The `insert` function in C++ allows you to add elements to a `set`, ensuring that no duplicates are stored.
#include <iostream>
#include <set>
int main() {
std::set<int> mySet;
mySet.insert(5);
mySet.insert(10);
mySet.insert(5); // This will be ignored since 5 is a duplicate
for (const auto& elem : mySet) {
std::cout << elem << " ";
}
return 0;
}
What is a C++ Set?
A C++ set is a part of the Standard Template Library (STL) that represents a collection of unique elements. It automatically sorts these elements, allowing for efficient operations related to searching, addition, and removal. Sets are implemented as balanced binary trees (usually Red-Black Trees), providing O(log n) time complexity for most operations.
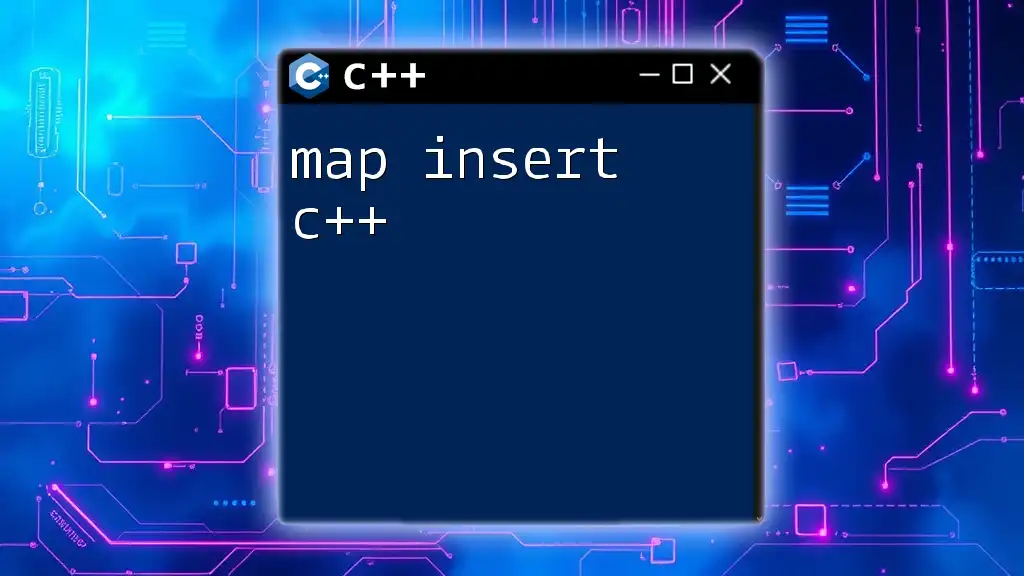
Why Use Sets in C++?
Using sets in C++ provides several advantages:
- Unique Element Storage: A set automatically ensures that no duplicates are stored within it, which can be vital for maintaining integrity in collections of data.
- Automatic Sorting: Elements in a set are kept in a specific order, making it easier to traverse or search through the dataset.
- Support for Efficient Algorithms: Many algorithms in C++ rely on the properties of sets, such as eliminating duplicates, counting unique elements, and performing quick lookups.
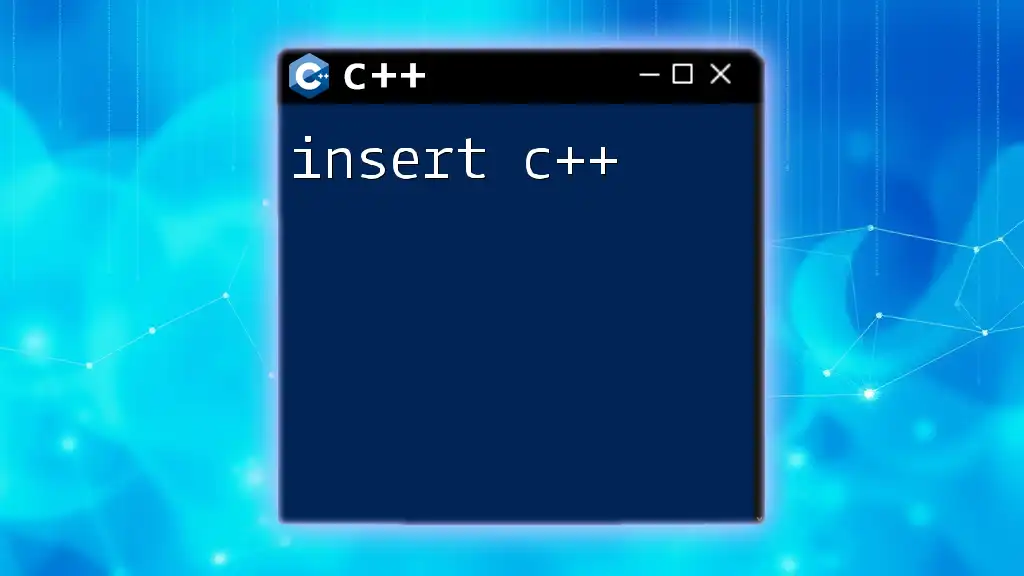
Declaring and Initializing a Set
Declaring a set in C++ can be done easily using its constructor syntax. For instance:
std::set<int> mySet = {1, 2, 3};
This code initializes a set of integers with three elements: 1, 2, and 3.
