A red-black tree is a balanced binary search tree that ensures efficient insertion, deletion, and lookup operations by maintaining specific properties regarding the coloring of its nodes.
Here's a simple C++ code snippet demonstrating the insertion of a value into a red-black tree:
#include <iostream>
struct Node {
int data;
bool color; // 0 for Red, 1 for Black
Node *left, *right, *parent;
Node(int value) : data(value), color(0), left(nullptr), right(nullptr), parent(nullptr) {}
};
class RedBlackTree {
public:
void insert(int data) {
Node* newNode = new Node(data);
// Insertion logic here (not fully implemented for brevity)
}
// Additional functions like balancing and rotations would be added here
};
int main() {
RedBlackTree tree;
tree.insert(10); // Example usage
return 0;
}
Understanding Red Black Trees in C++
What is a Red Black Tree?
A Red Black Tree is a type of self-balancing binary search tree where each node stores an extra bit for denoting the color of the node, either red or black. This additional information helps ensure that the tree remains approximately balanced, leading to efficient search, insertion, and deletion operations.
A Red Black Tree must satisfy the following properties:
- Each node is either red or black.
- The root is always black.
- Red nodes cannot have red children (i.e., no two red nodes can be adjacent).
- Every path from a node to its descendant NIL nodes has the same number of black nodes.
- NIL nodes are considered black.
These properties help maintain a balance in the tree, ensuring that the longest path from the root to a leaf is no more than twice as long as the shortest such path, thereby guaranteeing O(log n) time complexity for basic operations.
Why Use Red Black Trees?
Red Black Trees are an optimal choice for dynamic data structures due to their balancing characteristics. They offer significant advantages over other data structures like AVL Trees in certain scenarios:
- Faster Insertions and Deletions: While AVL Trees are more rigidly balanced (leading to fewer rotations during searches), Red Black Trees allow a more relaxed balance, facilitating quicker insertion and deletion without extensive rebalancing.
- O(log n) Operations: Both search and modification operations maintain O(log n) time complexity, ensuring efficient data management as the dataset grows.
- Memory Efficiency: The use of the color property allows Red Black Trees to be more memory-efficient when managing nodes.
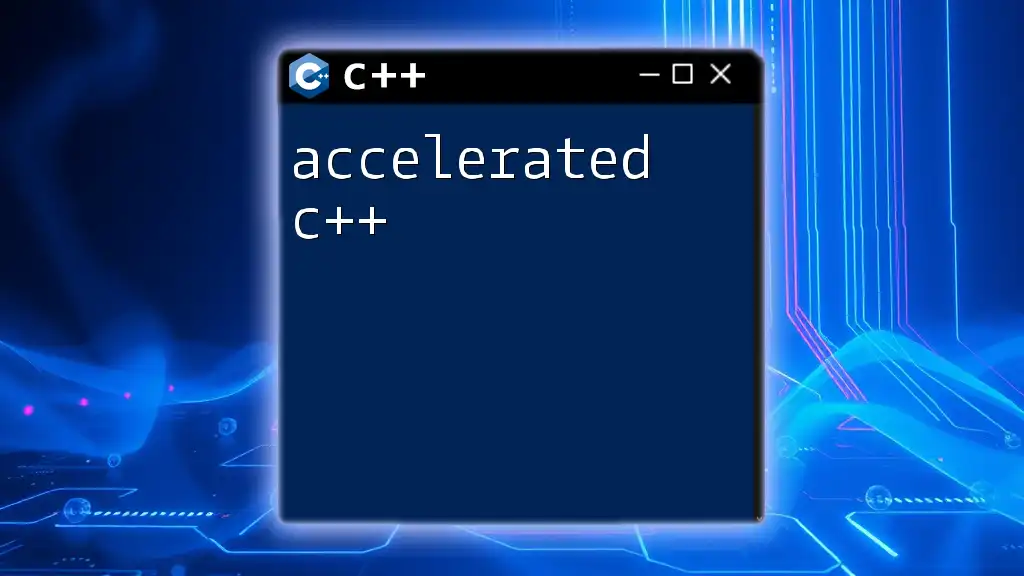
Key Concepts of Red Black Trees
Properties of Red Black Trees
The properties of black red trees are essential for maintaining a balanced structure. Here’s a deeper look at each:
- Node Colors: Each node is either red or black, influencing the tree's balancing mechanism.
