Deep learning in C++ involves utilizing libraries and frameworks, such as TensorFlow or PyTorch, to create and train neural networks for various applications, exemplified by the following code snippet that demonstrates a simple neural network setup using the DNN module in OpenCV.
#include <opencv2/dnn.hpp>
#include <opencv2/core.hpp>
int main() {
// Load a pre-trained neural network from a file
cv::dnn::Net net = cv::dnn::readNetFromONNX("model.onnx");
// Create an input blob for the network
cv::Mat inputBlob = cv::dnn::blobFromImage(image);
// Set the input for the network
net.setInput(inputBlob);
// Perform forward pass to get predictions
cv::Mat output = net.forward();
return 0;
}
What is Deep Learning?
Deep learning is a subset of machine learning that uses algorithms inspired by the structure and function of the brain, known as artificial neural networks (ANNs). Its significance is underscored by its pervasive applications across various domains, such as image recognition, natural language processing, and autonomous driving. Through hierarchical learning, deep learning models can process vast amounts of data, discerning complex patterns that traditional algorithms might miss.
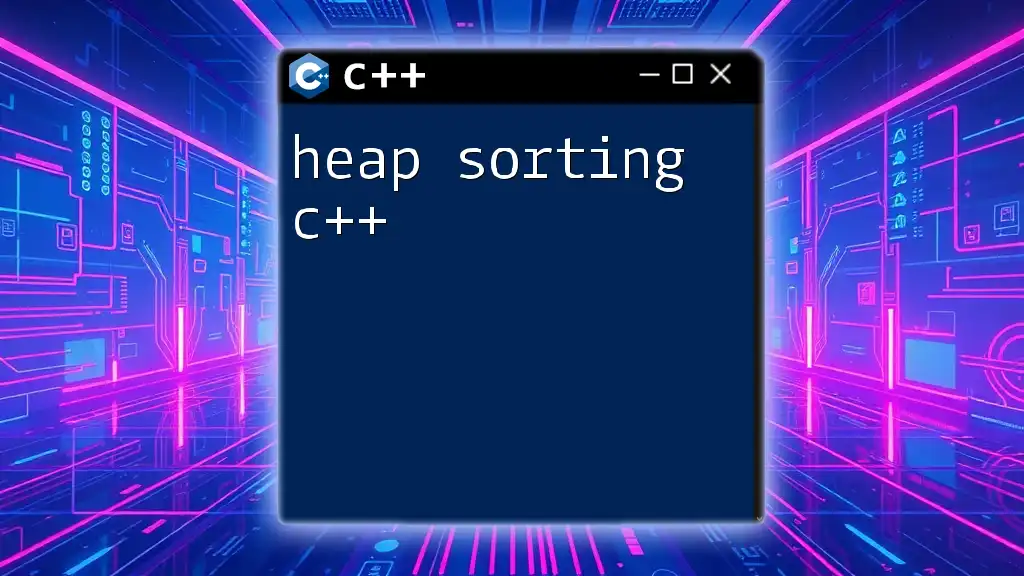
Why Use C++ for Deep Learning?
When it comes to deep learning C++, several critical benefits make this language a strong contender for developing high-performance models:
-
Performance Benefits: C++ is a compiled language, which means it translates code directly into machine language for faster execution. This efficiency is paramount in deep learning, where large datasets and complex computations are the norms.
-
Memory Management: C++ gives developers fine-grained control over resource management. Efficient memory usage is crucial for handling large tensors and arrays that are common in deep learning tasks.
-
Real-Time Processing: Many applications require real-time performance, like image or speech recognition. C++ excels in scenarios where latency must be minimized.
