Heap sorting in C++ is an efficient sorting algorithm that uses a binary heap data structure to sort elements in linearithmic time complexity, O(n log n). Here’s a simple implementation:
#include <iostream>
using namespace std;
// Function to heapify a subtree rooted with node i which is an index in arr[].
// n is the size of the heap
void heapify(int arr[], int n, int i) {
int largest = i; // Initialize largest as root
int left = 2 * i + 1; // left = 2*i + 1
int right = 2 * i + 2; // right = 2*i + 2
// If left child is larger than root
if (left < n && arr[left] > arr[largest])
largest = left;
// If right child is larger than largest so far
if (right < n && arr[right] > arr[largest])
largest = right;
// If largest is not root
if (largest != i) {
swap(arr[i], arr[largest]);
heapify(arr, n, largest);
}
}
// Main function to do heap sort
void heapSort(int arr[], int n) {
// Build heap (rearrange array)
for (int i = n / 2 - 1; i >= 0; i--)
heapify(arr, n, i);
// One by one extract an element from heap
for (int i = n - 1; i > 0; i--) {
swap(arr[0], arr[i]); // Move current root to end
heapify(arr, i, 0); // call heapify on the reduced heap
}
}
// Utility function to print an array
void printArray(int arr[], int size) {
for (int i = 0; i < size; i++)
cout << arr[i] << " ";
cout << endl;
}
// Driver code
int main() {
int arr[] = {12, 11, 13, 5, 6, 7};
int n = sizeof(arr) / sizeof(arr[0]);
heapSort(arr, n);
cout << "Sorted array is \n";
printArray(arr, n);
return 0;
}
Understanding the Concepts Behind Heap Sort
What is a Heap?
A heap is a specialized tree-based data structure that satisfies the heap property. There are two main types of heaps:
-
Max-Heap: In this structure, for any given node, the value of that node is greater than or equal to the values of its children. This property makes it easy to retrieve the maximum value.
-
Min-Heap: Here, the value of the node is less than or equal to its children, allowing for efficient extraction of the minimum value.
Visual representation of heaps provides insights into their hierarchical arrangement, where each parent node has its relationships with child nodes defined by their size in relation to one another.
Why Use Heap Sort?
Heap sort is a popular comparison-based sorting algorithm with several advantages:
-
Efficiency: The time complexity of heap sort is O(n log n), making it quite efficient for larger datasets.
-
In-place Sorting: Heap sort does not require additional storage as it sorts the list by rearranging the elements in the original array.
-
Consistent Performance: Unlike other algorithms such as quicksort, heap sort’s performance is not significantly affected by the order of the input data.
However, it’s also important to consider its space complexity, which remains O(1) since no extra space is needed to hold other data during the sorting process.
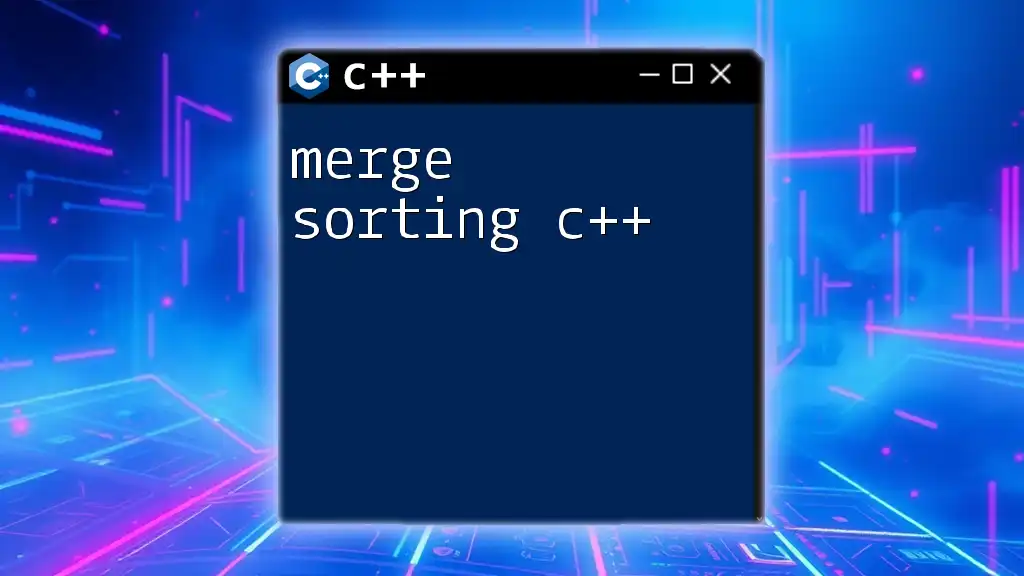
The C++ Heap Sort Algorithm
Steps Involved in Heap Sort
The heap sort algorithm consists of two primary phases:
-
Building the Heap: Convert the unsorted input into a valid heap (typically a max-heap).
