Huffman encoding in C++ is a lossless compression algorithm that uses variable-length codes for encoding symbols based on their frequencies, minimizing the total number of bits used.
Here's a simple example of Huffman encoding in C++:
#include <iostream>
#include <vector>
#include <queue>
#include <unordered_map>
using namespace std;
// A node of the Huffman tree
struct Node {
char data;
int freq;
Node *left, *right;
Node(char d, int f) : data(d), freq(f), left(nullptr), right(nullptr) {}
};
// Comparator for the priority queue
struct compare {
bool operator()(Node* l, Node* r) {
return l->freq > r->freq;
}
};
// Function to build the Huffman tree
Node* buildHuffmanTree(const unordered_map<char, int>& frequency) {
priority_queue<Node*, vector<Node*>, compare> minHeap;
for (const auto& pair : frequency) {
minHeap.push(new Node(pair.first, pair.second));
}
while (minHeap.size() != 1) {
Node *left = minHeap.top(); minHeap.pop();
Node *right = minHeap.top(); minHeap.pop();
Node *top = new Node('\0', left->freq + right->freq);
top->left = left;
top->right = right;
minHeap.push(top);
}
return minHeap.top();
}
// Example usage
int main() {
unordered_map<char, int> frequency = {{'a', 5}, {'b', 9}, {'c', 12}, {'d', 13}, {'e', 16}, {'f', 45}};
Node* root = buildHuffmanTree(frequency);
// Further code to generate Huffman codes would go here
return 0;
}
What is Huffman Encoding?
Huffman Encoding is widely regarded as one of the most efficient methods for lossless data compression. The algorithm was created by David A. Huffman in 1952, primarily aimed at reducing the size of data represented in binary form without losing any information. It works by assigning variable-length codes to input characters based on their frequencies of occurrence—frequent characters get shorter codes, while less frequent ones get longer codes.
Importance of Huffman Encoding in Lossless Compression
In the realm of data compression, achieving the smallest file size possible without sacrificing data integrity is crucial. Huffman Encoding stands out in this regard, as it optimally compresses data while ensuring that the original information can be perfectly reconstructed.
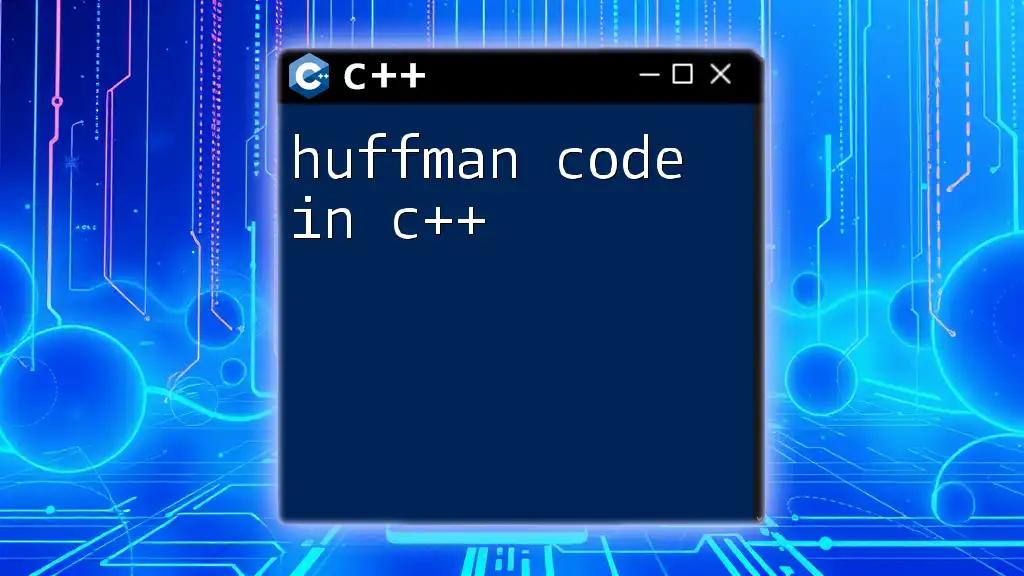
Understanding the Concept of Huffman Encoding
How Huffman Encoding Works
Huffman Encoding involves several fundamental steps:
-
Frequency Analysis: The algorithm begins with a frequency analysis of the characters in the input data. Each unique character's frequency is counted.
