String handling in C++ involves manipulating sequences of characters using the `std::string` class, which provides various functions for string operations such as concatenation, comparison, and substring extraction.
Here’s a simple code snippet demonstrating basic string handling:
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "World!";
std::string result = str1 + str2; // Concatenation
std::cout << result << std::endl; // Output: Hello, World!
return 0;
}
Understanding Strings in C++
In C++, string handling is a fundamental aspect of programming that deals with the manipulation and processing of sequences of characters. Understanding how to effectively handle strings is crucial for any developer. Strings can be broadly categorized into two types in C++: C-style strings and the `std::string` class.
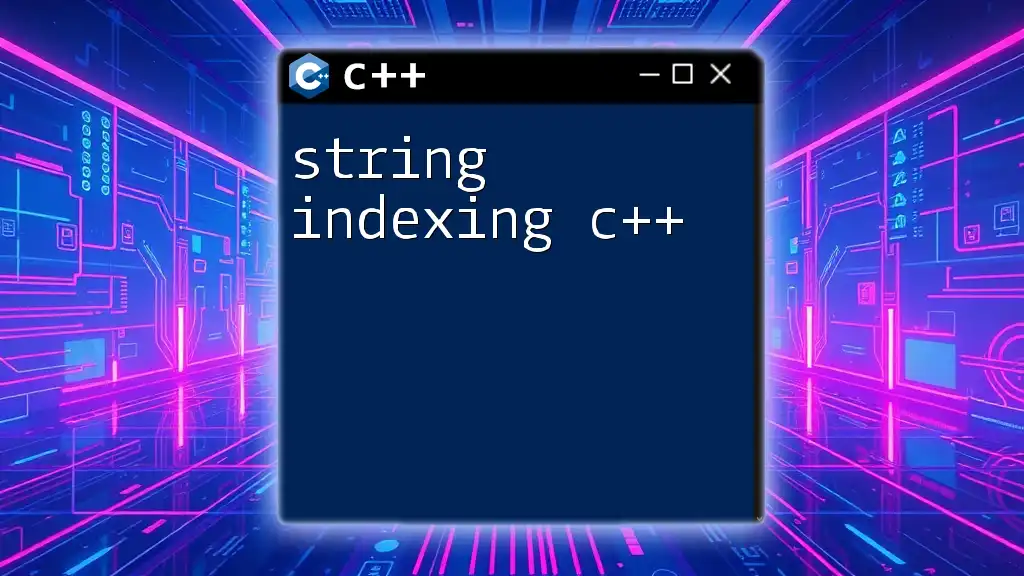
C-style Strings
Basics of C-style Strings
C-style strings are fundamentally arrays of characters terminated by a null character (`'\0'`). Unlike modern C++ strings, they lack built-in safety features for memory management, which can lead to potential issues if not handled correctly.
Here’s a simple way to declare and initialize a C-style string:
char str1[] = "Hello, World!";
char str2[20]; // declaration for usage
Common Operations
String Length
To determine the length of a C-style string, you can utilize the `strlen()` function from the `<cstring>` library. This function returns the number of characters in the string, not including the null terminator.
Example:
#include <cstring>
size_t length = strlen(str1);
String Copying
To copy one C-style string to another, the `strcpy()` function can be used. However, it's essential to ensure that the destination has enough space to accommodate the content being copied to avoid buffer overflows.
Example:
strcpy(str2, str1); // Safe operation is required
String Concatenation
Combining two C-style strings can be accomplished with the `strcat()` function. Ensure that the destination string can hold the original content and the additional data being appended.
Example:
strcat(str1, " How are you?");
Common Pitfalls
One of the significant challenges with C-style strings is buffer overflows, which may lead to undefined behavior if exceeded. To prevent this, consider using the safer `strncpy()` function, which specifies the maximum number of characters to copy.