-
Extracting the Elements: Repeatedly remove the maximum element from the heap, and rebuild the heap until all elements are sorted.
C++ Code Example for Build Heap
To illustrate the first stage, here is how to build a heap in C++ using a `heapify` function:
void heapify(std::vector<int>& arr, int n, int i) {
int largest = i; // Initialize largest as root
int left = 2 * i + 1; // left = 2*i + 1
int right = 2 * i + 2; // right = 2*i + 2
// If left child is larger than root
if (left < n && arr[left] > arr[largest]) {
largest = left;
}
// If right child is larger than largest so far
if (right < n && arr[right] > arr[largest]) {
largest = right;
}
// If largest is not root
if (largest != i) {
std::swap(arr[i], arr[largest]);
heapify(arr, n, largest);
}
}
C++ Code Example for Heap Sort
The `heapSort` function combines these processes to sort an array efficiently:
void heapSort(std::vector<int>& arr) {
int n = arr.size();
// Build heap (rearrange array)
for (int i = n / 2 - 1; i >= 0; i--) {
heapify(arr, n, i);
}
// One by one extract an element from heap
for (int i = n - 1; i > 0; i--) {
std::swap(arr[0], arr[i]); // Move current root to end
heapify(arr, i, 0); // call max heapify on the reduced heap
}
}
Example Usage of Heap Sort in C++
Putting it all together, here’s a complete example of how to implement heap sort in a C++ program:
#include <iostream>
#include <vector>
void heapify(std::vector<int>& arr, int n, int i);
void heapSort(std::vector<int>& arr);
int main() {
std::vector<int> arr = {12, 11, 13, 5, 6, 7};
heapSort(arr);
std::cout << "Sorted array is: \n";
for (int i : arr) {
std::cout << i << " ";
}
return 0;
}
This program initializes an array, applies heap sorting, and prints the sorted output.
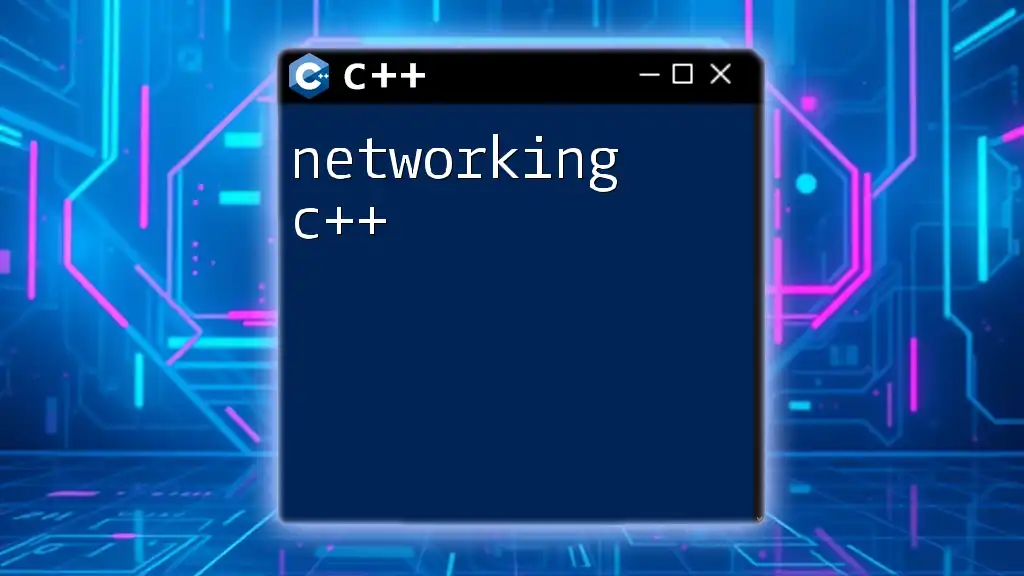
Detailed Explanation of the C++ Heap Sort Algorithm
Building the Heap
The heapify function ensures that the tree structure maintains the properties of a max-heap. The approach involves checking if the current node is less than its children; if it is, the values are swapped, and the function continues down the body of the heap. By starting the process from the last non-leaf node (calculated as `n/2 - 1`), the entire array is converted into a valid heap.