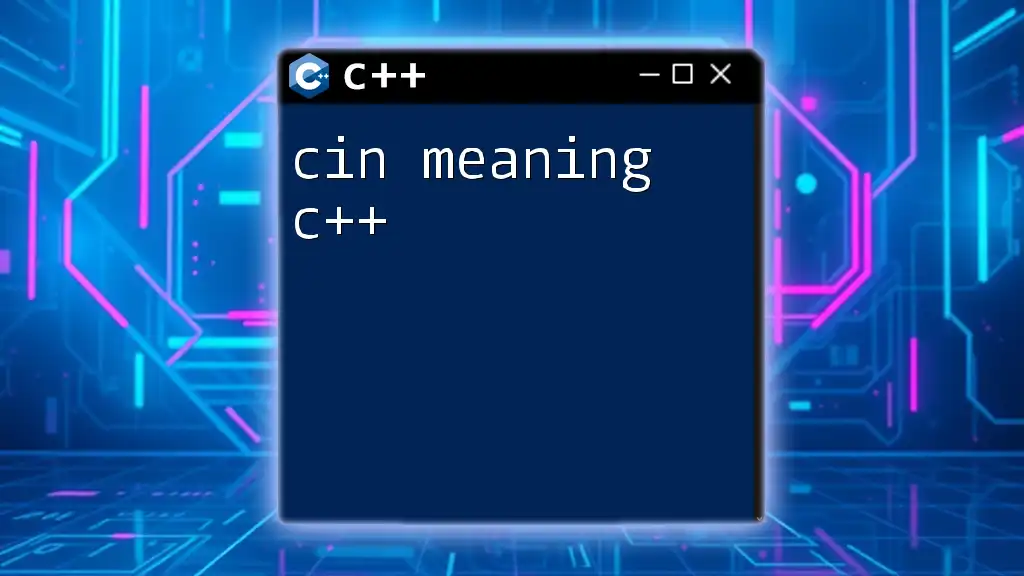
Setting Up Your C++ Environment for Deep Learning
Required Tools and Libraries
To capitalize on C++ for deep learning, several powerful libraries can streamline the development process:
- TensorFlow C++ API: This is ideal for those already familiar with TensorFlow but want to switch to C++ for better performance.
- Caffe: Known for its speed, especially in image processing tasks, making it a preferred choice in both academia and industry.
- MXNet: Offers scalable high-performance training, particularly in distributed systems.
For installing these libraries, follow their respective documentation for straightforward procedures.
Configuring Your Development Environment
Creating an effective development environment is crucial:
-
IDE Recommendations: Visual Studio, CLion, and Eclipse are robust choices, offering features such as debugging and integrated build systems that enhance coding efficiency.
-
Compiler Setup: Utilizing GCC or Clang will provide the best performance for compiling your C++ code, ensuring your deep learning applications run optimally.
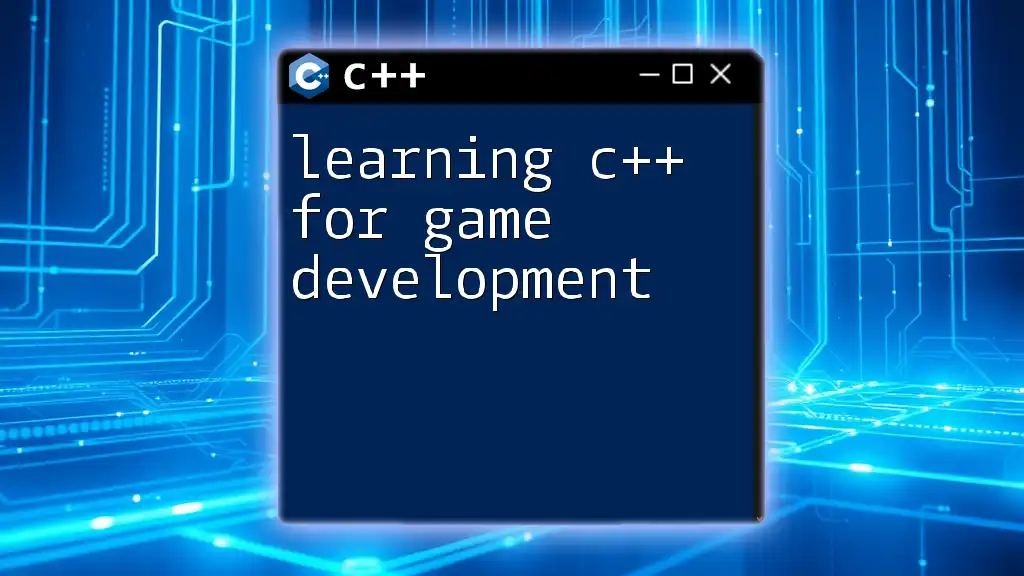
Core Concepts in Deep Learning
Neural Networks
At the heart of deep learning are neural networks. These models consist of interconnected neurons that simulate how the human brain operates. Each neuron processes input data, applies an activation function, and contributes to the final output.
Structure of a Neuron
A neuron comprises several essential components: inputs (features), weights (parameters), and the activation function. The weighted sum of inputs passed through an activation function determines the neuron's output.
Training Neural Networks in C++
Training involves adjusting weights based on error gradients calculated during backpropagation. This cyclic refinement allows the model to learn from its mistakes, gradually improving its predictions.
