In C++, to find the index of a substring within a string, you can use the `find` method of the `std::string` class, which returns the position of the first occurrence of the substring, or `std::string::npos` if the substring is not found.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::string sub = "world";
size_t index = str.find(sub);
if (index != std::string::npos) {
std::cout << "Index of substring: " << index << std::endl; // Output: 7
} else {
std::cout << "Substring not found!" << std::endl;
}
return 0;
}
Understanding the Concept of Indexing in C++
Indexing is a fundamental concept in programming that allows you to access individual elements within a data structure, such as an array or string. In C++, both C-style strings (character arrays) and C++ strings (`std::string`) utilize indexing. Each character in a string can be accessed by its position (also known as its index), which starts at zero. For instance, in the string "Hello", 'H' is at index 0, 'e' at index 1, and so on.
Here’s a quick example:
#include <iostream>
#include <string>
int main() {
std::string greeting = "Hello";
std::cout << "First character: " << greeting[0] << std::endl; // Output: H
return 0;
}
Understanding indexing is crucial when implementing search functions like "IndexOf". However, it's important to note that C++ does not have a built-in `IndexOf` function, unlike some other languages like C# or Java.
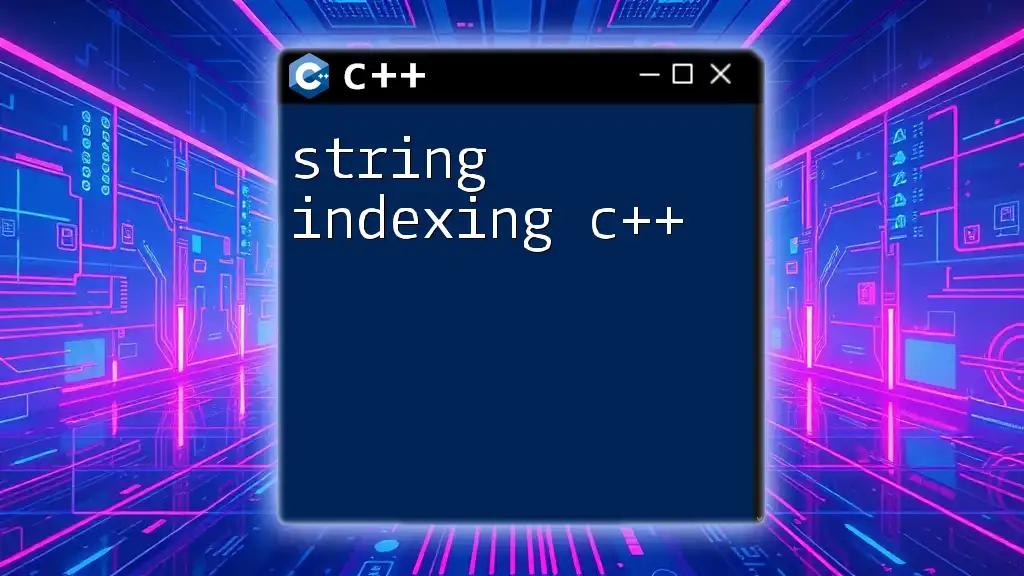
The Limitations of IndexOf in C++
Despite its widespread utility, C++ lacks a direct `IndexOf` method within the standard libraries for the `std::string` class. This absence can lead to confusion, particularly for those transitioning from languages with built-in support for such functionality.
Common misconceptions include assuming that there’s a ready-to-use function like `str.IndexOf()` available in C++. Therefore, it’s essential to recognize that you will need to create your own functionality to achieve similar results.
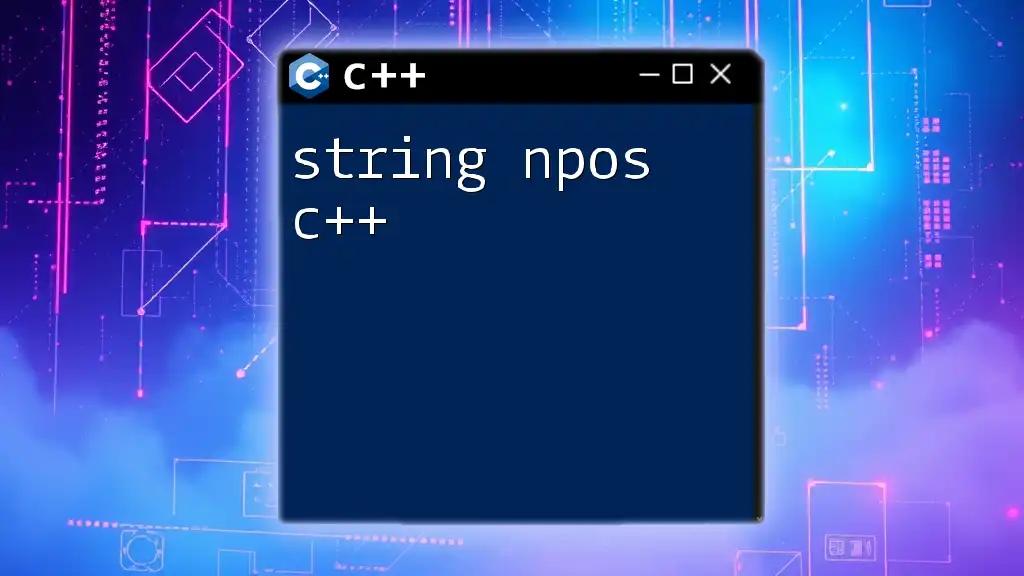
Implementing IndexOf-Like Functionality
Creating Your Own IndexOf Function
To implement a basic `IndexOf` function, you need to loop through the characters of the string and compare each one to the target character. If a match is found, return its index.