Sorting the Heap
Extracting each element involves several steps:
-
Swapping the Root: The root of the heap, which is the largest element, is swapped with the last unsorted element of the array.
-
Reducing Heap Size: The size of the heap is reduced by one, effectively ignoring the last sorted element.
-
Rebuilding Heap: The heap property is restored using the heapify function again, beginning from the root downwards.
By following these steps, the algorithm continuously tar pits the largest unsorted elements, sorting the entire array without the need for extra space.
Performance Analysis
Heap sort achieves O(n log n) time complexity in both average and worst-case scenarios, making it a reliable algorithm compared to others like quicksort, which can degrade to O(n²) in unfavorable conditions.
When choosing sorting algorithms, it is vital to analyze the specific use case and data characteristics since certain algorithms may perform better than others under different conditions. For instance, heap sort is particularly effective for datasets too large to fit in memory, where external sorting is required.
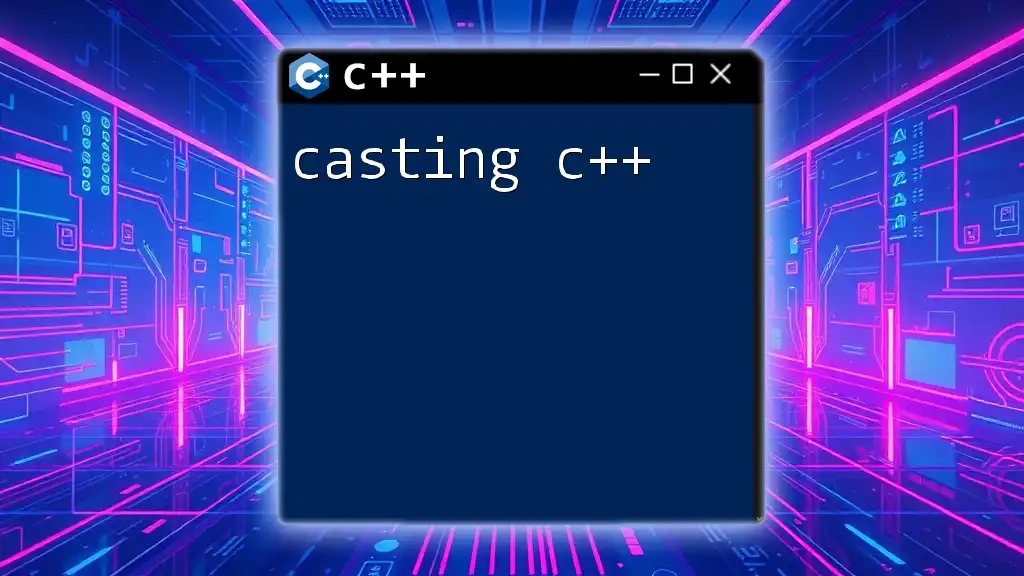
Common Pitfalls and Tips for Using Heap Sort in C++
Common Mistakes
Several common mistakes can arise when first implementing heap sort in C++:
-
Forgetting to Build the Heap: Neglecting to run the heapify process leads to suboptimal or incorrect sorting behaviors.
-
Errors in the Heapify Function: Misunderstanding the index calculations or failing to incorporate child comparisons correctly can cause heaps to necessitate further fixes.
-
Off-by-One Errors: Arrays indexed starting from zero can lead to miscalculations in children nodes if indices aren’t carefully handled.
Best Practices
To optimize your heap sort implementation:
-
Use `std::vector` for dynamic array sizes to leverage automatic memory management and avoid manual memory handling.
-
Write Clean Code: Include comments to explain the logic behind your decisions. Clear code helps both you and others understand algorithms better.
-
Testing: Validate your sorting algorithm with diverse datasets to ensure functionality across various input scenarios. Testing aids in identifying edge cases and optimizes performance.
