In C++, to find the index of a specific element in a vector, you can use the `std::find` algorithm from the `<algorithm>` header, which returns an iterator that can be converted to an index.
Here's a concise code snippet demonstrating this:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {10, 20, 30, 40, 50};
int value = 30;
auto it = std::find(vec.begin(), vec.end(), value);
int index = (it != vec.end()) ? std::distance(vec.begin(), it) : -1;
std::cout << "Index of " << value << " is: " << index << std::endl;
return 0;
}
Understanding C++ Vectors
What are Vectors?
Vectors in C++ are dynamic arrays provided by the Standard Template Library (STL). They can grow and shrink in size dynamically, offering flexibility that traditional arrays do not provide. Vectors can store elements of the same data type and are capable of managing memory automatically.
Compared to arrays, vectors offer several advantages:
- Dynamic Size: Unlike fixed-size arrays, vectors can expand or contract as you add or remove elements.
- Automatic Memory Management: The vector handles its own memory, reducing the risk of memory leaks.
- Useful Member Functions: Vectors come with a plethora of built-in functions that simplify array operations, such as `push_back()`, `pop_back()`, and `insert()`.
Basic Operations on Vectors
Vectors support a variety of operations, making them a versatile choice in C++ programming.
Adding Elements: To add an element to a vector, the `push_back()` function is commonly used.
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec;
vec.push_back(1);
vec.push_back(2);
vec.push_back(3);
for (const int &num : vec) {
std::cout << num << " "; // Output: 1 2 3
}
return 0;
}
Accessing Elements: You can access elements in a vector using the indexing operator `[]`.
std::cout << vec[0]; // Output: 1
Removing Elements: Elements can be removed from the end of a vector using `pop_back()`, or you can remove specific elements with the `erase()` member function.
vec.pop_back(); // Removes the last element
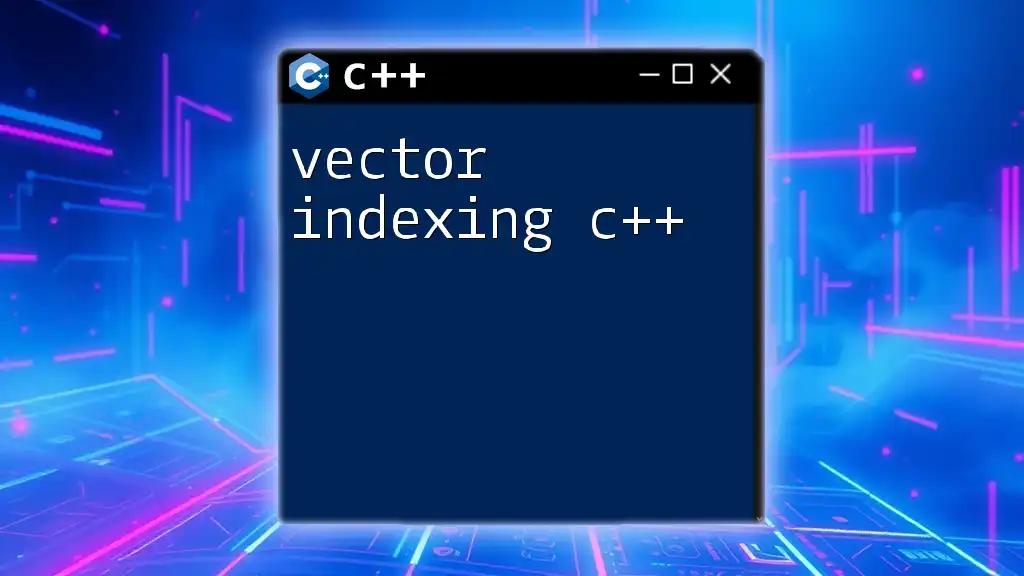
The Concept of IndexOf
What is IndexOf?
The `IndexOf` function is an essential utility that finds the position (index) of a given element within a vector. This functionality is crucial when you need to search for a specific item without iterating through the entire vector manually.