Here’s a simple implementation:
#include <iostream>
#include <string>
int indexOf(const std::string& str, char target) {
for (int i = 0; i < str.length(); i++) {
if (str[i] == target) {
return i; // Return index where character is found
}
}
return -1; // Indicate not found
}
In this code, we iterate over each character of the input string and check for a match with the `target` character. If found, we return its index; otherwise, we return -1 to signify that the character is not present.
Enhancing the IndexOf Function
To make our `IndexOf` function more versatile, we can expand it to search for a substring rather than just a single character. The C++ Standard Library provides the `find` method, which simplifies this task significantly.
Here’s the enhanced version:
int indexOf(const std::string& str, const std::string& target) {
size_t pos = str.find(target);
return pos != std::string::npos ? static_cast<int>(pos) : -1;
}
In this function, we leverage `std::string::find()`, which returns the starting index of the first occurrence of the target substring. If it’s not found, it returns `std::string::npos`, allowing us to determine whether the search was successful easily.
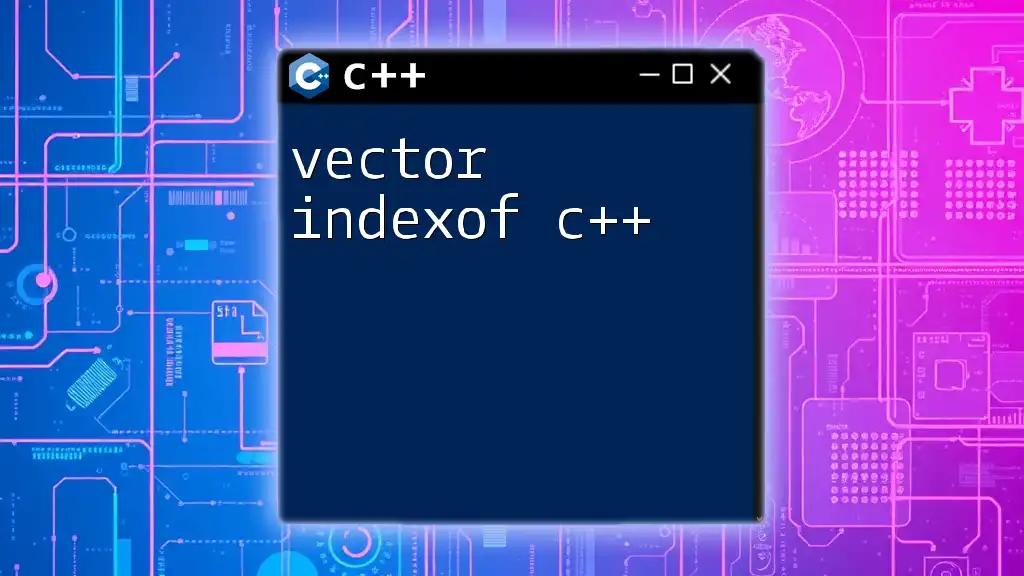
Practical Use-Cases for IndexOf in C++
Searching Through User Input
One practical application for `IndexOf` functionality is to search for a specific character or substring in user-provided text. This is useful in scenarios like filtering or validating input data.
Consider the following example, where we prompt the user for a string and a character to search for:
std::string userInput;
std::cout << "Enter a string: ";
std::getline(std::cin, userInput);
char searchChar;
std::cout << "Enter character to search for: ";
std::cin >> searchChar;
int index = indexOf(userInput, searchChar);
if (index != -1) {
std::cout << "Character found at index: " << index << std::endl;
} else {
std::cout << "Character not found." << std::endl;
}
This code snippet not only demonstrates the use of our `indexOf` function but also emphasizes the interactive nature of input processing in C++.
Text Processing Applications
Another significant use-case for `IndexOf` is in text processing. For example, you might need to analyze a document looking for specific keywords or phrases. By implementing an `IndexOf` function, you could quickly pinpoint the location of terms, facilitating a range of applications from search engines to text editors.