-
Construction of Huffman Trees: Based on these frequency counts, a binary tree known as a Huffman Tree is built. Each leaf node in the tree represents a character, and its path from the root determines the unique binary code assigned to that character.
-
Building the Codebook: Once the tree is built, you traverse the tree to generate a mapping of characters to their corresponding binary codes, forming what is known as a codebook.
Benefits of Huffman Encoding
The greatest advantage of Huffman Encoding is its efficiency. It typically results in smaller compressed file sizes compared to other common encoding methods, such as fixed-length encoding schemes.
Moreover, because Huffman Encoding is based on character frequencies, it adapts to different datasets, providing tailored compression that can lead to greater reductions in file size.
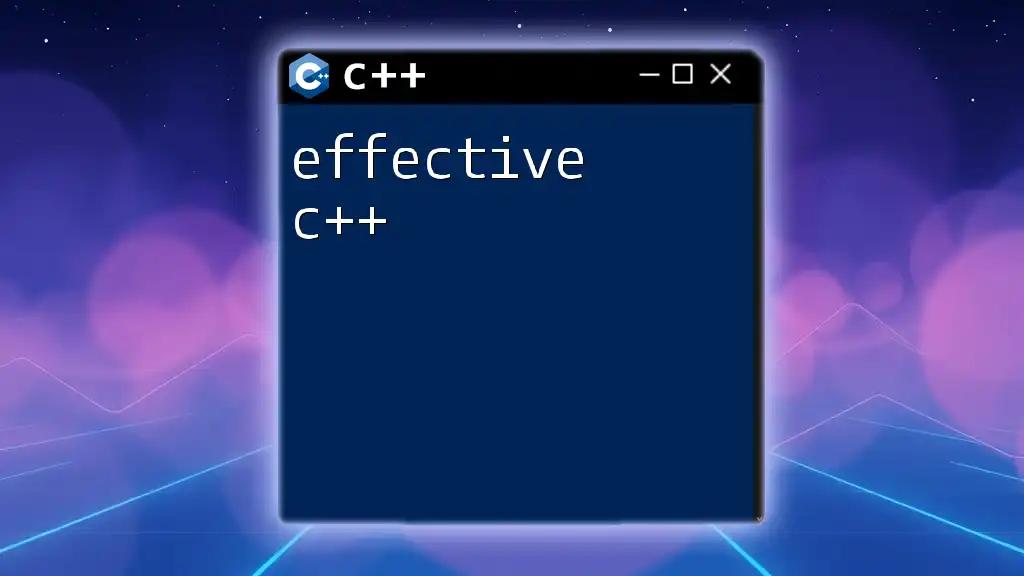
Implementing Huffman Encoding in C++
When it comes to Huffman encoding in C++, creating a robust implementation requires a systematic approach. Below we will break down the code structure, starting from defining necessary classes and functions.
Setting Up the Environment
Before diving into the code, ensure that you have a C++ development environment set up. Basic tools could include a code editor (such as Visual Studio Code) and a C++ compiler like GCC or Clang.
Code Structure for Huffman Encoding
A comprehensive implementation of Huffman Encoding will require several components:
- Node Structure for the Tree: This will represent each character and its associated frequency in the Huffman Tree.
- Priority Queue for Building the Tree: A priority queue is essential for constructing the Huffman Tree, facilitating the extraction of the two least frequent characters.
Defining the Node Class
At the heart of our implementation is the `Node` class, which defines each leaf in the Huffman Tree:
class Node {
public:
char character;
int frequency;
Node* left;
Node* right;
};
This class includes:
- `character`: The actual character being represented.
- `frequency`: The frequency of the character in the input string.
- Pointers to `left` and `right`: These will point to the left and right children when forming the tree.
Building the Frequency Table
To create our Huffman Tree, we first need a frequency table of characters found in the input data. This can be accomplished with the following function:
std::unordered_map<char, int> frequencyTable(const std::string &text) {
std::unordered_map<char, int> freqs;
for (char c : text) {
freqs[c]++;
}
return freqs;
}
This function iterates through the input string, counting the occurrences of each character and storing them in an unordered map.