Challenges with IndexOf in C++
C++ STL does not provide a native `IndexOf` method for vectors, which can pose a challenge when trying to locate elements efficiently. Many developers resort to manual iterations to find indices, which can lead to inefficient code if poorly implemented.
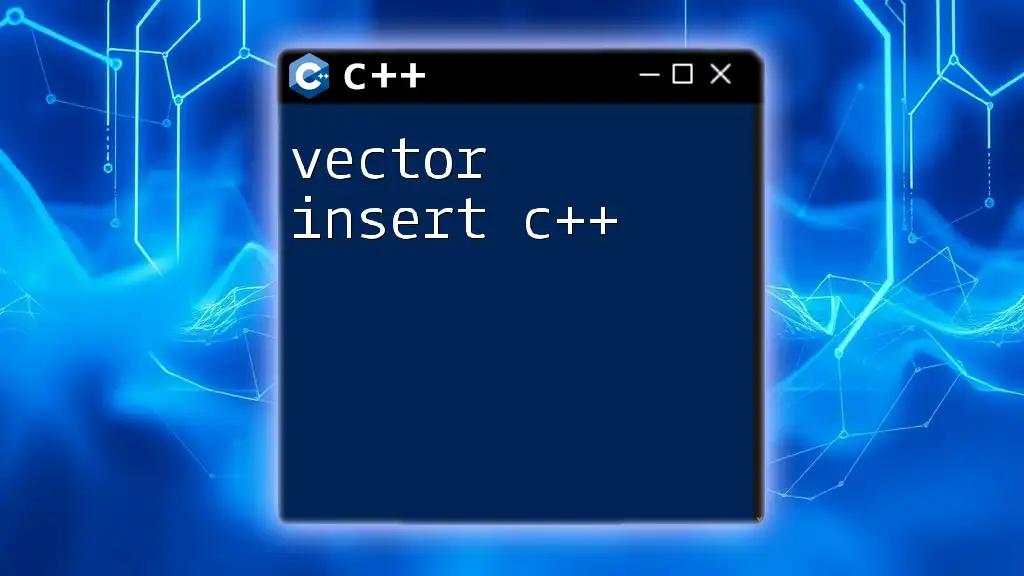
Implementing IndexOf in C++
Writing a Custom IndexOf Function
To handle the search for an element's index, we can create a custom `IndexOf` function. Below is a simple implementation that seeks out an integer value in a vector.
#include <iostream>
#include <vector>
int IndexOf(const std::vector<int>& vec, int target) {
for (size_t i = 0; i < vec.size(); i++) {
if (vec[i] == target) {
return i; // Return the index if found
}
}
return -1; // Return -1 if not found
}
In this function:
- We iterate through each element of the vector.
- Upon finding the target element, the function returns its index.
- If the target isn't found, it returns `-1`, indicating absence.
Example of IndexOf Implementation
The following complete example showcases how to implement and utilize the `IndexOf` function.
int main() {
std::vector<int> numbers = {10, 20, 30, 40, 50};
int index = IndexOf(numbers, 30);
std::cout << "Index of 30: " << index << std::endl; // Output should be 2
return 0;
}
This snippet initializes a vector, searches for the value `30`, and outputs its index, which serves as a practical application for the `IndexOf` function.

Enhancements for the IndexOf Function
Handling Multiple Occurrences
If you need to find all occurrences of an element in a vector, the `IndexOf` function can be modified to return a vector of indices.
std::vector<int> IndexOfAll(const std::vector<int>& vec, int target) {
std::vector<int> indices;
for (size_t i = 0; i < vec.size(); i++) {
if (vec[i] == target) {
indices.push_back(i);
}
}
return indices;
}
In this function, every time the target element is found, its index gets added to a new vector of indices, allowing you to get all positions where the target appears.
Using Templates for IndexOf
To make the `IndexOf` function more versatile, we can utilize C++ templates to handle various data types. This implementation allows the function to work with any type of vector, not just integers.
template <typename T>
int IndexOf(const std::vector<T>& vec, const T& target) {
for (size_t i = 0; i < vec.size(); i++) {
if (vec[i] == target) {
return i;
}
}
return -1;
}
This function now accepts any vector type, enhancing its usability across different contexts.
