C++ was invented by Bjarne Stroustrup in the early 1980s as an extension of the C programming language, incorporating object-oriented features and enabling better software development.
Here's a simple example of a C++ program that introduces a class and demonstrates basic object-oriented principles:
#include <iostream>
using namespace std;
class Dog {
public:
void bark() {
cout << "Woof!" << endl;
}
};
int main() {
Dog myDog;
myDog.bark();
return 0;
}
Bjarne Stroustrup: A Brief Biography
Early Life and Education
Bjarne Stroustrup was born on December 30, 1950, in Aarhus, Denmark. His early fascination with mathematics and programming was evident during his schooling. He pursued a degree in mathematics and computer science at the University of Aarhus, showcasing his brilliance and commitment to learning. Stroustrup then went on to complete his master's thesis in computer science, further solidifying his foundation in programming.
His academic journey continued as he moved to the United States to attend Columbia University, where he earned his Ph.D. in computer science. This trajectory helped him cultivate a unique understanding of both theoretical and practical aspects of programming.
Professional Journey
Stroustrup began his professional career at Bell Labs in Murray Hill, New Jersey, where he played a crucial role in the development of various programming technologies. It was here, in 1979, that he embarked on the monumental task of creating a programming language that combined the efficiency and power of C with the object-oriented features of Simula. This innovative endeavor marked the inception of C++, the language that would go on to shape software development for decades.
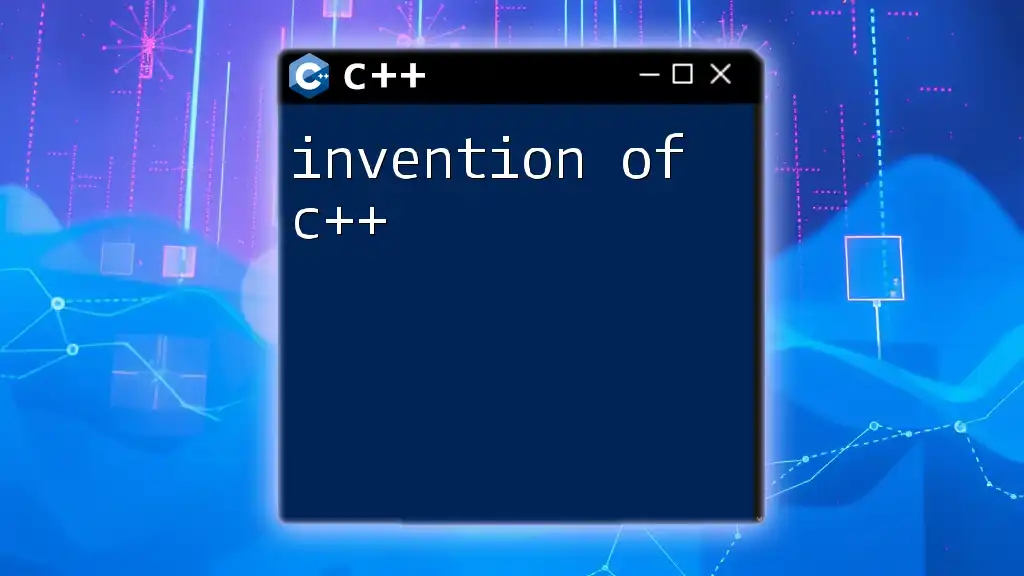
The Birth of C++
The Evolution of Programming Languages
To grasp the importance of C++, it is essential to understand the lineage of programming languages that preceded it. The late 1960s and 1970s were dominated by procedural programming languages like C and FORTRAN. Simula, created in the 1960s, introduced object-oriented programming (OOP), laying a crucial foundation that influenced many languages, including C++.
As programming needs evolved, developers sought a language that could offer both low-level access to memory (for performance) and high-level abstractions (for ease of use). Stroustrup saw an opportunity to bridge this gap and leverage the strengths of existing languages.
The Creation of C++
In 1983, after several years of innovation and prototyping, Stroustrup officially unveiled C++. His vision was to create a language that was efficient, flexible, and capable of addressing upcoming software challenges. The term "C++" reflects its evolution from C, with "++" symbolizing the incremental improvement of the language.
Stroustrup focused on incorporating powerful features like:
- Encapsulation: Allowing developers to bundle data and methods together.
- Inheritance: Facilitating the creation of new classes based on existing ones for code reuse.
- Polymorphism: Supporting the ability of different classes to be treated as instances of the same class through a common interface.