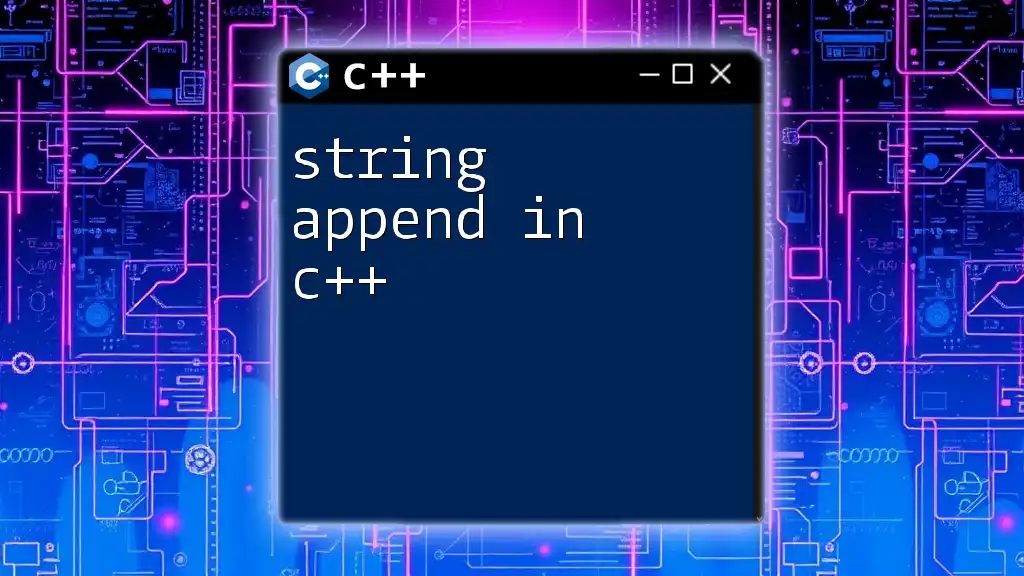
C++ std::string Class
Introduction to std::string
The `std::string` class is part of the C++ Standard Library and offers a more intuitive and flexible way of handling strings. It automatically manages memory and provides a variety of member functions for string manipulation, making it easier and safer to use.
Declaring and Initializing std::string
Creating strings using `std::string` is straightforward. You can initialize it with a string literal directly, providing clarity and ease of use.
Example:
std::string str = "Hello, World!";
Common Operations
Accessing Characters
You can access characters in an `std::string` using array syntax or the `at()` method. The `at()` method includes bounds checking, which can prevent runtime errors.
Example:
char firstChar = str[0]; // H
char secondChar = str.at(1); // e
String Length
To get the number of characters in an `std::string`, use the `.length()` or `.size()` method, both of which yield the same result.
Example:
size_t length = str.length();
String Concatenation
Combining `std::string` objects is as simple as using the `+` operator, simplifying the process of assembling strings.
Example:
std::string newStr = str + " How are you?";
Special Features of std::string
Substrings
The `std::string` class allows for easy extraction of substrings with the `.substr()` method, where you specify the starting position and the length of the substring.
Example:
std::string subStr = str.substr(7, 5); // World
String Search
Finding characters or substrings within an `std::string` is efficiently done using the `.find()` method, which returns its position or `std::string::npos` if not found.
Example:
size_t found = str.find("World");
String Replacement
To modify the contents of a string, use the `.replace()` method, which allows you to replace a portion of the string with another string.
Example:
str.replace(7, 5, "C++"); // Replaces 'World' with 'C++'
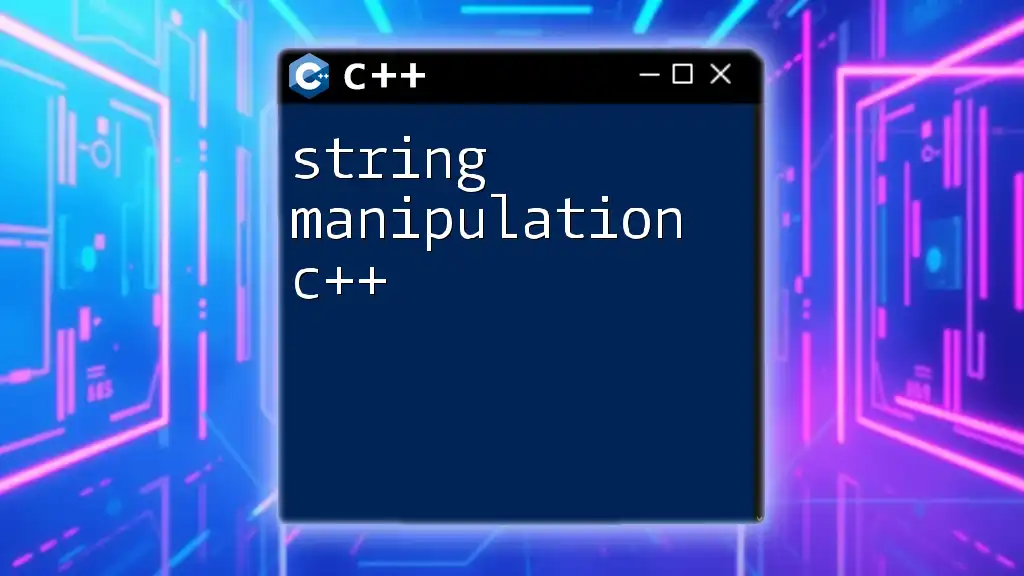
Input and Output with Strings
Reading Strings
For user input, the `std::cin` object is commonly used in conjunction with the `std::getline()` function to capture entire lines, allowing for spaces to be included.
Example:
std::string userInput;
std::getline(std::cin, userInput);
Displaying Strings
Outputting strings to the console is easily accomplished using the `std::cout` object.