Example Code Snippet: Implementing a Simple Neural Network
Below is a basic implementation of a neuron in C++:
#include <iostream>
#include <vector>
class Neuron {
public:
float weights;
float bias;
float output;
Neuron(int inputSize) {
// Initialize weights and bias (randomized for this example)
weights = static_cast<float>(rand()) / RAND_MAX;
bias = static_cast<float>(rand()) / RAND_MAX;
}
float activate(const std::vector<float>& inputs) {
output = 0.0;
for (size_t i = 0; i < inputs.size(); ++i) {
output += inputs[i] * weights;
}
output += bias; // Add bias
return output > 0 ? output : 0; // ReLU activation
}
void updateWeights(float learningRate, float error) {
weights += learningRate * error * output; // Gradient descent step
}
};
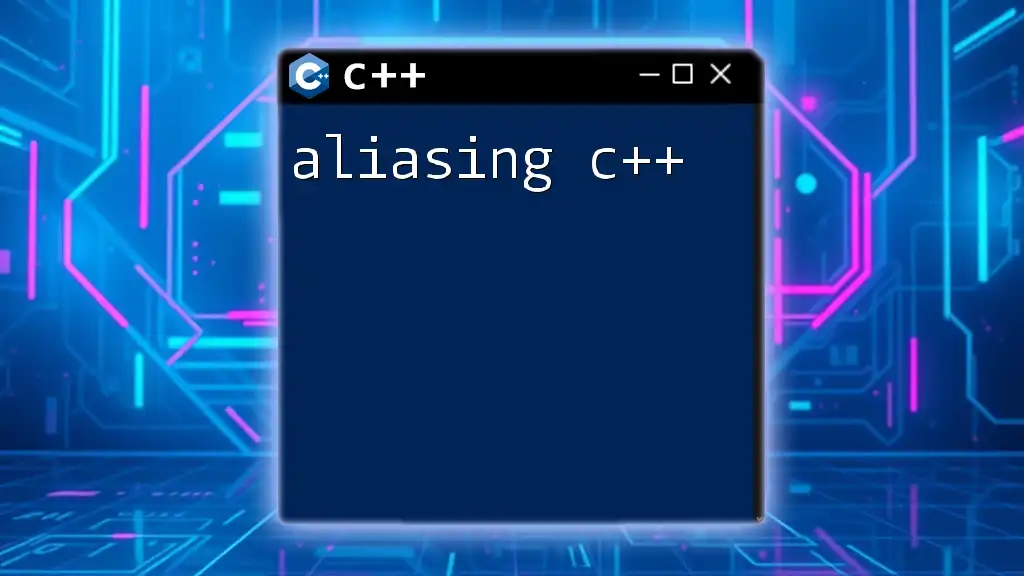
Implementing Deep Learning Algorithms
Convolutional Neural Networks (CNNs)
CNNs are pivotal in image processing. They consist of convolutional layers that capture spatial hierarchies in images through the use of filters.
Key Components of CNNs
Convolutional layers, pooling layers, and fully connected layers work together to learn representations at different levels of abstraction.
Example Code Snippet: Basic CNN Structure in C++
Here’s a simple structure for a convolutional layer:
class ConvLayer {
public:
void forwardPass(const std::vector<float>& input) {
// Implement forward logic here
}
void backwardPass(const std::vector<float>& outputGrad) {
// Implement backward logic here
}
};
Recurrent Neural Networks (RNNs)
RNNs are suitable for sequence data, making them a staple in natural language processing use cases. They differ from traditional neural networks due to their ability to maintain memory of past inputs.
Application in Natural Language Processing
RNNs can, for example, predict the next word in a sentence based on previous words, significantly improving the capabilities of language models.