Here is a simple code snippet that demonstrates a basic class structure in C++:
class Animal {
public:
void sound() {
std::cout << "Some generic animal sound!" << std::endl;
}
};
class Dog : public Animal {
public:
void sound() {
std::cout << "Woof!" << std::endl;
}
};
Dog myDog;
myDog.sound(); // Outputs: Woof!
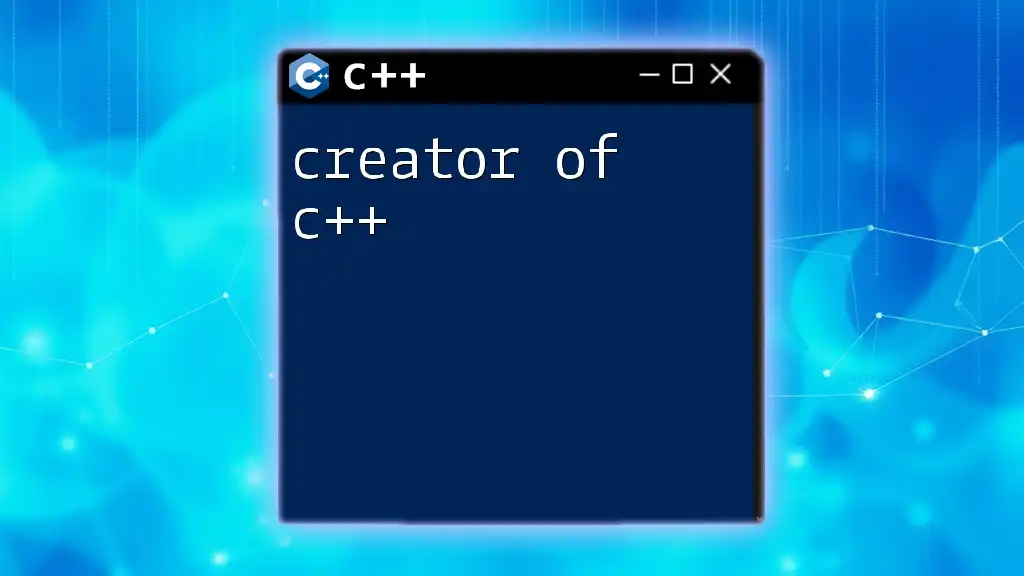
Key Features of C++
Object-Oriented Programming
One of the standout features of C++ is its support for Object-Oriented Programming (OOP). OOP allows developers to model real-world entities and concepts efficiently, which enhances code organization and manageability.
With C++, developers can define classes, create objects, and implement access specifiers (like `public`, `private`, and `protected`), which help control the accessibility of class members. This paradigm can lead to cleaner and more reusable code.
Performance and Efficiency
C++ was designed with performance in mind. Being a compiled language, it enables programmers to write code that closely interacts with hardware while retaining high levels of efficiency.
Stroustrup emphasized memory management features allowing developers to have fine-grained control over system resources. This efficiency makes C++ particularly suitable for systems programming, game development, and applications where performance is critical.
int* arr = new int[10]; // Dynamically allocate memory
// Use arr...
delete[] arr; // Deallocate memory
Standard Template Library (STL)
One of the most influential contributions of C++ is the Standard Template Library (STL), which offers a collection of common data structures and algorithms. The STL makes it easier for developers to write efficient and high-performance applications.
The STL includes:
- Containers: Such as vectors, lists, and maps.
- Algorithms: Sorting, searching, and manipulating data.
- Iterators: To navigate through complex data structures seamlessly.
