In C++, you can create a new line in your output by using the `std::endl` manipulator or the newline character `\n`.
Here's a code snippet demonstrating both methods:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Using std::endl
std::cout << "Hello, again!" << '\n'; // Using newline character
return 0;
}
What is an End Line in C++?
End lines in C++ are crucial for formatting the output displayed in the console. An end line serves as a delimiter that signals the conclusion of one line of text and the beginning of another. Proper use of end lines enhances the readability of output, making it easier for both programmers and users to understand the information presented.
Understanding how to control output formatting and line separations is essential for writing clean and structured code in C++. Different methods exist to achieve the desired end line effects, and recognizing their respective benefits can significantly impact your coding practices.
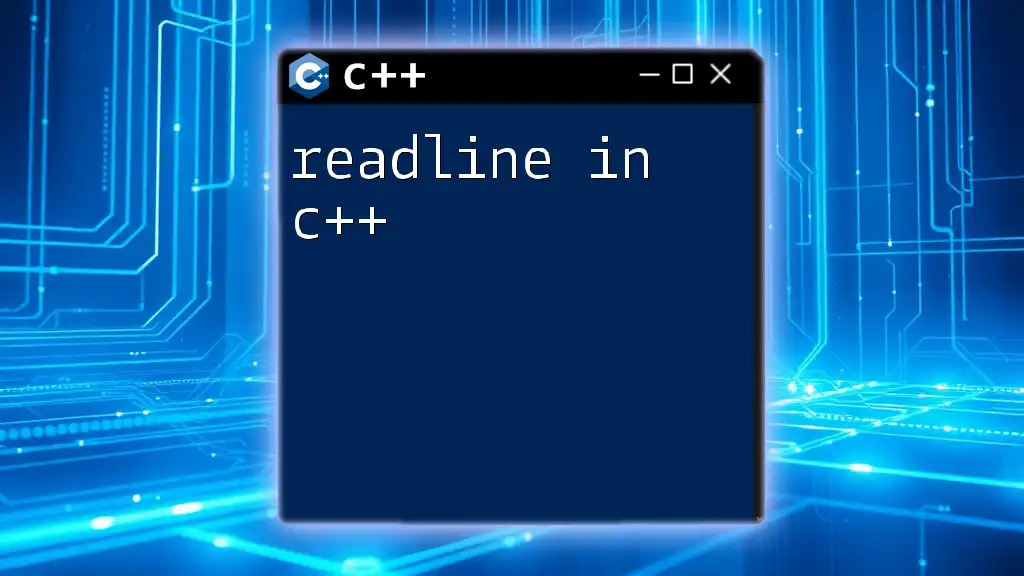
Different Methods to Create End Lines in C++
Using the `std::endl` Manipulator
What is `std::endl`?
`std::endl` is an output manipulator that not only inserts a new line in the output but also flushes the output buffer. This means any data that may be temporarily stored in the buffer is immediately sent to the console.
Syntax and Example
Consider the following code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
}
In this example, `std::endl` is used to create a new line after printing "Hello, World!" to the console. The output appears as:
Hello, World!
The added flush ensures that any buffered output is displayed right away, which can be especially useful in real-time applications.
Using the Newline Character `\n`
Understanding the Newline Character
Another way to create an end line in C++ is by using the newline character `\n`. This character acts as a simple line separator without flushing the output buffer, allowing for more performance-efficient coding in situations where immediate display of output is not required.
Syntax and Example
Here’s an example using the newline character:
#include <iostream>
int main() {
std::cout << "Hello, World!\n";
}
This snippet results in the same output:
Hello, World!
While both `std::endl` and `\n` achieve similar visual outcomes, understanding their differences is crucial for effective coding practices.
