In C++, `string::npos` is a constant that represents the largest possible value for a position in a string, commonly used to indicate that a search operation (like `find`) did not locate the specified substring.
Here's a code snippet demonstrating its usage:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
size_t pos = str.find("example");
if (pos == std::string::npos) {
std::cout << "Substring not found!" << std::endl;
} else {
std::cout << "Substring found at position: " << pos << std::endl;
}
return 0;
}
Understanding C++ Strings
What is a C++ String?
In C++, strings are represented by the `std::string` class, which is part of the Standard Template Library (STL). Unlike C-style strings that utilize character arrays, `std::string` offers a more powerful and flexible way to handle sequences of characters. Some key characteristics of `std::string` include:
- Dynamic Size: The length of a `std::string` can change dynamically during program execution, allowing you to easily add or remove characters.
- Rich Functionality: The `std::string` class provides a wide array of built-in member functions for manipulation, searching, and comparing strings.
- Memory Management: The C++ standard library handles the memory allocation and deallocation for `std::string` instances automatically, which minimizes potential memory leaks.
How Strings are Managed in C++
C++ manages strings using dynamically allocated memory. The `std::string` class internally keeps track of its own size and capacity, allowing for efficient resizing when necessary. It supports various operations like concatenation, substring extraction, and searching through its many member functions.

Delving into `npos`
What is `npos` in C++?
The term `npos` is used to signify a non-position within a string, specifically referring to a condition where a substring or character was not found in the target string. In C++, `npos` is defined as a constant value of type `size_t` which is available through the `std::string` class as `std::string::npos`.
This value is particularly useful to indicate failure in string operations without throwing an exception. The exact value of `npos` is one that is guaranteed to be larger than any valid position in the string, ensuring it acts as a definitive marker for the absence of a match.
Why is `npos` Important?
The primary significance of `npos` lies in its role in error handling and string searching. When performing operations like `find()`, a return value of `npos` indicates that the target string could not locate the specified substring. This capability is crucial for safely navigating strings without risking out-of-bound errors or undefined behavior.
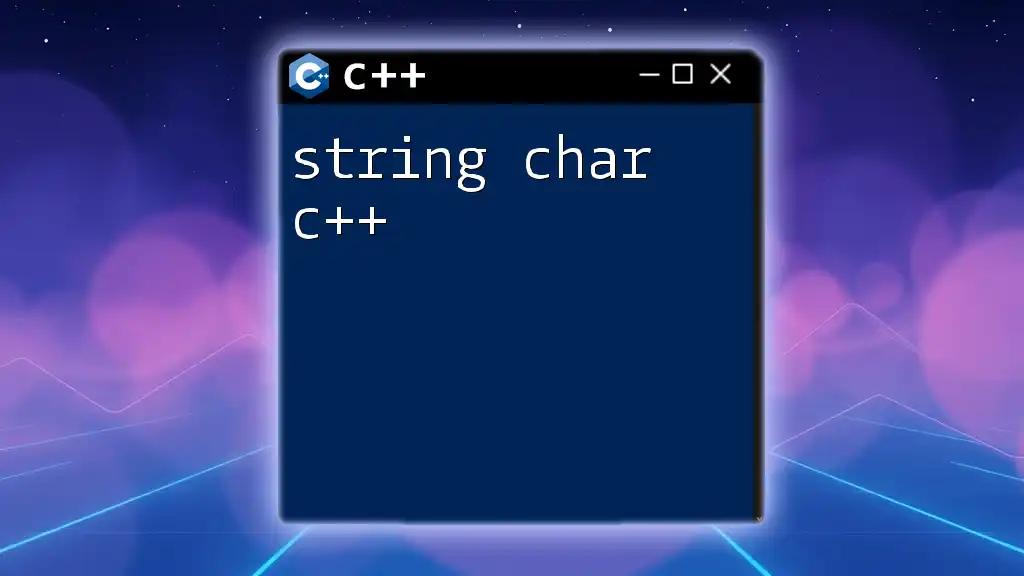
Using `npos` in C++
Basic Syntax of Using `npos`
The `string::npos` is often used in conditional checks to verify whether a substring search was successful. Here’s a simple example:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, C++!";
size_t position = str.find("World");
if (position == std::string::npos) {
std::cout << "Substring not found!" << std::endl;
} else {
std::cout << "Position found at: " << position << std::endl;
}
return 0;
}
In this code snippet, we attempt to find the substring "World" in the string "Hello, C++!". Since it doesn’t exist, `find()` returns `string::npos`, and we handle that case gracefully.
