In C++, a `char` is a single character type, while a `string` is a sequence of characters, and you can easily convert between the two using functions like `std::string` and `c_str()`.
Here's a simple code snippet demonstrating the conversion of a `char` to a `string` and back:
#include <iostream>
#include <string>
int main() {
char c = 'A'; // single character
std::string s(1, c); // convert char to string
std::cout << "Character: " << c << ", String: " << s << std::endl;
char newChar = s[0]; // convert string back to char
std::cout << "Converted back to Character: " << newChar << std::endl;
return 0;
}
Understanding Characters in C++
Definition of Character Data Type
In C++, the `char` data type is used to store a single character. This includes letters, digits, punctuation, and special symbols. The syntax for declaring a char variable is straightforward, for instance:
char letter = 'A';
A char occupies one byte of memory, which can represent 256 different values (ranging from -128 to 127 in signed form). This allows you to create variables capable of holding characters and perform comparisons, arithmetic, or manipulations accordingly.
Common Uses of Characters
Characters are typically used for:
- Storing single letters or symbols in various applications, such as games or user interfaces.
- Handling user input, where each character represents an individual key pressed.
For example, you may read a character input and decide if it is an alphabet or a digit for some validation.

Introduction to Strings in C++
Definition of String Data Type
In C++, a string can be defined in two main ways: using C-style strings or the `std::string` class from the C++ Standard Library.
Using C-Style Strings
C-style strings are arrays of characters terminated by a null character (\0). The syntax for declaring and initializing a C-style string looks like this:
char str1[] = "Hello, World!";
This creates an array of characters with the elements `'H'`, `'e'`, `'l'`, `'l'`, `'o'`, `','`, `' '`, `'W'`, `'o'`, `'r'`, `'l'`, `'d'`, `'!'`, and concludes with `\0` to mark the end of the string.
Important Functions Associated with C-Style Strings
Commonly used functions for manipulation include:
- `strlen()`: To get the length of the string.
- `strcpy()`: To copy one string to another.
- `strcat()`: To concatenate two strings together.
Introduction to the Standard Library String Class
The `std::string` class provides a more powerful and flexible way to handle strings in C++. It supports a variety of operations and simplifies memory management.
To declare a `std::string`, you can write:
#include <string>
std::string str2 = "Welcome to C++!";
Advantages over C-style strings include automatic memory management, dynamic resizing, and built-in methods for manipulation.
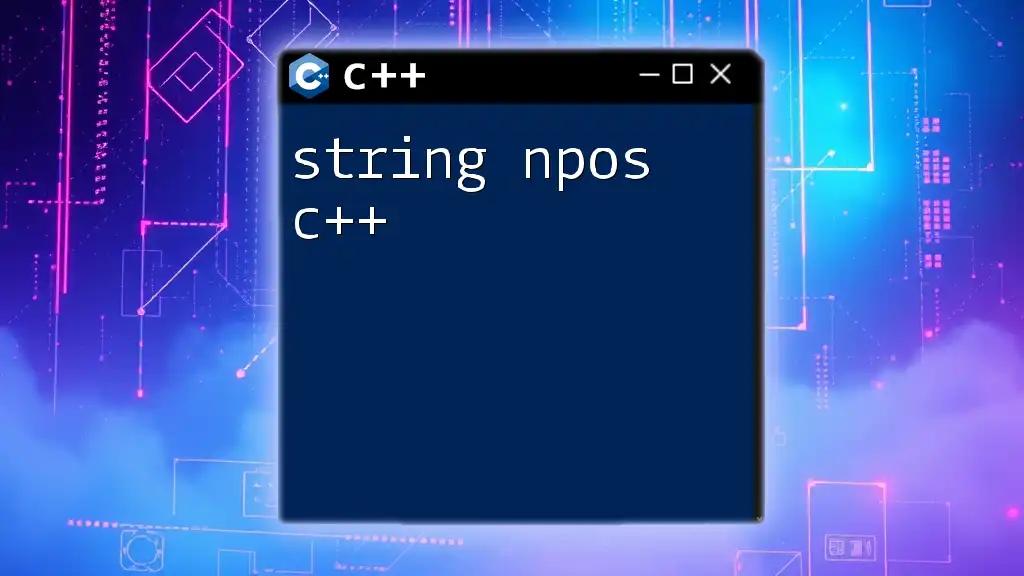
String Manipulations in C++
Basic String Operations
Concatenation of Strings
Joining strings together, also known as concatenation, can be easily performed using the `+` operator.
std::string hello = "Hello, ";
std::string world = "World!";
std::string greeting = hello + world; // Result: "Hello, World!"
