Learning C++ is a valuable investment for anyone interested in programming, as it enhances your understanding of fundamental concepts and empowers you to create performance-oriented applications.
Here's a simple C++ code snippet demonstrating a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Why C++?
Versatility of C++
C++ is a highly versatile programming language that finds applications across various domains, making it an essential skill for software developers. Here are some areas where C++ shines:
-
Game Development: C++ is a dominant language in the gaming industry due to its ability to handle complex graphics efficiently. Game engines like Unreal Engine leverage C++ for performance-intensive aspects, allowing developers to create immersive gaming experiences.
-
Systems Programming: Operating systems, file systems, and other system-level applications are typically written in C++. Its low-level capabilities allow developers to directly interact with hardware, offering more control over system resources.
-
Embedded Systems: In devices ranging from microwave ovens to complex medical equipment, C++ is used to develop software that requires efficiency and real-time performance, important for embedded programming.
-
Application Development: Many desktop and mobile applications rely on C++ for their performance. Applications like Adobe Photoshop and various high-frequency trading platforms utilize C++ for critical performance requirements.
Performance and Efficiency
When it comes to performance and efficiency, C++ has distinct advantages.
- Memory Management: C++ offers manual memory management, allowing developers to allocate and deallocate memory as needed. This can lead to more efficient use of resources compared to languages that use automatic garbage collection.
For example, consider the following code snippet, which demonstrates manual memory allocation:
#include <iostream>
int main() {
int* ptr = new int(5); // Manual memory allocation
std::cout << *ptr << std::endl; // Output: 5
delete ptr; // Manual memory deallocation
return 0;
}
With manual memory management, developers can optimize performance-critical applications, although it comes with increased complexity.
- Speed of Execution: C++ is often faster than many other programming languages due to its static type system and low-level capabilities, which allow direct interaction with hardware. This performance advantage makes it the language of choice for applications where speed is critical, such as video games or high-frequency trading systems.

Who Should Learn C++?
Career Opportunities
In today's job market, the demand for C++ programmers is significant. Many industries seek developers who can leverage C++ for robust and efficient applications. Here are some roles where C++ expertise is particularly valuable:
- Software Developer: Creating applications, tools, and systems that require reliable performance.
- Game Developer: Designing and implementing game mechanics and graphics.
- Systems Engineer: Developing and maintaining operating systems and core software.
C++ skills can also open doors to roles in sectors such as finance, telecommunications, and robotics, underscoring its versatility.
Pathway for Beginners
For those new to programming, learning C++ can provide a solid foundation for understanding other languages. It teaches essential concepts such as:
- Data Types and Structures: Understanding how data is stored and manipulated.
- Control Structures: Learning how to create programs that can make decisions and repeat actions.
By mastering C++, beginners can easily transition to languages like C#, Java, or Python, enhancing their marketability in the tech industry.

Getting Started with C++
Learning Resources
When embarking on your C++ learning journey, the abundance of resources can be overwhelming. Consider the following avenues for effective learning:
-
Books: Titles like "C++ Primer" and "Effective C++" are excellent for those starting out and looking to deepen their understanding.
-
Online Courses: Platforms like Coursera, Udemy, and Codecademy offer comprehensive C++ programming courses designed for learners of all levels.
-
YouTube Channels: Channels like The Cherno and freeCodeCamp feature in-depth tutorials and practical exercises to help reinforce your knowledge.
Essential Tools and Environment
To begin coding in C++, you will need an understanding of compilers and integrated development environments (IDEs). Here are some popular tools:
-
Compilers:
- GCC: The GNU Compiler Collection is widely used and open-source.
- Clang: Known for its fast compilation and modern features.
- Visual Studio: A popular IDE with a built-in compiler for Windows.
-
IDEs:
- Code::Blocks: An easy-to-use IDE ideal for beginners.
- CLion: A powerful IDE by JetBrains that supports various tools for C++ development.

Basics of C++
Syntax and Structure
C++ has a unique syntax that can initially seem challenging. Understanding its structure is essential for effective coding.
- Basic Syntax: Every C++ program starts with include statements and a main function. For example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this classic "Hello, World!" program, we include the iostream library to handle input and output, define the main function, and print the message to the console.
Fundamental Concepts
-
Data Types and Variables: C++ offers various data types like int, char, float, and double, which define what kind of data can be stored in a variable.
-
Control Structures: These structures allow programmers to dictate the flow of the program. For instance, if statements, loops (for, while), and switch cases help manage decisions and repetition in code.
-
Functions and Scope: Functions are blocks of code designed to perform specific tasks. Understanding how to define and call functions, as well as the concept of variable scope, is crucial in C++ programming.