- Black Root: The initial node ensures that the tree doesn't start off unbalanced.
- No Red Red Parents: This property aids in maintaining balance by preventing consecutive red nodes.
- Black Height: Equality in black heights across paths ensures that one path isn’t disproportionately longer than another.
- NIL Nodes: Treating these as black helps simplify certain tree operations, especially during rebalancing.
Rotations in Red Black Trees
Rotations are a crucial operation when maintaining the properties of Red Black Trees, allowing nodes to be realigned efficiently.
- Left Rotation: Moves a node down and its right child up to take its place.
- Right Rotation: Moves a node down and its left child up to take its place.
void leftRotate(Node*& root, Node*& pt) {
Node* pt_y = pt->right; // Set pt_y
pt->right = pt_y->left; // Turn pt_y's left subtree into pt's right subtree
if (pt->right != nullptr)
pt->right->parent = pt;
pt_y->parent = pt->parent; // Link pt's parent to pt_y
if (pt->parent == nullptr) {
root = pt_y; // Change root if needed
} else if (pt == pt->parent->left) {
pt->parent->left = pt_y;
} else {
pt->parent->right = pt_y;
}
pt_y->left = pt; // Put pt on pt_y's left
pt->parent = pt_y;
}
Implementing a Red Black Tree in C++
Basic Structure of a Red Black Tree
To effectively implement a black red tree in C++, we need a structure for the nodes, which includes properties for color, value, left and right child pointers, and a parent pointer.
enum Color { RED, BLACK };
struct Node {
int data;
Color color;
Node *left, *right, *parent;
Node(int data) : data(data), color(RED), left(nullptr), right(nullptr), parent(nullptr) {}
};
Insertion in Red Black Trees
Insertion Algorithm
The insertion algorithm involves adding a node like in a standard binary search tree, followed by adjusting the tree to maintain Red Black properties.
Code Example: Inserting a Node
The following code demonstrates how to insert a node into a Red Black Tree while ensuring all properties are preserved.
void fixViolation(Node*& root, Node*& pt) {
Node* parent_pt = nullptr;
Node* grand_parent_pt = nullptr;
while ((pt != root) && (pt->color == RED) && (pt->parent->color == RED)) {
parent_pt = pt->parent;
grand_parent_pt = pt->parent->parent;
if (parent_pt == grand_parent_pt->left) {
Node* uncle_pt = grand_parent_pt->right;
if (uncle_pt != nullptr && uncle_pt->color == RED) {
grand_parent_pt->color = RED;
parent_pt->color = BLACK;
uncle_pt->color = BLACK;
pt = grand_parent_pt;
} else {
if (pt == parent_pt->right) {
leftRotate(root, parent_pt);
pt = parent_pt;
parent_pt = pt->parent;
}
rightRotate(root, grand_parent_pt);
swap(parent_pt->color, grand_parent_pt->color);
pt = parent_pt;
}
} else {
Node* uncle_pt = grand_parent_pt->left;
if ((uncle_pt != nullptr) && (uncle_pt->color == RED)) {
grand_parent_pt->color = RED;
parent_pt->color = BLACK;
uncle_pt->color = BLACK;
pt = grand_parent_pt;
} else {
if (pt == parent_pt->left) {
rightRotate(root, parent_pt);
pt = parent_pt;
parent_pt = pt->parent;
}
leftRotate(root, grand_parent_pt);
swap(parent_pt->color, grand_parent_pt->color);
pt = parent_pt;
}
}
}
root->color = BLACK;
}
Deletion in Red Black Trees
Deletion Algorithm
Deleting from a Red Black Tree requires first performing a standard BST deletion followed by rebalancing the tree as necessary.