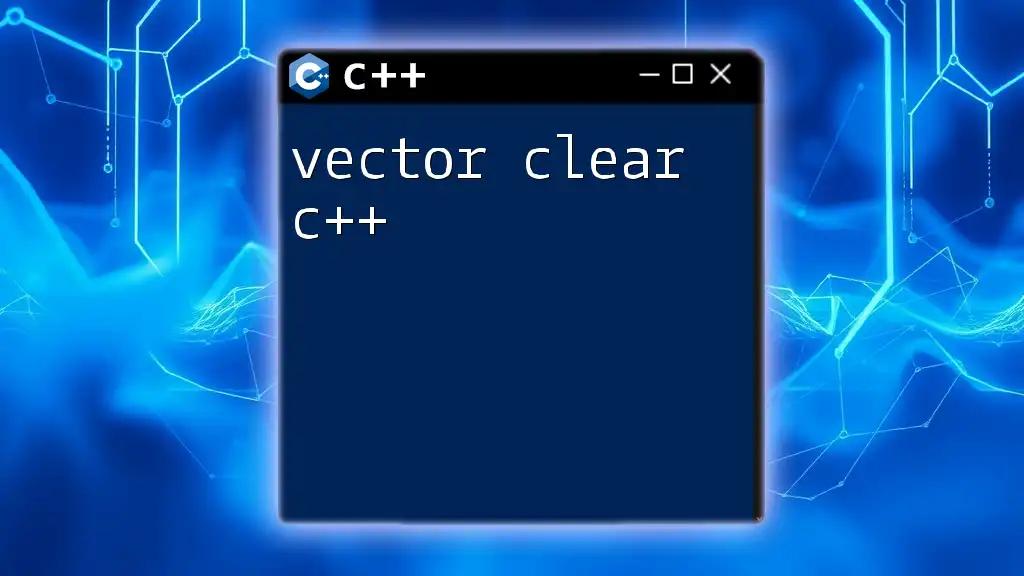
Alternative Methods to Find Index
Using Standard Library Functions
An alternative to creating your own `IndexOf` function is to utilize the powerful C++ Standard Library functions found in the `<algorithm>` header. The `std::find` function can be particularly useful.
#include <algorithm>
int IndexOfUsingFind(const std::vector<int>& vec, int target) {
auto it = std::find(vec.begin(), vec.end(), target);
if (it != vec.end()) {
return std::distance(vec.begin(), it);
}
return -1; // Not found
}
Here, `std::find` is employed to search for the element, simplifying the implementation significantly. However, while it's elegant, there’s a trade-off: this approach still requires a linear search, which can have performance implications similar to the custom implementation.
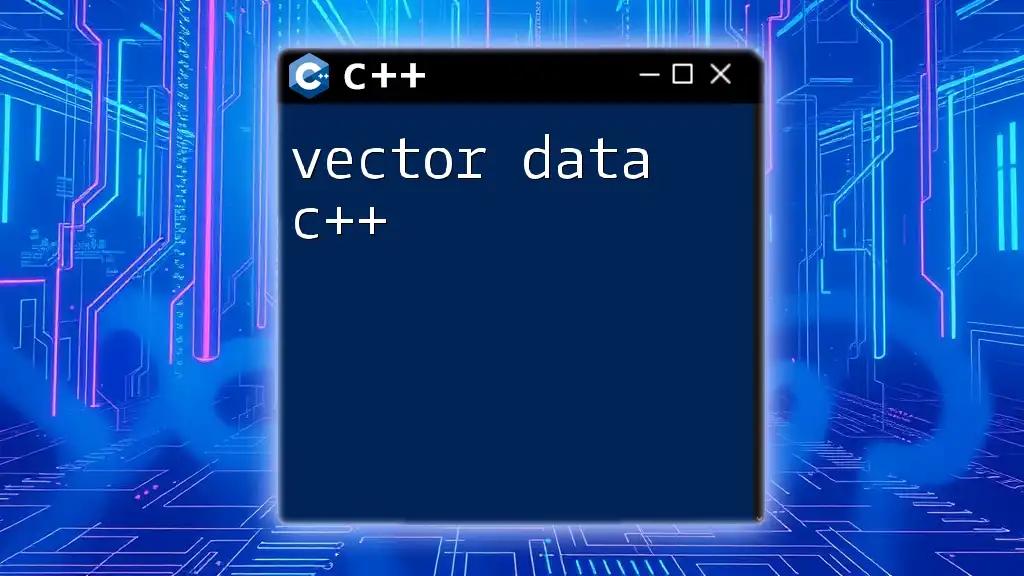
Performance Considerations
Time Complexity
The time complexity of both the custom and standard implementations of `IndexOf` is O(n), where n is the number of elements in the vector. This linear time complexity arises from the need to examine each element in the worst case.