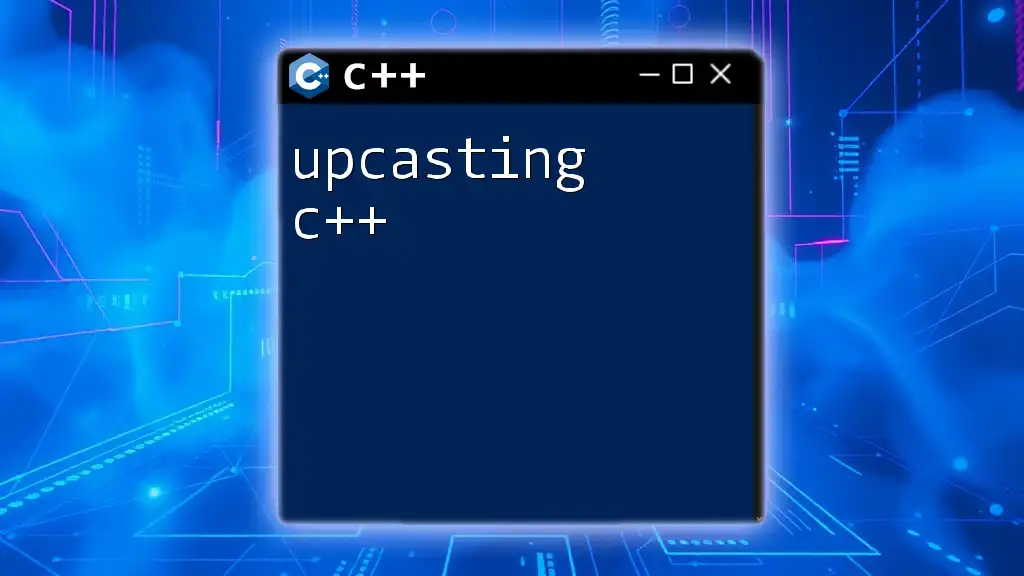
Conclusion
Heap sorting in C++ effectively combines the advantages of heap data structures with time-efficient sorting strategies. Understanding the mechanics behind the heap sort algorithm equips programmers to leverage its capabilities adeptly across diverse applications. Embrace the challenges of sorting in C++, not just through implementation but by continuous practice to improve proficiency and adaptability in real-world coding problems.
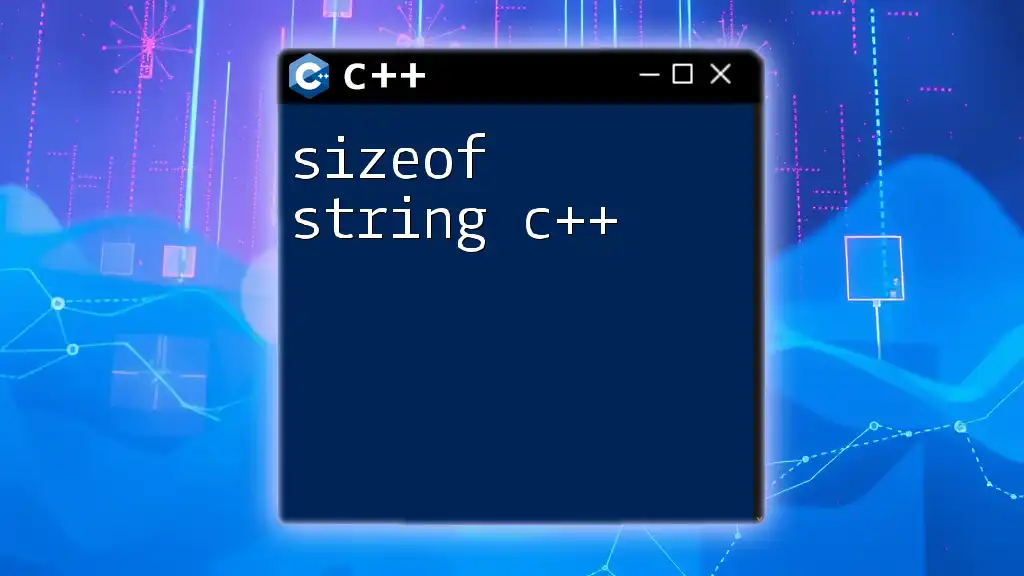
Additional Resources
For further exploration of heap sorting and other C++ programming concepts, consider consulting the official C++ documentation, enrolling in advanced programming courses, or reading proficiently crafted books on algorithms and data structures. Engaging with practical projects where heap sort can be deployed is equally beneficial for deepening your understanding and skill set.