Advanced C++ Concepts
Object-Oriented Programming (OOP)
C++ is well-known for its support of Object-Oriented Programming principles. The four core principles of OOP are:
-
Encapsulation: Bundling data and methods that operate on that data within one unit (an object).
-
Inheritance: Allowing a new class to inherit properties and behaviors from an existing class, promoting code reuse.
-
Polymorphism: Enabling a single function or operator to work in different contexts or types.
To illustrate the concept, consider the creation of a class:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Here, we define a Dog class with a method called `bark`. This encapsulation allows us to represent a dog and its behaviors cleanly.
Templates and Standard Template Library (STL)
C++ supports template programming, which allows developers to write generic and reusable code.
-
Understanding Templates: Templates enable functions and classes to operate with any data type. For example, a function that sorts an array can be written as a template to sort both integers and strings.
-
Using STL: The Standard Template Library (STL) provides a collection of pre-built classes and functions, including data structures like vectors, lists, and maps, as well as algorithms for sorting and searching.
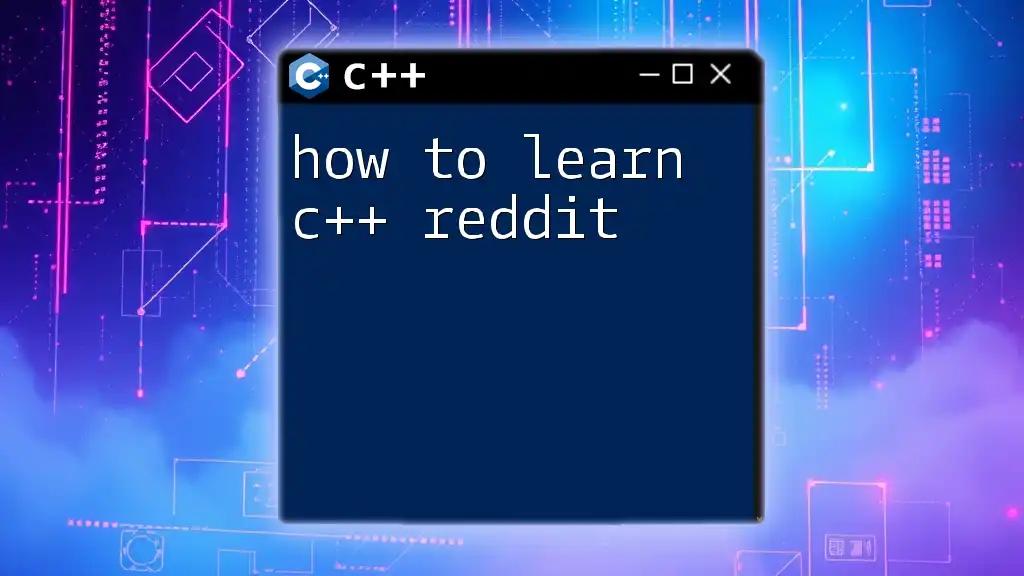
Challenges and Considerations
Learning Curve
While C++ offers many advantages, its complexity can pose challenges for beginners. Concepts like pointers, manual memory management, and deep understanding of classes may take time to master. However, these hurdles are surmountable with persistent practice and the right resources.
Alternatives to C++
For those still unsure about whether to dive into C++, it's worth considering alternatives.
-
Python: Known for its simplicity, Python might be a better choice for beginners looking for quick results in fields like data analysis or web development.
-
Java: With its readability and widespread use in enterprise environments, Java can also serve as an effective first language.
-
