The `set::erase` function in C++ removes elements from a set, either by value or by iterator, effectively managing the contents of the collection.
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5};
mySet.erase(3); // Removes the value 3 from the set
// Displaying the modified set
for (const int& num : mySet) {
std::cout << num << " ";
}
return 0;
}
What is a Set in C++?
A set in C++ is a container that stores unique elements following a particular order. It is part of the C++ Standard Library and is implemented as a balanced binary search tree (usually a Red-Black Tree), ensuring that all elements are in a sorted state.
-
Properties of a Set:
- Contains only unique elements, meaning duplicates are automatically ignored.
- Elements are sorted according to a specific comparison criterion (default is `operator<`).
- Provides fast operations for insertion, deletion, and search, all in logarithmic time complexity.
-
Benefits of Using Sets:
- Automatic management of duplicates.
- Efficient data retrieval due to its ordering properties.
- Straightforward syntax for common operations, enabling effective data handling in various applications.

What Does Erase Do in C++?
The erase function is utilized to remove one or more elements from a set. This functionality is crucial for maintaining the integrity of data by allowing programmers to manage the contents of a set dynamically.
Purpose of Erase in Managing Data
Using `set::erase`, you can:
- Remove specific values.
- Eliminate elements using iterators.
- Clear out a range of elements effectively.
By employing this function, you can control the data structure's contents as your application evolves.

Basic Syntax Overview
The syntax for the `set::erase` function varies with the method used for removal:
set.erase(value); // Erase element by value
set.erase(iterator); // Erase element by iterator
set.erase(iterator_start, iterator_end); // Erase elements in a range
Understanding Function Overloading
The `erase` function is overloaded, meaning you can use it in different forms, allowing flexibility depending on the situation. This is an hallmark of C++’s rich function overloading capabilities.
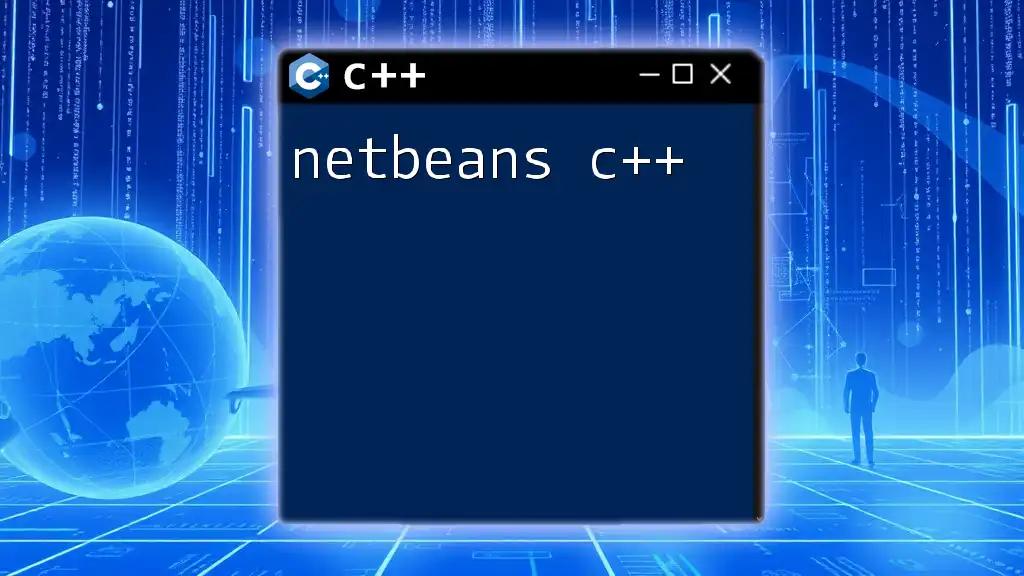
How to Use Set Erase in C++
Erasing an Element by Value
You can easily erase an element from a set using its value:
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5};
mySet.erase(3); // Erases element with value 3
for(auto elem : mySet) {
std::cout << elem << " ";
}
return 0;
}
In this example, the value `3` is removed from the set, and the output will show the remaining elements as `1 2 4 5`. This showcases how easily you can manage the contents of your set.
Erasing by Iterator
Using an iterator provides more control, especially when you want to operate on elements without explicitly knowing their values.
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5};
std::set<int>::iterator it = mySet.find(4);
if (it != mySet.end()) {
mySet.erase(it); // Erases element using iterator
}
for(auto elem : mySet) {
std::cout << elem << " ";
}
return 0;
}
In this case, by finding the iterator for value `4`, you can erase it seamlessly without manipulating the set directly by value. The resulting output will be `1 2 3 5`.
Erasing a Range of Elements
Suppose you need to delete multiple contiguous elements. The `erase` function supports removing a range as well:
#include <iostream>
#include <set>
int main() {
std::set<int> mySet = {1, 2, 3, 4, 5, 6, 7};
mySet.erase(mySet.find(2), mySet.find(5)); // Erases elements in the range [2, 5)
for(auto elem : mySet) {
std::cout << elem << " ";
}
return 0;
}
This example will erase the elements `2`, `3`, and `4`, leaving us with `1 5 6 7`. Using iterators for range-based erasure helps maintain the set's structure efficiently.

