A Huffman tree is a binary tree used in Huffman coding for lossless data compression, where each leaf node represents a character and its frequency, allowing for efficient encoding of data.
Here's a simple implementation of a Huffman tree in C++:
#include <iostream>
#include <queue>
#include <unordered_map>
struct Node {
char data;
int freq;
Node *left, *right;
Node(char d, int f) : data(d), freq(f), left(nullptr), right(nullptr) {}
};
// Comparator for priority queue
struct Compare {
bool operator()(Node* l, Node* r) {
return l->freq > r->freq;
}
};
// Function to build the Huffman tree
Node* buildHuffmanTree(const std::unordered_map<char, int>& freqMap) {
std::priority_queue<Node*, std::vector<Node*>, Compare> minHeap;
for (const auto& pair : freqMap) {
minHeap.push(new Node(pair.first, pair.second));
}
while (minHeap.size() > 1) {
Node* left = minHeap.top(); minHeap.pop();
Node* right = minHeap.top(); minHeap.pop();
Node* merged = new Node('\0', left->freq + right->freq);
merged->left = left;
merged->right = right;
minHeap.push(merged);
}
return minHeap.top(); // Root of the Huffman tree
}
This code snippet demonstrates how to build a Huffman tree from a frequency map of characters.
Overview of the Huffman Algorithm
Huffman coding is a widely used method for data compression that utilizes a specific algorithm to create a binary tree known as a Huffman Tree. The principle behind Huffman coding is simple yet effective: it assigns variable-length codes to input characters, where shorter codes are assigned to more frequent characters. This method is particularly advantageous when reducing the size of data files.
How Huffman Coding Works
The Huffman coding process can be broken down into several key steps:
-
Frequency Analysis: The first step involves counting how often each character appears in the input data to create a frequency table. This frequency data is crucial for constructing the Huffman Tree.
-
Generating the Huffman Tree: Once the frequency table is ready, the algorithm builds the Huffman Tree using a priority queue. The least frequent characters are placed at the leaves of the tree, while more frequent characters are placed closer to the root.