Here’s a simple example demonstrating the use of a vector from the STL:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " "; // Outputs: 1 2 3 4 5
}
return 0;
}
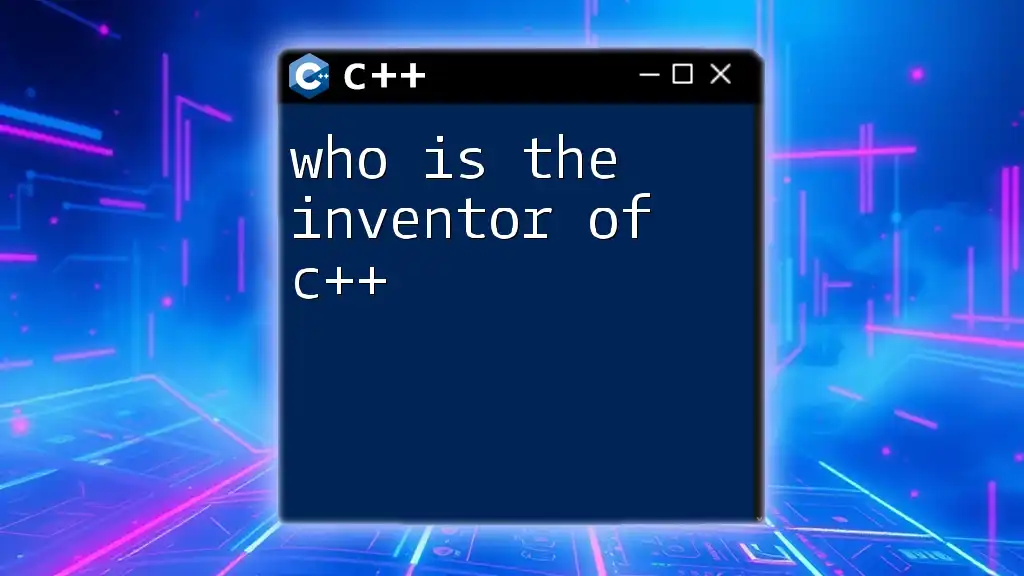
Contributions of C++ to Software Development
Impact on Modern Programming
C++ has profoundly influenced the landscape of modern programming, bridging the gap between low-level system programming and high-level application development. Many languages developed post-C++, such as Java and C#, drew inspiration from its features.
C++ has placed a strong emphasis on performance and efficiency, making it a governing choice for applications where speed is critical, such as real-time systems, video games, and large-scale applications.
C++ in Industry
Several prominent companies and systems utilize C++ extensively:
- Microsoft: for applications including Windows and game development via DirectX.
- Apple: in the development of their operating systems.
- Game Development Studios: leverage C++ for its robust performance in graphics rendering and real-time logic.
Real-world applications like Adobe Photoshop and the Unreal Engine showcase C++’s capability to handle complex operations while maintaining speed.
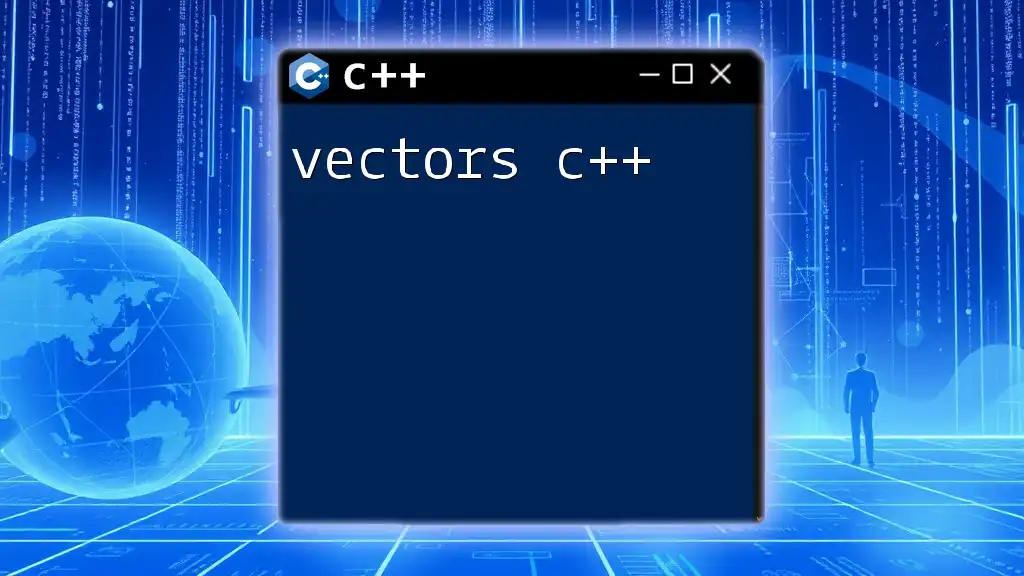
Legacy of Bjarne Stroustrup
Continued Influence
Stroustrup’s contributions to programming do not stop with the creation of C++. He has been actively involved in its development and standardization throughout the years, establishing himself as a leading voice in the programming community. He frequently presents at conferences and provides insights on best practices for using C++ effectively.
The Future of C++
The evolution of C++ continues with ongoing enhancements and updates. With newer standards such as C++11, C++14, C++17, and C++20, the language introduces features like lambdas, smart pointers, and improved type inference, making it more approachable for modern developers while retaining its core performance benefits.