Accessing Characters in a String
You can access individual characters in a string using indexing:
char firstChar = greeting[0]; // H
char secondChar = greeting.at(1); // e
The `.at()` method is safer as it checks bounds and will throw an exception if you access an out-of-bounds index.
Finding Substrings
To find the occurrence of a substring within a string, use the `.find()` method:
size_t position = greeting.find("World"); // position will be 7
If the substring is not found, it returns `std::string::npos`.
Changing Characters
You can easily modify characters in a string using indexing:
greeting[0] = 'h'; // Now greeting is "hello, World!"
Advanced String Functions
String Length
To find the length of a string, you can use both `.length()` and `.size()`, as they behave the same:
size_t length = greeting.length(); // Returns 13
String Comparison
C++ allows string comparisons out of the box, meaning you can use standard comparison operators:
if (str1 == str2) {
// Do something
}
String Iterators
Strings can be traversed using iterators, similar to other STL containers:
for (auto it = str2.begin(); it != str2.end(); ++it) {
// Process each character
}
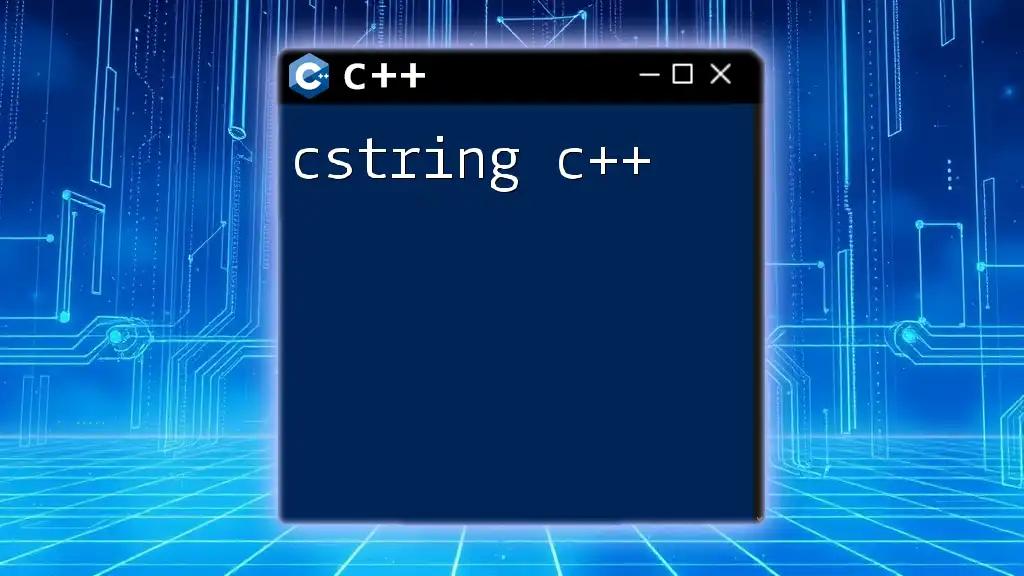
Character and String Conversion
From Char to String
You can convert a char to std::string easily:
char c = 'A';
std::string s(1, c); // Result: "A"
From String to Char
To extract a single character from a string, you can do the following:
std::string str = "Hello";
char c = str[0]; // Result: 'H'
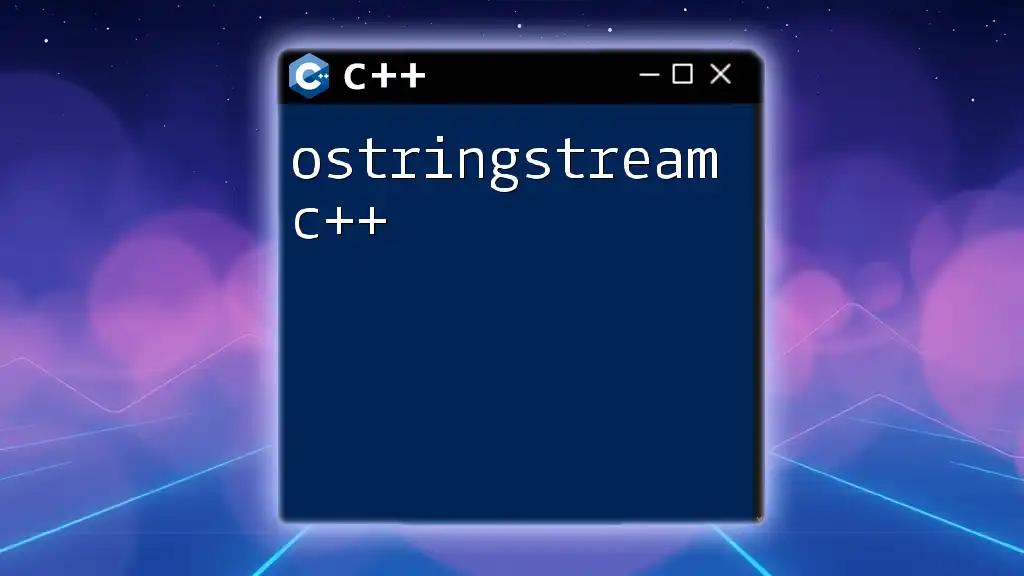
Conclusion
Understanding how to work with string char c++ is essential for effective programming in C++. By knowing the distinctions and manipulations available for characters and strings, you empower yourself to handle text, user input, and data more efficiently. Mastering these concepts will pave the way for your journey through advanced C++ programming.
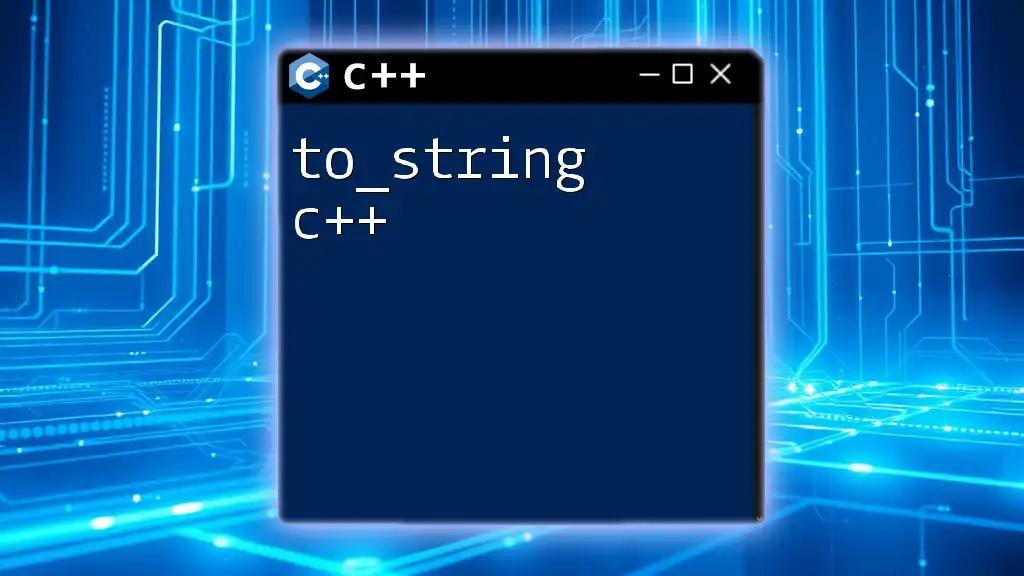
Further Reading and Resources
To deepen your understanding, consider reading the official C++ documentation and exploring recommended books. Engaging in community forums or online courses can also enhance your learning experience and help you master the intricacies of strings and characters in C++.