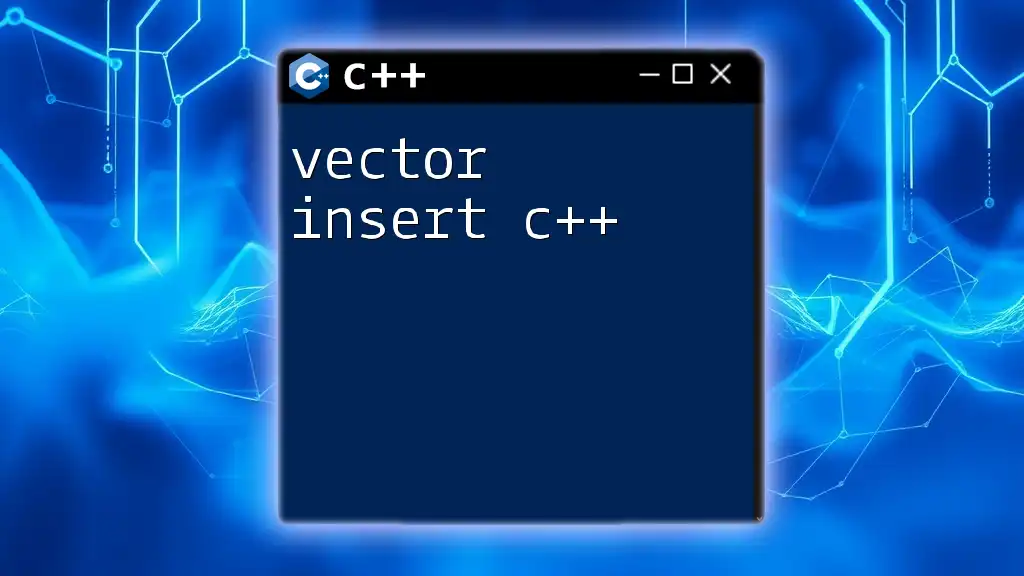
Common Methods Associated with Sets
Sets come equipped with a variety of methods that can be utilized for different operations. Among these, methods like `insert`, `erase`, `find`, and `clear` are commonly used. This article will focus particularly on the `insert` method, which is pivotal when adding elements to a set.
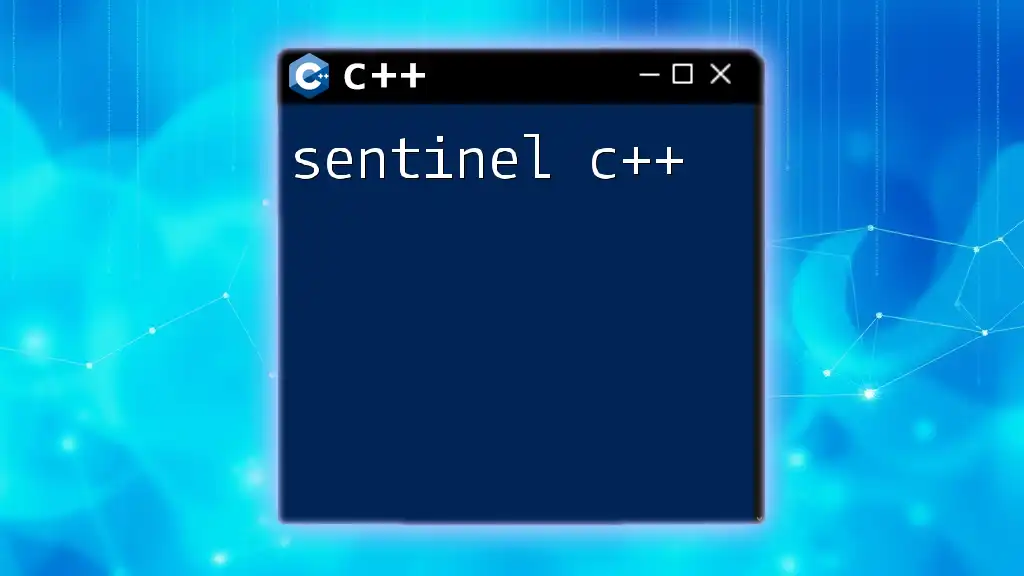
The `insert` Method in C++
Syntax of the `insert` Method
The syntax for the `insert` method is straightforward. It works as follows:
std::pair<set::iterator, bool> insert(const value_type& val);
This method employs a return type that consists of a pair: an iterator pointing to either the newly inserted element or to the element that already existed, and a Boolean that indicates whether the insertion was successful.