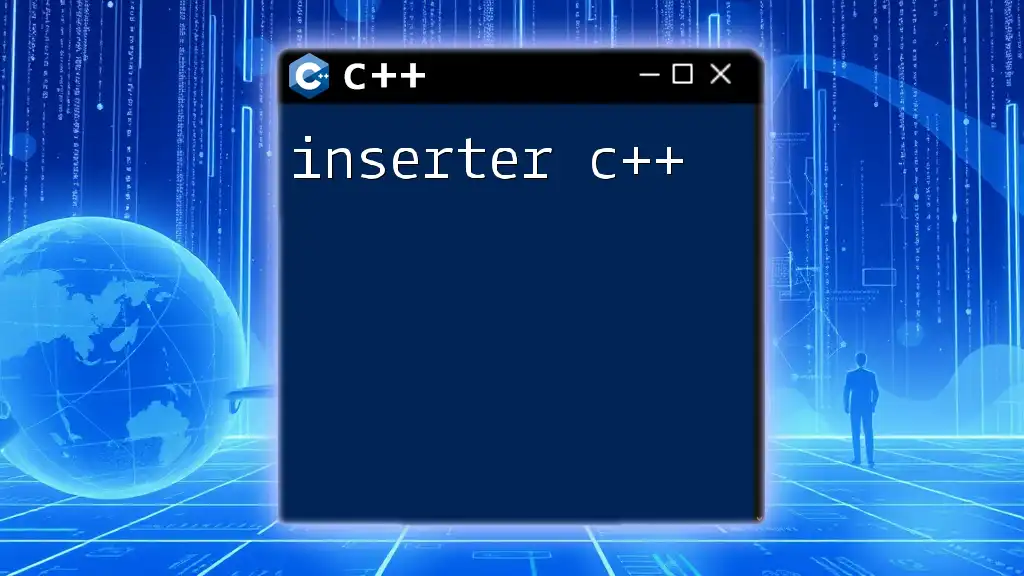
Conclusion
Bjarne Stroustrup, the inventor of C++, has left an indelible mark on the world of programming. His innovative vision led to the creation of a powerful language that continues to influence numerous domains of software development. Understanding Stroustrup's contributions and the evolution of C++ provides programmers with a richer perspective and a deeper appreciation of this versatile language. As C++ progresses, it embodies not just the past but also the future of programming, hinting at possibilities yet to come.
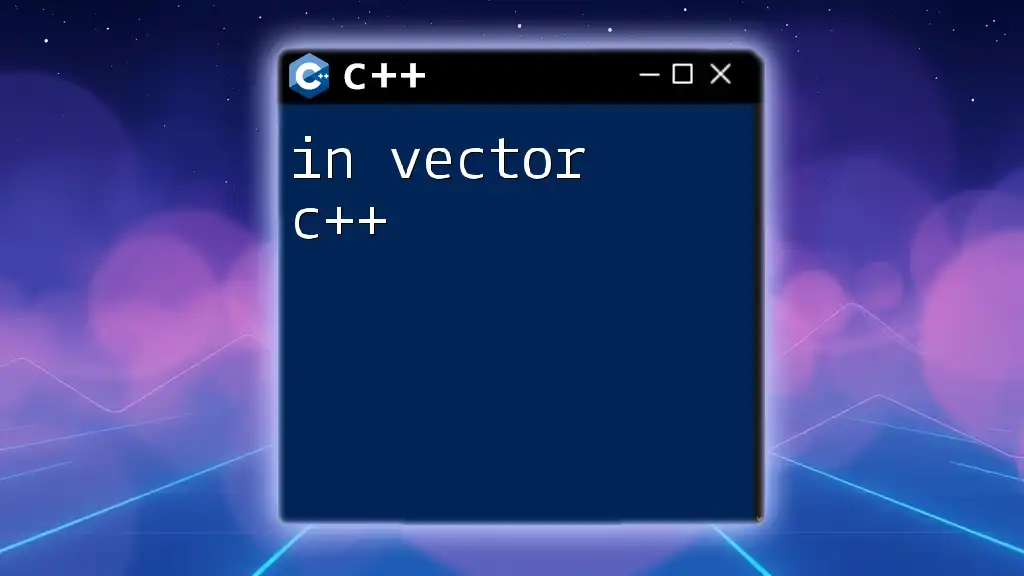
Resources and Further Reading
To further explore the amazing world of C++, consider diving into some of these resources:
- Books by Bjarne Stroustrup: Such as "The C++ Programming Language" and "Programming: Principles and Practice Using C++."
- Online platforms: Websites like Codecademy, Coursera, and Udemy offer courses focused on C++ programming.
- Communities and Conferences: Engaging with forums like Stack Overflow and attending programming conferences can enhance your knowledge and connect you with other C++ enthusiasts.