-
Assigning Codes: Each character in the input data gets a unique code based on its position in the Huffman Tree. A left edge might represent a '0', while a right edge might represent a '1', creating a binary representation for each character.
-
Encoding Data: The next step is encoding the input data using the generated codes, which leads to a compressed representation of the original data.
-
Decoding Data: Finally, to retrieve the original data, the encoded data must be decoded by traversing the Huffman Tree back to the leaves.
Building a Huffman Tree in C++
To implement Huffman coding effectively, it’s important to understand the structure of the Huffman Tree. Each node in this tree represents a character and its associated frequency. The following is a basic representation of a node class in C++ that will be used to build the tree.
struct Node {
char data;
int frequency;
Node *left;
Node *right;
Node(char data, int frequency) {
this->data = data;
this->frequency = frequency;
left = right = nullptr;
}
};
Creating the Frequency Table
The frequency table is essential as it holds the frequency count of each character in the input string. This count informs how the tree will be structured as characters with higher frequencies will be placed closer to the root of the tree. You can create the frequency table with the following code snippet:
std::unordered_map<char, int> createFrequencyTable(const std::string& data) {
std::unordered_map<char, int> frequency;
for (char ch : data) {
frequency[ch]++;
}
return frequency;
}
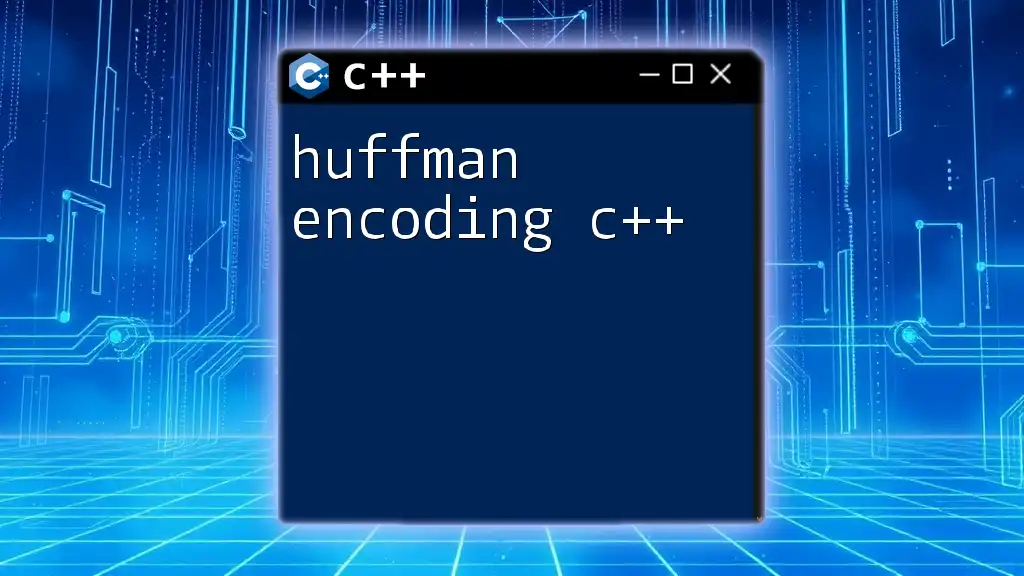
Implementing the Huffman Algorithm in C++
Building the Priority Queue
A priority queue is crucial to efficiently construct the Huffman Tree. It allows us to retrieve the node with the smallest frequency at each step of the algorithm. In C++, we can utilize `std::priority_queue` to achieve this:
auto cmp = [](Node* left, Node* right) { return left->frequency > right->frequency; };