Constructing the Huffman Tree
With the frequency table in hand, we can now build our Huffman Tree. The following function demonstrates this:
Node* buildHuffmanTree(const std::unordered_map<char, int>& freq) {
auto cmp = [](Node* left, Node* right) { return left->frequency > right->frequency; };
std::priority_queue<Node*, std::vector<Node*>, decltype(cmp)> pq(cmp);
for (auto pair : freq) {
Node* node = new Node{pair.first, pair.second, nullptr, nullptr};
pq.push(node);
}
while (pq.size() > 1) {
Node* left = pq.top(); pq.pop();
Node* right = pq.top(); pq.pop();
Node* merge = new Node{'#', left->frequency + right->frequency, left, right};
pq.push(merge);
}
return pq.top();
}
In this function, a priority queue is utilized to efficiently arrange the characters according to their frequencies. The two nodes with the lowest frequencies are repeatedly merged to build the Huffman Tree until only one node remains.
Generating Huffman Codes
With the Huffman Tree constructed, the next step is generating the codes for each character. This is accomplished using a recursive function:
void generateCodes(Node* root, const std::string &code, std::unordered_map<char, std::string> &codebook) {
if (!root) return;
// Leaf node
if (!root->left && !root->right) {
codebook[root->character] = code;
}
generateCodes(root->left, code + "0", codebook);
generateCodes(root->right, code + "1", codebook);
}
This function takes a `Node` representing the root of the Huffman Tree, a `code` string to accumulate codes, and a `codebook` to store the final mappings of characters to their codes. As you traverse down to the leaves, “0” and “1” are appended to the code based on direction in the tree, forming the binary representation in the codebook.
Final Codebook Example
Supposing an input string "hello," the Huffman encoding might produce a codebook something like this:
{
'h': "00",
'e': "01",
'l': "10",
'o': "11"
}
Characters are assigned binary codes based on their relative frequencies.
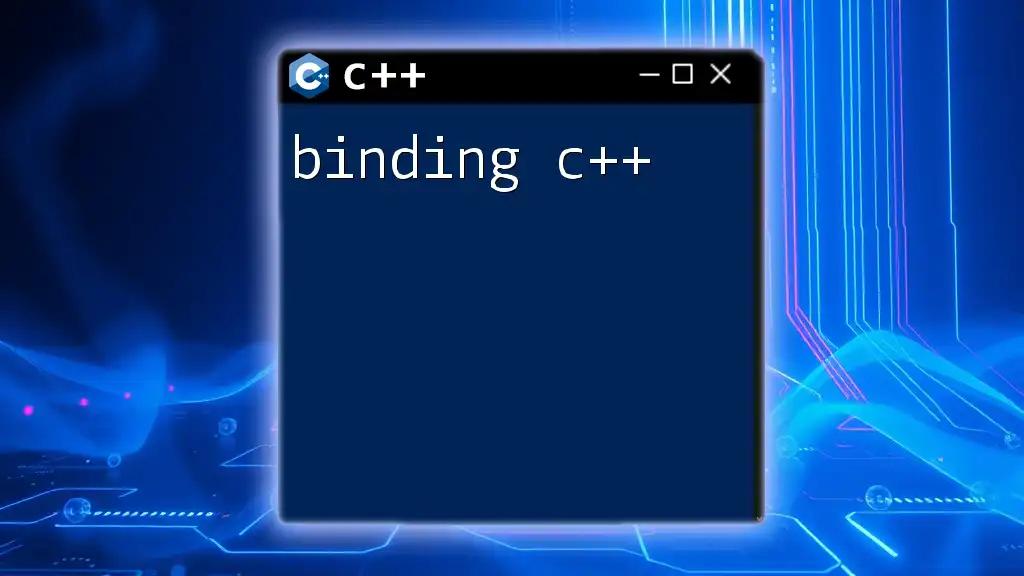
Encoding and Decoding Process
Encoding a Text Using Huffman Codes
The encoding process requires transforming the original text into its encoded binary representation, making use of the generated codebook:
std::string encode(const std::string &text, const std::unordered_map<char, std::string> &codebook) {
std::string encoded = "";
for (char c : text) {
encoded += codebook.at(c);
}
return encoded;
}
This function creates a new string by concatenating the binary codes for each character from the input text based on the codebook.