How to Use the `insert` Method
Inserting Single Elements
To insert a single element into a set, the `insert` method can be invoked directly:
std::set<int> mySet;
mySet.insert(5);
mySet.insert(10);
A crucial feature of sets is that they do not allow duplicates. Therefore, attempting to insert an element that already exists will not alter the set, ensuring that all elements remain unique.
Inserting Multiple Elements at Once
With the `insert` function, you can also add multiple elements efficiently at once. This can be done using initializer lists or containers such as vectors. For example:
std::vector<int> vec = {1, 2, 3, 4};
mySet.insert(vec.begin(), vec.end());
This code snippet demonstrates how to add elements from a vector to the set. Utilizing this approach is not only convenient but also enhances performance by minimizing the number of function calls.
Insert Result Handling
Understanding the Return Value
The `insert` method returns a pair where the first element is an iterator to the element in the set and the second element is a Boolean value that tells whether the insertion was successful.
Here's how you can handle the result:
auto result = mySet.insert(5);
if (!result.second) {
std::cout << "Element already exists." << std::endl;
}
In this example, if the value `5` is already present in `mySet`, the output would indicate that the element cannot be inserted again, thus reinforcing the property of uniqueness.
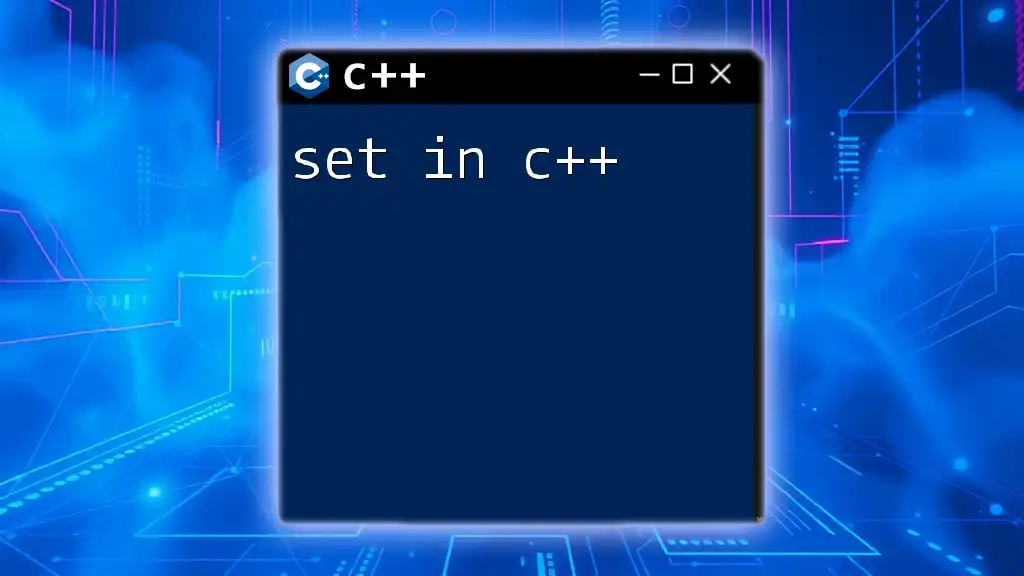
Special Cases with the `insert` Method
Inserting Elements of Different Data Types
One of the strengths of C++ sets is their ability to store various data types, provided that they comply with the requirements of sorting (usually through comparison operators). For instance, to create a set of strings, you can do:
std::set<std::string> strSet;
strSet.insert("Hello");
strSet.insert("World");
This usage demonstrates type safety and flexibility in terms of what can be stored.
Inserting Objects
You can also create a set that contains user-defined objects by implementing the comparison operator. Here’s an example:
struct Person {
std::string name;
int age;
bool operator<(const Person& other) const {
return age < other.age; // Defines order based on age
}
};
std::set<Person> people;
people.insert({"Alice", 30});
people.insert({"Bob", 25});
In this situation, the `Person` struct defines an operator `<` to enable the sorting of elements in the set based on the age attribute.