std::priority_queue<Node*, std::vector<Node*>, decltype(cmp)> minHeap(cmp);
Constructing the Huffman Tree
The core of Huffman coding involves constructing the tree using the priority queue. The following code outlines the process of building the Huffman Tree:
Node* buildHuffmanTree(const std::unordered_map<char, int>& frequency) {
std::priority_queue<Node*, std::vector<Node*>, decltype(cmp)> minHeap(cmp);
for (const auto& pair : frequency) {
minHeap.push(new Node(pair.first, pair.second));
}
while (minHeap.size() > 1) {
Node* left = minHeap.top(); minHeap.pop();
Node* right = minHeap.top(); minHeap.pop();
Node* newNode = new Node('\0', left->frequency + right->frequency);
newNode->left = left;
newNode->right = right;
minHeap.push(newNode);
}
return minHeap.top();
}
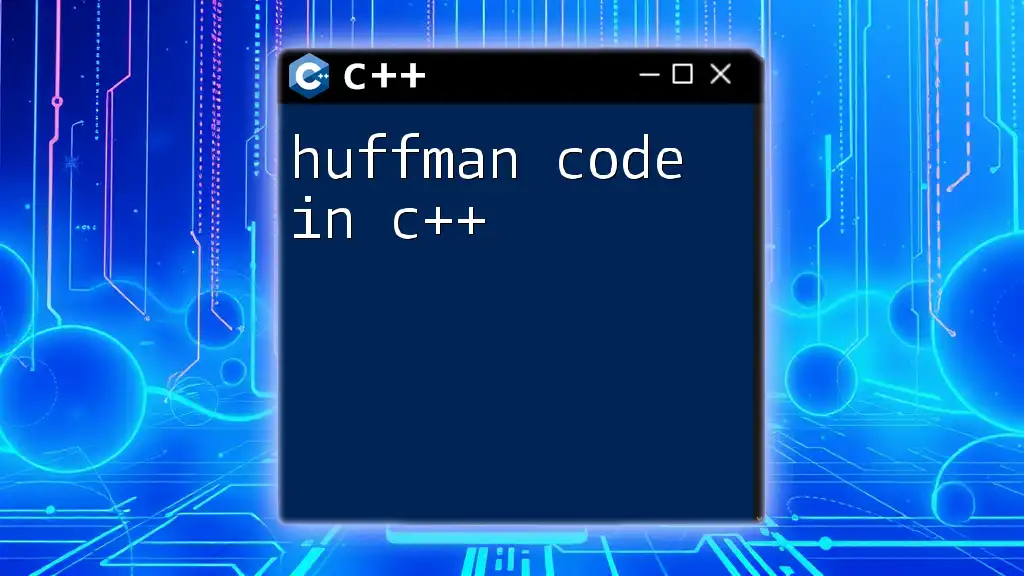
Encoding Data Using the Huffman Tree
Generating Huffman Codes
Once the tree is built, the next step is generating the Huffman codes through a depth-first traversal of the tree. As we traverse, we assign a '0' for left edges and a '1' for right edges. Here's an example of how to generate the codes:
void generateCodes(Node* root, std::string code, std::unordered_map<char, std::string>& huffmanCodes) {
if (!root) return;
if (root->left == nullptr && root->right == nullptr) {
huffmanCodes[root->data] = code;
}
generateCodes(root->left, code + "0", huffmanCodes);
generateCodes(root->right, code + "1", huffmanCodes);
}
Compiling the Full Encoding Process
With the codes generated, it's time to apply them to encode the original string. The following code snippet combines the frequency table, tree construction, and code generation processes into a complete function for encoding:
std::string huffmanCoding(const std::string& data) {
auto frequency = createFrequencyTable(data);
Node* root = buildHuffmanTree(frequency);
std::unordered_map<char, std::string> huffmanCodes;
generateCodes(root, "", huffmanCodes);
std::string encodedString;
for (char ch : data) {
encodedString += huffmanCodes[ch];
}
return encodedString; // Returning encoded string
}
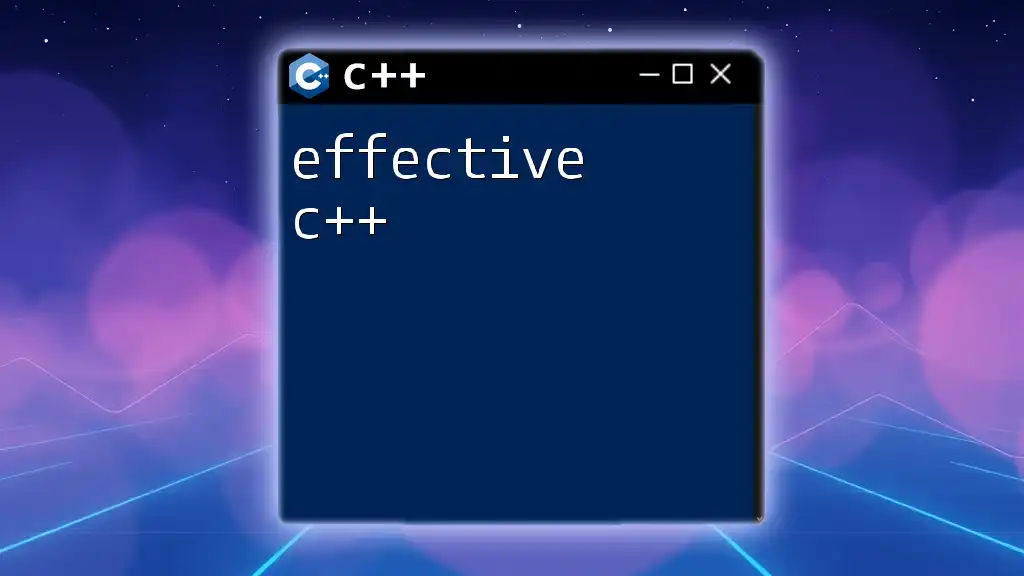