What Does Erase Return?
When using `set::erase`, the function provides specific return values depending on how you call it.
- Erase by value: The function returns the number of elements that were removed (either 0 or 1 since sets hold unique elements).
- Erase by iterator: After erasing, the iterator itself is gone; thus, it’s essential to ensure you check if the erase operation evaluates successfully.
Understanding these return values can inform you about the operation's success and the state of the set afterward.

Efficiency of Erase Operation in Sets
Complexity for erase operations in sets is generally O(log n) because of the underlying tree structure. However, it’s crucial to be aware of scenarios where frequent modification may impact performance. Frequent call to `erase` can lead to higher overhead, especially in performance-sensitive applications.
When Erasing Might Be Costly
- If elements are often removed, consider data structures more efficient for such operations, or pre-allocate resources.
- Understand that excessive deletions can lead to fragmentation, so maintaining a balanced approach to insertion and deletion is essential.

Common Errors with Set Erase
Trying to Erase Non-Existent Elements
If you attempt to erase an element that does not exist in the set, the operation completes with no effect. The return value will indicate no change, but errors in logic may stem from assuming deletion would cause a modification.
Mixing Up Iterators and Values
Always ensure you handle iterators correctly, especially not using an iterator that has been invalidated after an erase operation.
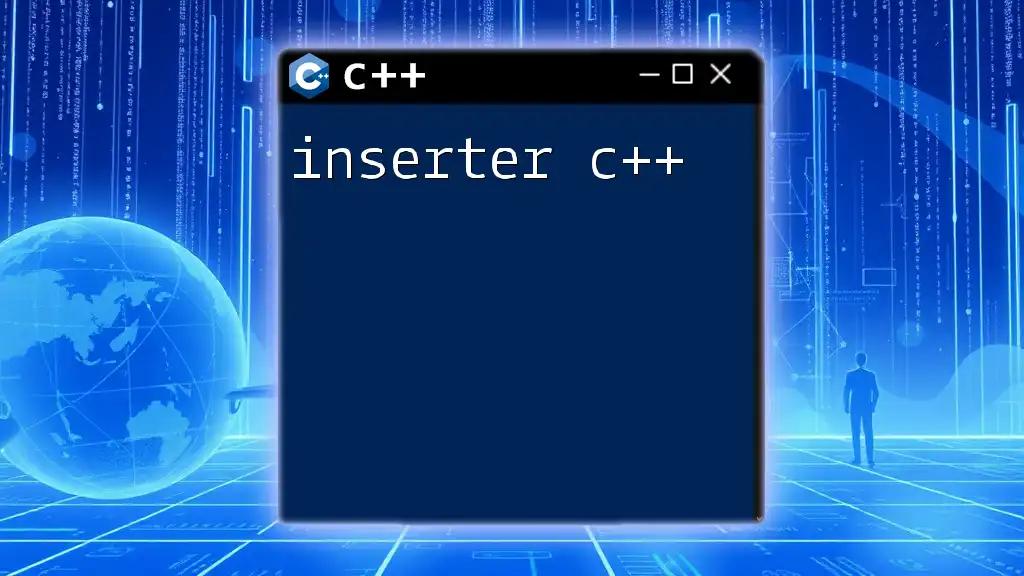
Best Practices for Using Set Erase