Common Functions Utilizing `npos`
- `find()` Function
The `find()` function is one of the most commonly used string functions that returns the position of a substring within another string. If the substring isn’t found, it returns `npos`. Here’s how it works:
std::string text = "Searching for something";
size_t pos = text.find("something");
if (pos != std::string::npos) {
std::cout << "Found at position: " << pos << std::endl;
}
-
`rfind()` Function
Similar to `find()`, the `rfind()` function searches for a substring but starts from the end of the string. If it’s not found, it also returns `npos`. -
`find_first_of()` and `find_last_of()`
These functions can be used to find the first or last occurrence of any character from a set. If nothing is found, a return value of `npos` signals that too.
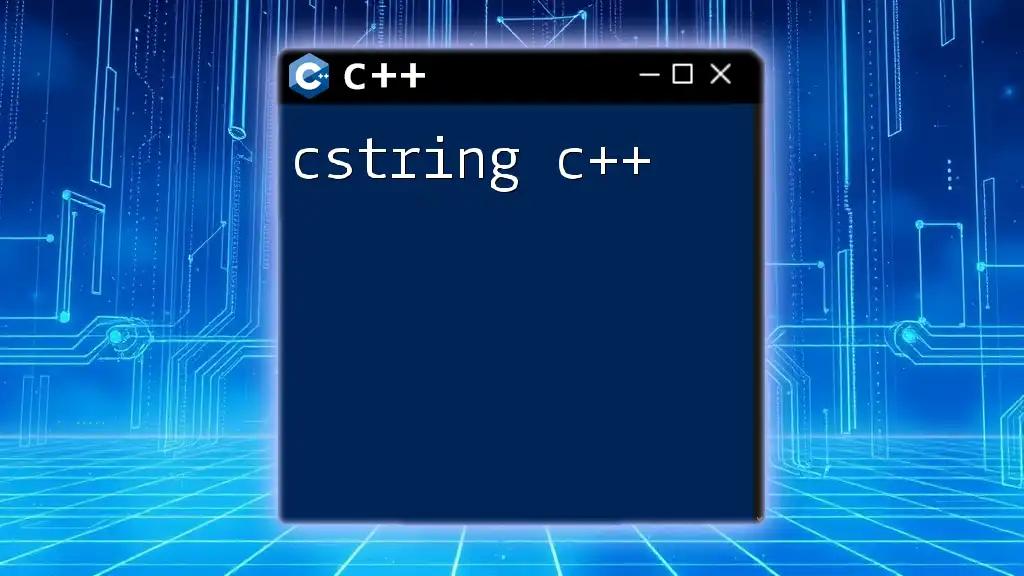
Advanced Use Cases
Using `npos` in Loops
In more complex scenarios, you may want to utilize `npos` in iteration or looping constructs. For instance, if you need to find all occurrences of a specific character or substring, you could do so with a loop:
std::string str = "abcabcabc";
size_t pos = str.find("a");
while (pos != std::string::npos) {
std::cout << "Found 'a' at position: " << pos << std::endl;
pos = str.find("a", pos + 1);
}
This loop continues searching for the character "a" until no more instances are found.
