In C++, you can insert elements into a `std::vector` using the `push_back` method, which adds an element to the end of the vector.
#include <vector>
int main() {
std::vector<int> numbers;
numbers.push_back(5); // Inserts 5 into the vector
return 0;
}
Understanding Insert Operations in C++
What is the Insert Operation?
The insert operation in C++ is a fundamental action that allows programmers to add elements to various data structures. This capability is crucial for dynamic programming, where data grows or changes over time. For example, managing collections of data—like user inputs, inventory items, or any dynamic data set—often necessitates the ability to insert new elements seamlessly.
When to Use Insert Operations
Insert operations are particularly useful in scenarios where the order of elements matters, or when data needs to be frequently updated. For instance, when implementing a list of tasks or maintaining a customer queue, insertions play a pivotal role. It is also worth comparing insertion with other operations, such as appending or deleting, where different methods may be more efficient depending on the use case.
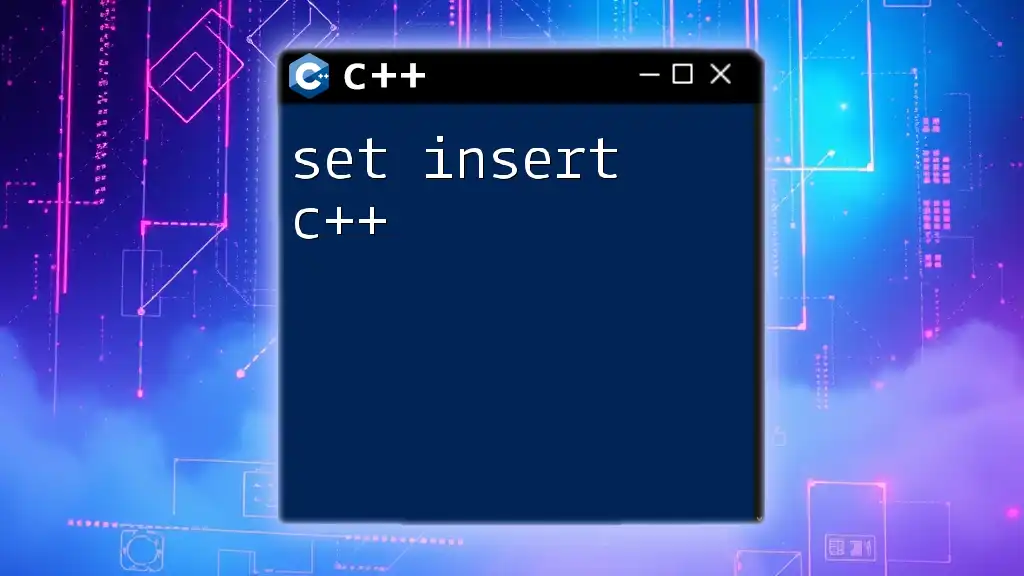
Standard C++ Containers and Insertion
Introduction to C++ Standard Library Containers
C++ provides several standard library containers designed to handle various types of collections, including vectors, lists, and deques. Each container has its own strengths and weaknesses regarding insertion operations. Understanding these differences will enable you to choose the most appropriate container for your specific needs.
Inserting Elements in Vectors
Description of Vectors
Vectors are sequence containers that dynamically resize themselves. They are essentially arrays that grow as new elements are added.
Methods for Inserting in Vectors
-
Using `push_back()` Method
The `push_back()` method allows you to add an element to the end of a vector, resulting in efficient operations under most circumstances. For example:std::vector<int> vec; vec.push_back(10); // Inserts 10 at the end
-
Using `insert()` Method
The more versatile `insert()` method allows insertion at any specified position within the vector. This can be useful when the order is crucial:vec.insert(vec.begin() + 1, 20); // Inserts 20 at index 1
Inserting Elements in Lists
Description of Lists
Lists are sequence containers that allow for flexible insertion and removal of elements in a linked list format, typically providing better performance for insertions and deletions than vectors.
Methods for Inserting in Lists
-
Using `push_front()` and `push_back()`
With lists, you can quickly add elements at either end using `push_front()` or `push_back()`. This offers greater efficiency for certain applications:std::list<int> lst; lst.push_front(5); // Inserts 5 at the beginning lst.push_back(15); // Inserts 15 at the end
-
Using `insert()` Method
Similar to vectors, lists also support the `insert()` method, allowing elements to be added at arbitrary positions:auto it = lst.begin(); lst.insert(it, 10); // Inserts 10 at the beginning
Inserting Elements in Deques
Description of Deques
Deques (double-ended queues) are dynamic arrays that allow insertion and deletion at both ends and provide flexibility to grow from both sides.