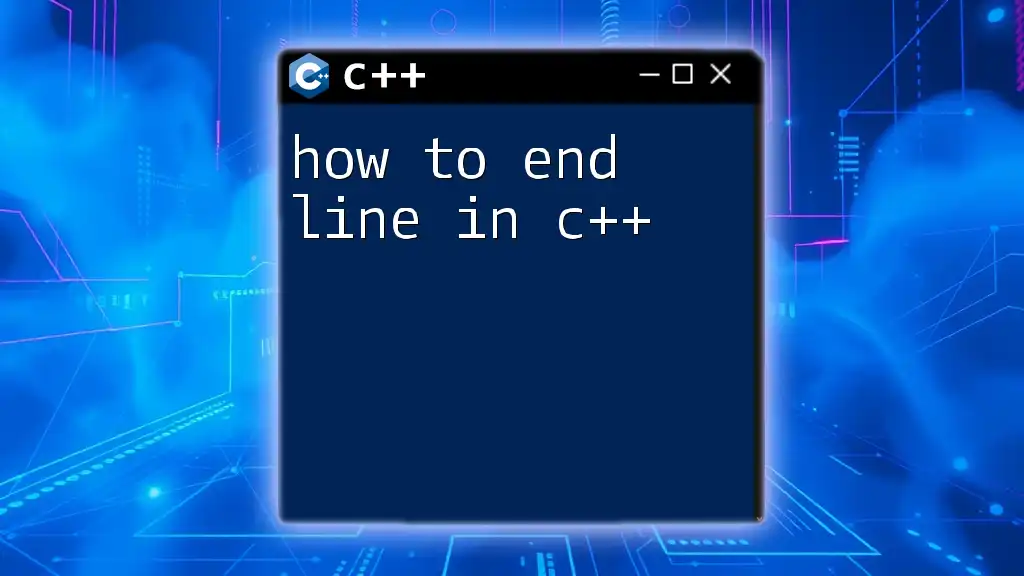
Comparing `std::endl` and `\n`
Performance Considerations
A significant difference between `std::endl` and `\n` lies in how they handle buffer flushing. When using `std::endl`, the output buffer is flushed immediately, which can slow down your program if called excessively. In contrast, `\n` adds a new line without flushing, making it a more efficient choice in loops or high-frequency output scenarios.
Use Cases
-
When to Use `std::endl`
Use `std::endl` in situations where it is critical to ensure that the output is displayed without delay—such as in logging status updates or debugging information where real-time feedback is essential. -
When to Use `\n`
Opt for `\n` in performance-critical applications or loops where continual output is required, and immediate flushing is unnecessary.
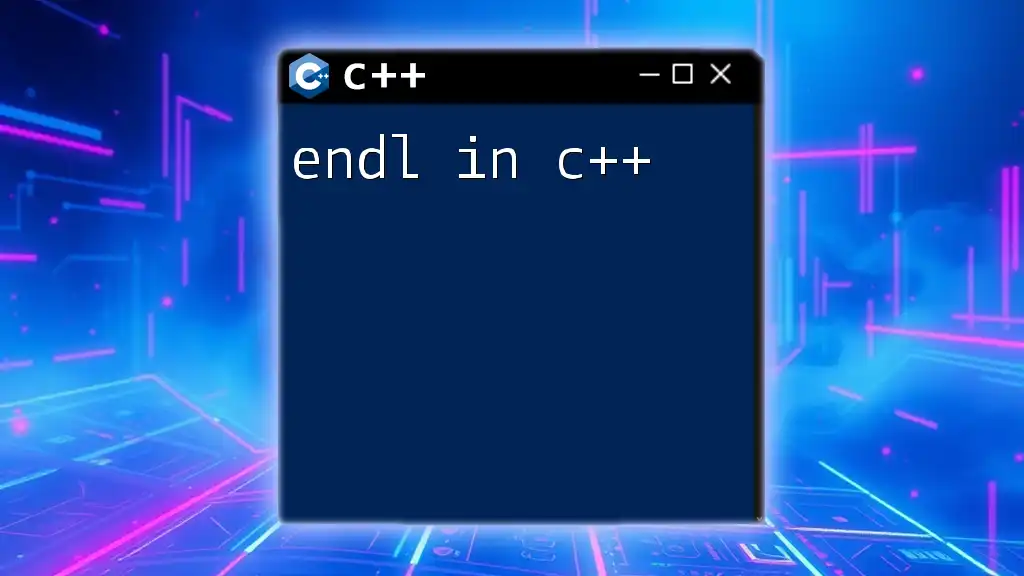
Customizing Output with Other Manipulators
Using `std::flush`
Explanation of `std::flush`
`std::flush` is another output manipulator that flushes the output buffer without adding a new line. It is useful when you want to ensure that the output appears immediately but do not wish to start a new line.
Syntax and Example
Here’s how you can use `std::flush`:
#include <iostream>
int main() {
std::cout << "Processing...\n";
std::cout << std::flush; // Manually flushing the output
}
This code will output "Processing..." followed by a newline, ensuring that the message is visible in real-time without adding an extra line after it.
Combining Output Manipulators
You can also combine multiple output manipulators to create complex output sequences and manage formatting efficiently:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl << "This is a second line." << std::flush;
}
In this example, the message is displayed with both a new line and an immediate flush, ensuring proper formatting and visibility.