Decoding the Encoded Text
To retrieve the original message, a decoding function is necessary:
std::string decode(const std::string &encodedText, Node* root) {
std::string decoded = "";
Node* curr = root;
for (char bit : encodedText) {
curr = (bit == '0') ? curr->left : curr->right;
if (!curr->left && !curr->right) { // leaf node
decoded += curr->character;
curr = root; // go back to root for the next character
}
}
return decoded;
}
In this function, you traverse the Huffman Tree according to the bits in the encoded string, and once a leaf node is reached, the corresponding character is added to the decoded string.
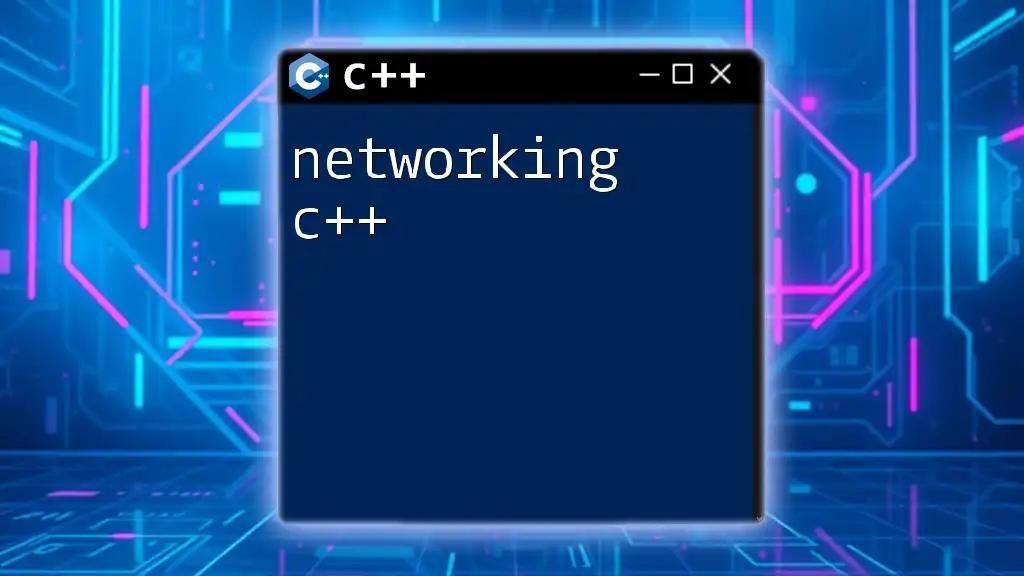
Example of Huffman Encoding in Action
Below is a complete example that unites all elements for a functional Huffman Encoding in C++ application:
#include <iostream>
#include <unordered_map>
#include <queue>
#include <string>
class Node {
public:
char character;
int frequency;
Node* left;
Node* right;
};
// ... (Include all the discussed functions here)
int main() {
std::string text = "hello";
std::unordered_map<char, int> freq = frequencyTable(text);
Node* root = buildHuffmanTree(freq);
std::unordered_map<char, std::string> codebook;
generateCodes(root, "", codebook);
std::string encodedText = encode(text, codebook);
std::string decodedText = decode(encodedText, root);
std::cout << "Original text: " << text << std::endl;
std::cout << "Encoded text: " << encodedText << std::endl;
std::cout << "Decoded text: " << decodedText << std::endl;
return 0;
}
Testing Huffman Encoding
This implementation serves as a solid test case for verifying the behavior of the algorithm. For the input "hello," the program should yield a smaller encoded string alongside the ability to perfectly reconstruct the original string.