Decoding Huffman Encoded Data
The Decoding Process
Decoding the data involves traversing the Huffman Tree according to the binary code. Each time we hit a leaf node, we can retrieve the original character. Below is a code snippet that implements this decoding process:
std::string decodeData(Node* root, const std::string& encodedString) {
std::string decodedString;
Node* current = root;
for (char bit : encodedString) {
current = (bit == '0') ? current->left : current->right;
if (!current->left && !current->right) {
decodedString += current->data;
current = root;
}
}
return decodedString;
}
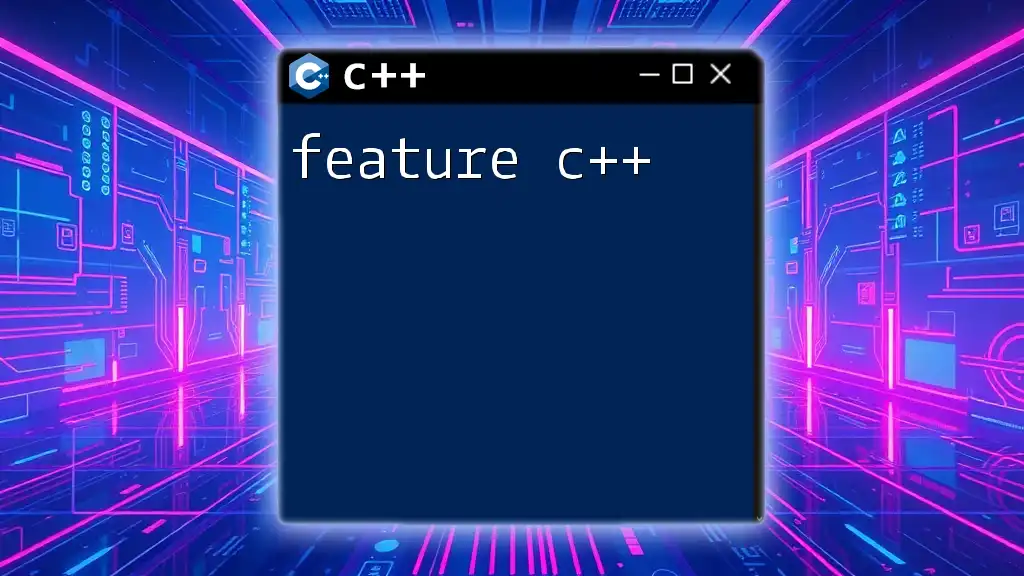
Complete Example of Huffman Coding in C++
To solidify the understanding of the entire process, here’s a complete example that illustrates both encoding and decoding using the Huffman algorithm in C++:
int main() {
std::string data = "Example string for Huffman coding";
// Encode the data
std::string encodedString = huffmanCoding(data);
// Decode the data
Node* root = buildHuffmanTree(createFrequencyTable(data)); // Rebuild tree for decoding
std::string decodedString = decodeData(root, encodedString);
std::cout << "Encoded: " << encodedString << std::endl;
std::cout << "Decoded: " << decodedString << std::endl;
return 0;
}
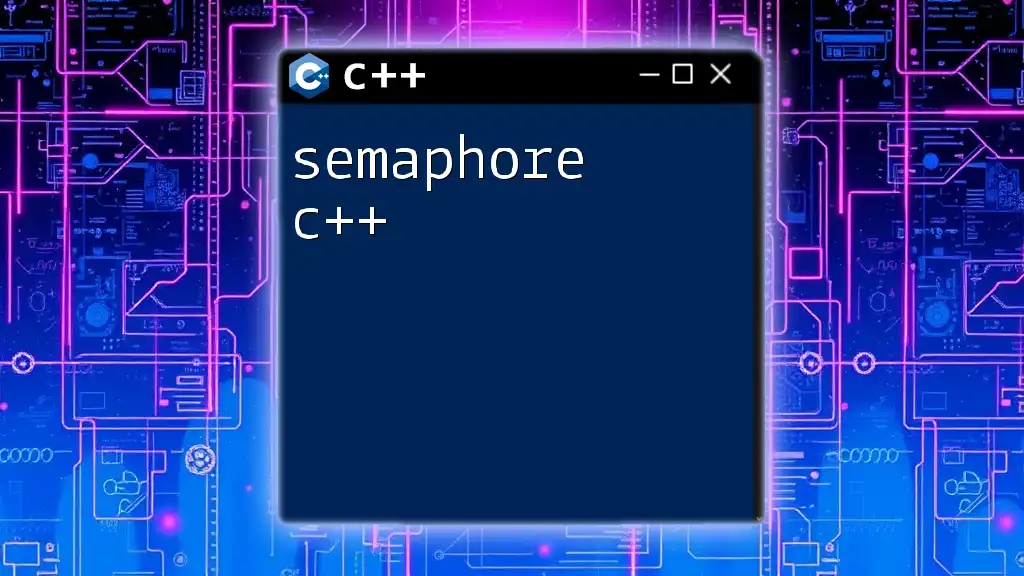
Conclusion
Huffman coding is a powerful method for data compression that utilizes the properties of a binary tree. By understanding how to build a Huffman Tree in C++, along with encoding and decoding processes, developers can efficiently manage and reduce data size for various applications. The versatility of the Huffman algorithm makes it a fundamental concept in computer science and a valuable tool in modern computing.
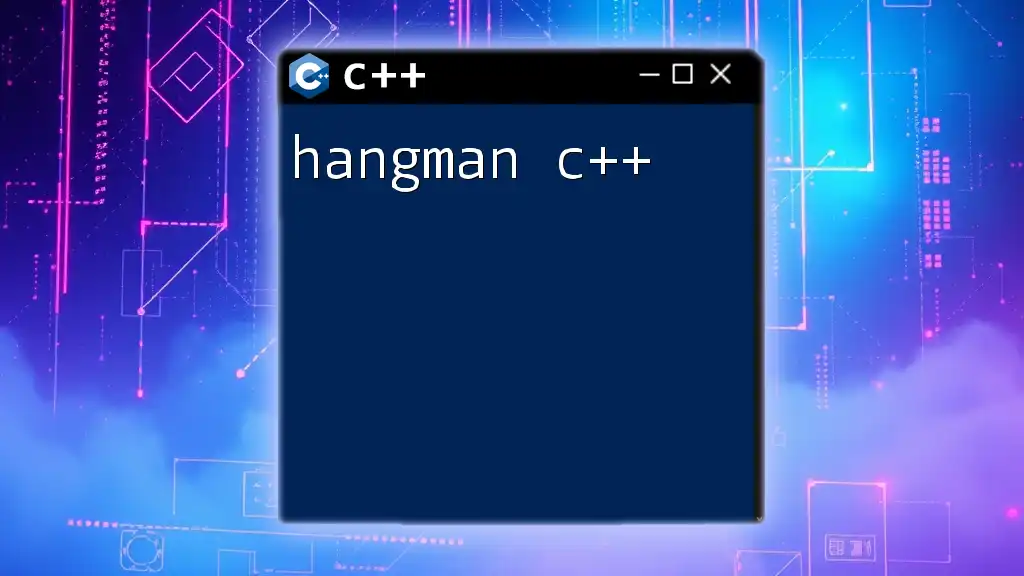
Additional Resources
To delve deeper into Huffman coding and its applications, consider exploring online resources, tutorials, and books that focus on data structures and algorithms in C++. Understanding Huffman coding not only enriches your programming skills but also enhances your ability to work with data efficiently.