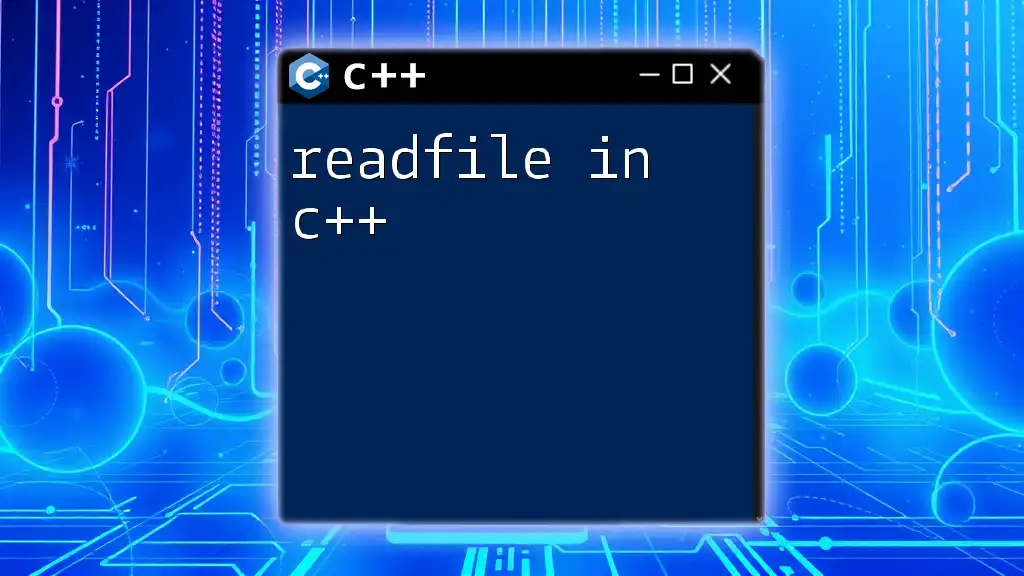
Best Practices for Using End Lines in C++
When programming in C++, finding the balance between readability and performance is paramount. While clarity in output formatting can improve user experience, aggressive use of `std::endl` can degrade performance.
Encouraging consistency in how you handle end lines across your code can enhance maintainability. Use output methods appropriate to the context, ensuring that your code remains both efficient and clear. For instance, if the output is primarily for debugging, `std::endl` may be suitable in limited contexts, while standard application outputs could rely predominantly on `\n`.
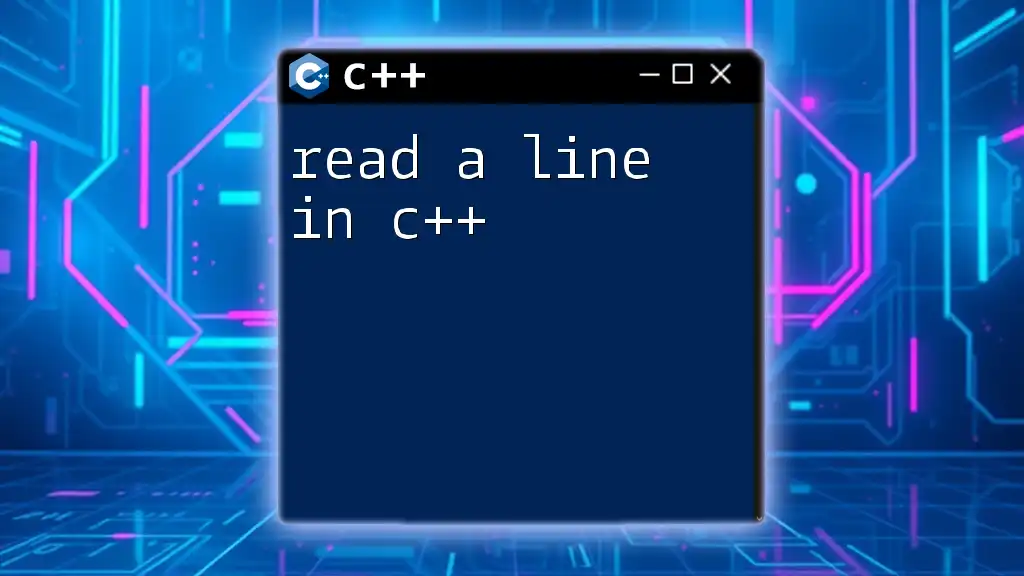
Conclusion
End lines in C++ may seem like a minor detail, but they play a significant role in output formatting and overall program clarity. Understanding the differences between `std::endl` and `\n`, as well as when to use other manipulators like `std::flush`, can greatly improve the effectiveness of your C++ programming.
By practicing and experimenting with these methods, you can become adept at utilizing end lines to enhance both the functionality and readability of your code.