A binary search tree (BST) in C++ is a data structure that maintains sorted data for efficient insertion, deletion, and lookup operations.
Here's a simple example of a BST implementation in C++:
#include <iostream>
using namespace std;
struct Node {
int data;
Node* left;
Node* right;
};
Node* newNode(int data) {
Node* node = new Node();
node->data = data;
node->left = node->right = nullptr;
return node;
}
Node* insert(Node* root, int data) {
if (!root) return newNode(data);
if (data < root->data) root->left = insert(root->left, data);
else root->right = insert(root->right, data);
return root;
}
void inorder(Node* root) {
if (root) {
inorder(root->left);
cout << root->data << " ";
inorder(root->right);
}
}
int main() {
Node* root = nullptr;
root = insert(root, 5);
insert(root, 3);
insert(root, 8);
inorder(root); // Output: 3 5 8
return 0;
}
What is a Binary Search Tree?
A Binary Search Tree (BST) is a special kind of binary tree where each node includes a key, and the keys in the left subtree are less than the node’s key, while keys in the right subtree are greater. This property allows for efficient searching, inserting, and deleting of nodes, making it an essential structure for many algorithms and applications.
Benefits of Using BST in C++
Using a BST in C++ offers several advantages, notably:
- Efficiency: Operations such as searching, inserting, and deleting nodes can be performed in average time complexity of O(log n), which is significantly faster than linear search methods such as arrays.
- Dynamic Data Management: Unlike static structures like arrays, BSTs allow dynamic memory allocation, giving flexibility to manage data of varying sizes.
- Ordered Data: BST inherently maintains the ordering of keys, facilitating easier implementation of sorted data retrieval methods.
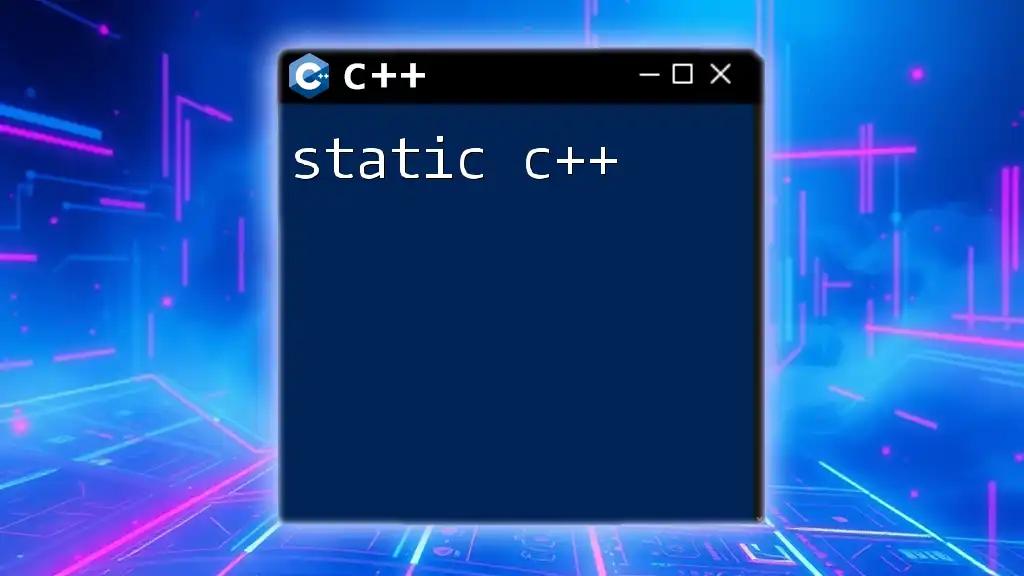
Understanding BST Terminology
Understanding the basic terminology of BSTs is crucial for mastering their use:
- Node: The basic unit of a BST that contains the key, and pointers to left and right child nodes.
- Root: The topmost node of the BST from which all elements are descended.
- Leaves: Nodes that do not have any children, located at the bottom of the tree.
- Height: The number of edges on the longest path from the root to a leaf.
- Depth: The number of edges from the node to the root.
Balanced vs. Unbalanced Trees
A balanced BST features equal height for all leaves, which ensures operations remain efficient. Conversely, an unbalanced tree risks degrading performance to O(n) in the worst case, resembling a linked list.

Implementing a Basic BST in C++
To implement a BST tree in C++, you'll want to first set up your development environment, which can be achieved through various IDEs like Visual Studio or Code::Blocks. Here’s how you can implement a fundamental BST:
Basic Structure of a Node in C++
Defining the basic structure of a node sets the foundation for the BST:
struct Node {
int key;
Node* left;
Node* right;
};
In this structure, each `Node` contains an integer key and pointers to the left and right children.
Creating a BST Class
Next, you’ll create a `BST` class that defines the behavior of your tree.
class BST {
Node* root;
void insert(Node*& node, int key);
public:
BST();
void insert(int key);
};
Here, the `BST` class holds a pointer to the root node. The `insert` function will be public to allow for external access while the actual insertion logic remains encapsulated.