Methods for Inserting in Deques
-
Using `push_front()` and `push_back()`
Deques also support the `push_front()` and `push_back()` methods, making them ideal for scenarios that require frequent insertions at both ends:std::deque<int> deq; deq.push_front(3); // Inserts 3 at the beginning deq.push_back(7); // Inserts 7 at the end
-
Using `insert()` Method
For index-based insertion, you can use the `insert()` method:deq.insert(deq.begin() + 1, 5); // Inserts 5 at position 1
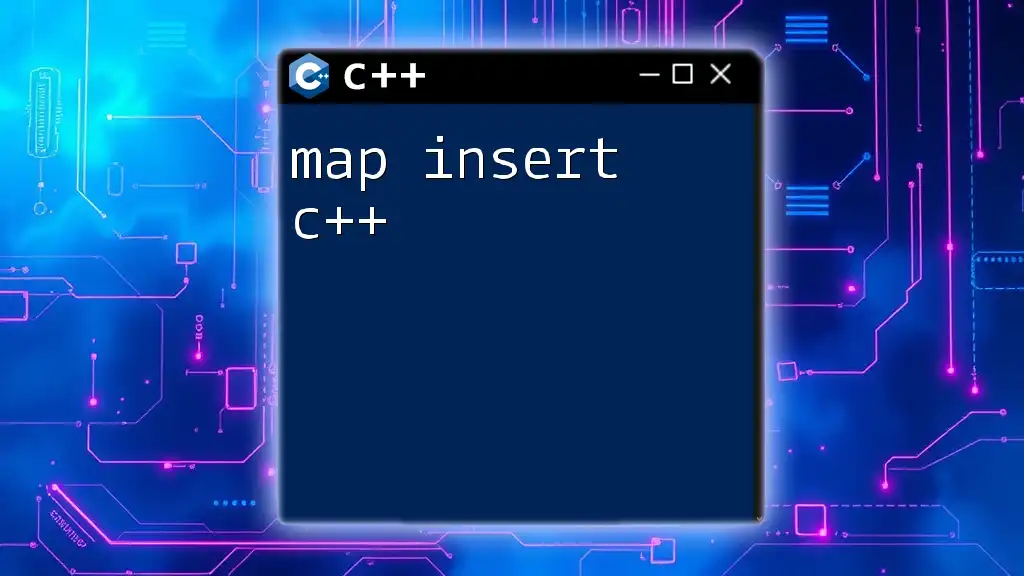
Inserting Elements in Other Data Structures
Inserting in Sets
Introduction to Sets
Sets are container types that maintain unique elements, automatically sorting them as they are added.
Methods for Inserting in Sets
- Using `insert()` Method
The `insert()` method allows you to add elements to a set:std::set<int> mySet; mySet.insert(2); // Inserts 2, maintains uniqueness
Inserting in Maps
Introduction to Maps
Maps are associative containers that store key-value pairs, with each key pointing to a unique value.
Methods for Inserting in Maps
-
Using `insert()` Method
The `insert()` method lets you add new key-value pairs:std::map<int, std::string> myMap; myMap.insert(std::make_pair(1, "One")); // Inserts key-value pair
-
Using `emplace()` Method
The `emplace()` method can be more efficient as it constructs the element in place:myMap.emplace(2, "Two"); // Inserts new pair
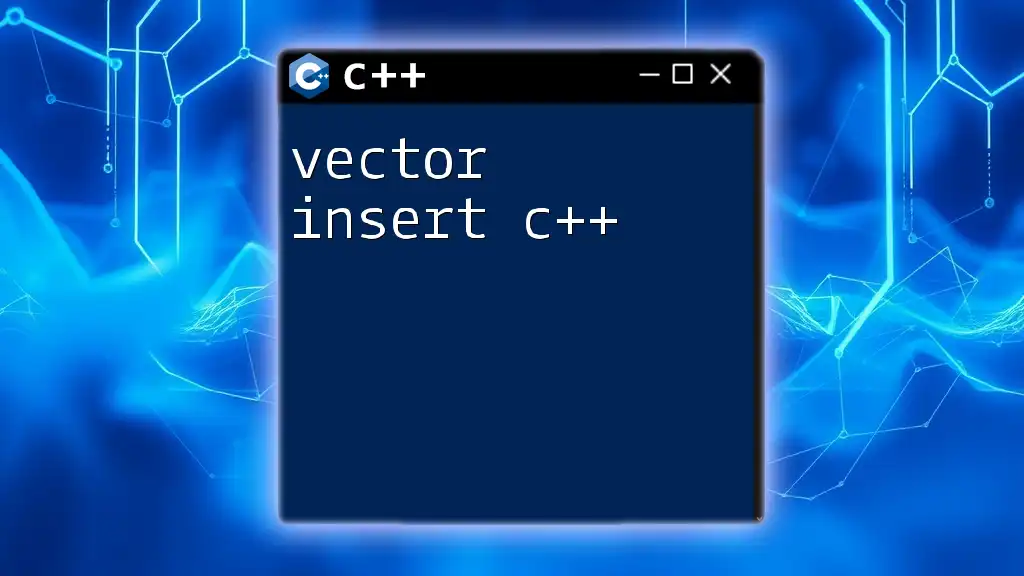
Best Practices for Insert Operations in C++
Performance Considerations
Understanding the time complexity associated with different containers is vital. For example, inserting an element in a vector at the end is an O(1) operation, while inserting in the middle can be O(n) due to the need to shift elements. Choosing the right data structure based on these performance metrics can significantly enhance your application’s efficiency.