Memory Considerations
Vectors are generally more memory-efficient than arrays due to their dynamic nature, but this comes at a cost. Each time you add elements, memory is allocated dynamically, which can result in memory reallocation and copying of existing items under certain conditions. Understanding these nuances is important for optimal performance.
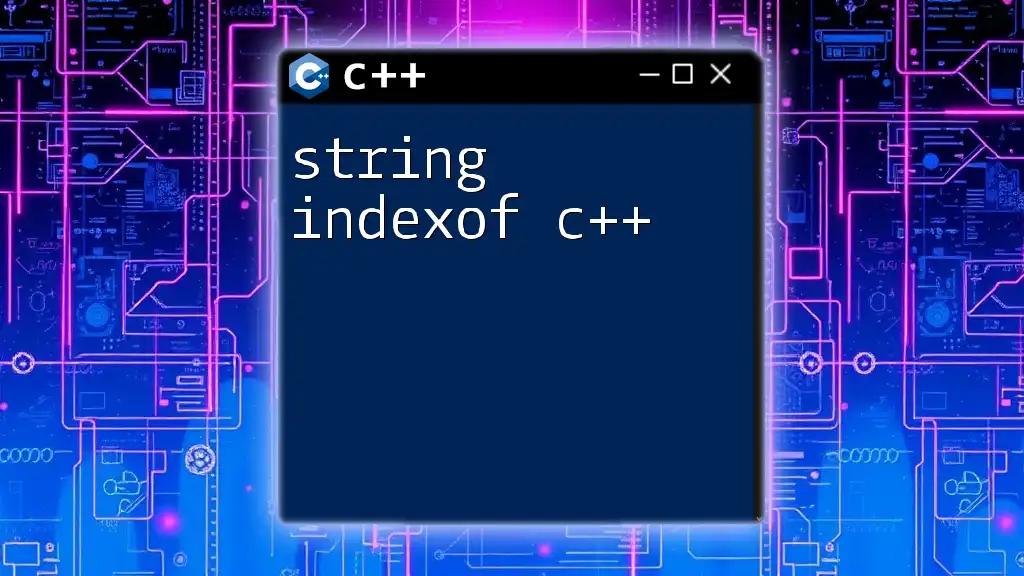
Conclusion
Implementing an `IndexOf` function for vectors in C++ is a critical skill that enhances your programming acumen. Whether you decide to write your own version or leverage STL library functions, understanding how to effectively find the index of elements within vectors is essential for efficient coding.
Practice these concepts, iterate with variations of your implementations, and explore the potential of your code. Your journey into the world of vector indexof C++ is just beginning!