Core Operations of BST in C++
A BST supports three fundamental operations: insertion, searching, and deletion.
Insertion Operation
Inserting elements in a BST involves comparing the keys and placing them accordingly:
void BST::insert(int key) {
insert(root, key);
}
void BST::insert(Node*& node, int key) {
if (node == nullptr) {
node = new Node{key, nullptr, nullptr};
return;
}
if (key < node->key) insert(node->left, key);
else insert(node->right, key);
}
In this insertion process, if the current node is `nullptr`, a new `Node` is created. If the key to be inserted is less than the current node's key, the process recurses down the left subtree; otherwise, it goes down the right subtree.
Searching in BST
Searching for a key in a BST uses a simple logic:
Node* search(Node* node, int key) {
if (node == nullptr || node->key == key)
return node;
if (key < node->key)
return search(node->left, key);
return search(node->right, key);
}
This method traverses the tree based on comparisons with the current node's key, returning the node if found or `nullptr` if the key doesn’t exist.
Deletion Operation
Deletion can be more complex since there are three scenarios to handle:
- The node to be deleted is a leaf (no children).
- The node has one child.
- The node has two children.
Here’s how deletion is handled:
Node* deleteNode(Node* root, int key) {
if (root == nullptr) return root;
if (key < root->key) root->left = deleteNode(root->left, key);
else if (key > root->key) root->right = deleteNode(root->right, key);
else {
if (root->left == nullptr) return root->right;
else if (root->right == nullptr) return root->left;
Node* temp = minValueNode(root->right);
root->key = temp->key;
root->right = deleteNode(root->right, temp->key);
}
return root;
}
Here, if the node matches the key, we determine its children. If both children exist, we find the node's in-order successor to replace the key. This seamless approach helps maintain the BST property.
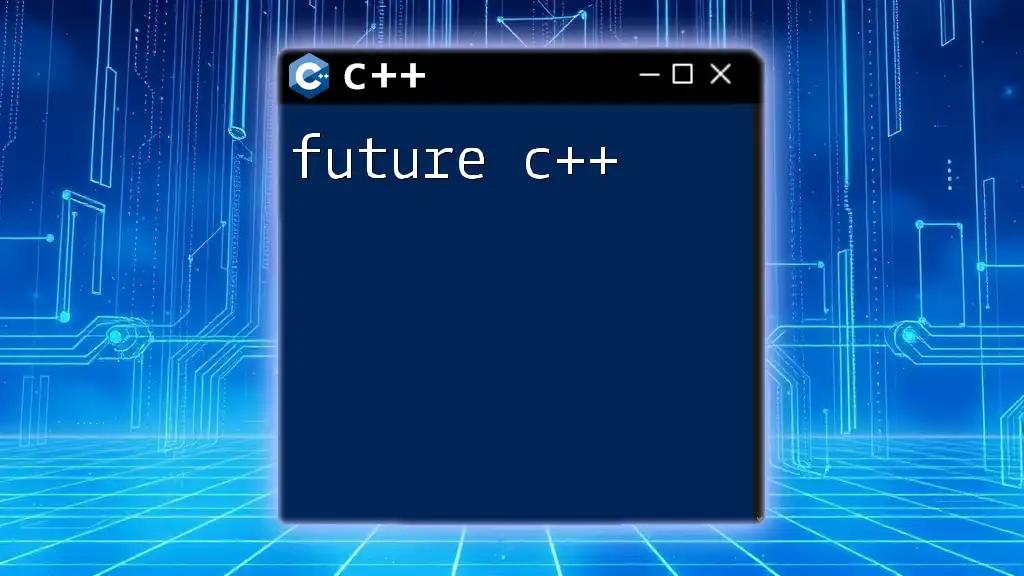
Advanced BST Concepts
Balanced vs. Unbalanced BST
Maintaining a balanced BST is crucial for ensuring performance. Balanced BSTs such as AVL or Red-Black trees ensure that the height differences between the left and right subtrees are minimized, which guarantees O(log n) operations even in the worst case.
Traversal Methods
Traversal helps in visiting nodes in a specific order. Common traversal methods include:
-
In-order Traversal: Visits nodes in ascending order.
void inOrder(Node* node) { if (node == nullptr) return; inOrder(node->left); std::cout << node->key << " "; inOrder(node->right); }
-
Pre-order Traversal: Visits the root first, then left and right subtrees.
-
Post-order Traversal: Visits left and right subtrees before the root.
Each traversal has specific use cases, such as generating sorted output or enabling structured data processing.

Applications of Binary Search Trees in C++
Binary Search Trees find utility in several applications, including:
- Databases: For managing sorted data with efficient searching capabilities.
- Memory Management: Allocating memory blocks and efficiently tracking free blocks.
- Auto-sorting Functionalities: BSTs facilitate quick data organization for search functionalities in applications like searches and dictionary implementations.