Code Example: Deleting a Node
Here is an example of how to delete a node while ensuring the properties of the tree are preserved:
void deleteNode(Node*& root, int data) {
Node* v = BSTDelete(root, data);
if (v == nullptr) return;
Node* u = v->parent;
Color uOriginalColor = u->color;
// Further reductions will go here to balance the tree as necessary
}
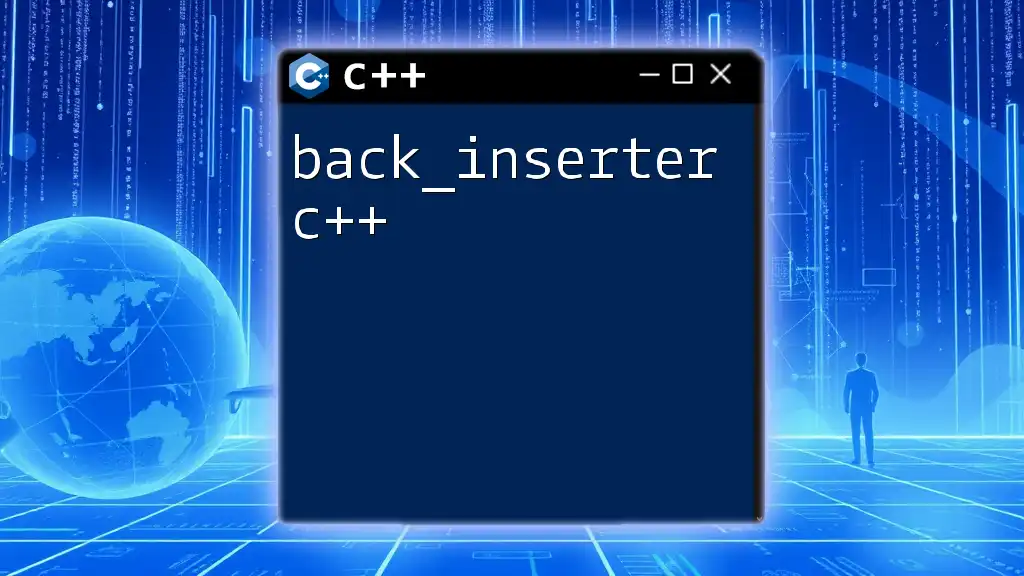
Traversing a Red Black Tree
Common Traversal Methods
Traversal methods, such as in-order, pre-order, and post-order, can be employed to retrieve data from the tree systematically.
