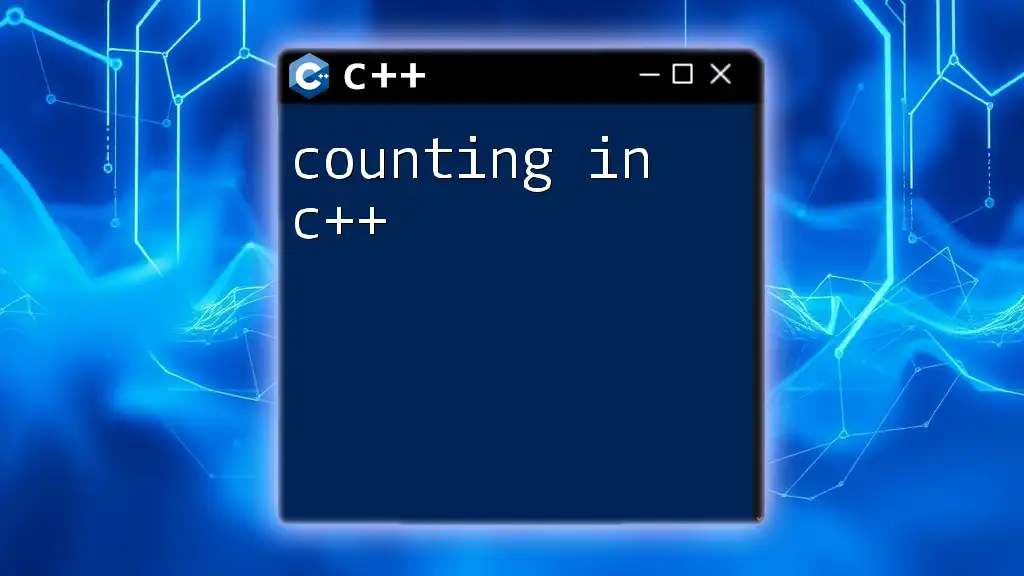
Call to Action
Share your experiences with object slicing in the comments below. Have you encountered any challenges or solutions related to this topic? Your insights could benefit others looking to navigate the world of C++ effectively.
Further Reading
Explore additional articles that delve deeper into advanced C++ concepts and object-oriented programming to expand your knowledge and expertise.