Combining `npos` with Other String Functions
You can also combine `npos` with other string functions for seamless manipulation. For instance, using `substr()` alongside `npos` can help slice strings effectively. Here’s an example:
std::string data = "Example string for testing npos.";
size_t pos = data.find("string");
if (pos != std::string::npos) {
std::string substring = data.substr(pos);
std::cout << "Substring: " << substring << std::endl;
}
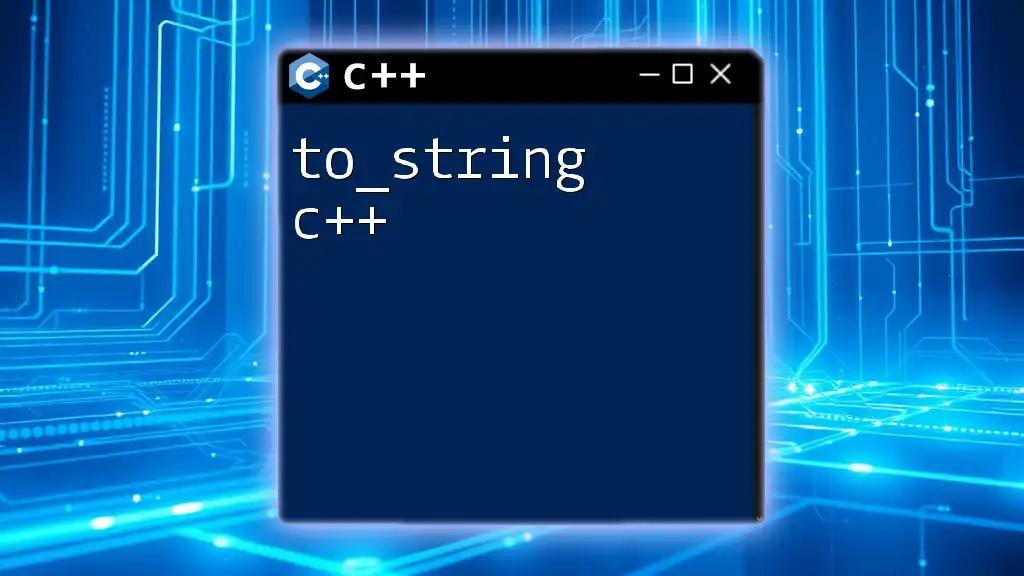
Best Practices
When to Use `npos`
Using `npos` is a best practice whenever you need to check for the absence of a substring in C++. It clarifies your intention and avoids potential errors stemming from incorrect index handling.
Common Pitfalls to Avoid
- Ignoring Return Values: Always check against `npos` when using functions like `find()` and `rfind()`. Not doing so can lead to unexpected behaviors.
- Mismatched Types: Ensure you are comparing results against `std::string::npos` without mismatching types, to avoid implicit conversions that might introduce bugs.
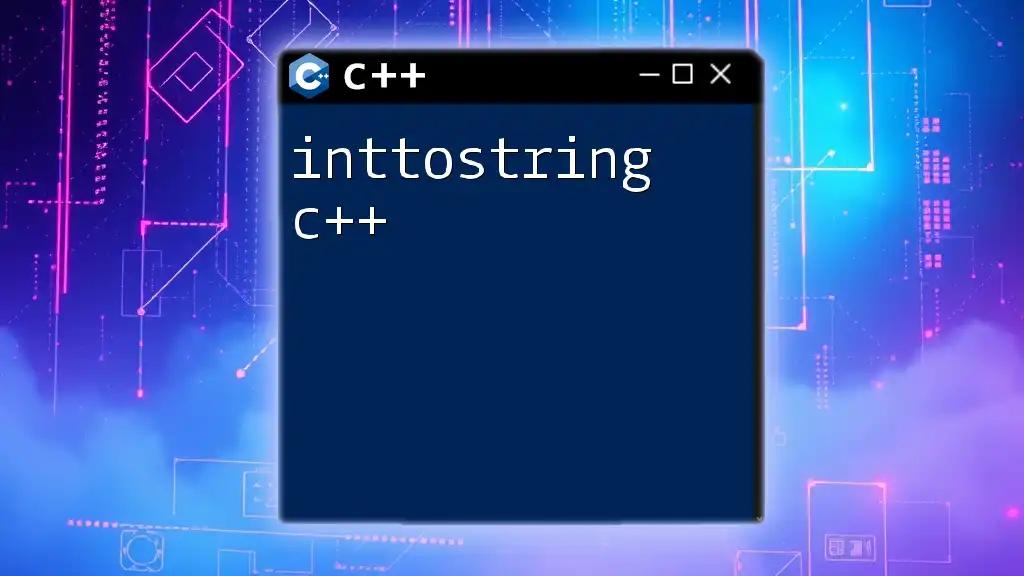
Conclusion
In summary, understanding `string::npos` in C++ is essential for effective string manipulation. This concept allows developers to gracefully handle occurrences of substrings, manage errors, and write safer code. By practicing its use and exploring related string functions, you can enhance your skills and confidence in working with strings in C++.

Further Resources
To deepen your understanding of C++ strings and the broader Standard Template Library, consider exploring additional books, online tutorials, and community forums. Engaging with these resources can greatly assist your journey towards mastering C++.