- Timing of Erase Operations: Research when to best perform deletions; grouping many operations may lead to better performance than individual continuous calls.
- Maintain Set Integrity: Regularly check the state of your set after major modifications to avoid logic errors in your application.
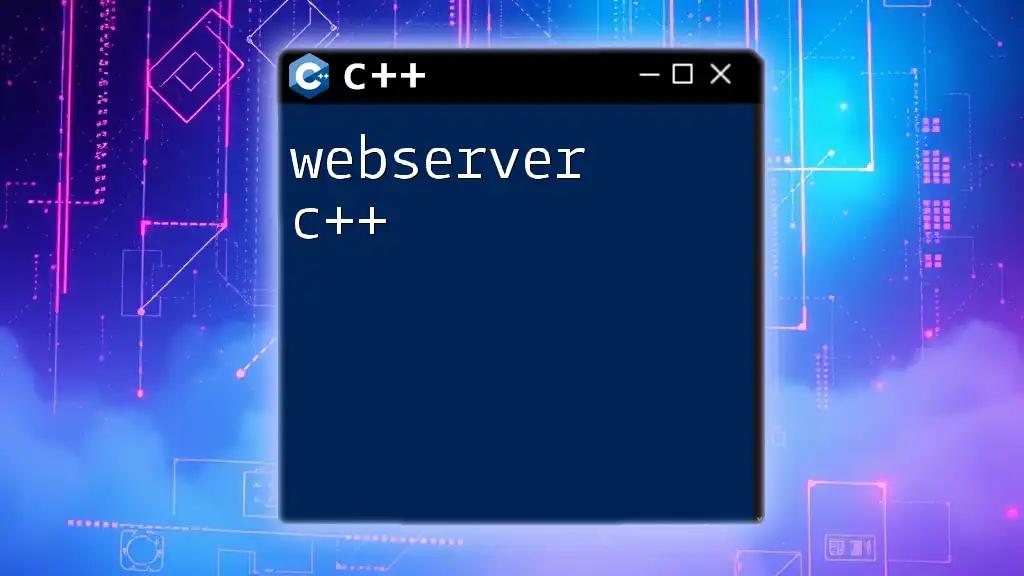
Recap of Key Points
In summary, the `set erase` functionality in C++ allows for elegant and effective management of unique elements, letting you handle data dynamically. Understanding its operation—from syntax to efficiency and potential pitfalls—is vital for exploiting the full capability of sets.

Final Thoughts
Experimenting with the `erase` function provides valuable insight into managing the contents of a set in C++. Play with various scenarios and data sets to become proficient in this operation and understand the role it plays in larger data management strategies.

Frequently Asked Questions (FAQs)
Can I erase all elements in a set?
Yes, you can use the `clear()` method to remove all elements from a set.
Is the erase operation safe with respect to iterators?
If an iterator is removed by the erase operation, it becomes invalidated. Always ensure you retrieve a valid iterator before erasing it.
How does `erase` impact memory management in C++ sets?
Memory is managed automatically, but removing elements causes reallocations which may affect performance. Regularly review your code’s complexity, especially in memory-intensive scenarios.
By understanding and effectively utilizing the `set erase c++` capabilities, you can maintain cleaner, more efficient, and more dynamic data structures in your applications.