Example:
std::cout << "You entered: " << userInput << std::endl;
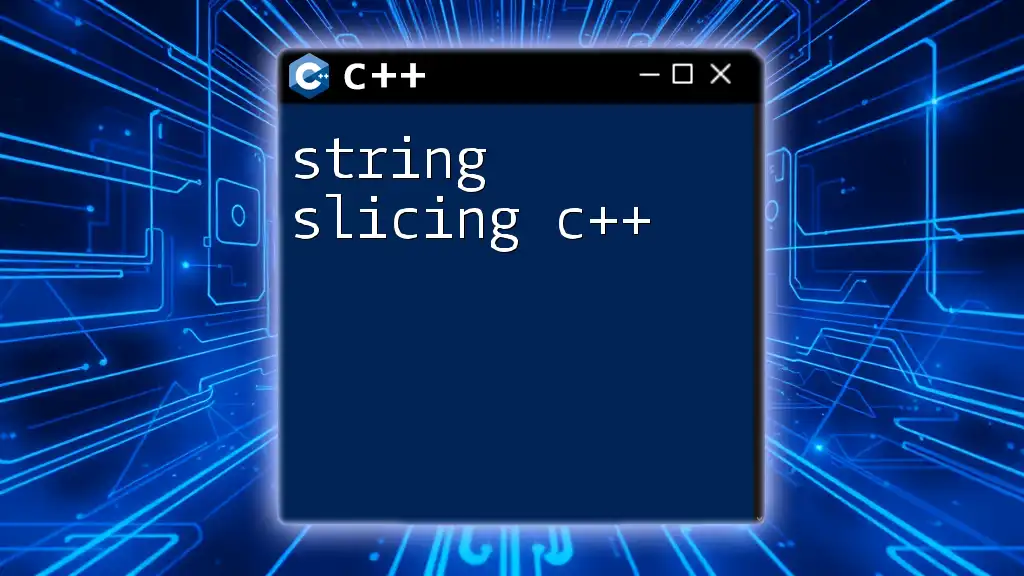
Advanced String Handling Techniques
String Streams
`std::stringstream` objects provide a way to combine strings and numbers seamlessly. String streams are incredibly useful for formatting output or building strings from various data types.
Example:
std::stringstream ss;
ss << "Number: " << 42;
std::string combined = ss.str();
Converting Between Types
You can convert strings to numeric types and vice versa using functions like `std::stoi`, `std::stof`, etc., which are part of the C++ Standard Library. This is particularly useful for extracting numerical input from user strings.
Example:
int number = std::stoi("42");
Multilingual Support
For applications requiring multilingual support, you can utilize wide strings (`std::wstring`). These strings can hold Unicode characters, allowing for broader character representation.
Example:
std::wstring wstr = L"Hello, 世界!";
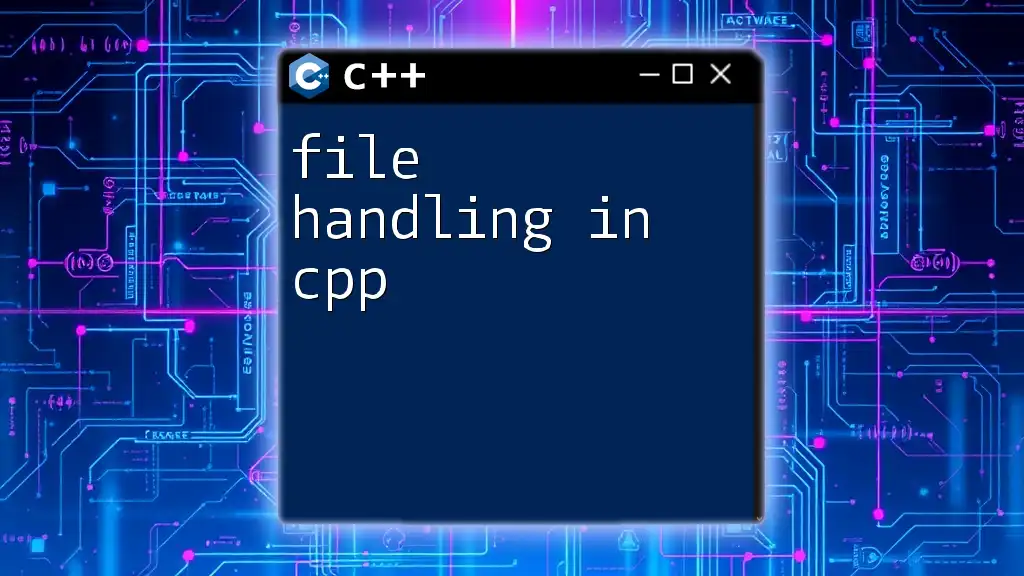
Conclusion
Effective string handling in C++ is vital for any programmer. Mastering both C-style strings and `std::string` will enable you to handle text data efficiently and safely. Understanding the strengths and weaknesses of each approach allows you to choose the best method for your specific needs. As you practice with these concepts, you will become proficient in a skill that is crucial in software development.