Example Code Snippet: Simple RNN Cell in C++
Here's a basic structure for an RNN cell:
class RNNCell {
public:
float hiddenState;
void forward(const std::vector<float>& input) {
// Logic to compute hidden state
}
};
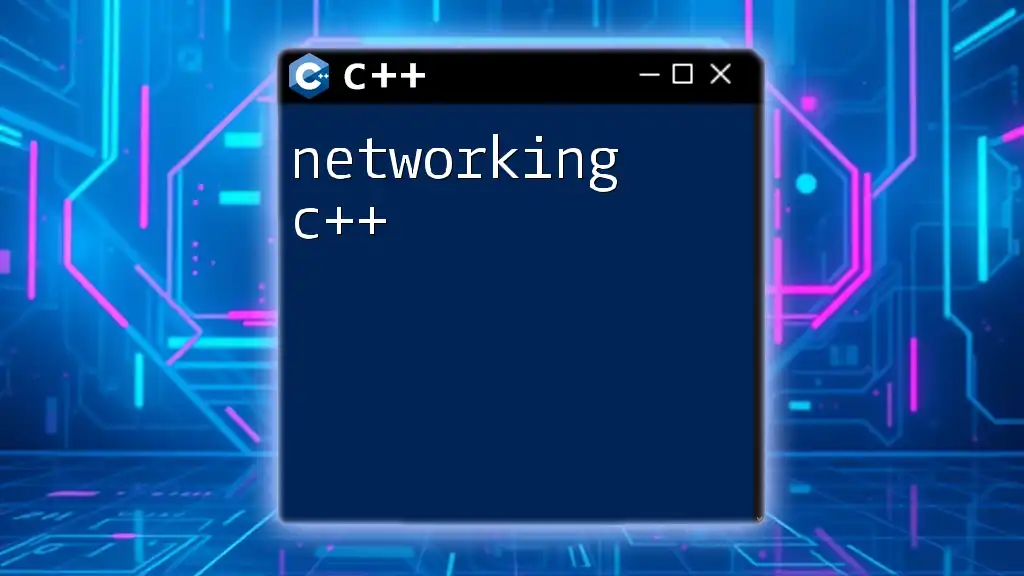
Libraries and Frameworks for Deep Learning in C++
Overview of Popular Deep Learning Frameworks
Deep learning frameworks dramatically simplify complex tasks:
- TensorFlow C++ API: This allows for leveraging TensorFlow's capabilities while programming in C++. It supports complex operations and neural network architectures.
- Caffe: Synonymous with performance, especially in training models for computer vision tasks.
- Pytorch C++: Combines the ease of Pytorch with C++ performance, making it an attractive option for research and applications.
Comparison of Libraries
Each library has unique strengths. TensorFlow is robust and widely-used, whereas Caffe shines in image-related tasks. Pytorch offers flexibility and dynamic computation, catering well to experimental applications.
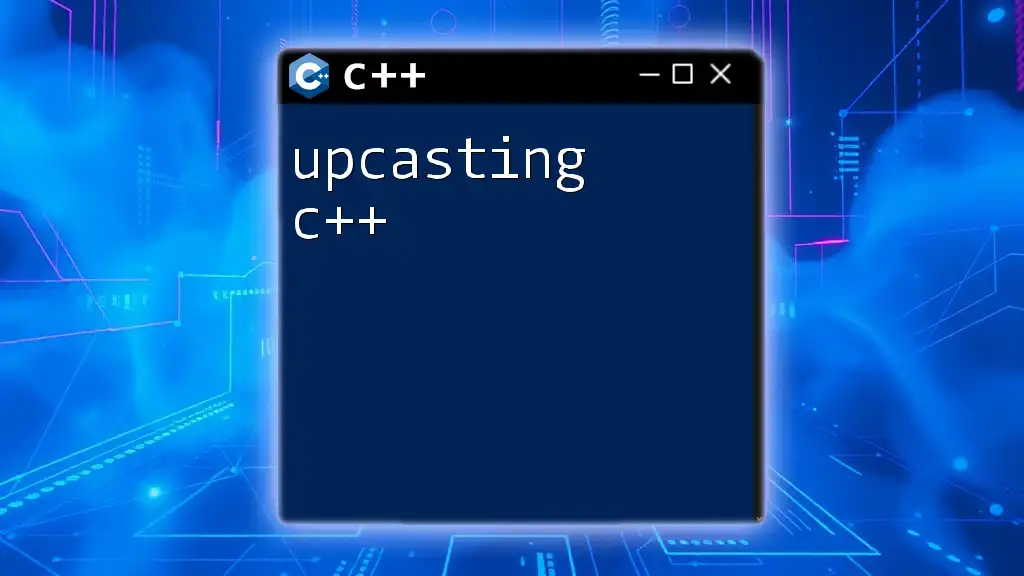
Practical Applications of Deep Learning with C++
Image Classification
Implementing CNNs for image classification is a compelling application. By training models on labeled datasets, businesses can automate tasks like content moderation.
Example Code Snippet: Training Loop
This snippet illustrates a basic training loop for a CNN:
for (int epoch = 0; epoch < numEpochs; ++epoch) {
for (auto& batch : trainingData) {
// Forward pass
auto predictions = model.forwardPass(batch.inputs);
// Calculate loss
float loss = computeLoss(predictions, batch.labels);
// Backward pass
model.backwardPass(loss);
}
}
Natural Language Processing
Building RNNs for text generation showcases C++'s strength in handling sequence data. Such models can learn contexts and create coherent sentences or generate programming code.