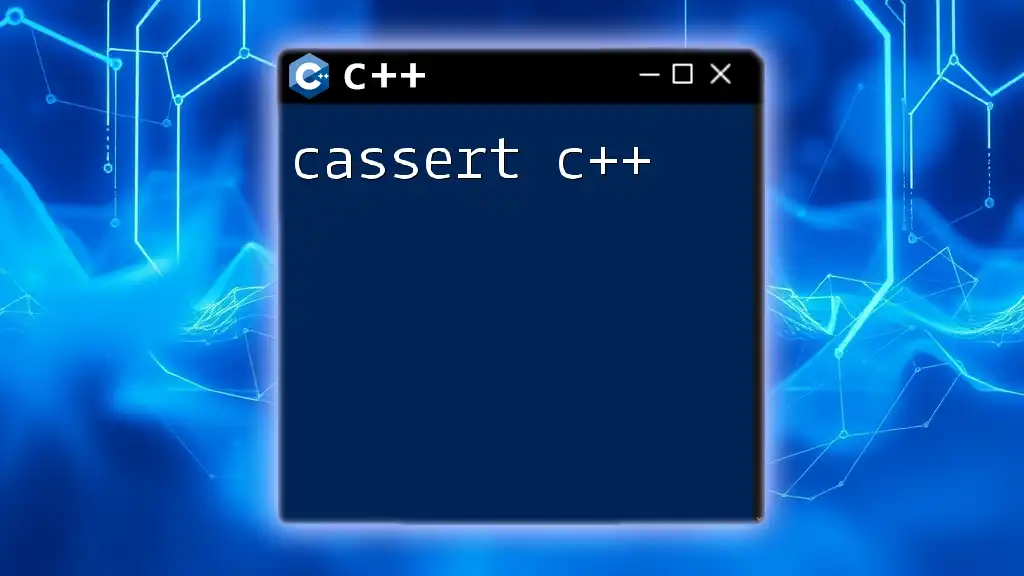
Performance Considerations
Time Complexity of the `insert` Method
When discussing efficiency, it’s essential to understand that the `insert` operation in a C++ set has an average-case time complexity of O(log n) due to the underlying tree structure. However, in the worst-case scenario, it can also take O(n) time if the tree is unbalanced.
Tips for Effective Usage of Sets
- Consider using sets when you need to maintain a collection of unique elements.
- Analyze scenarios where quick lookup times are essential; sets provide these through their ordered structure.
- Be mindful of memory usage, especially when inserting a large number of elements.
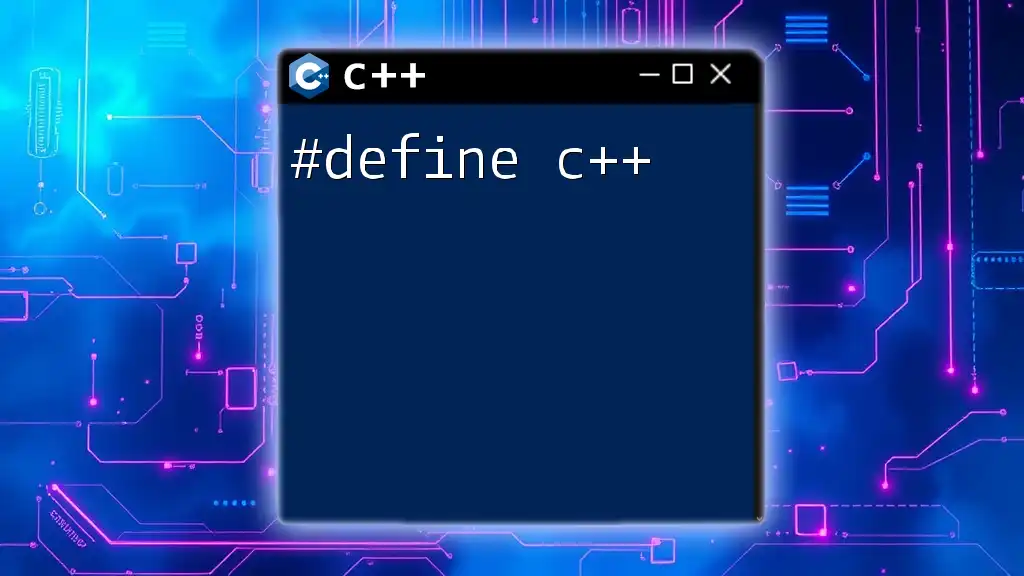
Conclusion
This guide has provided an in-depth examination of the `set insert c++` operation, outlining its usage, syntax, and the benefits of utilizing sets in C++. By understanding how to properly use and handle sets, you empower yourself to leverage their unique properties in various programming scenarios. As you continue to experiment with these concepts, consider exploring the additional features of sets and other STL containers to become more proficient in C++.
Additional Resources
For further learning, you may want to explore the official C++ documentation or seek out tutorials focusing on the Standard Template Library (STL) and its various components. These resources can provide more examples and insight into using C++ effectively.