Code Example: Traversal Implementations
Implementing these traversal methods can enhance how we manage and obtain data:
void inOrder(Node* root) {
if (root == nullptr) return;
inOrder(root->left);
std::cout << root->data << " ";
inOrder(root->right);
}
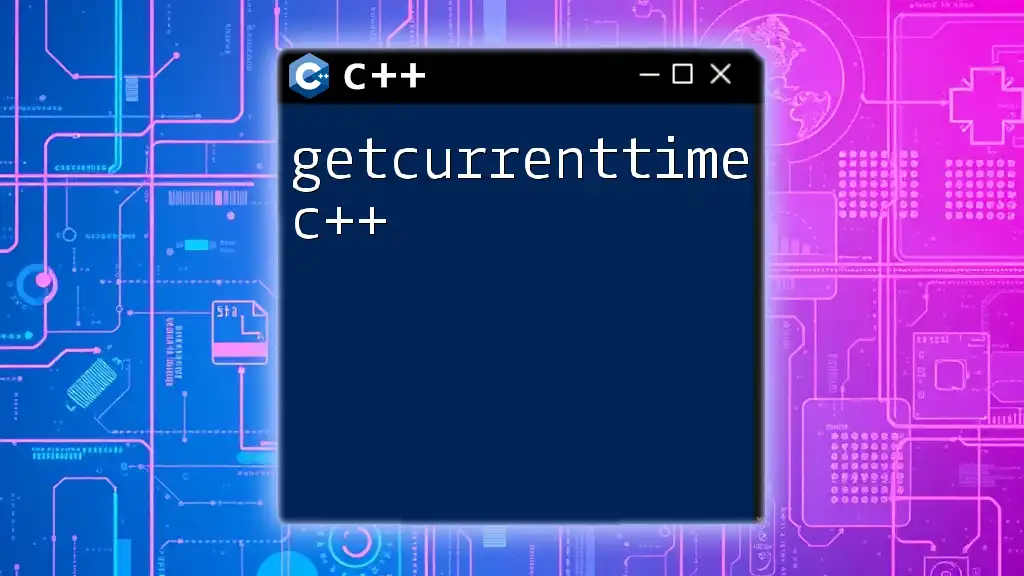
Real-World Applications of Red Black Trees
Use Cases
Red Black Trees find extensive use in many real-world applications, including:
- Databases: They facilitate efficient sorting and searching.
- Associative Arrays: Useful in situations where orders and value mappings are essential.
Comparison with Other Data Structures
When compared to other data structures like AVL Trees or simple Binary Search Trees, red black trees often provide a balance between faster modifications and read operations.
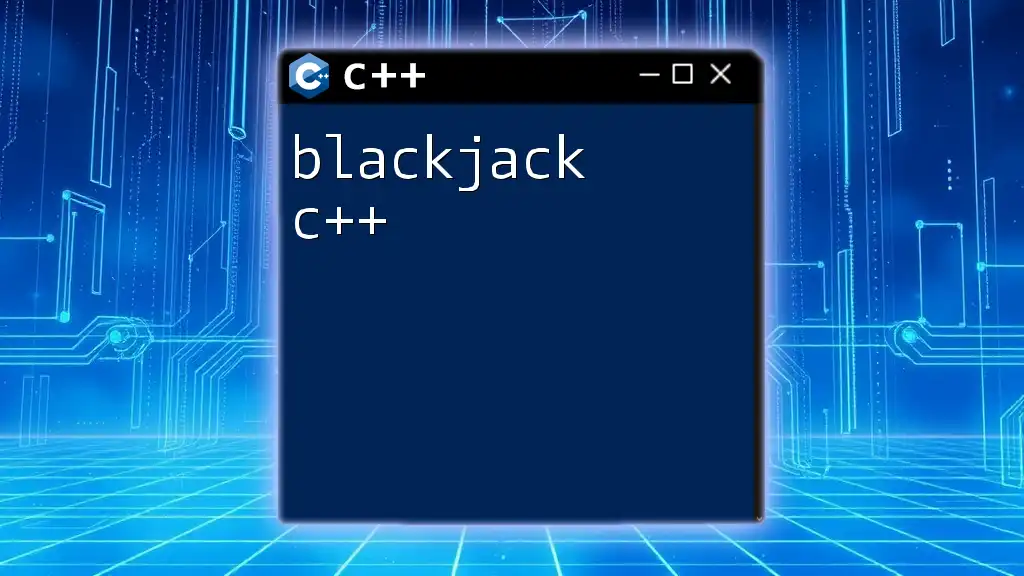
Conclusion
The black red tree in C++ is a powerful data structure that offers efficient data management and balanced operations. Its ability to maintain order while allowing for dynamic updates makes it invaluable in various applications. Practicing its implementation can greatly enhance your programming capabilities and data structure knowledge.
For those interested in exploring further, various resources are available to deepen your understanding and mastery of red black trees in C++. Be sure to combine practical implementation with theoretical knowledge for the best outcomes!