Choosing the Right Container
When deciding which container to use for your insertion operations, consider factors like expected frequency of modifications, memory usage, and whether order matters. For high-frequency insertion and retrieval where the order is not critical, lists or deques may be more suitable than vectors.
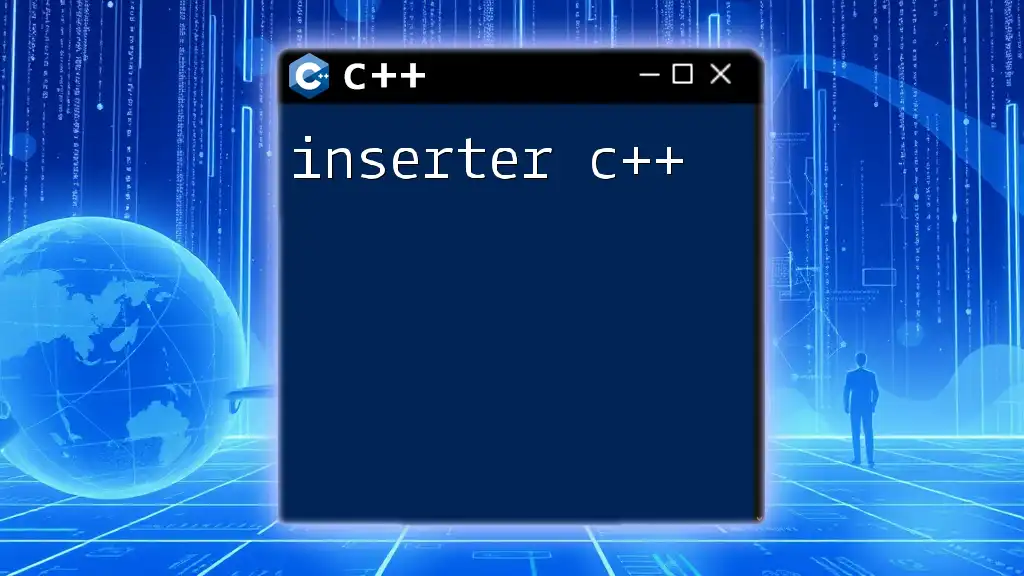
Common Mistakes to Avoid
Overuse of `push_back` in a Loop
Using `push_back()` in a tight loop can lead to performance bottlenecks if the vector has to resize multiple times. Instead, reserve memory beforehand with `reserve()`:
std::vector<int> vec;
vec.reserve(100); // Reserves space for 100 elements
Forgetting to Check for Duplicate Elements
In sets and maps, forgetting to check for duplicates can lead to unexpected behavior. Understanding how these containers handle duplication is essential for maintaining data integrity and performance.
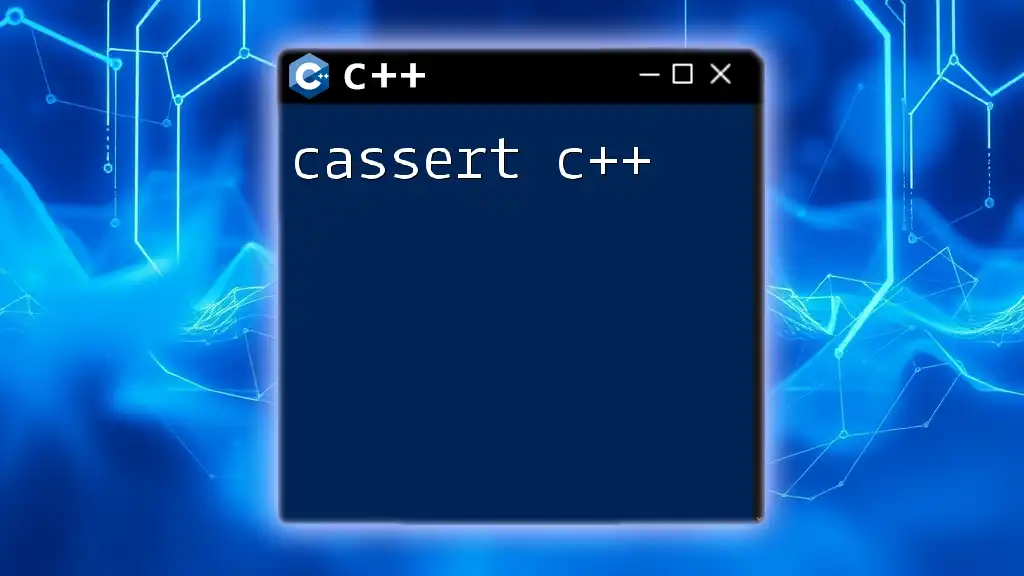
Conclusion
In summary, mastering the `insert` operations in C++ is essential for effective data management. By understanding the characteristics of different containers and the various methods available for inserting elements, you can make informed decisions that enhance your programming efficiency and application performance. Explore further resources and practice regularly to solidify your knowledge and skills in this critical aspect of C++.