Rust: A newer language that emphasizes safety and performance, Rust might appeal to those interested in low-level programming without some of the complexities of C++.
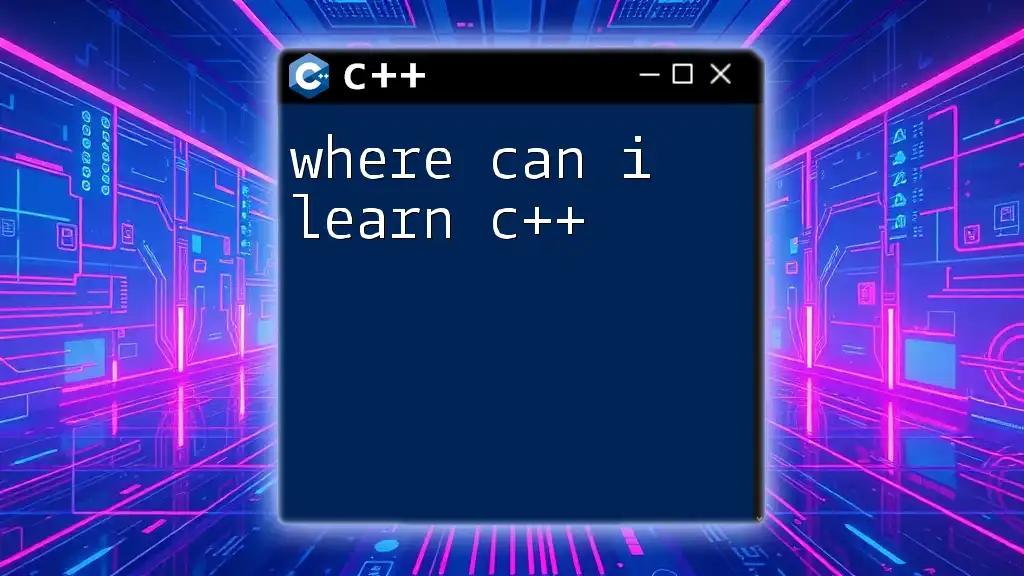
Conclusion
The decision of whether to learn C++ boils down to personal interests and career goals. C++ is a powerful language that unlocks numerous opportunities in various industries, particularly in performance-critical areas like game development and systems programming.
By mastering C++, you lay the groundwork for understanding more advanced programming principles and languages, making it a solid investment in your programming journey.
As you consider your next steps, evaluate how C++ aligns with your career aspirations and programming interests. Whether you're looking for a comprehensive online course or useful resources, the C++ community offers many avenues to support your learning. Dive in, and you'll discover a world of programming possibilities awaits!
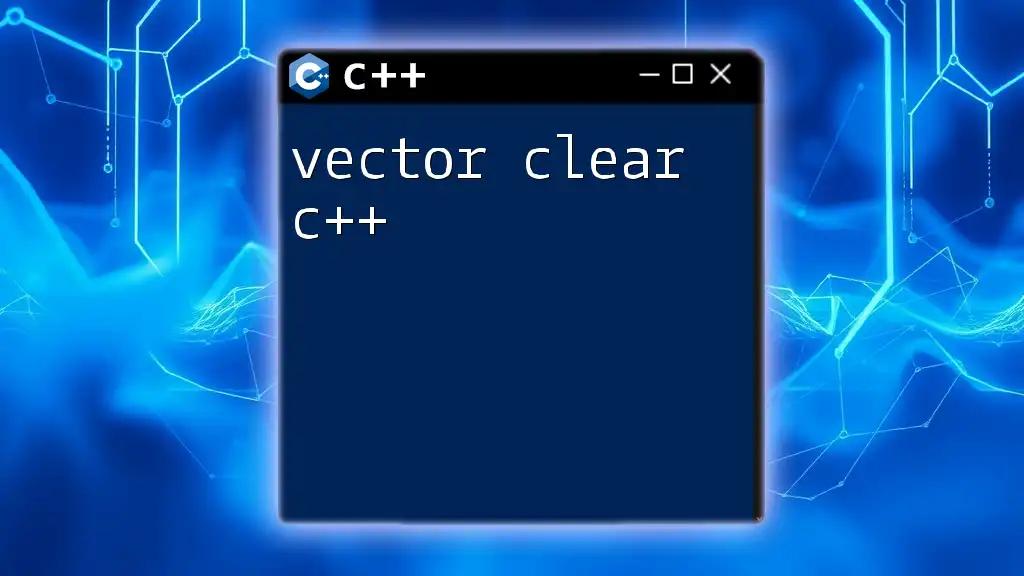
FAQ Section
Is C++ still relevant today?
Absolutely! C++ remains one of the most widely used programming languages for performance-critical applications and is crucial for systems-level coding.
How long does it take to learn C++?
The time it takes can vary widely based on prior experience, but with consistent study and practice, many learners can expect to achieve a strong foundational understanding within a few months.
Can I learn C++ on my own?
Yes, many people successfully learn C++ through self-study. Utilize available resources like books, online courses, and tutorials to guide your journey.

Additional Resources
For further reading and community support, explore forums like Stack Overflow, Reddit’s r/cpp, and other coding communities dedicated to C++. Engaging with others can provide invaluable insights and assistance as you learn.
Also, consider interactive learning platforms like Codecademy and LeetCode for hands-on practice in coding challenges using C++. The more you practice, the more fluent you will become in C++.