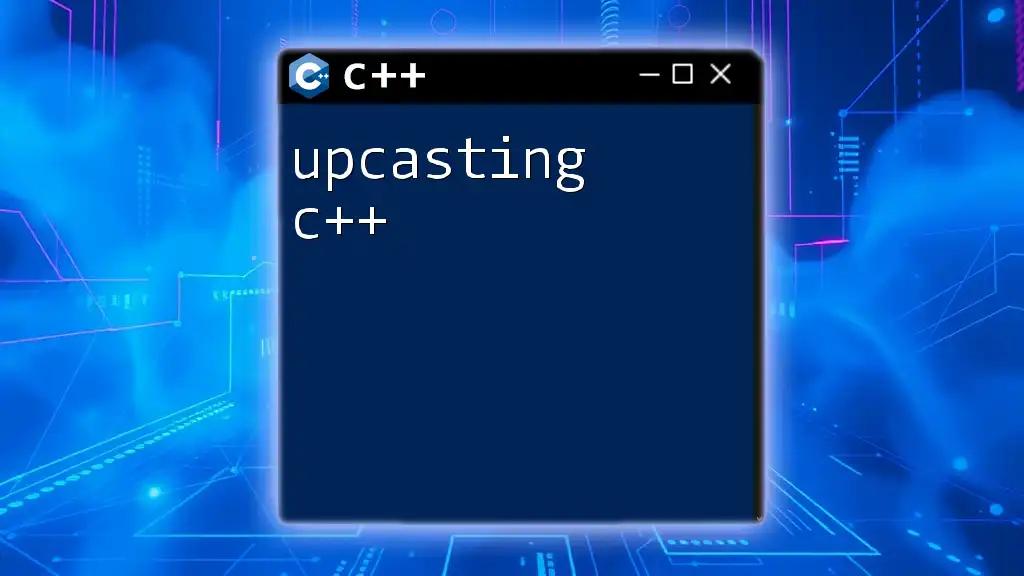
Performance Considerations and Optimizations
Time and Space Complexity
The time complexity for constructing the Huffman Tree is O(n log n), where `n` is the number of unique characters. The generation of codes and encoding text can both be done in O(m) time, where `m` is the length of the text.
Potential Enhancements
For larger datasets, consider implementing more sophisticated data structures or techniques, such as dynamic programming approaches or adopting a more efficient way to manage the priority queue for better performance in specific scenarios.
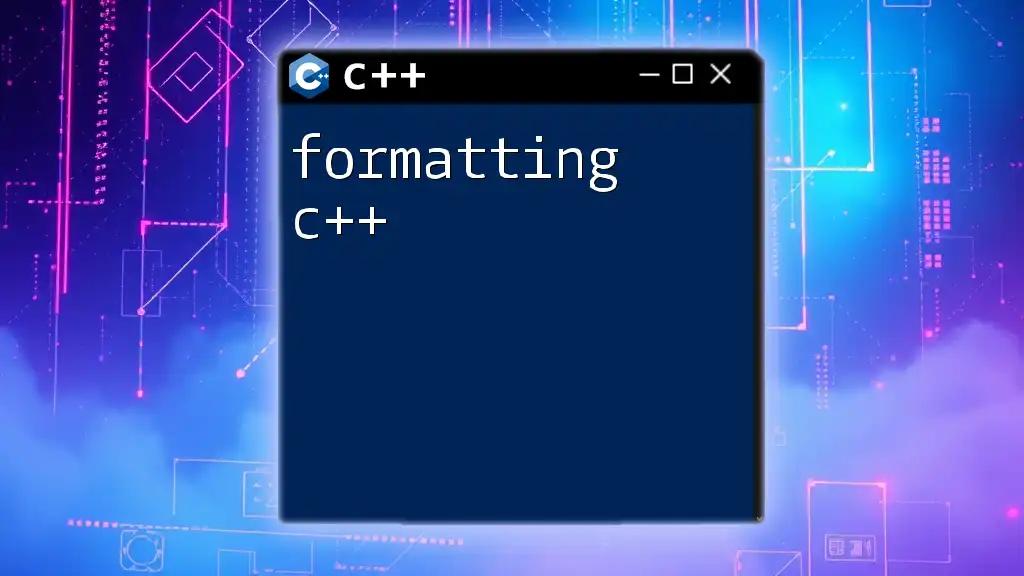
Conclusion
In summary, Huffman Encoding provides an efficient approach to compressing data while maintaining data integrity. The key steps include building a frequency table, constructing the Huffman Tree, and generating the mapping of characters to binary codes. Ultimately, mastering this algorithm can significantly enhance your proficiency in data compression techniques in C++. For those looking to deepen their understanding of C++ and encoding methods, there are many resources available to explore further.