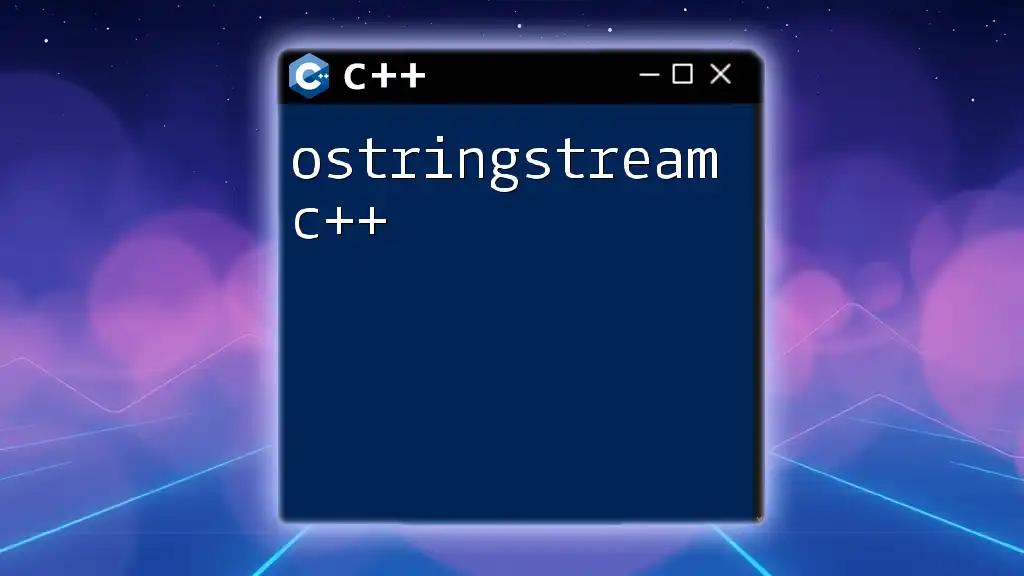
Best Practices for Using IndexOf in C++
Efficient Searching in Large Strings
When searching through large strings, efficiency becomes paramount. While the methods discussed work well for small to medium-sized strings, large text processing may require specialized algorithms that offer better performance, such as the Knuth-Morris-Pratt or Boyer-Moore algorithms.
Error Handling
Robustness should also be a priority in your implementation. Always consider edge cases, such as searching for an empty string or characters in invalid contexts. Using exception handling can help ensure that your program does not crash under unexpected conditions.
try {
int index = indexOf(userInput, searchChar);
// Handle index appropriately
} catch (const std::exception& e) {
std::cerr << "Error occurred: " << e.what() << std::endl;
}
This defensive programming approach will enhance the reliability of your string manipulation functions.

Common Pitfalls to Avoid with IndexOf in C++
While working with an `IndexOf` implementation, watch out for common pitfalls. Off-by-one errors can easily occur, particularly if you are not consistent in how you utilize or return indices.
Also, be mindful of case sensitivity. The default string operations in C++ will distinguish between uppercase and lowercase letters. To implement case-insensitive searching, consider converting the string and target to a common case before comparing.

Conclusion
The ability to find the index of characters and substrings within strings is vital for any C++ programmer. While the language does not provide an out-of-the-box `IndexOf` function, the implementation techniques outlined in this guide equip you to handle such tasks effectively.
Mastering string manipulation, including functions like `IndexOf`, is fundamental not only for everyday programming tasks but also for more complex applications in software development. Embrace these concepts, practice coding, and explore the versatility of C++ string operations!
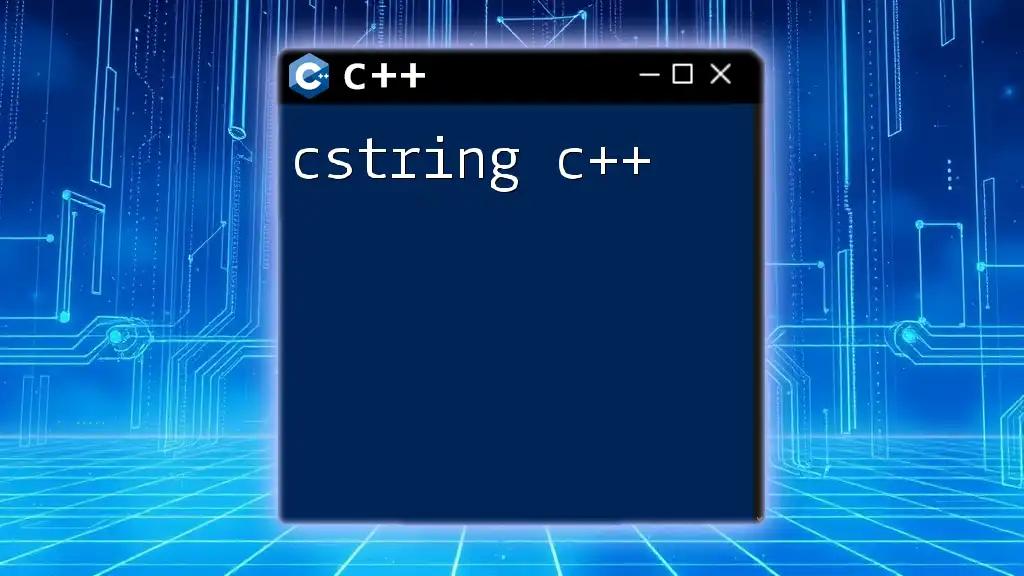
Additional Resources
To strengthen your knowledge, consider exploring further reading materials, tutorials, and online compilers where you can experiment with string manipulation hands-on. With practice, you'll become proficient in handling strings and implementing your own helpful functions!