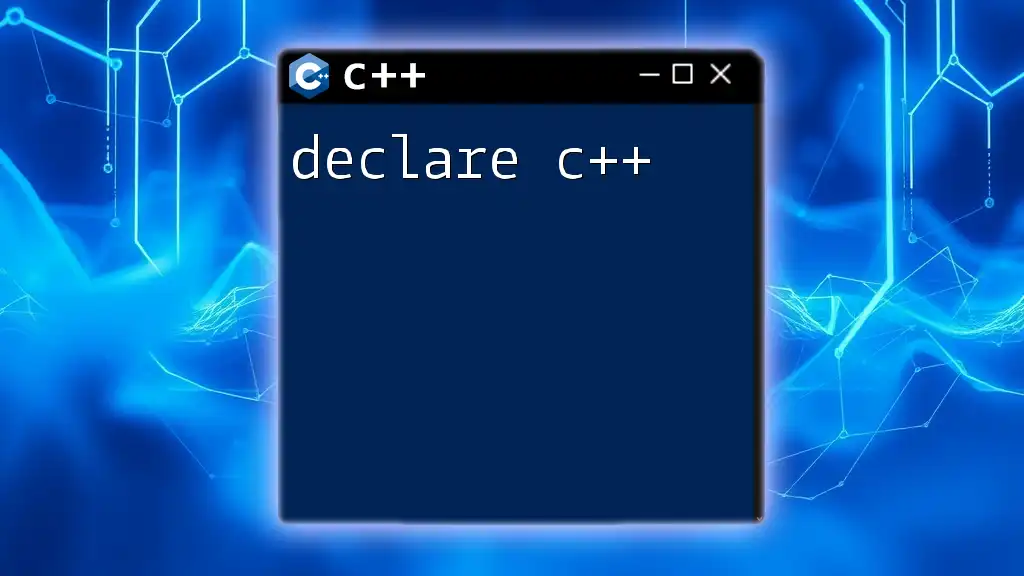
Best Practices for Deep Learning in C++
Optimizing Performance
Profiling and benchmarking your deep learning model is essential to identify bottlenecks. C++ offers numerous profiling tools that can aid in enhancing performance.
Testing and Debugging
Common pitfalls include memory leaks and incorrect tensor sizes. Implementing unit testing allows for quicker identification and resolution of errors, fostering a more robust development process.
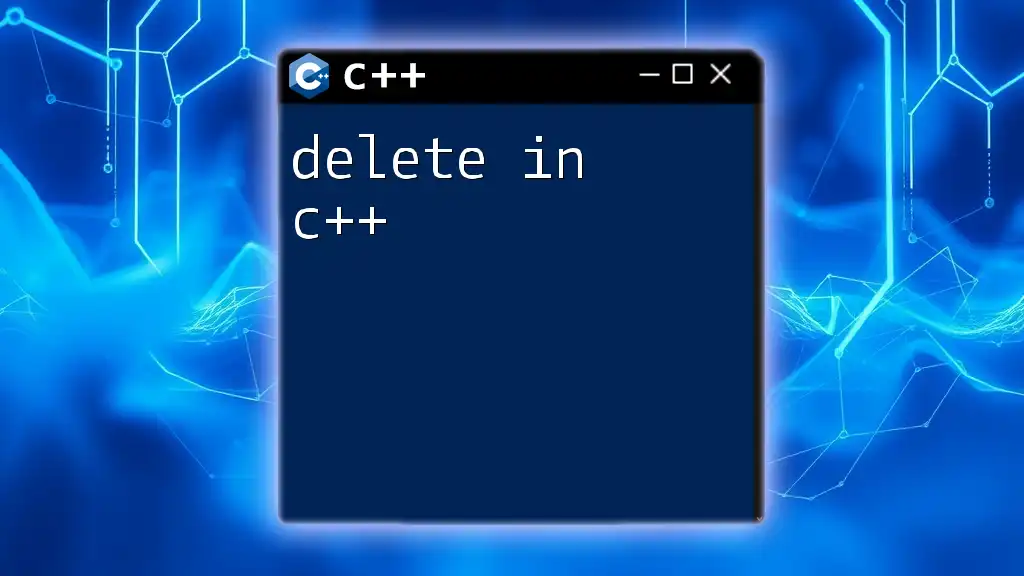
Conclusion
The intersection of deep learning C++ has significant implications for securing impressive performance and managing resources effectively in machine learning projects. As the landscape of AI evolves, the role of C++ in it remains imperative. With a deep understanding of the core concepts and practical implementation strategies, you are better equipped to step into the world of deep learning with confidence.
Resources for Further Learning
To deepen your knowledge, consider exploring recommended books, reputable online courses, and join communities focused on C++ machine learning. Engaging with fellow enthusiasts and professionals can provide invaluable insights and support as you embark on your journey in deep learning.