Real-world Examples
Large organizations utilize BST in their backend systems for optimized data querying and sorting technologies, enabling rapid data retrieval and modification.
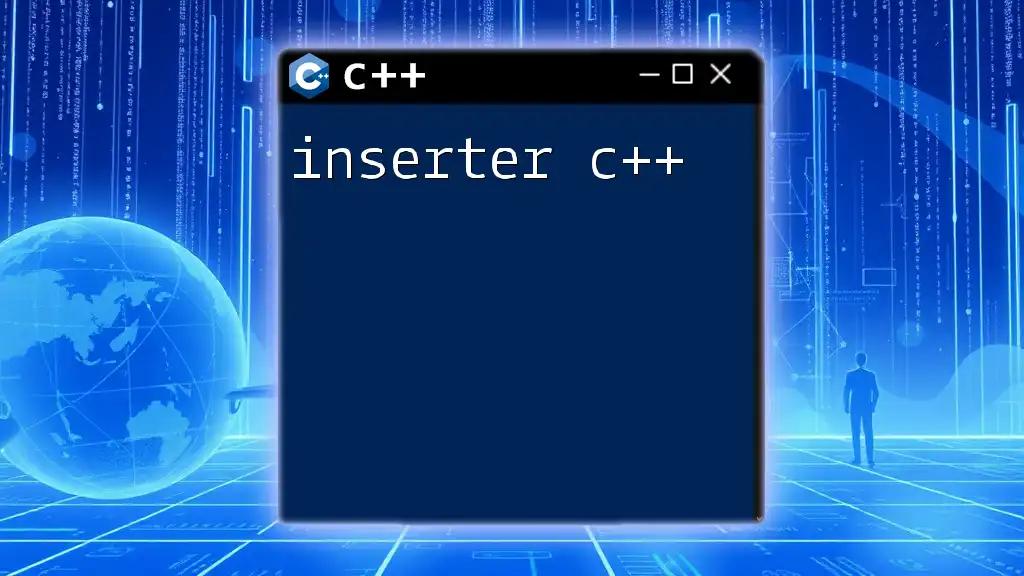
Troubleshooting Common Issues in BST Implementation
While working with BSTs, developers may encounter issues such as improper node linking or inefficient traversals. Debugging can be facilitated by carefully visualizing the tree structure at each operation step.
Performance Bottlenecks
Monitoring run-time complexities is crucial. In unbalanced trees, operations could degenerate to O(n), resembling a linked list. Regular rotations or rebalancing can help maintain optimal performance.
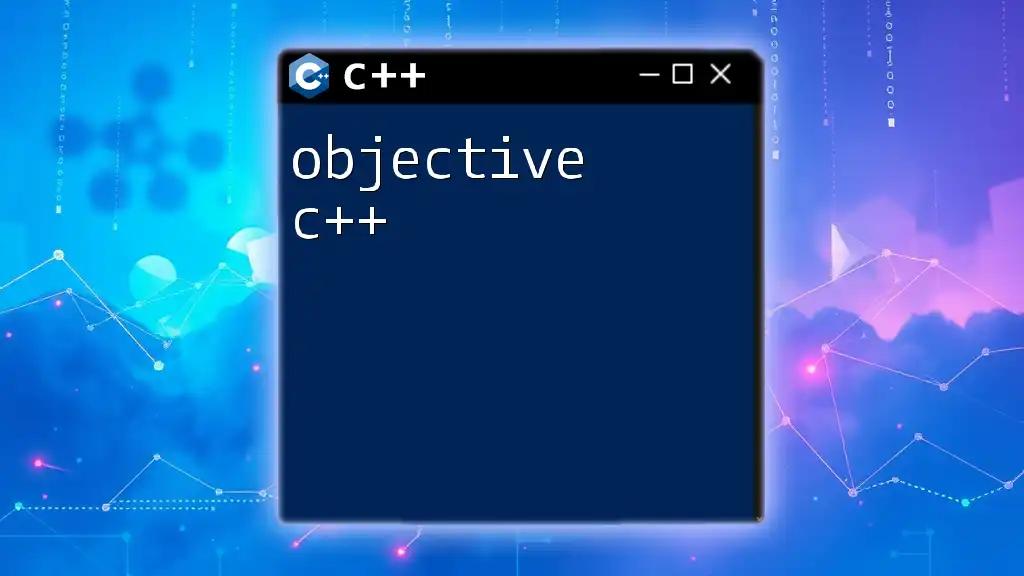
Conclusion
Understanding BST tree in C++ empowers developers to implement data handling structures that are efficient and flexible. Mastering fundamental operations enables further exploration of advanced tree structures, leading to more powerful data management techniques in various applications. For anyone looking to enhance their programming skills, the opportunities with BST are vast and invaluable.

References
For those eager to dive deeper into the world of BSTs and C++, consider exploring recommended books, online tutorials, and official C++ documentation to further expand your knowledge.