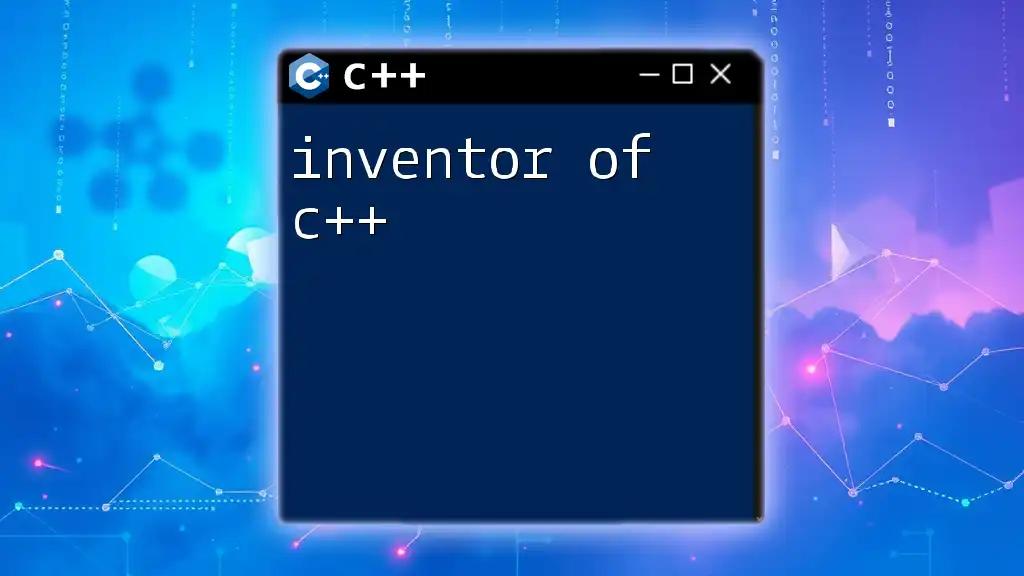
Additional Resources
Recommended Books and Tutorials
Explore various resources that dive deeper into C++ STL, vectors, and algorithm efficiency. You can find reliable books and online courses that can enrich your understanding and offer intricate details on C++ programming.
Useful Links
For further reading, review the official C++ documentation and related tutorials that cover advanced topics, such as templates, vector manipulations, and more.
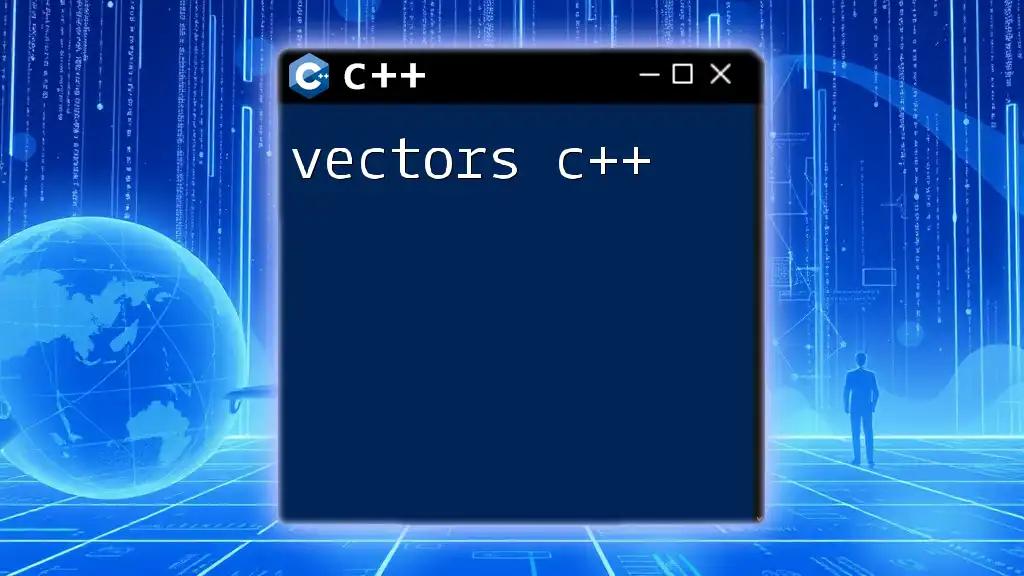
Call to Action
We’d love to hear your experiences! Share your implementations or any challenges you faced in the comments below. Don’t forget to follow us for more insightful C++ tutorials and tips!